A list is a type of data that contains an ordered sequence of elements. Lists are a useful data type because they allow you to store multiple related values in one variable. With a list, you could store the names of 10 pairs of shoes in one variable; you could store a list of purchases you have made at a store in one variable.
While the built-in list data type is already powerful, for more advanced use cases you may find it lacking. That’s where NumPy’s array data type comes in. The NumPy library can be used to create arrays with multiple dimensions with ease.
In this guide, we’re going to talk about what NumPy arrays are, why they are useful, and how you can work with them in your code. Let’s get started!
What is a NumPy Array?
A NumPy array is the array object used within the NumPy Python library. NumPy, which stands for Numerical Python, is a package that’s often used for scientific and mathematical computing. It is accompanied by a range of tools that can assist with data analysis and advanced math.
In vanilla Python (Python without any external packages), arrays are powerful but they can be slow to process. NumPy arrays, on the other hand, aim to be orders of magnitude faster than a traditional Python array.
This performance boost is accomplished because NumPy arrays store values in one continuous place in memory. This makes it easy for Python to access and manipulate a list.
How to Declare a NumPy Array
To get started, let’s set up a NumPy array. For this tutorial, we’re going to store string values in our array. These string values are a list of the sweet treats offered at a local coffee shop. We’ll start by importing the NumPy library:
import numpy as np
This line of code imports numpy
from Python and assigns the library the name np
. This means that whenever we need to work with our array we only need to call np
.
Next, we’re going to declare our array using the array interface:
treats = np.array(["Blueberry Muffin", "Cinnamon Bun", "Jammy Shortbread"]) print(treats)
Our array contains four string values. Like a traditional Python array, we have enclosed all the items in our list in square brackets. To declare a NumPy array, we’ve used the array method that’s part of np
. This creates an ndarray
object, which is the built-in NumPy array type.
Our code returns a copy of our original array, sored as a NumPy array:
['Blueberry Muffin' 'Cinnamon Bun' 'Jammy Shortbread']
That’s it: we now have an array that we can work with.
NumPy Arrays: Dimensions
When we talk about dimensions in NumPy, we don’t mean new worlds like you would see in the movies. A dimension in an array is one level of depth within that array. When the term dimension is used, it refers to nested arrays. These are arrays that contain arrays.
An array can have any number of dimensions. Most of the arrays that you’ll work with will either be 1-D, 2-D, or 3-D arrays. The “D” stands for dimension.
NumPy 1-D Array
In our first example, we created a 1-D array. This is an array that contains 0-D arrays (or items) as its elements. Most of the arrays that you’ll work with will be 1-D.
Let’s create an array which stores the prices of the treats at the coffee shop:
import numpy as np prices = np.array([1.95, 2.00, 2.05]) print(prices)
Our code returns an array in one dimension, storing our values: [1.95 2. 2.05].
To access an element from a 1-D array, you can use the same syntax as you would with a Python list. Let’s retrieve the second item in our list:
print(prices[1])
Our code returns the item with the index value 1, which is: 2.
To learn more about Python arrays, read our beginner’s guide to Python arrays.
NumPy 2-D Array
Not all arrays are 1-D. Suppose that we wanted to store an array which contained two arrays. One array stores the sweet treats sold at the coffee shop; the other array stores a list of coffees sold at the shop. Together, these are part of a menu items
array.
Let’s create this array using NumPy:
import numpy as np menu_items = np.array([ ["Blueberry Muffin", "Cinnamon Bun", "Jammy Shortbread"], ["Cappuccino", "Espresso", "Mocha"] ]) print(menu_items)
The resulting array is:
[['Blueberry Muffin' 'Cinnamon Bun' 'Jammy Shortbread'] ['Cappuccino' 'Espresso' 'Mocha']]
Our newly-created array has two dimensions. The first array within our array contains a list of sweet treats; the second array contains a list of coffees. Notice that both of these arrays are enclosed within a pair of square brackets which connects the two arrays.
Retrieving items from a 2-D array works slightly differently in NumPy than it does in Python. To access elements from a 2-D array, you need to separate the index numbers of the value that you want to retrieve from the array.
Consider this code:
print(menu_items[0, 2])
Our code returns: Jammy Shortbread. We’ve retrieved the item with the index value 2 that is stored inside the array with the index value 0. In this case, we’ve retrieved the last item in the array which stores the sweet treats sold at the coffee shop.
