Object-Oriented Programming languages use objects that contain both data and code. The principles of object-oriented programming are encapsulation, abstraction, polymorphism, and inheritance.
Object oriented programming (OOP) is a programming model that centers around individual objects or data. Instead of using step-by-step instructions like in procedural programming, OOP allows programmers to treat variables, data structures, and functions as ‘objects’ that interact with one another.
OOP is designed to promote code reusability. Many programmers prefer using OOP languages to build complex applications because they can easily add or make changes without having to alter the existing code. Understanding how object oriented programming languages work and why they are useful can help you ace tech job interviews.
In this article, we’re going to take a look at what object oriented languages are and examine their pros and cons. We’ll also take a look at a shortlist of some of the most popular OOP languages in use today.
What Is Object Oriented Programming?
Object oriented programming is one of two primary programming paradigms, and is a way of looking at and accessing data. OOP is built around objects, which are data structures that contain both data (properties or attributes) and code (procedures or methods). In most OOP languages, almost everything is an object that can have both values and executable code.
The objects in OOP can be thought of as actual objects. They can have properties and functions. They also tend to follow a certain set of principles. Think of an object, like a watch. That watch has properties. It’s made of metal, black, and has a specific weight. It also displays time and can affect itself by spinning gears to change the position of its hands.
Now that we have looked at the definition of object oriented programming, or OOP, the section below will discuss some examples of OOP languages. We will also look at the fundamental building blocks of this programming paradigm and see what it means to use object oriented programming in real-world applications.
What Are Object Oriented Programming Languages?
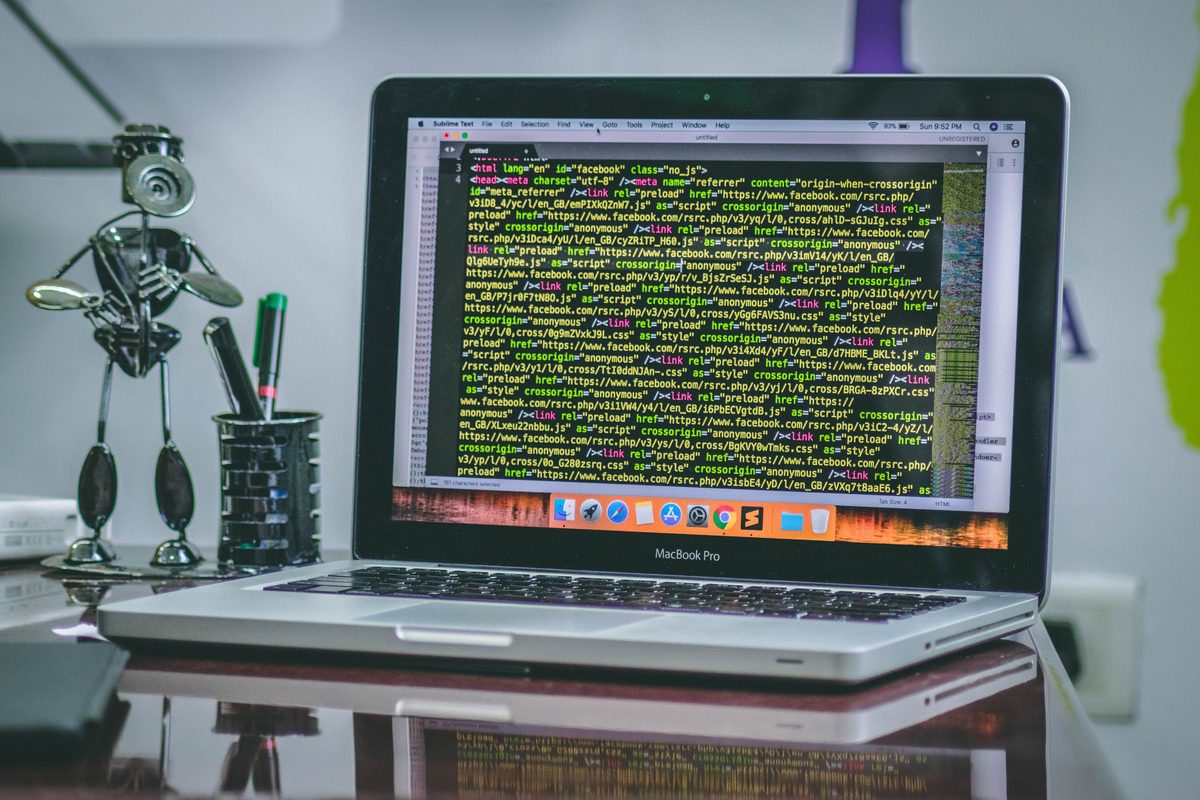
Object oriented programming languages are coding languages that support the object oriented style of programming. OOPs focus more on objects and their interactions rather than logic or functions. The following is a list of object oriented programming languages that are commonly used today.
