The append() Python method adds an item to the end of an existing list. The append() method does not create a new list. Instead, original list is changed. append() also lets you add the contents of one list to another list.
Arrays are a built-in data structure in Python that can be used to organize and store data in a list. Instead of storing similar data in multiple variables, you can store them in a list. Lists can hold any data type, like strings and numbers.
The append() method lets you add items to an existing list. This means that you do not have to create a new list every time you want to update the contents of your list. In this guide, we’re going to discuss how to use the append() method.
Python List Refresher
Python lists can store zero or more items of a specific data type, such as strings, JSON objects, or numbers. For example, you can use a list to store a list of names or addresses. Lists are mutable. This means you can change their contents.
To create a new list in Python, you need to separate a list of values with commas and enclose it in square brackets []. Let’s create a list in Python, using this code:
toy_animals = ["Shark", "Dragon", "Bear", "Cat", "Giraffe"]
When we print out our list to the console, we can see the list that we’ve defined:
print(toy_animals)
Output:
["Shark", "Dragon", "Bear", "Cat", "Giraffe"]
Each item on our list has an index number, which can be used to reference an individual list item. These index numbers start with the value and increase for each item in a list. For our above toy_animals list, index numbers are assigned like this:
“Shark” | “Dragon” | “Bear” | “Cat” | “Giraffe” |
0 | 1 | 2 | 3 | 4 |
The first item on our list Shark has the value 0. The last item Giraffe has the value 4. We can use these index numbers to access and manipulate our list. So, if we want to access the element with the index number 1, we can use the following code:
print(toy_animals[1])
Output:
"Dragon"
Lists are mutable, which means that you can add and remove items to an existing list. Now that we’ve explored the basics of lists and list indexing in Python, we can start using the append()
and insert()
methods.
Python Append to List Using Append()
The Python append() list method adds an element to the end of a list.
The append() method accepts the item that you want to add to a list as an argument. The list item can contain strings, dictionaries, or any other data type.
Let’s start add elements to the list of toy animals we started building earlier. Our list contains five items, with index values ranging between 0 and 4.
toy_animals = ["Shark", "Dragon", "Bear", "Cat", "Giraffe"]
Suppose that we have a new toy animal, the Meerkat, which we want to add to our list. We could use the append() method to add the animal to our list like so:
toy_animals.append("Meerkat") print(toy_animals)
We have added a single element to our list. Now our list contains six objects. Meerkat appears at the end. It’s worth noting that the append() function has altered our original list. A new list was not created.
Append Python List to Another List
You can append the contents of an existing list to another list using the Python list append() method.
We want to add three new toy animals to our list: Rabbit, Dolphin, and Kangaroo. Instead of adding each animal individually, we can add them into a new list. Then, we can append the new list to our old one.
Consider the following code:
new_animals = ["Rabbit", "Dolphin", "Kangaroo"] toy_animals.append(new_animals) print(toy_animals)
We have defined a new list called new_animals. This list contains the three animals to which we want to add to our original list.
We pass the new_animals list as an argument in the append() method. This lets us add the contents of new_animals to our toy_animals list. Then, we print the contents of the toy_animals list to the console.
Let’s run our code and see what happens:
["Shark", "Dragon", "Bear", "Cat", "Giraffe", "Rabbit", "Dolphin", "Kangaroo"]
The three animals are added to the end of our list. Remember, append() adds items to the end of a list. You cannot specify the position at which an item is added.
View the Repl.it from this tutorial:
Conclusion
The Python list append() method lets you add an item to the end of a list. You can specify an individual item to add one values to a list. You can specify a list of items to add multiple values to a list. In other words, you can add a single element or multiple elements to a list using append().
Now you’re equipped with the knowledge you need to add items to a list like a Python expert.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
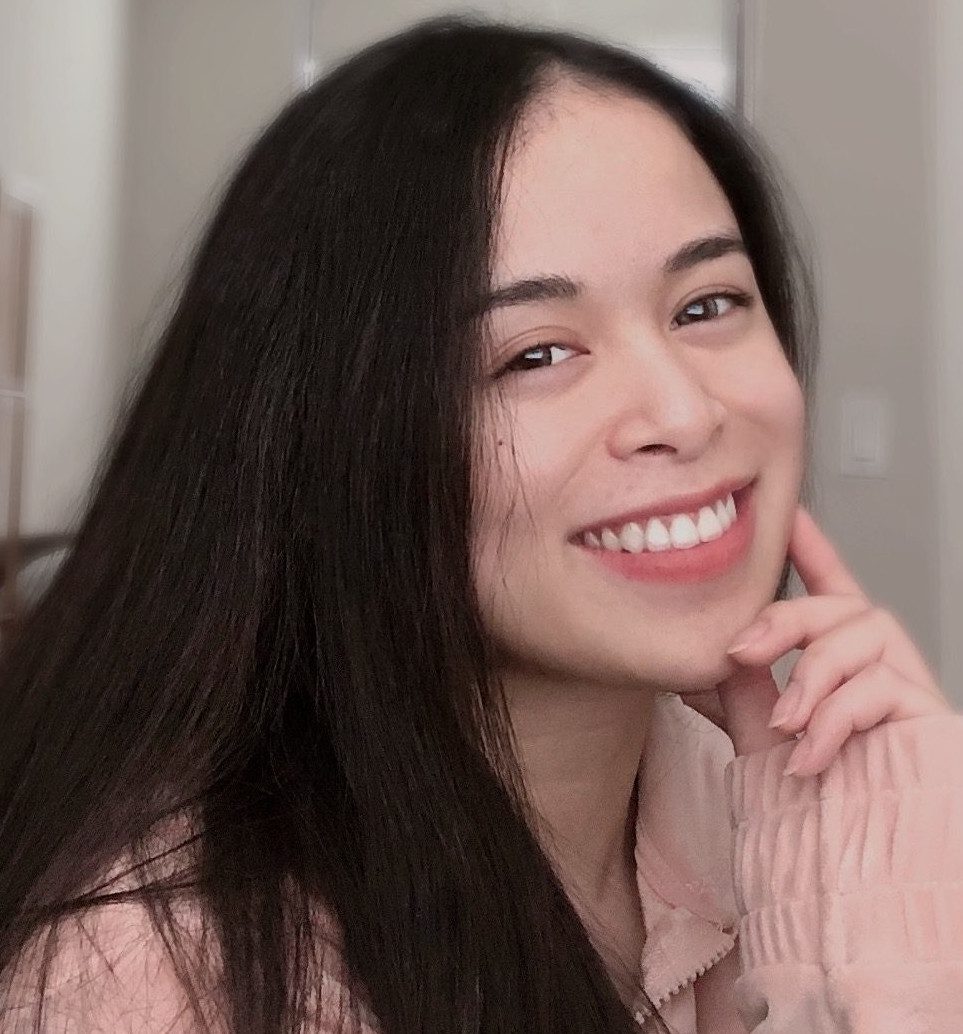
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot