Python arrays are a data structure like lists. They contain a number of objects that can be of different data types. In addition, Python arrays can be iterated and have a number of built-in functions to handle them.
Python has a number of built-in data structures, such as arrays. Arrays give us a way to store and organize data, and we can use the built-in Python methods to retrieve or change that data. For example, if you have a list of student names that you want to store, you may want to store them in an array.
Arrays are useful if you want to work with many values of the same Python data type. This might be student names, employee numbers, etc. They allow you to store related pieces of data that belong. And they make it easy to perform the same operation on multiple values at the same time.
In this tutorial, we are going to break down the basics of arrays in Python and how they work. We’ll also cover a few built-in Python functions that you can run on your list to add and remove data.
Elements of an Array in Python
In programming, an array can be used to store a list of data. If you have a bookshelf that you want to categorize, you may want to store the titles of each book in an array. If you have a vinyl collection, you may want to store your data in an array.
Python Array Syntax
Here is the basic syntax to declare an array in Python:
students = ['Alex', 'Bill', 'Catherine', 'Andy', 'Molly', 'Rose'] print(students)
We have created an array! When we print our list of students, our output is the same as the array with data we declared:
['Alex', 'Bill', 'Catherine', 'Andy', 'Molly', 'Rose']
Each item in a list can be referenced, or the list can be referenced as a whole. This means that we are able to perform operations on specific items in our array.
This works because each value in an array has an index number, which tells us where the item is within an array. Indexes start with and correspond with a certain item in an array.
Here is the index of array values we declared above :
“Alex” | “Bill” | “Catherine” | “Andy” | “Molly” | “Rose” |
0 | 1 | 2 | 3 | 4 | 5 |
Calling a Python Array
Because each item has its own index number, we can access each item in our array. We can do so by calling our list and specifying an index number, like so:
print(students[2])
Our code will return the name of the student whose index value is two. Here is the output from our code:
"Catherine"
We can also use negative index numbers to access the items in our list, which allows us to count backward. If we have a long list—say, 100 items—it may be easier for us to count backward than to count from the start. Here are the reverse index numbers for our array above:
“Alex” | “Bill” | “Catherine” | “Andy” | “Molly” | “Rose” |
-6 | -5 | -4 | -3 | -2 | -1 |
If we want to get the second from the last item in our Python list, we would use the following code:
print(students[-2])
Our code returns the following:
"Molly"
Slicing Items in a Python Array
We can also slice our Python lists to get multiple values. Let’s say we wanted to get the names in the middle of our list of students. By using a slice operation, we can retrieve multiple values in the list by their index number. These values will be retrieved by creating a range of index numbers, separating them using a colon.
Here’s an example of a slice in Python:
print(students[1:5])
Our code returns the following:
['Bill', 'Catherine', 'Andy', 'Molly']
As you can see, our code has returned every name between the index numbers of 1 and 5 on our list. It’s worth noting that the first index number—where the slice starts—is inclusive, and the second index number is exclusive. This means that our code returned the four values between 1 and 5, inclusive of 1.
If we want to get all of the items before a certain point in an array, we can use this code:
print(students[:2])
Our code returns the following:
['Alex', 'Bill']
As you can see above, our code has retrieved all the items whose index value is before 2
.
Modifying Items in a Python Array
We can also use indexing to change the values within our list. We achieve this by referencing each item by its index value and assigning it a new value. In the below example, we will replace the name Rose
with the name Amy
:
students[5] = "Amy"
When we print our list (using print(students)
), the name Rose
will no longer appear, and Amy
will be in its place:
['Alex', 'Bill', 'Catherine', 'Andy', 'Molly', 'Amy']
Python Array Append and Pop
We have dealt with the same array with which we started. But what if we want to add a new item to our array? That’s where the append()
function comes in. Using the append()
array operation allows us to add an element to the end of our array, without declaring a new array.
