A Python comment is a line of text in a program that is not executed by the interpreter. Comments are used during debugging to identify issues and to explain code. Comments start with a hash character (#).
How to Comment in Python
Comments denote notes or code you do not want Python to run. For example, say you are writing a complex procedure over a period of hours. You may want to write a few comments to keep track of what each line of code does so that you don’t lose track.
Writing comments is useful for a number of reasons. If you are working with a big Python program, commenting can help you keep track of what is going on. Commenting also helps team members ensure everyone understands each other’s work on a collaborative project.
Every developer eventually encounters a situation where they must include comments when writing code.
But how do you comment on code in Python? Like every other programming language, Python supports commenting. In this guide, we’re going to talk about writing comments in Python.
What is a Python Comment?
A Python comment is a line of text that appears in a program but is not executed by the program. You can declare a comment using a hashtag (#). Comments can appear on a new line or at the end of an existing line of code.
Comments are used to explain how code works and for testing purposes.
Here’s an example a single line comment in Python:
# This is a demo comment
You can see the # hash mark denotes our comment. We have separated our comment text and the # sign with a space. This is not necessary but it makes each comment easier to read.
This line is ignored by the compiler when we run our code. Comments are to be read by humans. They are not for a program to execute.
Comments should be added at the same indent level as the code to which the comment is referring. For example, say you are writing about a line of code within an if statement. You would indent your comment the same way you did with your code.
Python Comments Example
Let’s say that you are writing a for loop, and you want to remind yourself how it works. You may want to write a few comments. When you come back to look at your code later, you’ll be able to read your notes.
In a Python for loop, you may have comments that look like this:
# Define students variable as an array of strings students = ["Nathan", "Alyssa", "Matthew", "Malcolm", "Alexis", "Katie"] # Loop through each item in the students array and print out each name for s in students: print("Student Name: ", s)
Our comments help us understand the purpose of our code and keep track of what is going on.
Let’s use an example to illustrate the indentation structure of comments in Python. In the below example, our program loops through two Python arrays and gives each student a pass or fail for their test. Passes and fails are determined based on a students’ numerical score on their test:
# Define an array with student names students = ["Nathan", "Alyssa", "Matthew", "Malcolm", "Alexis", "Katie"] # Define an array with student grades numericalGrades = [55, 72, 64, 86, 86, 59] # Loop through every student in "students" array for s in range(0, len(students)): # Check if the student's numerical grade is equal to or above 60 if numericalGrades[s] >= 60: # Print a message saying the student has passed their test print(students[s], "passed their test.") else: # Print a message saying the student has failed their test print(students[s], "failed their test.")
This program takes a list of students and their grades. Then, our program loops through the list of students. We check if each student has passed or failed their test.
In the above example, you can see that we comment on the same indentation level as the line to which we are referring. When we are discussing our Python if statement, for example, we have indented our comment as well as our code.
By writing these single line comments, our program is more readable both for the author of the code. Our program is also easier for anyone else who may look at our program to read.
Python Inline Comments
Python Inline comments are written on the same line as a statement, after the code has been written. Inline comments should explain the purpose of a line of code or how it works.
Here is the syntax for an inline comment:
print(students) # Print the variable "students"
Inline comments are useful if you need to explain complicated parts of the code. They are also useful if you think you may forget how a specific line of code works in the future.
Inline comments are also useful if you are working in a team and need to make sure everyone understands a specific line.
Unlike other types of comments, only use inline comments occasionally. This is because too many inline comments makes a program difficult to read.
Python Multiline Comments
Python multiline comments, or Python block comments, explain code that is either more complicated or whose purpose is not immediately obvious. You may use a block comment to explain a unique function that uses an external library, for example.
Block comments are multi-line comments in Python and apply to some or all of the code below them. Block comments, like any other comments, are also indented at the same level as the code the comment is discussing.
Multiline Comment Example
Here is an example of a multiline string that explains a Python sort() function:
# The sortStudents function will parse arguments via the "student" variable. The # function simply returns the length of each student's name. We reference the sortStudents # function in the sort() function later in the code, which iterates over each item in the # "students" array and executes our sortStudents function. def sortStudents(student): return len(student) students = ["Nathan", "Alyssa", "Matthew", "Malcolm", "Alexis", "Katie"] students.sort(key=sortStudents)
Our comment spans multiple lines and describes the purpose of the code written below it.
