You can copy a list in Python using the copy() method, slicing, the list() method, and a list comprehension. The copy() method is the most direct way of copying a Python list.
When you’re working with a list in Python, you may encounter a situation where you want to create a copy of that list.
For instance, you may be creating a program that, using last year’s student roster, generates an updated roster for the new school year. This program should preserve the old class roster.
How to Copy a List in Python
There are four methods you can use to copy a list in Python:
- Using the slicing syntax
- Using the copy() method
- Using the list() method
- Declaring a list comprehension
This tutorial will discuss, using examples, how to employ copy(), slicing, list comprehension, and list() to create a copy of a list in Python.
You can use the equals sign to create a copy of a list. But, the new list will be linked to the old one. This means that if you change the new list the old one will be changed too. The new list refers to the same object as the old list.
Copy List Python: copy() Method
The Python copy() method creates a copy of an existing list. The copy() method is added to the end of a list object and so it does not accept any parameters. copy() returns a new list.
Python includes a built-in function to support creating a shallow copy of a list: copy(). You can use the copy() method to replicate a list and leave the original list unchanged.
The copy() method takes in no parameters. You add it to the end of a list name. Here’s the syntax for creating a new object Python copy() method:
new_list = old_list.copy()
Python copy() Method Example
Let’s walk through an example to show how you can use the copy() method. Say that we operate a fruit stand and are looking to update our product list to reflect the new fruits in season for summer.
To do so, we want to create a new list of products based on the old one. We want to save our old one for later use. This will allow us to keep track of what products we sold last season in an old list. We’ll also be able to keep track of what we are selling this season in a new list.
We can use the copy() method to duplicate a list and accomplish this task. Here’s an example of using copy() to create a copy of our list of fruit product offerings:
spring_fruits = ['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry'] summer_fruits = spring_fruits.copy() print(summer_fruits)
Here’s the result of our program:
['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry']
On the first line of our code, we define a Python variable called spring_fruits. This variable keeps track of the fruits we sold in spring.
Then, we use the copy() method to create a new list called summer_fruits which contains all the items in the spring_fruits array. Finally, we print the summer_fruits array to the console. As you can see, our summer_fruits list contains the same values as our original list—the spring_fruits list.
Now that we have a copy of our list, we can modify it to reflect our new fruit offerings. We can do so using the append() and remove() methods.
Copy List Python: Slicing
Slicing is a technique in Python that you can use to retrieve items from a list. Using the slicing technique, you can retrieve individual items, multiple items, or all items stored within a list. If you’re interested in learning more about slicing, read our guide to Python list methods.
Let’s use the example above to show how we can use slicing to copy a list. Say that we are operating a fruit stand. We want to create a new list for summer products that contains all the spring products that we sold. We could do so using slice cloning.
Here’s the code we would use to create a copy of our list using slice cloning:
spring_fruits = ['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry'] summer_fruits = spring_fruits[:] print(summer_fruits)
Our code returns:
['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry']
Our code is similar to the example we used above to demonstrate the copy() method. The difference is that, rather than using copy(), we use Python’s cloning notation.
The cloning notation is a colon enclosed within square brackets ([:]), as you can see in the example above. This method creates a copy of the old list.
Python Copy List: list() Method
You can also use the list() function to create a copy of a list in Python. The list() method of copying takes in one parameter: the object(s) you want to convert into a list. Here’s the syntax for the list() method:
list(list_name)
Let’s use our fruit stand example to illustrate this method in action. Here’s an example of using list() to create a list of summer fruits based on our list of spring fruits:
spring_fruits = ['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry'] summer_fruits = list(spring_fruits) print(summer_fruits)
Our code returns the following new list:
['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry']
As you can see, assigning list(spring_fruits) to summer_fruits in our code created a copy of the spring_fruits list and saved it as summer_fruits. We then printed our new list to the console using the print() function.
Python Copy List: List Comprehension
You can concisely create lists using the Python list comprehension method. This method uses a for…in expression.
