Both Python *args and **kwargs let you pass a variable number of arguments into a function. *args arguments have no keywords whereas **kwargs arguments each are associated with a keyword.
Traditionally, when you’re working with functions in Python, you need to directly state the arguments the function will accept.
In some cases, where you may want to accept more arguments in the future, it is impractical to state all the parameters that you want to use with a function directly.
That’s where the Python *args and **kwargs keywords come in. These keywords allow you to pass an undefined number of arguments to a function.
This tutorial will discuss, with reference to examples, the basics of the *args and **kwargs keywords in Python, and how they compare.
Python *args
The Python *args method represents a varied number of arguments. It lets you pass arguments to a function when you are unsure how many arguments you want to pass.
The term “args” is a placeholder. In your function, you can replace the name of “args” with any value. You could use “numbers” to represent a list of numbers or “student” to represent a students’ details.
The syntax for the *args method is:
def our_function(*numbers): for n in numbers: print(n)
This function accepts a variable number of arguments. So, we could pass zero arguments, one argument, two arguments, and so on. Our function prints out to the console each argument that we pass into our function.
The value after the asterisk, “numbers” represents our arguments.
The args keyword is useful if you are performing mathematical operations, such as if you want to add up a variable amount of numbers.
*args Python Example
Let’s write a program that adds together the values of multiple arguments. This lets us perform a mathematical sum without having to use a plus sign for each value that we want to add.
Here’s an example of a function that is capable of adding together multiple arguments:
def addNumbers(*args): total = 0 for a in args: total = total + a print(total) addNumbers(9, 10, 12) addNumbers(13, 14, 15, 15)
Our code returns:
31 57
First, we declare a Python function called addNumbers(), which contains the code for our number adding program. This function has one argument specified: *args.
The *args keyword is used to represent a variable number of potential arguments that could be passed into the function.
Then, we declare a Python variable called total which keeps track of the total number we are adding. Initially, this variable is set to 0.
We use a for loop to go through every argument in the args keyword, and we add each number to our total. Notice that when you are referring to the arguments stored in *args, you use the “args” keyword. Next, we print out the total number our program has calculated.
In our main program, we call the addNumbers() method twice. When we first call the function, we specify three numbers we want our function to add together. Next time we call the function, we specify four numbers we want our function to add together.
Because we used *args, we can send an unlimited number of arguments to our function. Our program works no matter how many arguments we pass.
Python **kwargs
The **kwargs keyword represents an arbitrary number of arguments that are passed to a function. **kwargs keywords are stored in a dictionary. You can access each item by referring to the keyword you associated with an argument when you passed the argument.
The syntax for **kwargs is:
def our_function(**record): for key, value in record.items(): print("{} (key) and {} (value)".format(key, value))
This code lets us pass a variable number of keyword arguments into our function. The name **kwargs is just a substitute. You can replace “kwargs” with any term you wish.
We use the name after the asterisk as a label for our keyword arguments. In this case, our label is “record”. Values in a **kwargs method are stored in key-value pairs. This is why we need to use items() to iterate over all of the arguments we passed to our function.
**kwargs Python Example
Let’s walk through a basic example of a function using **kwargs to illustrate how the keyword works.
