There are two ways to find the average of a list of numbers in Python. You can divide the sum() by the len() of a list of numbers to find the average. Or, you can find the average of a list using the Python mean() function.
Finding the average of a set of values is a common task in Python.
For example, you may have a list of product sales and want to find out the average purchase price. Or, for a diversity report, you may want to calculate the average age of employees who work for your organization.
Python Average
There are two methods employed to find the average of a list of numbers in Python:
- By calculating the sum of the list and then dividing that number by the length of the list. The length of the list is how many values are in the list. or;
- By using the statistics.mean() method.
While both methods return the average of a list of numbers, there are different factors you should consider when choosing which one to use.
In this tutorial, we discuss how to use the aforementioned approaches to find the average of a list in Python. We’ll walk through two examples to help you get started.
Python Average: Len() and Sum()
The formula for calculating the average of a list of values is the sum of all terms divided by the number of those terms. We can use the Python sum() and len() values to calculate the average of the numbers in a list.
The Python len() method calculates and returns a count of numbers in a list. len is short for length, referring to the length of—or number of—items in a list.
The sum() method in Python calculates the total sum of all values in a list.
We can use these functions together to calculate the average value from a list of values.
The basic formula for calculating an average in Python is:
avg_value = sum(list_of_values) / len(list_of_values)
This approach is common because you do not have to import any external values to calculate an average.
sum() and len() Function Example
Let’s say that we run a coffee shop and want to know the average price of all orders placed so far today. To calculate this number, we could use the sum() and len() methods that we just discussed:
orders = [2.50, 4.50, 2.00, 2.75, 2.50, 5.00, 8.00, 2.25] average = sum(orders) / len(orders) print("The average coffee order price today is $" + str(round(average, 2)))
We print the average value to the console:
The average coffee order price today is $3.69
On the first line of our code, we define a Python variable. This variable stores a list of order prices. Then, we calculate the average of those orders by dividing the sum of all orders by the length of our order list.
At the end of our code, we print out a message to the console that tells us the average price of coffee orders.
We use the Python round() method to round our average to two decimal places. The str() method to converts our average value into a string that we can print to the console.
Now we know the average coffee order price so far today is $3.69.
Python Average: statistics.mean()
The Python statistics library contains a function called statistics.mean() that calculates the mean value of a list of values. The term mean is used in mathematics to describe the average of a list of values.
The syntax for the statistics.mean() method is:
statistics.mean(list_of_values)
The mean() method takes in one parameter: the list of items whose average you want to calculate.
Before we use this method, we need to import the statistics module (statistics) in Python. This is a built-in module that can be used to perform various calculations in Python.
This is an important difference between using statistics.mean() and the sum() and len() method. The sum() and len() method can be used without importing any libraries. But, you need to import statistics to use statistics.mean().
If you do not mind importing another library, this method works fine. Because you can calculate an average value without any libraries, you should consider doing so before using the statistics library.
We can import the statistics module using a Python import statement:
import statistics
We can now access the mean() function in the statistics library.
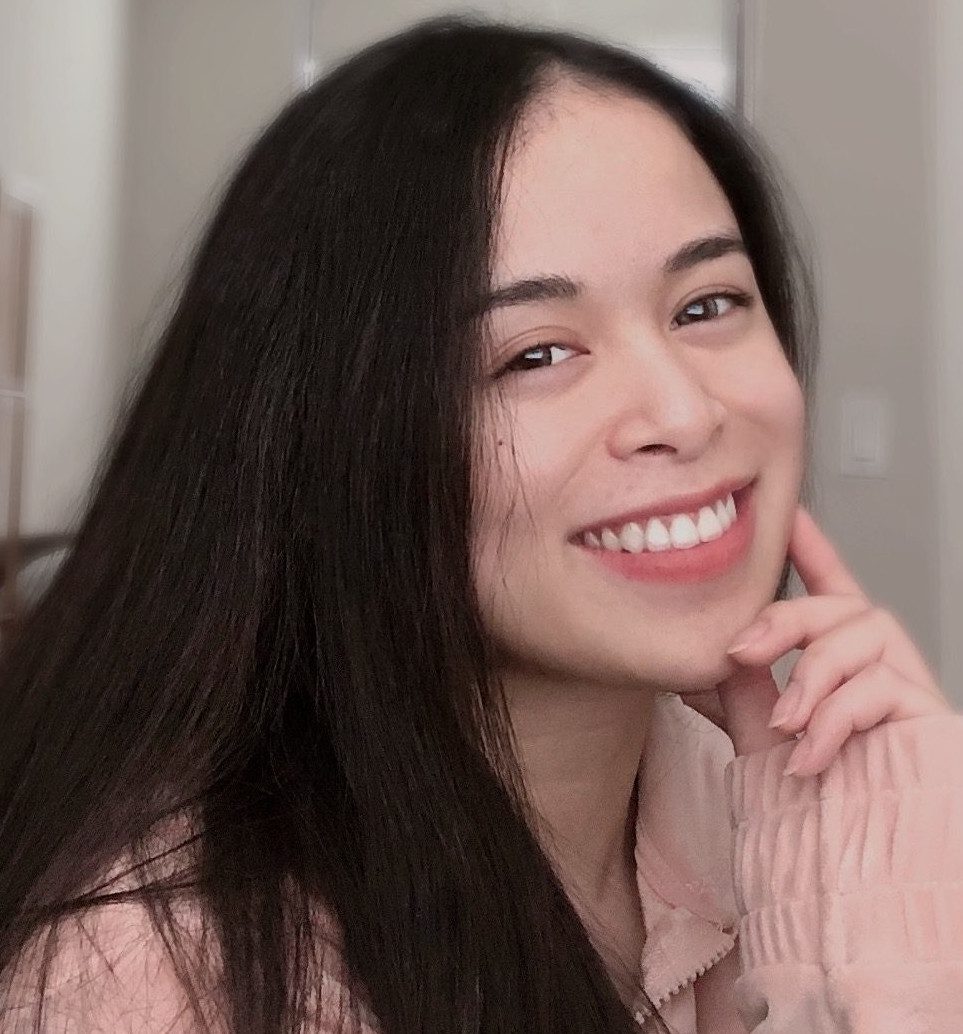
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
statistics.mean() Example
Now we’re ready to start calculating averages using mean().
Let’s take the example of coffee orders that we used above. Say we want to calculate the average price of the coffee orders placed so far today. We could use the following code to calculate the average price:
import statistics orders = [2.50, 4.50, 2.00, 2.75, 2.50, 5.00, 8.00, 2.25] average = statistics.mean(orders) print("The average coffee order price today is $" + str(round(average, 2)))
Let’s look at the result of our program:
The average coffee order price today is $3.69
Our program returns the same text and result it did in our first example.
Calculate Average of a Tuple
We can use the statistics.mean() method to calculate the mean value of a tuple, which is an unchangeable, ordered sequence of items. Here’s an example of statistics.mean() being used to calculate the average coffee order price, with our information stored in a tuple:
import statistics orders = (2.50, 4.50, 2.00, 2.75, 2.50, 5.00, 8.00, 2.25) average = statistics.mean(orders) print("The average coffee order price today is $" + str(round(average, 2)), ".")
Our code returns the same response as our last example because we’re working with the same numbers:
The average coffee order price today is $3.69.
A tuple may be used instead of a list if you are working with values that should not change. So, because our coffee order prices will not change after an order has been processed, a tuple is appropriate.
Mean of Negative Values
You can use mean() to calculate the mean value of a set of negative numbers.
Let’s say that we have a list that stores the lowest daily temperatures recorded during a specific week in winter. We could use the following code to calculate the average of that week’s lowest daily temperatures:
import statistics temperatures = [-12, -14, -2, -5, -8, -4, -9] average = statistics.mean(temperatures) print("The average of this week's lowest daily temperatures is", round(average, 2), "degrees Celsius.")
Our code returns:
The average of this week's lowest daily temperatures is -7.71 degrees Celsius.
Conclusion
You can calculate the average of a list of values using the sum() and len() methods or the statistics.mean() method. The statistics.mean() method needs to be imported into your program. You can use the sum() and len() method without importing any external libraries into your code.
Do you want to learn more about how to code in Python? Read our How to Learn Python guide. This guide contains a list of top learning resources you can use to advance your knowledge.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.