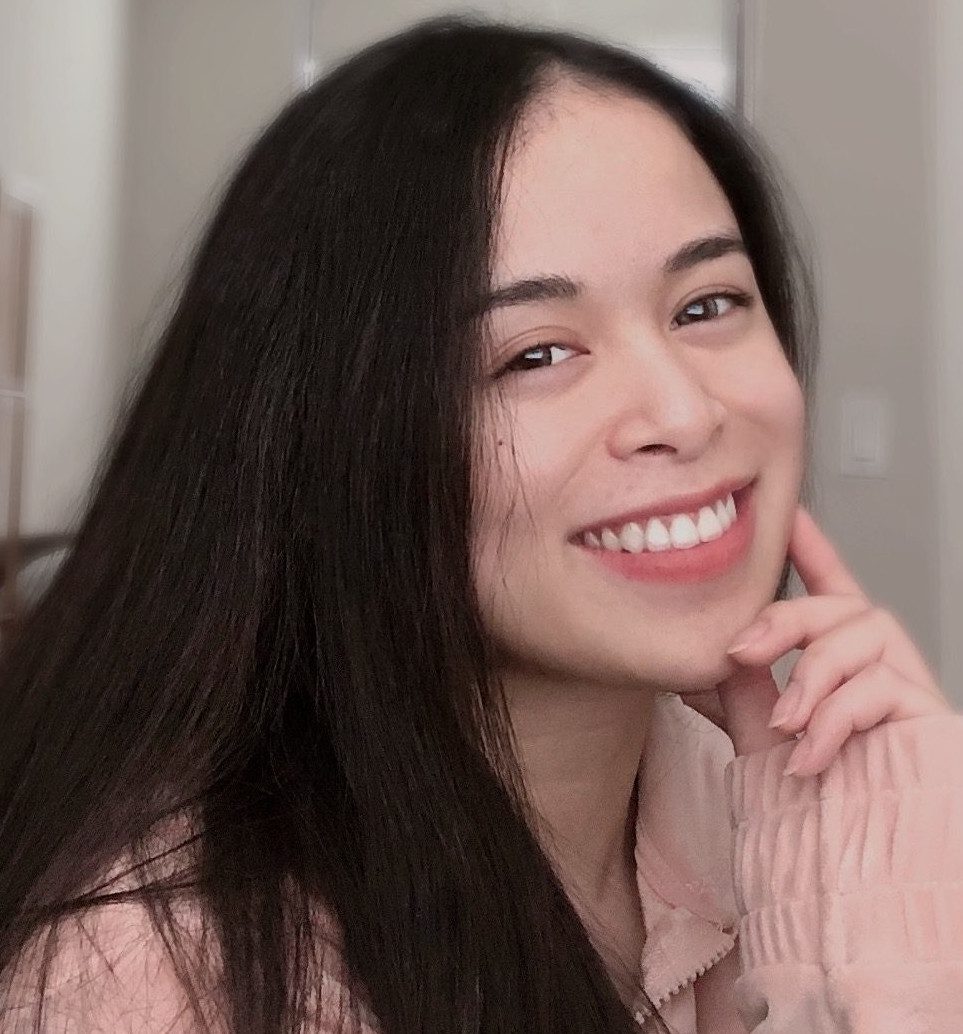
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here is an example of the append()
function that will add the name John
to our list of students:
students = ['Alex', 'Bill', 'Catherine', 'Andy', 'Molly', 'Rose'] students.append('John') print(students)
Our code returns the following:
['Alex', 'Bill', 'Catherine', 'Andy', 'Molly', 'Rose', 'John']
If we wanted to remove items from our array, we could use the Python pop function. Python pop()
works in the same way as append()
, but it works as a remove method that deletes an item from an array. In addition, pop()
takes an index number as an argument, rather than a string. Here is an example of pop()
in action:
students = ['Alex', 'Bill', 'Catherine', 'Andy', 'Molly', 'Rose'] students.pop(0) print(students)
Our code removes the individual element with the index value of 0
from our array (which corresponds with the name Alex
), and returns the following:
['Bill', 'Catherine', 'Andy', 'Molly', 'Rose']
Python Array Methods
We have scratched the surface of array methods in Python, and there’s a lot more to explore. The basic array methods you need to know are append()
and pop()
, which allow you to add and remove items from an array. You also need to know the basic structure of arrays, as we have discussed.
If you want to explore arrays in more depth, here are the main array methods that you may want to investigate:
Method Name | Description |
append() | Adds an item to an array |
pop() | Removes an item from an array |
clear() | Removes all items from an array |
copy() | Returns a copy of an array |
count() | Returns the number of elements in a list |
index() | Returns the index of the first element with a specific value |
insert() | Adds an element to the array at a specific position |
reverse() | Reverses the order of the array |
sort() | Sorts the list |
View the Repl.it from this tutorial:
Conclusion
Arrays are very flexible data structures in Python that we can use to work with lists of data. In this tutorial, we have demonstrated how arrays can be used to store multiple values and how they are accessed. We’ve discussed how to retrieve a Python array using its index numbers. We also covered how to modify items in an array.
We discussed how to add and remove items from an array using the append()
and pop()
functions, respectively. Now, you’re prepared for a variety of situations where you may need to use an array in Python!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.