One of the most popular types of programming languages is OOP–that is, object-oriented programming. Much of the world runs on this paradigm. And if you’ve just started your tech career, you may be expected to know the answers to some OOP interview questions.
To start, let’s figure out what object-oriented programming languages are, then we will consider some of the most common OOP interview questions.
What is OOP?
OOP stands for Object-Oriented Programming, and it’s a type of programming paradigm. Languages that are object-oriented focus on objects rather than logic alone. Objects can hold both data and code, and in many OOP languages, almost everything is an object.
These objects can interact with each other, and with themselves. This is the counterpart to the other main branch of programming: functional programming. In functional programming languages, the focus is on the flow of information and has immutable variables.
OOP Interview Questions
Now that we have some foundation in what object-oriented programming is all about, let’s finally get to the list of OOP interview questions.
Name some object-oriented programming languages.
Here are some common object-oriented programming languages:
- Python
- C++
- Java
- Ruby
- JavaScript
What are the pillars of OOP theory?
The four fundamental concepts are:
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
What is abstraction?
With data abstraction, one can pull a small amount of relevant data from a larger amount of data. This allows you to see and use only the important information and leave some implementation details hidden out of the way.
What is encapsulation?
Encapsulation refers to the idea that you can bundle data with code, and it’s a central tenet of object-oriented languages. It also allows for data hiding so that only the object with secure information can access that secure information.
What is inheritance?
Inheritance is just what it sounds like. It allows objects and classes to copy data and functions from other parent classes. And it can serve as a template for objects and classes.
What is polymorphism?
Polymorphism is the idea that one thing can appear in many forms. For object-oriented languages, this means that once designed, an instance of code can be used more than once in more than one situation.
What is an object?
Objects are sets of both data and code, and function much like real-world objects. They can have both properties (like color, size, or weight) and a function (like stapling, heating, or typing).
What is a class?
A class is an object that serves as a blueprint for more objects. It gives the basic definition and fundamental components for the objects created with it and is one of the core parts that allow inheritance. For example ‘soda’ or ‘computer’ could be classes, while ‘Fanta’ and ‘Thinkpad’ could be objects under those classes.
What is a superclass?
A superclass—also called a base class—is a class that is a parent for more classes rather than objects. It usually contains the most basic code and data that will be used by every class and object under it. Using the above example, ‘beverage’ and ‘machine’ could be superclasses for ‘soda’ and ‘computer.’
What is a subclass?
A subclass is a class that falls under a superclass. It inherits from the superclass and is considered to have an “is-a” relationship with the superclass.
What are access modifiers?
Access modifiers affect the scope of a method or variable, and change what is allowed to see and modify these methods and variables. They include:
- Private
- Protected
- Public
- Friend
- Protected Friend
What is a sealed modifier?
Sealed modifiers are access modifiers that cannot be inherited. Sealed modifiers can also be applied to properties, events, and methods.
What is a constructor?
A: A constructor is a method used to create objects or classes, and initialize their state as required. It’s called at the time of the object’s creation.
What is a copy constructor?
A copy constructor is a special method that creates an object that is a copy of an object.
What is a parametric constructor?
This is a constructor that passes on certain defined parameters to the new object or class.
What is a destructor?
A destructor is a method called when an object is destroyed.
What is an inline function?
An inline function lets the compiler insert the entirety of a function wherever it is used in the uncompiled code.
What is a friend function?
A friend function is a function that is permitted to access public, private, and protected information in the class it is ‘friends’ with. This function must be defined inside the aforementioned class.
What is operator overloading?
Operator overloading adds additional functionality for operators specific to certain classes. This allows the same operator to be used in different cases and perform different functions.
What is function overloading?
Function overloading is much like operator overloading. It means creating a function that can be used in different situations and can have different parameters depending on where it’s used.
List the operators that cannot be overloaded
The following cannot be overloaded:
- Scope Resolution (represented by ‘::’)
- Member Selection (represented by ‘.’)
- Member selection through a pointer to function (represented by ‘.*’)
What is an abstract class?
An abstract class is a special type of class that contains one or more abstract methods and cannot create instances. Abstract classes cannot create objects, but they do allow for inheritance.