List of Object Oriented Programming Languages
- C++
- C#
- Dart
- Java
- JavaScript
- Objective-C
- PHP
- Perl
- Python
- Ruby
Fundamental Building Blocks of Object Oriented Programming
Computers are powerful machines. With a computer, we can calculate numbers extremely quickly and produce amazing programs for many applications. However, to take advantage of this power, we need to communicate with the computer using something less painful than manually typing ones and zeros.
OOP aims to solve this issue by allowing programmers to break down items into smaller, more manageable objects that can be developed and tested separately. This not only simplifies the development process but also adds scalability to the project. To master object oriented programming, it is recommended that you start with the fundamentals of OOP as listed below.
Classes
Classes in object oriented programming serve as the blueprint for the entire structure of the code. Classes are data types that are defined by the developer. It can be viewed as one of the most crucial fundamentals of object oriented programming. An OOP class is a template that can be used to create objects, provide values to attributes, and implement methods or functions.
Objects
In this type of programming, an object is an instance, or a variation, of a class with clearly-assigned data. If you were to set the class to ‘animals’, an example of an object that would serve as an instance would be ‘cat’. An object can be attributed to real-world items or abstract entities.
Methods
Object oriented programming methods are procedures related to classes. They describe the behavior of an object. Put simply, a method is an action that an object can perform. A ‘person’ class could have methods like ‘eating’ or ‘singing’. An object oriented programming method is often compared to a function but they differ in that the latter is task-based.
Attributes
In OOP, attributes are characteristics that can be found inside a class or an object. Attributes can be used to differentiate one class from another. Using the same example of a ‘person’ class as the above, the possible attributes could be ‘height’, ‘age’, and ‘size’.
Object Oriented Programming Principles
There are four object oriented programming principles. These OOP principles, also known as OOP concepts, are common properties that define them and make them significantly more efficient. They are as follows.
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
Let’s explore these four principles of object oriented programming in greater depth.
The 4 Pillars of OOP
- Encapsulation. Encapsulation is one of the four pillars of OOP. It is the concept of binding data to functions that work as a security function to keep that data safe. For example, many OOP languages only give access to specific methods in the class owning the data. This is often done explicitly to keep certain data safe.
- Abstraction. In object oriented programming, abstraction is the idea that if you’re doing one thing too often, it should be its own entity. For example, if a programmer is repeatedly rewriting the same function for different objects, that function could be abstracted to become its own entity.
- Inheritance. Inheritance in OOP is what it sounds like. It is most often defined as a “is a” relationship. It lets certain classes be under the umbrella of other classes. These classes can inherit information and functionality from that class, while also being able to expand on that functionality. It lets us reuse code that we’ve already typed in other classes.
- Polymorphism. Polymorphism in OOP means ‘to take more than one shape’. It refers to the ability to handle objects differently depending on what they are. It allows us to define different methods for handling objects based on their derived class. For example, if we needed to find the size of two different objects, we can use polymorphism to create a function that can accept both objects and still get the appropriate measurements.
Object Oriented Programming Languages: Rundown of Top OOPs
Java, Python, C++, and C# are some of the most popular object oriented programming languages in the world. In fact, these four OOP languages dominated the latest TIOBE index which measures the popularity of programming languages based on metrics like the number of professionals and available resources. The full list of top OOPs is as follows.
- Java
- Python
- C++
- Ruby
- C#
- PHP
- TypeScript
Java
Java is a top OOP language, with OOP Java being one of the most used and in-demand languages of all time. Java’s motto is “write once, run anywhere” and that is reflected in the number of platforms it runs on. You can use Java for software, game, backend, and Android development, among others. Object oriented programming in Java shares the same fundamentals as other OOP languages.
