New features have been added to the JavaScript language with the release of ECMAScript6 (ES6). One such feature affects how we declare variables. With the addition of let
and const
, came all sorts of questions about when to use each of the variable declarations.
In this post, we talk about each of the options, with respect to their definition and use in the JavaScript environment.
Pre-ES6: Var
Prior to the release of ES6, the keyword used to declare variables was var
.
var hello = "Hello, World"; console.log(hello);
Vars can also be redeclared and updated with no errors.
var hello = "Hello, World"; console.log(hello); //Hello, World var hello = "Hello, Mars"; console.log(hello); //Hello, Mars
The first problem with var is evident: redeclarations can cause bugs in your code if a variable has already been assigned to the same name. There can be unintended consequences if something is redeclared and you didn’t realize that variable name had already been used earlier in the codebase. Along with this, too, comes some other idiosyncrasies when it comes to scope, and hoisting.
Scope
Scope tells us what variables are available for us to use – we have variables that can be globally or locally scoped. What happens if we have the same variable declaration, but one is global and the other is local?
var hello = "Hello, World"; console.log(hello); //Hello, World function helloWorld(){ var hello = "Hello, Again!"; console.log(hello); //Hello, Again! return hello; } helloWorld() console.log(hello); //Hello, World
When we have our var declared outside the function, it’s globally available to us global-scoped. When “hello” is declared inside the function, it is only available to the function – function- or local-scoped.
When we have a variable declaration that is the same, but the scope is different, the var inside the function does nothing to change the assignment of the global var, even with the invocation of the helloWorld()
function. Because the var is declared inside the function with the name “hello”, it doesn’t look to the global scope for the other definition.
Hoisting
When a variable is hoisted in JavaScript, the declaration of functions and variables are moved to the top of their scope prior to code execution. The variable is declared but not initialized, so the initial value of the variable is undefined.
console.log(hello); //undefined var hello = "Hello, World";
Here, “hello” is hoisted and declared at the top of your scope. So essentially, the computer is interpreting the code as this:
var hello; console.log(hello);//undefined var hello = "Hello, World!"
The JavaScript engine sees that hello exists but it doesn’t know what hello is defined as until the next pass through.
Ultimately, the developers that helped to create the ECMAScript Standard realized that there were some little problems with var that could cost time and money. They then set out to create stricter keywords. This led to the creation of “let” and “const” in ES6.
ES6: Let
The let
keyword is very similar to the var keyword in many ways. The main differences lay within how errors are returned and how each keyword is scoped.
let hello = "Hello, World"; console.log(hello);
Variables declared and initialized with the let
keyword can be reassigned, but they can not be redeclared.
let hello = "Hello, World"; hello = "Hello, Mars!"; //this does not throw an error console.log(hello); let hello = "Hello, Again!"; //this will throw an error
When you run the code above in a JavaScript console, the last line will throw an error:
SyntaxError: Identifier 'hello' has already been declared
Unlike var
, declaring variables using let
will not allow for a declaration to the same variable name again. You can, however, reassign it if you wish, as shown in the example above.
Scope
In regards to scope, let
is very similar to var
. In addition to the rules, being globally- or locally-scoped gives us, there is an added constraint with let
. Variables declared inside a block of code are only available to that block of code. This is similar to functional scope because functions are blocks of code that can be carried even further by having separate blocks inside that function.
let hello = "Hello, World"; function helloWorld(){ if(hello) { let hello = "Hello Inside Block" console.log(hello, "inside block"); //Hello Inside Block inside block } console.log(hello, "outside block")//Hello, World outside block } helloWorld()
There is no error in this code since both instances of hello are treated as separate variables since they have different scopes.
Hoisting
Hoisting is another area where let and var declarations are similar. “var”, when hoisted to the top, is initialized as undefined. However, “let” throws a Reference Error if you try to use it before it is initialized.
console.log(hello); //ReferenceError: Cannot access 'hello' before initialization let hello = "Hello, World";
Know that the let keyword is the better choice in terms of syntax. This is because errors are thrown where you might try to redeclare a variable or use it before the initialization process.
ES6: Const
The const
keyword has even stricter guidelines than the let
keyword. With const
, variables cannot be redeclared or reassigned. A TypeError is thrown if you try to reassign to a const
.
const HELLO = "Hello, World"; HELLO = "Hello, Mars"; //TypeError: Assignment to constant variable.