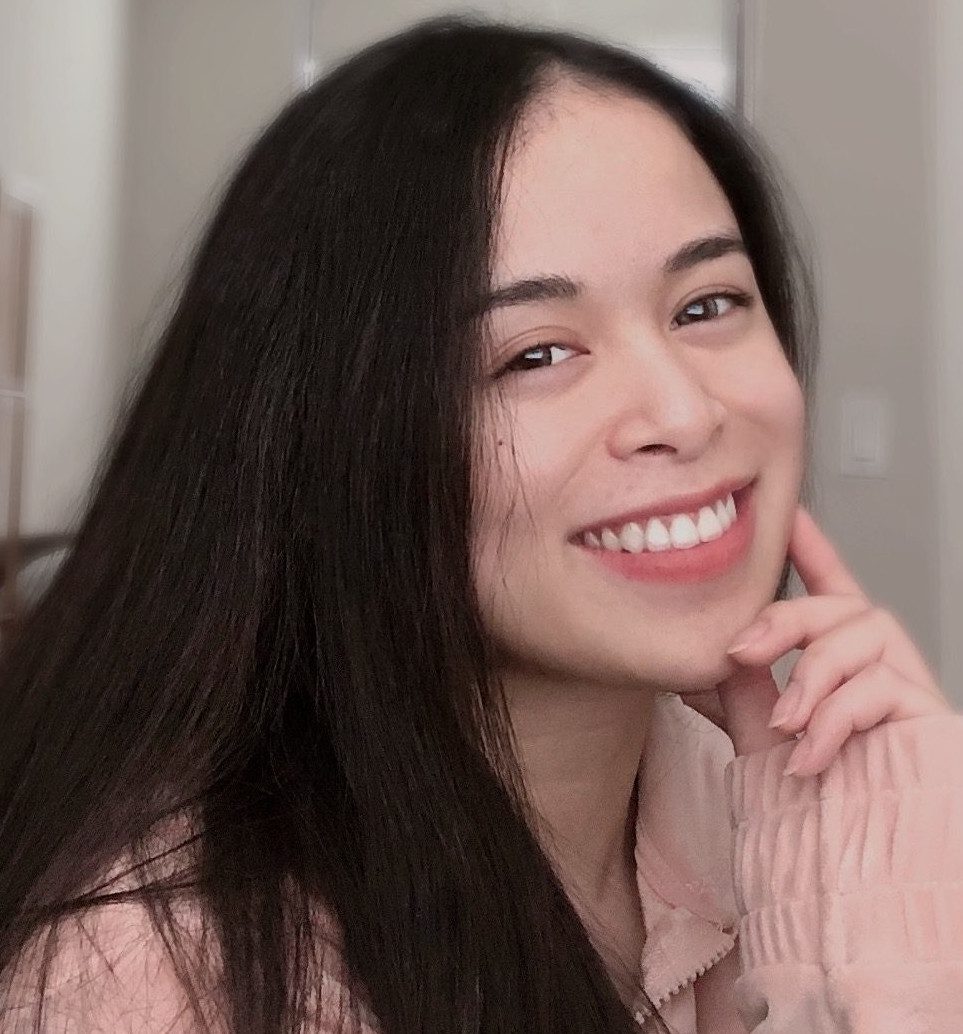
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
What is an interface?
An interface is a collection of one or more abstract methods.
What is the difference between a structure and a class?
A structure is used for grouping data and is public by default. Classes group both data and methods and are private. Classes are also used for inheritance and encapsulation, while structures are not.
What is a ternary operator?
A ternary operator is an operator that takes three arguments rather than two.
What is the finalize method?
The finalize method is used for cleanup operations at the end of your code and it saves memory by cleaning up unused resources. It’s protected and only accessible via this class or a subclass.
What is exception handling?
Exceptions are events that occur when the program runs. These events can be errors, runtime exceptions, etc. Exception handling handles these exceptions with try, throw, and catch keywords.
What is early binding?
Early binding is when variables are assigned values before the program is compiled and run: directly in the code.
What is late binding?
Late binding is when variables are assigned values after the program has been compiled: on or during runtime.
What is static binding?
Static binding is when the type of a variable is determined by compile time. These are usually variables that are explicitly set in your code
What is dynamic binding?
This is when the type of a variable is determined at runtime. This is usually done implicitly.
What is a pointer?
A pointer is a memory address that holds the exact location of the actual value in computer memory.
What is the use of the ‘this’ keyword?
‘This’ refers to the current class or object, as opposed to a global class or object.
What is a virtual function?
A virtual function is a member of its class and can be overridden in derived classes.
What is the ‘super’ keyword used for?
This is a special keyword that allows access to methods that have been overwritten.
What are tokens?
Tokens are instructions recognized by the compiler that cannot be broken down in any way. Some examples of tokens are keywords, constants, identifiers, string literals, and operators.
This is, of course, not a complete list of OOP interview questions. And good working knowledge is still required to start in any field that uses object-oriented programming languages. These are just a few questions to jog your memory and prepare you for some tricky questions that potential employers may ask you.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.