Suppose we are building a program that displays information about a customer’s order at a coffee shop. We want to pass the customer’s order information to a function, which will then print out the data to the console. Let’s create a function:
def printOrder(**kwargs): print(kwargs) printOrder(coffee="Mocha", price=2.90, size="Large")
Our code returns:
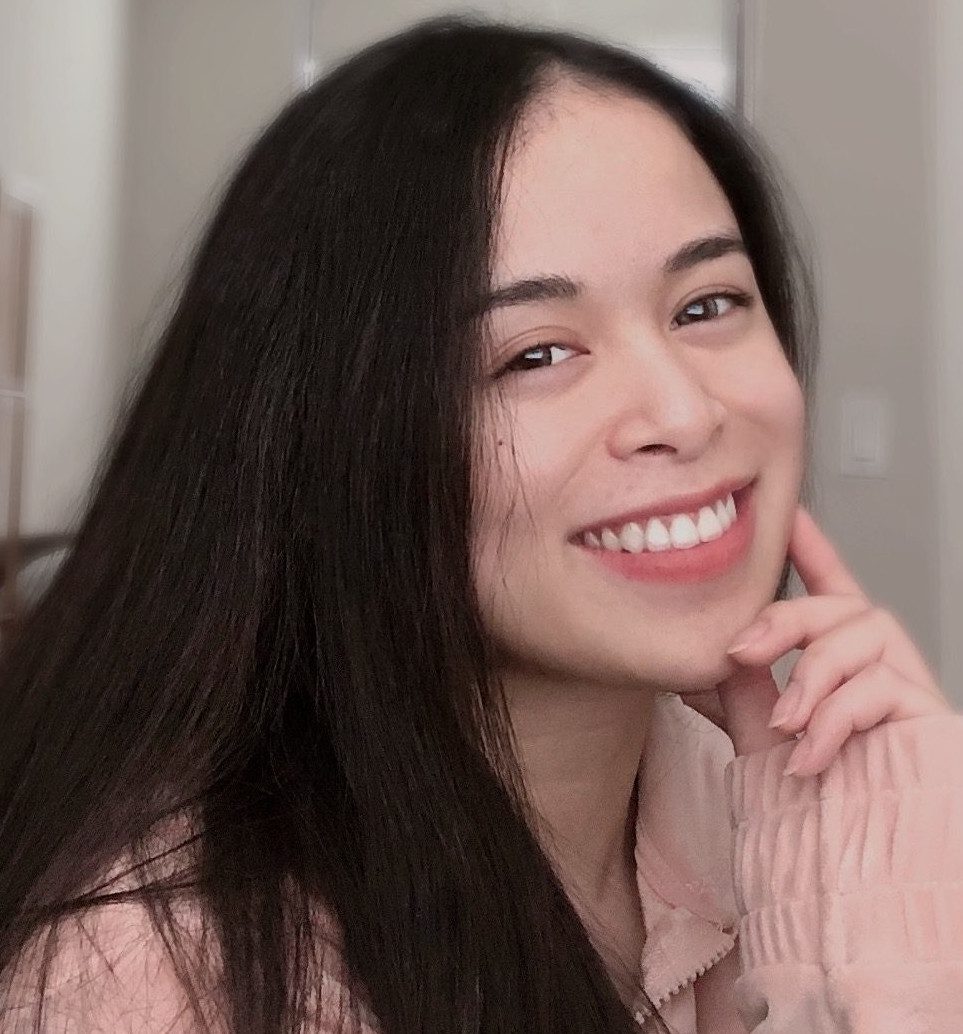
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
{'coffee': 'Mocha', 'price': 2.9, 'size': 'Large'}
First, we declare a function called printOrder() which uses the **kwargs keyword to accept a variable number of arguments. This function prints out the value of “kwargs” (which stores all the keyword arguments passed to our function).
Then, we call our function and pass three arguments: coffee, price, and size. When we pass these values, they are sent to our function as a dictionary.
Our code returned a Python dictionary with three arguments that we specified, alongside their values. If we wanted to, we could have passed even more or even fewer arguments, because **kwargs represents a variable number of arguments.
Because our kwargs are stored in a dictionary, we can access their values individually like we would with any Python dictionary.
Improving Our Code
Now, suppose we wanted to print out the details about the coffee order in a more structured way. We could do so using this code:
for key, value in kwargs.items(): print("{} is equal to {}".format(key, value)) printOrder(coffee="Mocha", price=2.90, size="Large")
Our code returns:
coffee is equal to Mocha price is equal to 2.9 size is equal to Large
Let’s break down our code. First, we declare a function called printOrder() which uses the **kwargs keyword to accept keywords. Then, we created a for loop that iterates through every item in our kwargs dictionary.
We use the Python dictionary items() method to access each key and value in our dictionary.
This for loop prints out the name of each key in our dictionary (coffee, price, and size). Then, out code prints “ is equal to ”. Finally, our code prints the value that corresponds to each key (“Mocha”, 2.90, and “Large”, respectively).
If we wanted to pass four keywords to our program, or two, we could. This is because **kwargs supports keyworded variable length argument lists, like *args.
Python *args vs. **kwargs
The arguments sent to a function using **kwargs are stored in a dictionary structure. *args sends a list of arguments to a function.
Also, send arguments using **kwargs, you need to assign keywords to each of the values you want to send to your function. You do not need to specify keywords when you use *args.
A single asterisk denotes *args whereas **kwargs uses a double asterisk. This is an important distinction because both “args” and “kwargs” are placeholders. You can replace these words with any value.
How to Order Python Arguments
Arguments in a Python function must appear in a specific order. This order is as follows:
- Formal arguments
- *args
- Keyword arguments
- **kwargs
So, if you’re working with both *args and **kwargs, you would use this code:
def printOrder(*args, **kwargs):
In addition, if you want to specify default values for certain arguments that may arise, you can use this code:
def printOrder(coffee, *args, coffee_order="Espresso", **kwargs):
This function expects to receive an argument called coffee, and the default value of any argument called coffee_order will be Espresso. However, you can specify as many arguments as you want with this function, because *args and **kwargs are used.
Conclusion
The *args and **kwargs keywords allow you to pass a variable number of arguments to a Python function. The *args keyword sends a list of values to a function. **kwargs sends a dictionary with values associated with keywords to a function.
Both of these keywords introduce more flexibility into your code. This is because you don’t have to specify a specific number of arguments upfront when writing a function.
To discover the best Python courses and learning resources, check out our How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.