Python
Python is an object oriented programming language with a strong foothold in machine learning, artificial intelligence, and data science. It is also a versatile and beginner-friendly language with a syntax that is often compared to English. With Python, OOP strongly contributes toward code reusability. This allows developers to maintain and manage code efficiently within a short period.
C++
C++ is a superset of C which means it has the speed of C but with added functionality. C++ is known for being a compiled, reliable, and powerful language. It’s even used to build compilers and interpreters for other languages. Object oriented programming in C++ helps ensure a smoother, more efficient development process.
Ruby
Ruby is another OOP language that was built for simplicity. With that said, Ruby is an incredibly powerful language. The creator of Ruby, Yukihiro “Matz” Matsumoto, has said, “Ruby is very simple in appearance, but is very complex inside, just like our human body.” It is commonly used with Ruby on Rails to build dynamic web applications.
C#
C# is an object oriented programming language designed by Microsoft. It was designed to improve upon existing concepts in C. C# powers the Microsoft .NET framework alongside many web apps, games, desktop apps, and mobile apps. Some of the most common OOP concepts in C# are encapsulation, inheritance, and polymorphism.
PHP
PHP is an OOP language that is considered to be a server-side scripting language. PHP can be embedded into HTML and used to build dynamic web apps. PHP, which stands for Hypertext Preprocessor, is also said to be one of the easiest programming languages to learn. However, note that PHP does not support all object oriented features such as multiple inheritances.
TypeScript
TypeScript is a superset of JavaScript with static typing. It is designed to help developers launch large scalable projects and web applications with ease. Because TypeScript is built upon the concepts of JavaScript, which is another language that supports OOP features, it can be said that TypeScript is object oriented to a certain extent.
Is JavaScript Object Oriented?
JavaScript is object oriented but it is not a class-based language like most OOP languages. You may find this confusing especially since classes are said to be one of the key characteristics of OOP. However, there isn’t a standard specification that a language should ‘follow’ to be OOP as long as it uses an object-focused model and supports modularity and code reusability.
According to most developers, JavaScript ticks these boxes. Technically, JavaScript is a prototype-based language, which is a style of OOP. Instead of using classes to define objects, object oriented programming in JavaScript allows you to use a constructor function and reuse it.
Advantages of Object Oriented Programming Languages
The most popular advantages of object oriented programming are its ease of use and security. Many professionals found that they can build large, scalable, and secure projects in a short period of time when using a language that supports object oriented programming. Here are other benefits of OOP that can show you why object oriented programming is worth learning.
