Attributes are values or functions associated with an object, a data type, or a class. If you call an attribute on a value whose data type or class does not support that attribute, you’ll encounter an AttributeError.
This guide discusses what an AttributeError is and what it means. We’ll walk through an example of an AttributeError so you can learn how to fix one in your code.
What is a Python AttributeError?
A Python AttributeError is raised when you try to call an attribute of an object whose type does not support that method. For instance, trying to use the Python append() method on a string returns an AttributeError because strings do not support append().
In a Python class, you can define methods and values that are shared by the objects of that class. This is why some people think of classes as blueprints for objects.
Calling a method or a class is another way of saying you are referencing an attribute of that class. One way to think about an attribute is like a physical attribute of a person. Some people have blue eyes. Some people have pink-dyed hair. These are all attributes.
In a Python class, an attribute could be “eye_color”. This attribute could define the color of a person’s eyes. An attribute could also be a function. A function called changeEyeColor() could change the value of “eye_color”.
Data types have attributes. For instance, you can use the Python join() method to convert a string into a list. String objects support the join() method.
If you try to reference a function or a value not associated with a class object or a data type, you’ll encounter an AttributeError.
Attribute Error Python Example
Let’s write a program that merges two lists of shoes. Two shoe stores are going through a merger and want to make a list of all the unique shoes they sell.
To start, let’s define a Python set that contains the shoes from store one, Harrisons Shoes:
harrisons_shoes = {"Nike Air Force 1 07", "Nike Air Max Plus", "Adidas Gazelle"}
We use curly braces to define our set. Next, let’s define a set with the names of the shoes offered by the store that is merging with Harrisons. This shoe store is called the Shoe Emporium:
shoe_emporium = {"Adidas Samba", "Adidas Superstar", "Nike Air Max Plus"}
Because these two collections are sets, they can only store unique values. That means when we add them together, we’ll get a set with no duplicate values.
To add our sets together, we’re going to use the built-in function called extend():
harrisons_shoes.extend(shoe_emporium) print(harrisons_shoes)
The extend() method adds all the shoes from the “shoe_emporium” set to the “harrisons_shoes” set. We use a Python print() statement. This lets us we can see all the shoes in our new set. Let’s run our code and see what happens:
Traceback (most recent call last): File "main.py", line 3, in <module> harrisons_shoes.extend(shoe_emporium) AttributeError: 'set' object has no attribute 'extend'
Our code returns an AttributeError.
AttributeError Python Solution
Our error message tells us we cannot use the method extend() on an object whose data type is a set. This is because extend() is a list method. It is not supported by sets.
If we want to merge our two sets, we have to use an addition sign:
harrisons_shoes.update(shoe_emporium) print(harrisons_shoes)
This will add the contents of the “shoe_emporium” set to the “harrisons_shoes” set. We then print all the values in the “harrisons_shoes” set to the console. Let’s run our new program:
{'Nike Air Force 1 07', 'Adidas Superstar', 'Adidas Samba', 'Nike Air Max Plus', 'Adidas Gazelle'}
Our program returns a set with all the shoes from our two original sets. While there were six values in our original two sets, now there are only five. This is because two of the shoes were the same and sets can only store unique values.
Our program returns a set with all the shoes from our two original sets. While there were six values in our original two sets, now there are only five. This is because two of the shoes were the same and sets can only store unique values.
Similar AttributeErrors to Explore
AttributeErrors are incredibly common. They can arise when you try to call attributes of data types and classes that do not support the attribute you are referring to.
These errors may also be caused if you make a typo when referring to an attribute. Python will interpret your code as-is. If you make a typo, it will appear to Python that you are referring to an attribute that does not exist.
For instance, using the Python split() method to split a list is common. But, split() is a string method and so it cannot be used to split a list.
For further reading, consider researching the following errors:
- AttributeError: ‘list’ object has no attribute ‘split’
- AttributeError: ‘module’ object has no attribute ‘urlopen’
Conclusion
Attribute errors in Python are raised when an invalid attribute is referenced. To solve these errors, first check that the attribute you are calling exists. Then, make sure the attribute is related to the object or data type with which you are working.
If the attribute you want is associated with a built-in type and does not exist, you should look for an alternative. There are alternatives for many attributes that exist for one data type you can use on another data type. For instance, there is no extend() method with sets but you can use union() to join to sets.
To learn more about writing Python code, read our How to Learn Python guide.
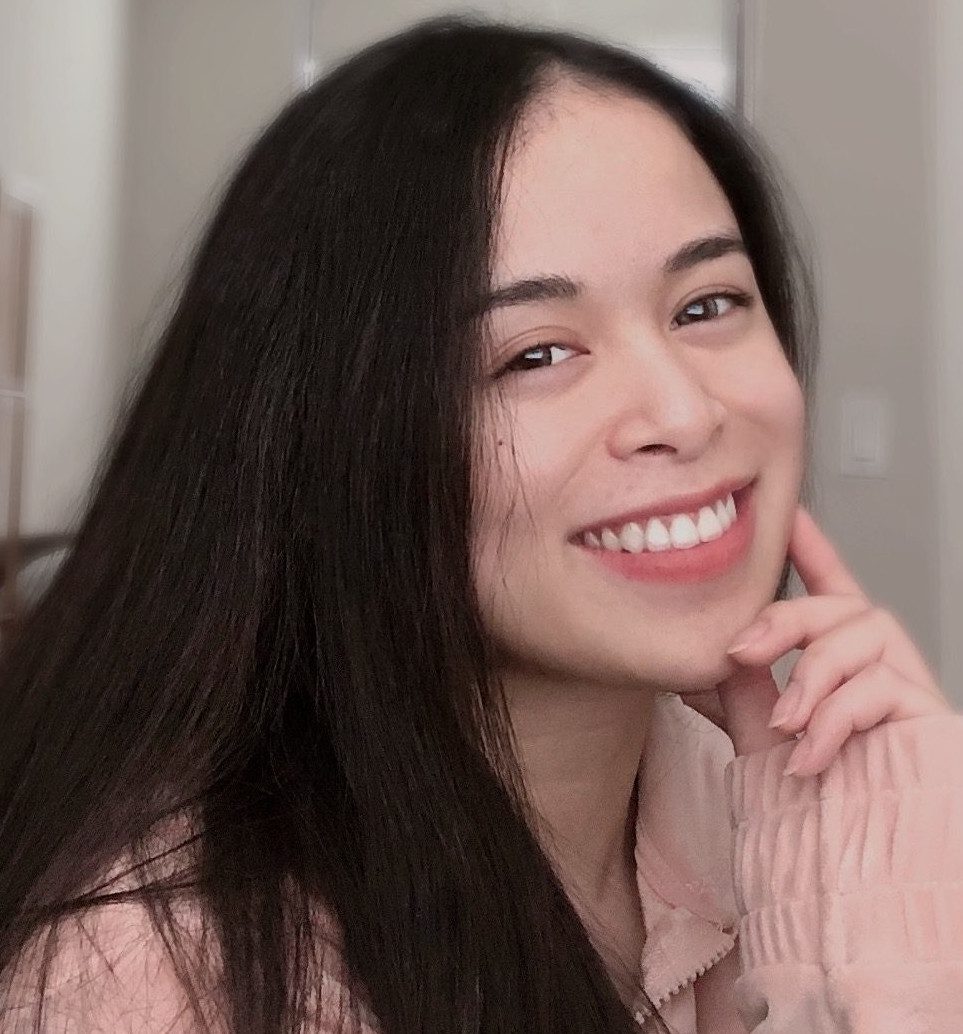
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.