That being said, when working with objects or arrays, properties of the object or array can be updated. As long as the basic structure of the object or array doesn’t change, you can always update it.
const business = { "id": 1, "name": "Brainsphere", "website": "http://walmart.com", "email": "mhallad0@narod.jp" } business.email = "info@brainsphere.com"; //this is okay since we are updating property business.website = "http://brainsphere.com"; console.log(business); business = { // this is not okay since we are trying to reassign the entire object "id": 2, "name": "Walmart", "website": "http://walmart.com", "email": "info@walmart.com" } console.log(business);
As shown in the example, reassigning business to a new set of properties results in a TypeError: Assignment to constant variable.
error. Consts cannot be reassigned or redeclared except in cases where you update the individual property in an object.
Scope and Hoisting
Just like the let
keyword, const
is block-scoped and is not initialized when hoisted so it will throw an error if you try to use it prior to initialization.
The const
keyword is great to use until you can’t use it anymore. This is when you need to reassign or update your variable.
Conclusion
In this article we went over the differences between let
, var
, and const
. Out of all of the keywords available to us now, it’s preferable to not use var
unless you must. Use let
or const
instead.
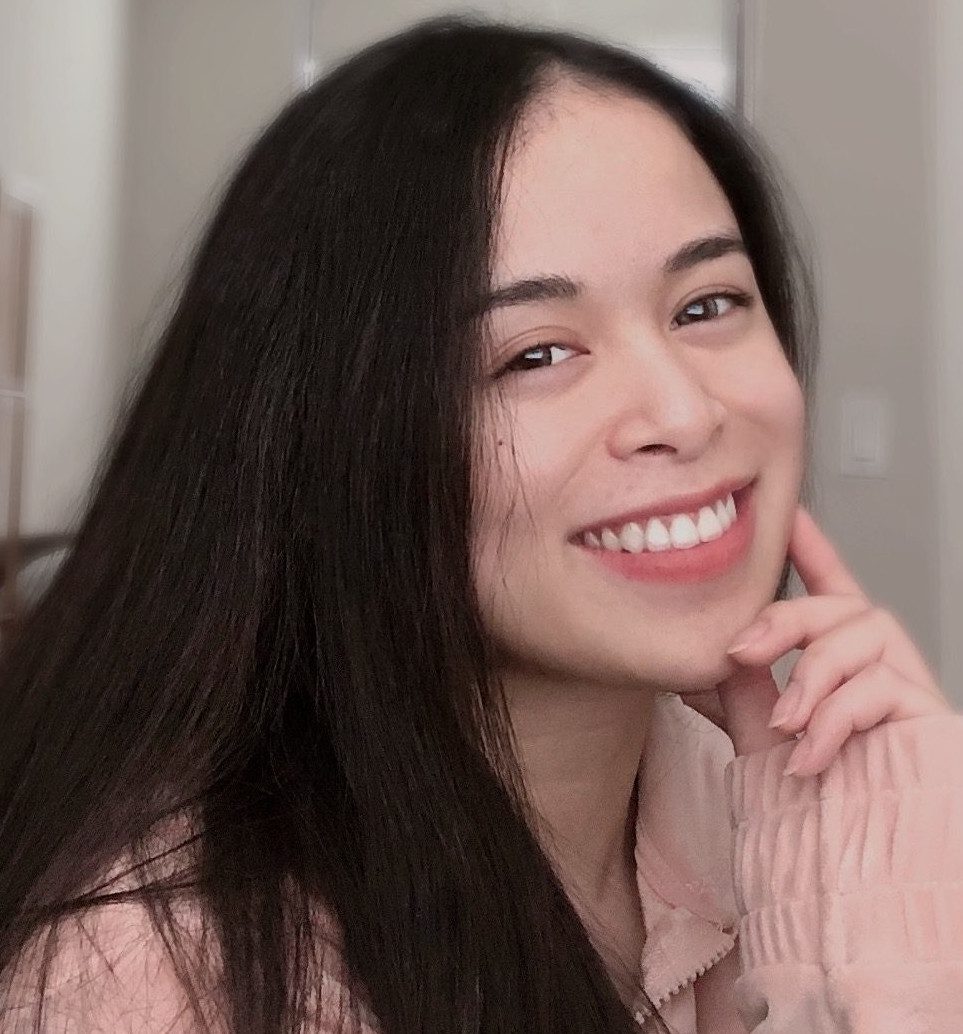
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.