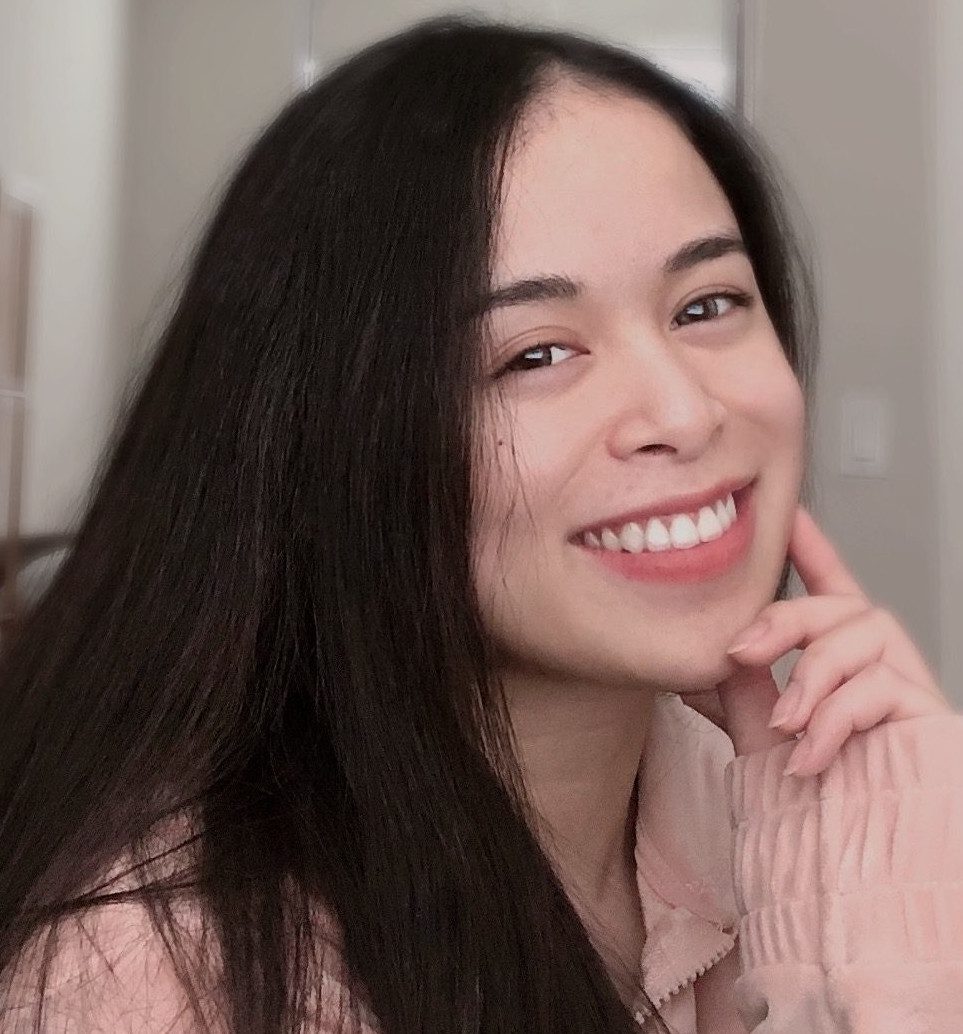
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
- Reusability. Code reusability is at the heart of object oriented programming. Because of polymorphism and abstraction, you can make one function that can be used over and over again. You can also copy information and functionality that’s already been written with inheritance. This saves time and reduces complexity.
- Parallel Development. There is enough groundwork for parts of the program to be developed separately from each other and still function under object oriented principles. This makes concurrent development much easier for larger development teams.
- Maintenance. OOP code is much easier to maintain. Instead of having to individually fix a hundred different instances where a function is called, you can fix one modular and polymorphic function. This is a huge advantage of OOP.
- Security. Object oriented languages are often favored because of their built-in security features. With encapsulation, you bind data to functions, which limits access to data. Unless specified, other methods and classes cannot access private data by default.
- Modularity. Object oriented programming languages break down an application into objects and classes. This is a major benefit of object oriented programming because it gives your application a more modular structure. Modular code is easier to read, write, and manage.
Disadvantages of Object Oriented Programming Languages
While OOP languages can be powerful, they aren’t necessarily useful for every situation. As such, knowing what and how you want to code remains a priority. This list highlights some of the disadvantages of object oriented programming for you to consider.
- Often Messy. Because object oriented languages are so customizable and scalable, it can be easy to lose an understanding of how the code works. OOP code can function in many ways. There are many methodologies for programming in OOP that don’t work well with other methodologies, are inefficient, or are difficult to use.
- Requires More Planning. OOP languages are modular. As such, going in without a clear design ahead of time is a recipe for disaster. Creating an efficient program requires a solid plan, more so than with other programming paradigms.
- Opacity. This is as much a pro as it is a con. Objects and functions can operate independently. They can take in information and usually return reliable results. As a result, they can end up being black boxes, meaning that what they do isn’t always clear.
- Performance. Object oriented languages often take a performance hit. Programs made in OOP languages are often larger and require more computational effort to run than functional languages. However, this isn’t always true or important. C++, for example, is an OOP language, but it’s one of the fastest languages available. The difference in performance only becomes apparent in instances where extreme speed is required.
What Is the Best Way to Learn Object Oriented Programming Languages?
