The Python Boolean data type has only two possible states, the keywords False
and True
. Booleans cannot hold any other value, and are the smallest data type. Booleans are essential to many aspects of programming, like the use of if statements.
Note on capitalization. The word Boolean, named after the mathematician George Boole, is always capitalized. In addition, the words True and False are capitalized in Python because they are reserved keywords in the programming language.
To help you get started with Python Booleans, we wrote this tutorial on Boolean basics. We’ll discuss how to use Booleans to evaluate variables and expressions. And we’ll show how Booleans can be used with Python if statements to control the flow of a program.
Python Boolean
Booleans are used to represent truth values, and they derive from mathematics and arithmetic. In fact, Booleans are the building blocks of complex algorithms. When you’re programming, you use Booleans to evaluate expressions and return an outcome that is either True or False.
Here’s an example of a Boolean Python uses to store whether it is summer:
is_summer = False
Our parameter evaluated to False, indicating that it is not summer. The following are the elements of this Python statement:
Element | Example |
variable | “is_summer” |
operator | “=” |
value | “False” |
When programming, we assign the Python Boolean values to a variable; the value can only be equal to True or False.
Comparisons in Python
Comparisons are a feature of programming that allow you to compare operands and return a True or False value based on the comparison.
For instance, say you are building a program that calculates the tallest building in the world. For your program to work, you may want to compare multiple Python Boolean parameters or values to find out the tallest. To do this, you could use the Boolean data type.
Python Operators
In addition to arithmetic operators, there are a number of Python logical operators and comparison operators. You can use these operators to compare values, or operands. A table of the comparison operators and associated Python symbols follows:
Comparison Operator Symbol | Description |
== | equal to |
!= | not equal to |
> | greater than |
< | less than |
>= | greater than or equal to |
<= | less than or equal to |
To further illustrate how Booleans work in Python, let’s use another example. Say we own an ice cream store and want to know whether a loyalty member has purchased more than eight pints. This is important because each customer gets a free pint of ice cream after purchasing eight pints.
We could check whether a customer has purchased more than eight pints of ice cream using the following lines of code:
pints_of_ice_cream_purchased = 6 print(pints_of_ice_cream_purchased > 8)
The output of our code is False
.
As you can see, our program uses the greater than operator to check if our customer has purchased more than eight pints. In this case, our customer has only purchased six pints of ice cream, so our operand evaluated to False.
What if we wanted to check whether our customer has purchased exactly eight pints of ice cream? We could use the equal to (==) operator, like this:
pints_of_ice_cream_purchased = 6 print(pints_of_ice_cream_purchased == 8)
The outcome of our code is False
.
Because our customer has only purchased six pints of ice cream, our statement pints_of_ice_cream_purchased == 8
evaluated to False
. If our customer had purchased eight pints of ice cream, our statement would have evaluated to True
.
Similarly, Booleans can be used to evaluate the outcome of a string comparison. Say we want to know whether a customer has ordered the Triple Chocolate Fudge
flavor of ice cream, which is on the secret menu. We could perform this comparison using the following code:
order = "Triple Chocolate Fudge" print(order == "Triple Chocolate Fudge")
The output of our code is True
.
Our customer has ordered the Triple Chocolate Fudge
ice cream flavor, so when our program evaluates this, it returns: True
.
It’s worth noting that string comparisons are case-sensitive. So, if we had input the customer’s order as triple chocolate fudge
, the above code would have returned False
.
Evaluate Variables Using Boolean
Python includes a built-in function called bool()
that you can use to evaluate a variable or value. bool()
takes in one argument: the value or variable you want to evaluate.
Here’s the syntax for the bool()
method:
bool(value)
The bool()
method can be useful in a number of cases.
Firstly, if you want to perform any comparison, you can enclose it within the bool()
method, which returns a Boolean value. For example, say you want to know whether a customer has purchased 50 or more pints of ice cream at your store. If so, you want to send that customer a thank-you card. You could figure this out using the following code:
pints_of_ice_cream_purchased = 51 print(bool(pints_of_ice_cream_purchased >= 50))
The output of our code is True
.
