The Python any()
and all()
functions evaluate the items in a list to see which are true. The any()
method returns true if any of the list items are true, and the all()
function returns true if all the list items are true.
Often, when you’re programming, you may want to check whether any or all of the values in a list evaluate to True. For instance, if you’re creating a program that tracks the racing history of a driver, you may want to calculate whether that driver has lost any or all of the races in a particular season.
That’s where the Python built-in functions any()
and all()
come in. any()
iterates through every item in an object and returns True if any item is equal to True. all()
goes through every item in an object and returns True only if every item in the object is equal to True.
This tutorial will discuss how to use the any()
and all()
methods in Python, and explore an example of each of these methods in a program.
Python Any
The Python any()
method calculates whether any value in an iterable object—a list, string, or tuple—is equal to True and returns True; otherwise, any()
returns False.
The any()
method accepts one parameter: the object with the values you want to search. Here’s the syntax for the any()
method:
any(iterable_object)
any()
returns True if at least one item in an iterable is True.
Otherwise, any()
will return False if all the elements are not True, or if an iterable object is empty.
Any() With an Array
Let’s say that we work for a data science company that is analyzing the scores for racers. We have been tasked to find out whether John Appleseed has ever ranked in the top 10 of an Indianapolis 500 race. We have a list of his entries in the race which stores True if he ranked in the top 10, and False if he did not.
To find out whether he has ever ranked in the top 10 of an Indy 500 race, we could use the following code:
race_wins_indy_500 = [False, False, True, False] print(any(race_wins_indy_500))
Our code returns: True
.
n the first line of our code, we define a list of values that stores whether he ranked in the top 10 in each time he entered the Indy 500 race. Then, we use the any()
method to check if any item in the list is True. In this case, he has ranked in the top 10 for the Indy 500 race once, so the any()
method returned True.
Any() With a Dictionary
We can also use the any()
method in a dictionary. Say that we have a record of John Appleseed’s entry at an individual Indy 500 race—the 2018 race—and we want to see whether he ranked in the top 10 for the race. We could do so using this code:
indy_500_2018_john = { 'driver_name': 'John Appleseed', 'race_year': 2018, 'top_ten': True } print(any(indy_500_2018_john))
Our code returns: True. Because our dictionary contains a True value, the any()
method returns True.
Any() With a String
Similarly, any()
can be used with a string or a tuple. In the case of a string, the any()
method will return True if the string contains a value; otherwise, it will return False.
Let’s say that we want to check whether a string that stores the player’s top 10 ranking for the latest Indy 500 race contains a value. We could use the following code to accomplish this task:
top_10_ranking = "" print(any(top_10_ranking))
Our code returns: False. Because John Appleseed did not win the latest Indy 500 race, the variable top_10_ranking
is empty. So, because the string is empty, our program evaluates to False.
Python All
all()
is a built-in Python function that returns True when all items in an iterable object are True, and returns False otherwise. In addition, if the iterable object is empty, the all()
method will return True.
The syntax for the all()
method is the same as the any()
method. all()
takes in a single parameter, which is the iterable object through which the all()
method should search. Here’s the syntax for the all()
method:
all(iterable_object)
all()
returns True when:
- All values are equal to True
- The iterable object is empty
Otherwise, all()
returns False.
All() With an Array
Let’s walk through an example of how all()
can be used in Python. Say that we have a list of Boolean values which store whether or not John Appleseed has ranked in the top 10 in any Indy 500 race.
If we want to find out whether he has ranked in the top 10 in all of his races, we could use the all()
method. Here’s an example program that uses all()
to check if John Appleseed has ranked in the top 10 in every one of his Indy 500 races:
race_wins_indy_500 = [False, False, True, False] print(all(race_wins_indy_500))
Our code returns: False. On the first line, we declare a list that stores whether or not he ranked in the top 10 in an Indy 500 race. Then, we use all()
to check whether every value in the list is equal to True. In this case, only one value is equal to True, so our program returns False.
All() With a Dictionary
Say that we have a dictionary that stores John Appleseed’s 2018 Indy 500 race record. We want to know whether or not every value in the dictionary evaluates to True. To find this out, we could use the following code:
indy_500_2018_john = { 'driver_name': 'John Appleseed', 'race_year': 2018, 'top_ten': True } print(any(indy_500_2018_john))
Our code returns: True. Because every value in the dictionary evaluates to True — there are no blank or False values in the dictionary — our code returns True.
