The Python assert keyword tests if a condition is true. If a condition is false, the program will stop with an optional message. Assert statements are used to debug code and handle errors. You should not use an assert statement in a production environment.
Debugging is a crucial part of programming in any language. When debugging programs in Python, there may be times when you want to test for a certain condition. If that condition is not met, the program should return an error. That way, you know what to fix in your code.
That’s where the Python assert keyword comes in. The assert statement lets you test for a particular condition in Python. It is used commonly during Python debugging to handle errors.
This Python tutorial will explore the importance of assertion and how to use the assert statement. We’ll walk through a few examples of using the assert expression in a program to help you get started.
Assertion in Python
The assert statement tests for conditions in Python and fix bugs more quickly. If the condition you are testing with the assert statement evaluates to True, the program will continue to execute. If the assertion evaluates to False, the program will raise an AssertionError (+/- an optional message).
Assertions are used to identify and remove certain conditions when code is run. For instance, say you are running an operation on a list that will change the list’s values. You may want to use an assertion to ensure certain conditions are met before the list is changed. This will prevent making changes to your list that may not be correct.
Assertions are not a replacement for program errors such a SyntaxError or a NameError. If you’re looking to test a block of code, using “try … except” would be more appropriate. But if you want to check for a certain condition in your code, an assert statement may be more useful.
You should use assertions sparingly and only during debugging. This is important for two reasons.
First, assertions help developers find the cause of a bug before it impacts other code. Assertions do not raise just any error in a program. A developer must define an assertion in their code.
Second, Python interpreter users can disable assertion statements. Therefore, a number of problems can arise if you rely on assertions too heavily for error handling and a user disables assertions.
You should never use assertions to validate data outside a development environment. This is because disabling assertions could have a significant impact on how your program runs. If you rely on assertions, and they are disabled, your program could return new errors that you do not expect.
Python Assert Statements
An assert statement checks whether a condition is true. If a condition evaluates to True, a program will keep running. If a condition is false, the program will return an AssertionError. At this point, the program will stop executing.
In Python, assert is a keyword. This means that you will see the assert keyword appear in a special color when you use it. It uses the following syntax:
assert condition, message
Here are the two parameters assert can take in:
- condition: the condition for which you want to test (required)
- message: the message you want to display upon execution of the assert statement (optional)
Assert Python Example
Let’s use an example to illustrate the assert keyword in action. Say that we are creating a program that calculates whether a student passed or failed a certain test. A grade of 52 or higher is a pass, while any grade lower than 52 is a fail.
Checking for an Error
We want to make sure that we have inserted a user’s data into the program before we perform any calculations. Otherwise, our program may say that a user failed the test even though the data is not present.
We could create check for an error using the following code:
student_grades = [57, 74, 49, 0, 87, 66, 89] for g in student_grades: assert student_grades[g] != 0 if student_grades[g] >= 52: print('This student passed.') else: print('This student failed.') print('Program complete')
When we run our code, the program returns the following output:
This student passed. This student passed. This student failed. ... assert student_grades[g] != 0 AssertionError
On the first line of our code, we declare a list of student grades.
We create a for loop that iterates through the list of student grades. This for loop contains an assert statement that will raise an error if the statement student_grades[g] != 0 evaluates to False. In other words, if a student’s grade is 0, the program will return an AssertionError.
We define an if statement. This statement prints a message to the console if a student scored 52 or higher on the test (This student passed.). We display a different message if a student scored less than 52 (This student failed.). Finally, our program prints out Program complete to the console.
As you can see, our program started going through the list of student grades and calculating whether they passed or failed the test. However, when our program reached the fourth value in our list, it raised an AssertionError. This is because our student grade was equal to 0.
We wanted to make sure that each student’s numerical grade was present before calculating their grade. If a student’s grade was not present, we should use 0 in place of their numerical grade.
This would allow us to make sure that we do not give a student an incorrect grade. This is likely because this program is still in testing. We are still making changes to our program.
If this were a final program, we would want to use a more robust error-handling method than assert. But, for the purposes of debugging, assert is acceptable.
Adding a Custom Error Message
Now, let’s say that we want to include a custom error message in our program. If a user’s grade is equal to 52, we want an error to be raised. This error should say Student grade is 0. This error will make it clear to us, the developers, what problem our program encountered.