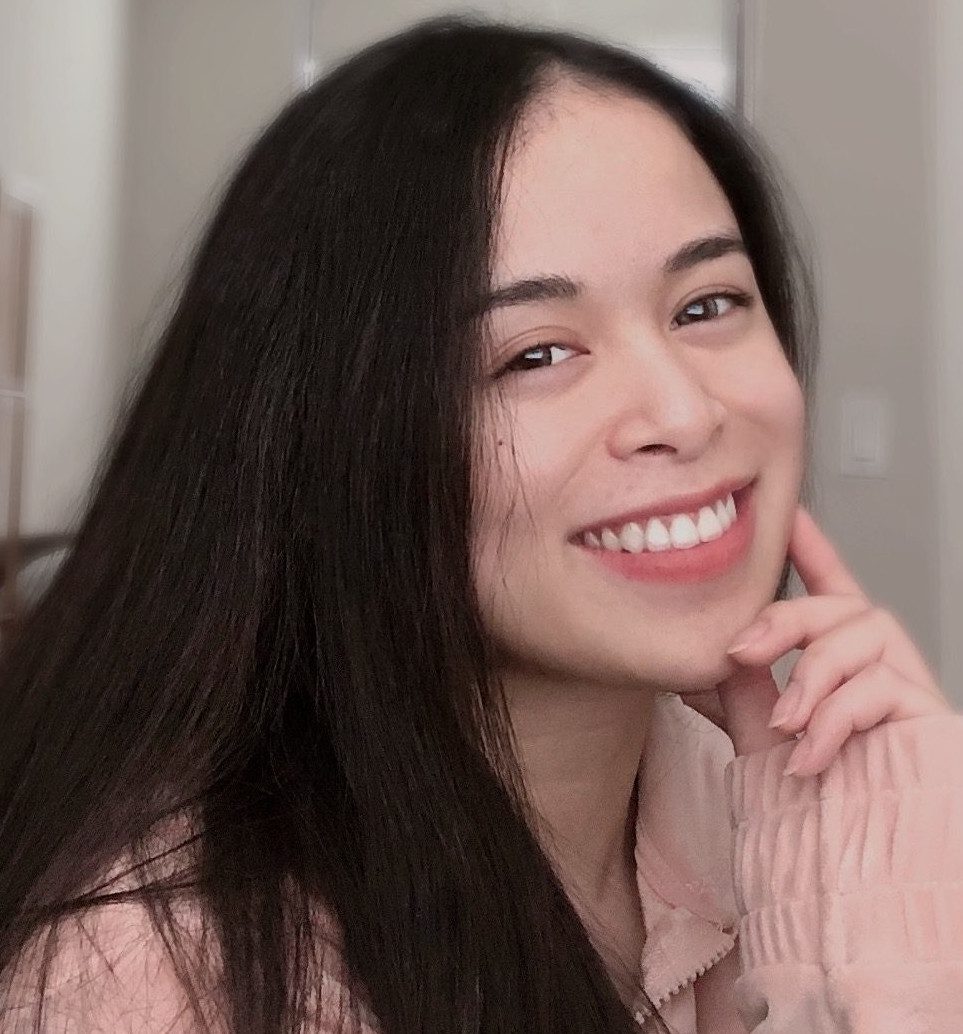
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our customer has purchased more than 50 pints of ice cream, so our statement evaluates to True
. It’s worth noting that you don’t need to use the bool()
method for a comparison like this. But it can be helpful, as it clearly shows the purpose of your code.
Secondly, if you want to check if a value is empty, None, or False, you can use the bool()
method. This is the most popular use of the bool()
method. This method converts a value into a Boolean according to “standard truth” testing. So, you can use the method, for example, to convert integers or floating point numbers into Booleans.
Let’s say we have a list of ice cream orders by a loyalty customer. We want to check whether they ordered with their loyalty card. We could perform this evaluation using the following lines of code:
ice_cream_orders = [] print(bool(ice_cream_orders))
Our code returns False
.
In this case, our loyalty customer has not placed any orders using their card, so our bool()
method returns False
.
Boolean Flow Control
The Boolean value is an important part of flow control in programming. This is because Booleans evaluate whether a condition is True or False. And that information can be used to judge whether a specific block of code should be run.
In Python, conditional statements in the header line, such as if statements, run code if a certain condition or set of conditions is met. These statements only work because Booleans evaluate the condition specified in the statement.
Let’s use an example to illustrate how this works. Say we are creating a program that takes in a customer’s email address and checks whether they are signed up for our rewards program. If the customer is signed up, we want to print a message to the console that states This customer is a Loyalty Customer!
Otherwise, we want to print No record.
To do this, we could use the following lines of code:
loyalty_customer = True if loyalty_customer = True: print("This customer is a Loyalty Customer!") else: print("No record.")
Our code returns True
.
In our program, our if
expression (in the header line) evaluates whether the variable loyalty_customer
is equal to True
. If loyalty_customer
is equal to True
, the if
statement will evaluate to True
. Thus, the message This customer is a Loyalty Customer!
on the next line will be printed to the console. If loyalty_customer
is equal to False
, then the if
statement will evaluate to False
. In that case, our program will print No record.
Booleans allow us to evaluate statements and can be used with conditionals to check whether a certain statement is True
or False
.
Conclusion
Booleans are an important data type in programming that can indicate whether a value is True
or False
.
Booleans are often used to evaluate statements using comparison operators and can also be used to control the flow of a program. In addition, Python has a built-in method called bool()
to check whether an item is empty or equal to None or False.
This tutorial explored comparison operators with Booleans, flow control with Booleans, and the Python bool()
method. Now you’re equipped with the knowledge you need to work with Booleans in Python like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.