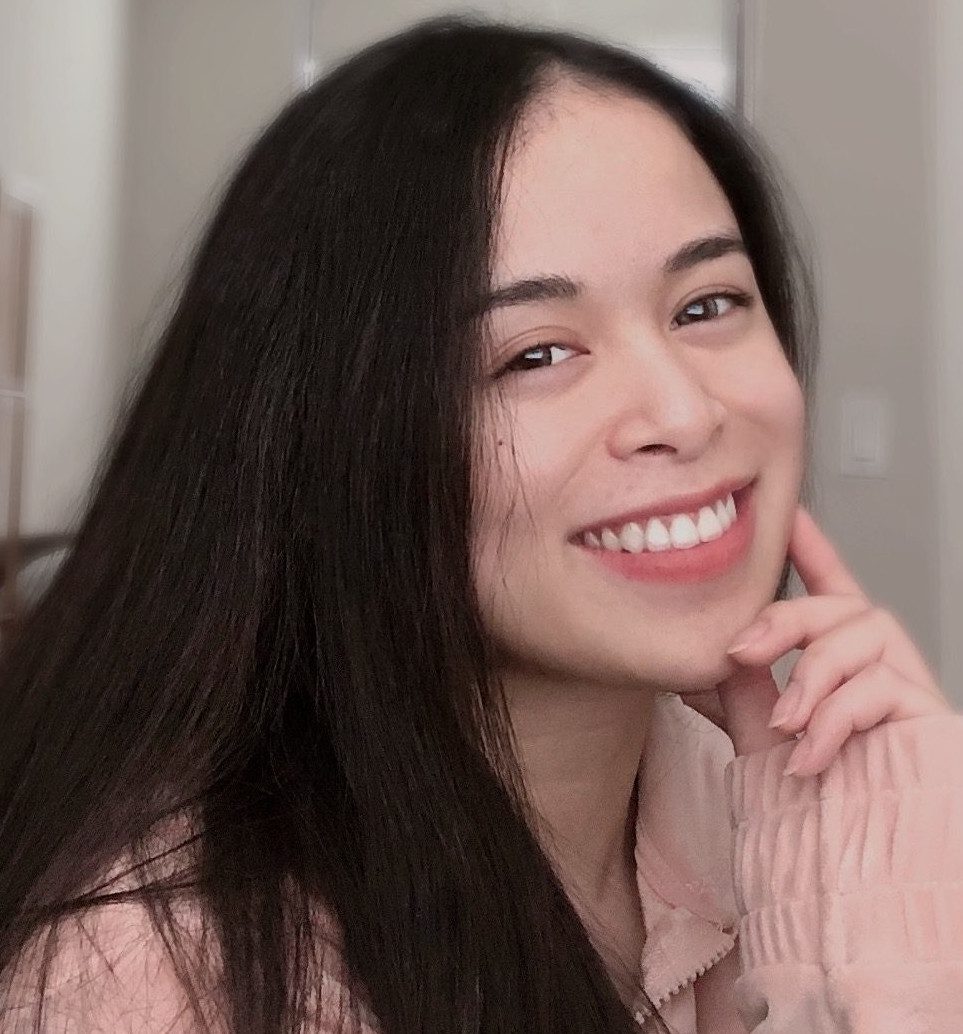
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
While some developers may understand this code, other programmers may be confused about how it works or why it has been written. Using a block comment is a great way to explain an entire procedure. Block comments are useful for explaining any other code that whose purpose is not immediately obvious to a developer.
Conclusion
That’s how you comment in Python! Using comments, you are able to make your programs more readable for everyone, including yourself.
Well-written comments can help you keep track of your code as you go. They allow you to maintain a record that can be referred back to later if you are struggling to understand your code later on.
Are you up for a challenge? Write a block comment at the top of one of your programs. This comment should include:
- Your name
- When you created the file
- The name of the file
- The purpose of the file
Try to run your code. If your program does not run because of the comment, you have made an error. If your program runs as normal, congratulations! You’ve written a comment successfully.
In this tutorial, we have explored how to write comments, inline comments, and block comments in Python. We also discussed how some developers write comments for testing. Now you can write comments like a Python expert!
Looking to master the Python programming language? For support on the top Python courses, books, and learning resources, check out our comprehensive How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.