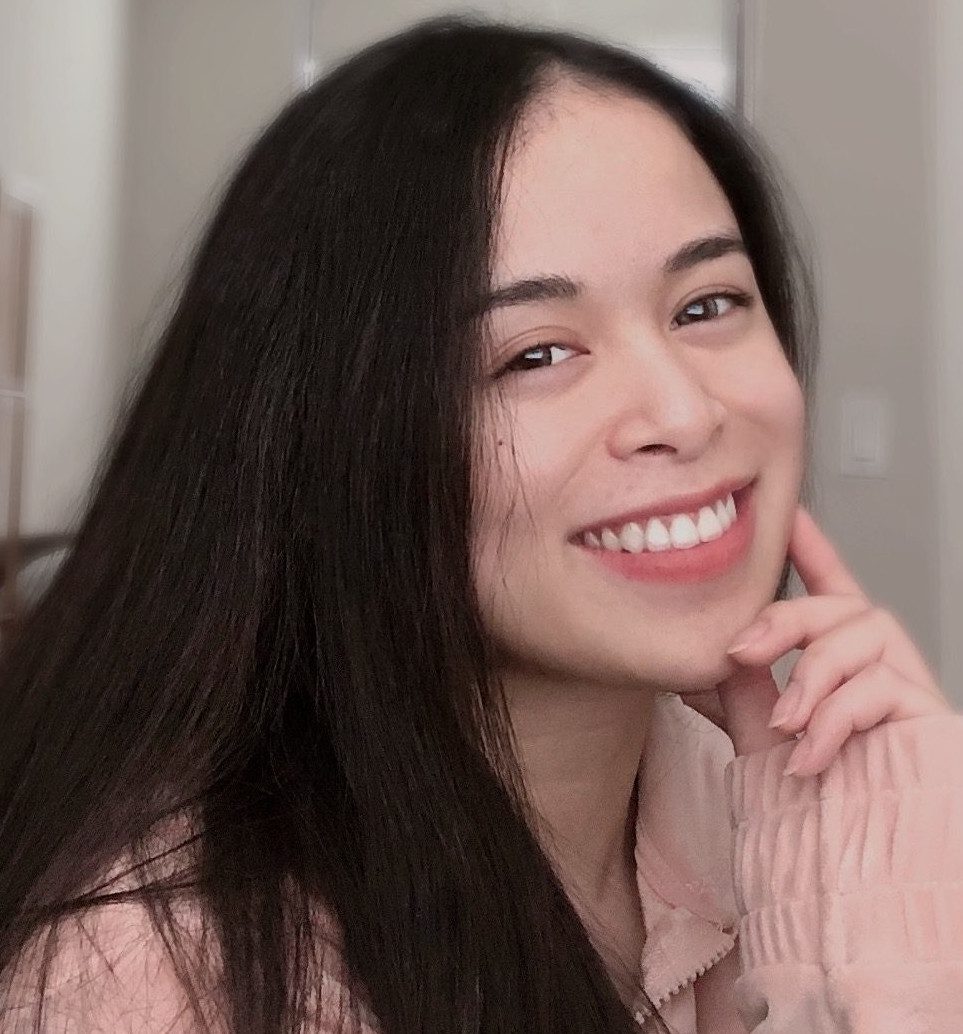
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
List comprehension works by iterating through all items in an existing list using a for loop and populating a new list with those items.
Here’s the syntax for list comprehension in Python:
new_list = [i for i in old_list]
We can use our fruit stand example to illustrate this method in action. Here’s an example of using list comprehension to create a new list of summer fruits based on our list of spring fruits:
spring_fruits = ['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry'] summer_fruits = [i for i in spring_fruits] print(summer_fruits)
Our code uses list comprehension to create a new list, called summer_fruits, and returns the following:
['Apricot', 'Avocado', 'Kiwi', 'Grapefruit', 'Cherry', 'Strawberry']
As you can see, our program has created a summer_fruits list that contains all the values that are in our original spring_fruits list.
Conclusion
This tutorial looked at four approaches that you can use to create a copy of a list in Python. We discussed how to use the copy() method, the slice cloning technique, the list() function, and the list comprehension method.
You’re now equipped with the knowledge you need to create a copy of a list in Python! If you’re looking for learning resources or courses to help you learn Python, check out our How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.