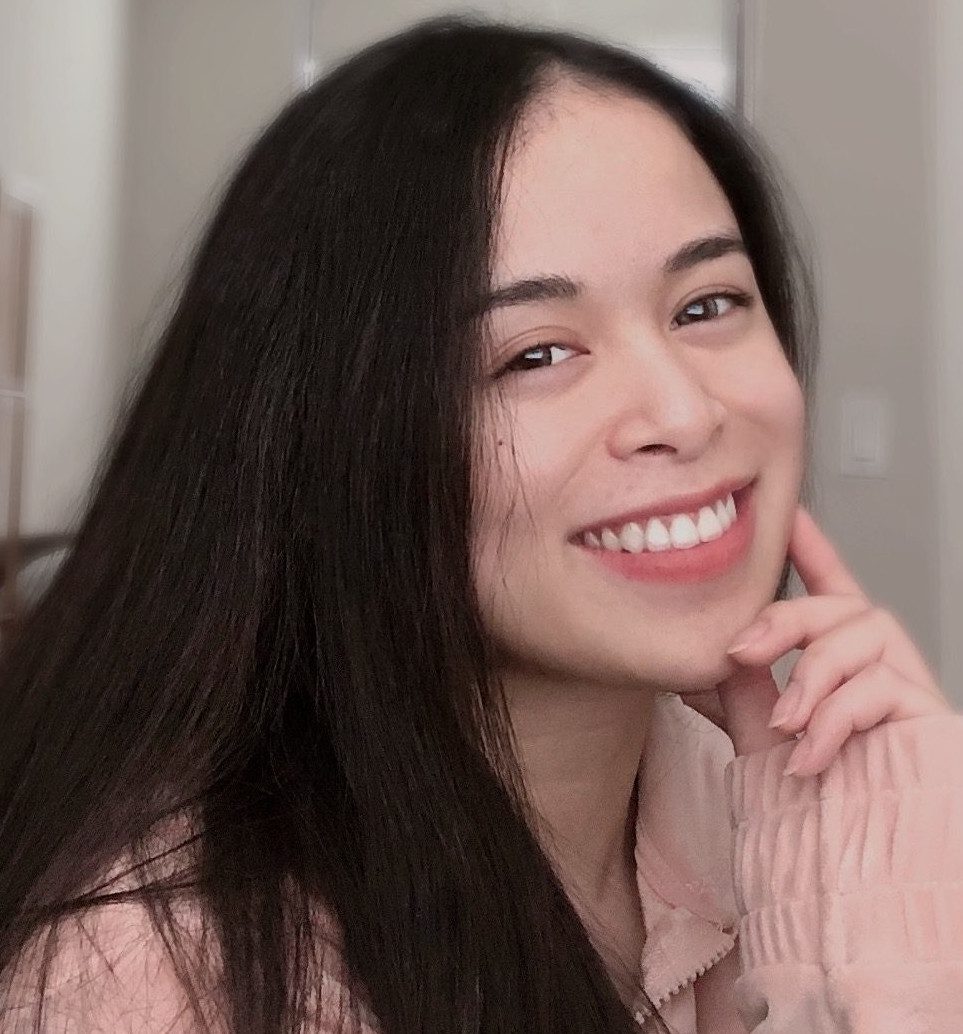
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
NumPy 3-D Array
Let’s add in another dimension! NumPy arrays can contain 3-D arrays. This is an array which contains 2-D arrays.
Suppose that we want to store the following values:
- Sweet and non-sweet food offerings (paired, but in separate arrays); and
- Caffeinated and non-caffeinated drinks (paired, in separate arrays);
All of these values should be stored within one array. The dimensions of this array are:
- 1-D: All menu items
- 2-D: Sweet and non-sweet foods, caffeinated and non-caffeinated drinks
- 3-D: Sweet foods, non-sweet foods, caffeinated, non-caffeinated drinks
Let’s create this array using NumPy. Paste in the following code into a Python file:
import numpy as np menu_items = np.array([ [ ["Blueberry Muffin", "Cinnamon Bun", "Jammy Shortbread"], ["Smoked Bacon Roll", "Tuna Melt Panini", "Cheese and Tomato Toastie"] ], [ ["Cappuccino", "Espresso", "Mocha"], ["Apple Juice", "Water", "Orange Juice"] ] ]) print(menu_items)
Our code returns:
[[['Blueberry Muffin' 'Cinnamon Bun' 'Jammy Shortbread'] ['Smoked Bacon Roll' 'Tuna Melt Panini' 'Cheese and Tomato Toastie']] [['Cappuccino' 'Espresso' 'Mocha'] ['Apple Juice' 'Water' 'Orange Juice']]]
We’ve created a 3-D array with all of the information we discussed earlier. This array is a comprehensive list of all the menu items offered by the coffee shop.
Accessing items from a 3-D array works similarly to the syntax you use to access items from a 2-D array. The difference is that you’ve got to specify a third index number to retrieve an item from a 3-D array. Let’s retrieve “Mocha” from our array:
print(menu_items[1, 0, 2])
Our code returns: Mocha.
1 is the index number of the 1st dimension we want to access (1 corresponds with our drinks); 0 is the index number of the 2nd dimension (0 corresponds with the caffeinated drinks); 2 is the index number of the 3rd dimension (2 corresponds with Mocha).
Count the Dimensions in an Array
NumPy arrays can start to look pretty complicated when you start adding new dimensions. We haven’t even explored arrays that have more than three dimensions! Luckily for you, there’s a handy shortcut you can use to calculate how many dimensions an array has.
Paste in the following code into a Python file:
import numpy as np menu_items = np.array([ ["Blueberry Muffin", "Cinnamon Bun", "Jammy Shortbread"], ["Cappuccino", "Espresso", "Mocha"] ]) print(menu_items.ndim)
Let’s run our code. The value “2” is returned. This tells us that our array contains two dimensions, which we can see is true by analyzing our above array.
Conclusion
NumPy arrays are a flexible way of storing similar values. They are faster and more efficient than traditional Python arrays. You can work with multiple dimensions using NumPy arrays with ease; this is more difficult to do in vanilla Python.
Now you’re ready to start using NumPy arrays like an expert programmer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.