Today we’re going to be talking about classes. No, we don’t mean school classes.
In programming, classes have their own definition. A class is a blueprint created by a coder for an object. It defines the data that can be stored in an object and the methods which can be performed on that particular object.
Python is an object-oriented programming language. This means that it uses a pattern of development where objects and classes are used to store data.
The object-oriented approach helps reduce repetition in your code, which makes it easier to read and maintain your code. Classes and objects are a part of many programming languages, such as Java, Python, and C++.
In this guide, we’re going to discuss what classes are, how they work, and how you can declare one in Python. We’ll walk through a few examples to help you along the way.
What is a Class?
A class is a blueprint that you use to define an object.
Imagine that you are looking at a blueprint for a house. That blueprint will outline where the doors will go, where windows will be placed, and so on. A class in programming is similar. A class provides a blueprint on the data that can be stored in an object.
Classes are defined using the class
keyword. Let’s get started by defining a class called Player which has two functions:
class Player:
""" This is a class for a player """ name = "Luke" def wield_sword(self): print("You have wielded your sword.") def drink_health_potion(self): print("You have consumed a health potion.")
This class represents a player in a video game. It contains both attributes and methods. Attributes are often called instance variables when they are inside a class.
One method allows you to wield a sword and the other method allows you to drink a health potion. The class contains one class variable: name. This stores the default name for our player.
Notice that we’re using the word self
a few times in this code. Self is what it sounds like: a representation of the class itself. Self contains all of the values that are stored within a particular class, so you can access them all inside your methods.
When we run our code, nothing happens. What’s going on? Remember how we said that a class was a blueprint? That’s the reason nothing is happening. We’ve got to do something with our blueprint in order for it to become useful.
What is an Object?
An object is a single instance of a class. It is defined using the blueprint created by a class.
Let’s create an object for a new player in our game, Luke:
luke = Player()
We’ve just created an object called Luke. Our object can now access all of the data that is stored within our class, as well as the methods associated with our class:
luke = Player() print(luke.name) luke.wield_sword()
Our code returns:
Luke
You have wielded your sword.
In our code, we’ve printed out the value of the name
attribute in our Luke object. To access the attributes in a class, you must state the name of the class, followed by a dot, then the instance attribute that you want to access. This is often called dot notation
.
By default, the value of name
was set to Luke. We’ll talk about how to change this in a minute.
We’ve also called our wield_sword()
class. When we called this class, the contents of the wield_sword()
method inside our Player class were executed. That caused a message to be printed to the console stating” “You have wielded your sword.”
You can declare as many objects of a class as you’d like: that’s why classes are so powerful. You can reuse the same methods and default values that are stored within a class across multiple different objects.
Class Constructors
Constructors allow you to initialize data inside a class. A constructor method is executed immediately after you declare an object of a particular class.
The name for a constructor is __init__()
. You may be used to seeing functions with double underscores (__) in your code. These functions are called special functions because they have a predefined purpose. In this case, the purpose of __init__
is to initialize our class.