Here’s the code we would use to add an error message to our above code:
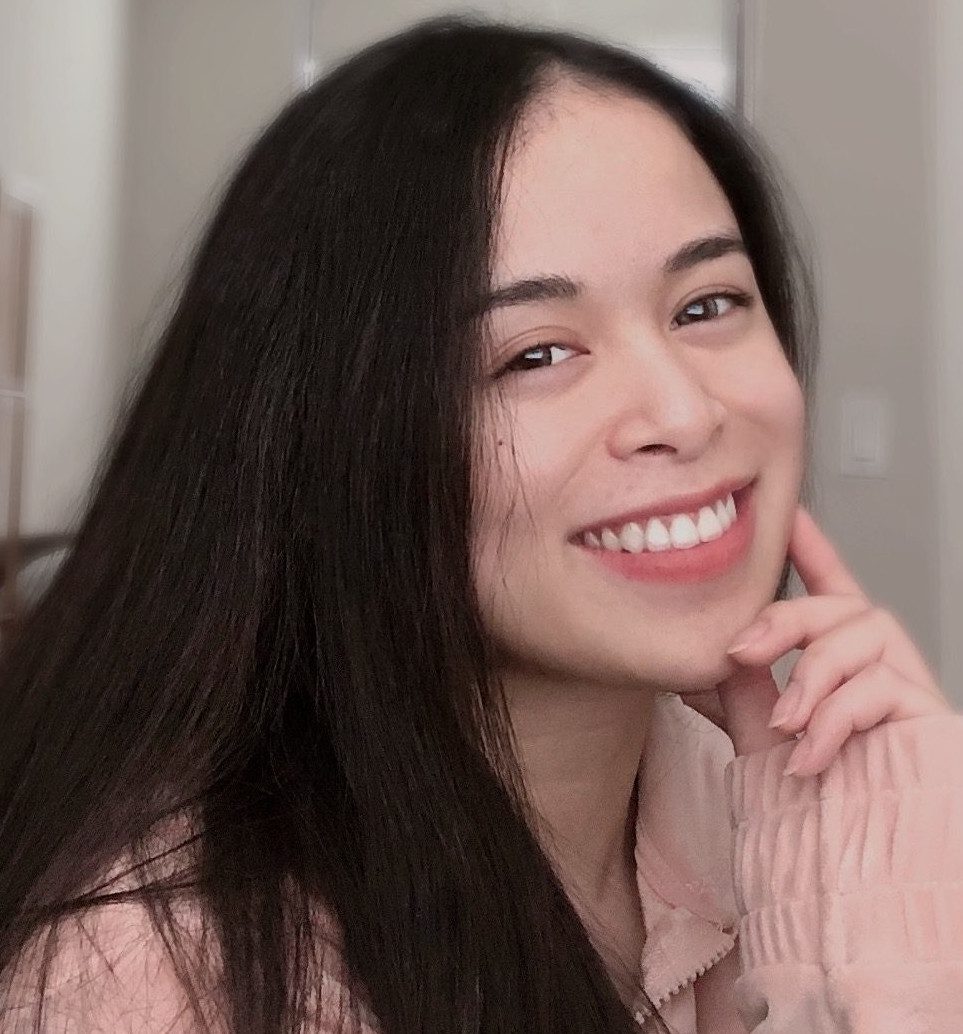
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
... assert student_grades[g] != 0, "Student grade is 0." if student_grades[g] >= 52: print('This student passed.') else: print('This student failed.') …
Now, when we run our program, we get the following result:
This student passed. This student passed. This student failed. … AssertionError: Student grade is 0.
The program still raised an AssertionError. This time our displayed an additional error message that we defined in our code. The additional message can help the developer better understand the problems in their program, which can make debugging more efficient.
Conclusion
Python assertions are a useful debugging tool that can help you write more maintainable code. They make it easier to test for certain conditions in your code.
We suggest you use Python assertions sparingly. Since users can disable assertions, we suggest you never use them to perform tasks such as data validation. If you rely on assertions and they are disabled, your program may not run correctly.
This guide explored the usefulness and limitations of the Python assert keyword and how to use it. In addition, we discussed an example of the assert keyword in use. We also covered how to define custom error messages using assert.
Knowing how to use the assert statement is a good skill to have. Learning it is another step on your journey toward becoming a Python expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.