All() With a String
Similarly, we could use all()
on a string using the same syntax as we did for the any()
method.
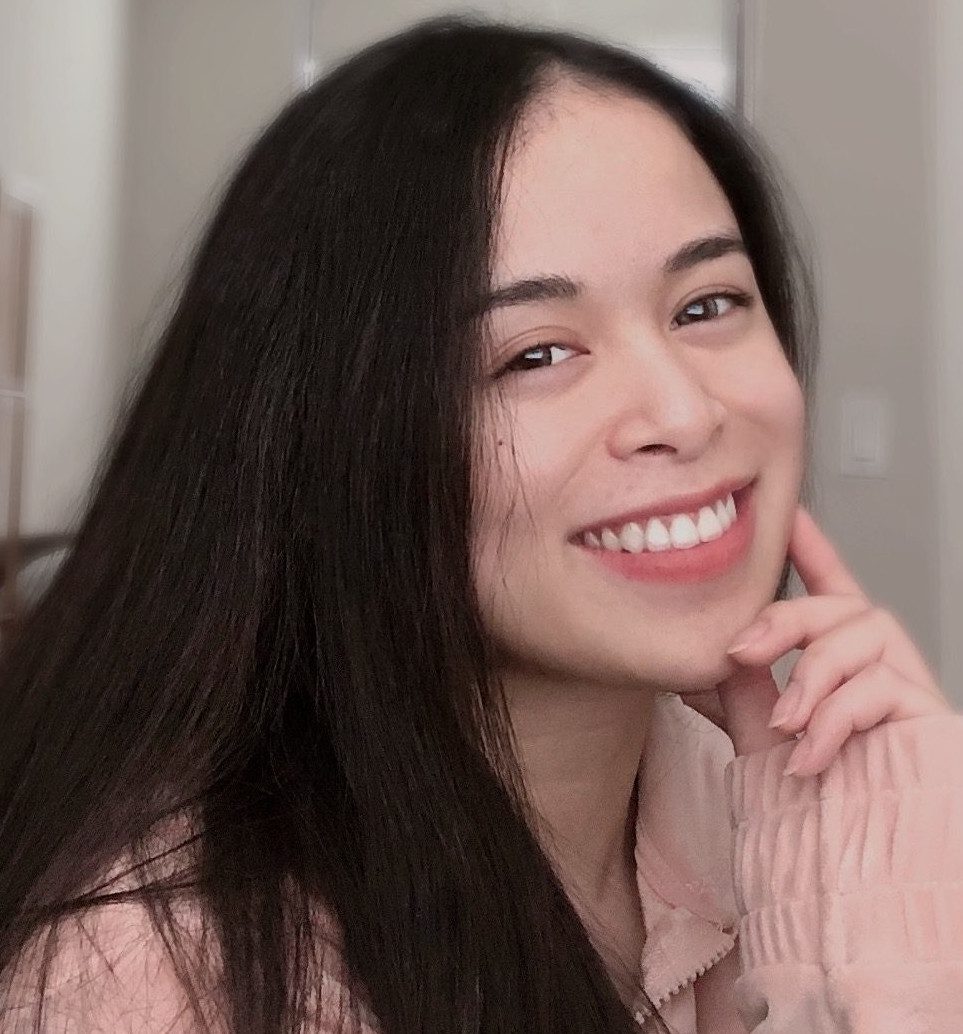
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Say we have a string that stores the name of the racer we are analyzing. If we wanted to check whether this string was empty, we could use the all()
method. As we discussed earlier, all()
returns False if a string is empty. Here’s an example of all()
being used to check if the name of the racer we are analyzing is empty:
racer_name = "John Appleseed" print(all(racer_name))
Our code returns: True. The string assigned to the variable racer_name
contains a value, which means that it evaluates to True when the all()
method is executed.
Conclusion
The any()
method can be used to check if any item in an iterable object evaluates to True. The all()
method can be used to check if all items in an iterable object evaluate to True.
This tutorial discussed how to use both the any()
and all()
methods in Python, and explored a few examples of these methods being used with different types of iterable objects. Now you have the knowledge you need to start using any()
and all()
in Python like a pro!
Are you interested in becoming a professional Python developer? Download the free Career Karma app today and talk with one of our expert career coaches about how you can get started on the journey toward your new career.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.