Update the Player class that we created earlier to include this method at the top of the class:
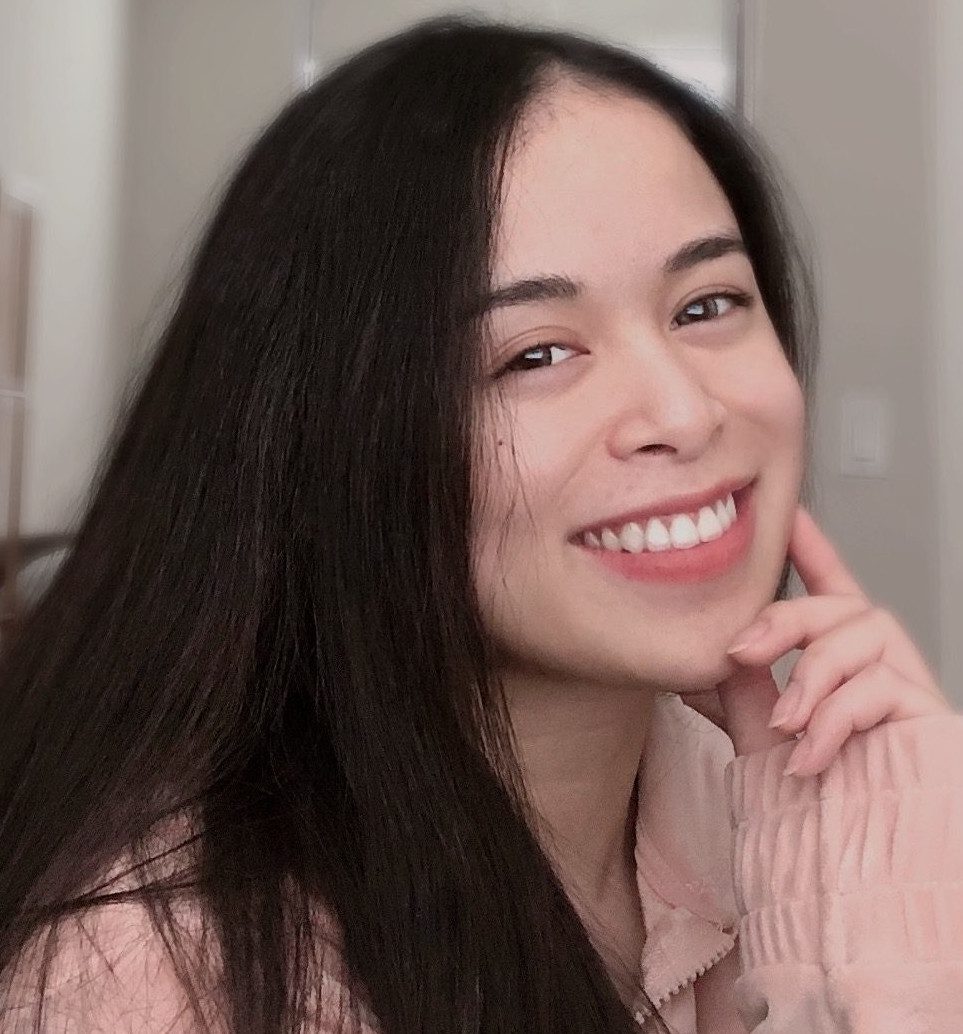
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
def __init__(self): print("Your character has been created.")
This method must come before any variables or methods are defined in your class. Let’s run our main code from earlier again:
luke = Player() print(luke.name) luke.wield_sword()
Our code returns:
Your character has been created.
Luke
You have wielded your sword.
We didn’t call the __init__
method in our main program but it ran anyway. That’s because constructors are automatically executed when you create an object of a class.
Earlier, we assigned the value of the name
variable in our class to Luke. While this is useful if we want to call our player Luke, it’s not practical if players can have different names.
That’s where constructors come in. Let’s create a constructor that allows us to assign a name to our player:
class Player: def __init__(self, name): self.name = name
This code allows us to pass a parameter into each Player object. This parameter will represent the name of the player. We’ll then create a method that tells us our player’s name:
class Player: def __init__(self, name): self.name = name def get_name(self): print("Your name is " + self.name)
Let’s create a new instance of our Player class for a player named Leah:
leah = Player("Leah") leah.get_name()
Our code returns:
Your name is Leah.
We’ve assigned the value of the name
variable in our object to Leah. This means that wherever we reference it in our class, its value is Leah. The constructor is executed as soon as we create an object of our class, so we don’t have to worry about calling it.
We then call the method get_name()
which prints out the name associated with our player.
You can add in as many parameters to a class as you want:
class Player: def __init__(self, name, player_type): self.name = name self.player_type = player_type def get_name(self): print("Your name is " + self.name) def get_player_type(self): print(self.name + " is a " + self.player_type + ".")
In this example, our Player class supports two parameters. One represents the name of a Player (name) and the other represents the type of that player (player_type
). Let’s create an object of this class:
leah = Player("Leah", "Mage") leah.get_name() leah.get_player_type()
Our code returns:
Your name is Leah.
Leah is a Mage.
Both of the initial values we specified are accessible in our class.
Conclusion
Classes allow you to reduce repetition in your code. Once you’ve defined a class, you can use its structure to define as many objects as you want. As a result of the reduced repetition, your code should be easier to read, and therefore easier to maintain.
Now you’re ready to start writing your own Python classes like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.