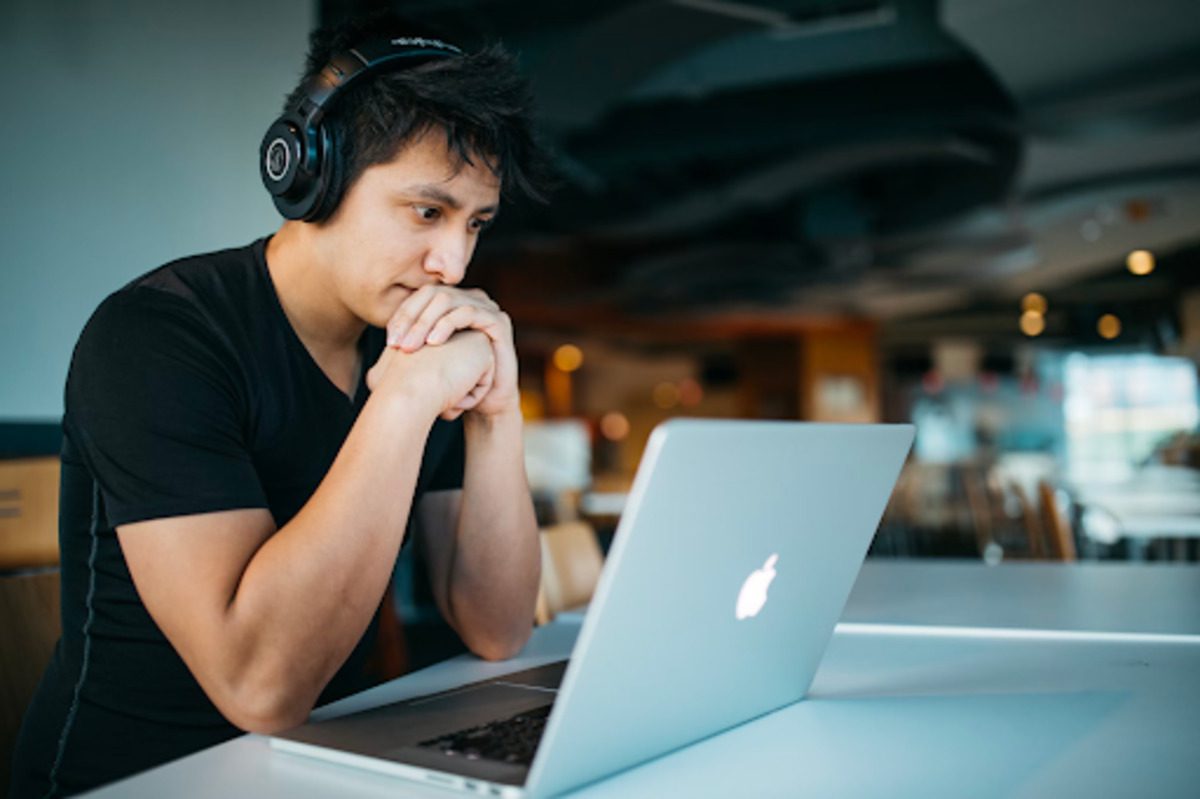
One of the best ways to learn object oriented programming is by going to a coding bootcamp. Coding bootcamps offer career-focused training that can help you acclimate to coding in the real world. They also offer immersive, cost-efficient programs that can be completed in a short amount of time. This is a great stepping stone for those looking to break into tech.
There are other ways for you to master object oriented programming. If you want to learn OOP online, you can check out video tutorials, short-term training, or intensive courses offered by many massive open online course (MOOC) providers. Ultimately, the best choice for you would depend on the language you wish to learn, your preferred learning style, and your career goals.
Now you have a firm grasp of what an object oriented programming language is and what it’s good for. The next step is to choose a language to learn. Because object oriented programming languages are popular among industry professionals, you will also have access to a huge ecosystem of supportive communities that can offer additional support.
Object Oriented Programming Languages FAQ
There are five types of programming languages, four of which are more commonly used today. The four popular types of programming languages are procedural programming, functional programming, object oriented programming, and scripting programming languages. The different types of coding languages follow different programming paradigms, each of which is better suited for different projects and applications.
How long does it take to learn object oriented programming?
It doesn’t take long to learn object oriented programming, especially if you already have some coding experience. Even if you are new to coding, you can learn object oriented programming fast by choosing a beginner-friendly language that supports object oriented programming like Python. It is said that Python’s English-like syntax makes it easy to read, write, and maintain.
What is the difference between object oriented programming and procedural programming?
The difference between object oriented programming and procedural programming is the paradigm that guides the structure of the code. Object oriented programming (OOP) leans on objects and the way they interact, while procedural programming uses a set of variables that follow a step-by-step approach. Choosing between procedural programming vs object oriented programming depends on the project that you are trying to build.
What was the first object oriented programming language?
Simula is widely accepted as the first object oriented programming language, invented by Ole-Johan Dahl and Kristen Nygaard in 1967. However, C++ is considered one of the first widely-used object oriented programming languages in the modern world.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.