Software developers commonly use design patterns when architecting a new application or website. A high-level design pattern is a very general, reusable template utilized to solve a problem.
This article takes a look at the model-view-controller (MVC) design pattern: what it is, why it might be useful, and how to get started when learning how to architect MVC applications.
What is the MVC Design Pattern?
If there is one thing to remember about this design pattern, it’s that the MVC design pattern requires each individual component to work independently to contribute to the design pattern’s overall purpose: to create awesome graphical user interfaces.
The model-view-controller design pattern was introduced by Trygve Reenskaug in the 1970s when worked for Xerox. MVC was later solidified as a user interface paradigm by two computer scientists who wrote an article on the subject in The Journal of Object-Oriented Programming in 1988.
There are three main components to the MVC Framework:
- Model
The model is purely the application logic. It is not part of the user interface (UI), but it is the template for what the UI will present. Think of this as a database, the schema for the database, and the rules for how to add objects to the database. - View
The view of the application is probably exactly what you think: the physical representation of our data on a screen. This could be a graph or table of some sort, for example. - Controller
The controller is the traffic cop of your operation. When a frontend talks to the backend, a controller receives a message that will tell the backend how to interact with the model layer.
What is MVC Used for?
MVC is used to accelerate the development process. A template for you to follow when creating web applications saves the overwhelming problem of figuring out “how to get started”. We turn to these patterns to cut down on the amount of time it would take to solve the problem.
While each individual component of the MVC design pattern does rely on each other to overall work, they are independent blocks of code. The separation of concerns makes code cleaner, shorter, and more reusable.
It also makes the logistics of working on one application with multiple developers by allowing developers to work on different features without having to worry about stepping on another’s code.
Learning MVC
Learn the model-view-controller pattern if you intend to become any sort of web developer. Knowing about this design architecture will make solving problems easier. It is one of the most popular ways to architect a web application out there.
How Long Does It Take to Learn the MVC Design Pattern?
How long it takes to learn is a matter of thinking about the solution to a problem in a different way — if you already know how to code. You can learn the MVC design pattern as you learn how to code. Be aware of the architecture and how it should be laid out as you learn what it takes to put an application together.
Your mileage may vary depending on the time and consistency in your practice.
How to Learn MVC: Step-by-Step
I recommend following a path that walks through how a user’s interactions changes to an application to learn this architecture.
If you are a code newbie, I recommend you learn at least one programming language first. JavaScript, Python or C# are great options for you to learn so you can advance to exploring the model-view-controller while you build projects.
- User
- How does a user interact with a web page?
- How do these user events work in the language you are using this paradigm in?
- Things to help you get started: HTTP Requests, JavaScript event loop, fetch API, axios, etc.
- Controller
- What are the main parts of a controller?
- When the controller receives a request, what happens next?
- Model
- What is a model and what does it do to control the database?
- When the model receives instructions to update the database, what happens next?
- View
- The view receives information from the model that the database has been updated
- The view changes what is seen on the screen for the user to see as a result of the original user interaction.
The Best MVC Courses and Training Programs
Everyone learns a little bit differently. Compiled here are some of the best MVC tutorials and training that could be found. Many of them use the more popular MVC user paradigm frameworks. Some courses and training have a fee, but there are a few that are free as well. This list is in no particular order.
Online MVC Courses
Here are some of the best courses available online that teach MVC as part of the course:
NodeJS – The Complete Guide (MVC, REST APIs, GraphQL, Deno)
- Udemy
- 40.5h of video lectures
- Prerequisites: General knowledge of how the web works is recommended and basic JavaScript knowledge (NO NodeJS knowledge is ok)
- $9.99–$94.99 (Buy when on sale!)
Maximilian Schwarzmüller’s course on NodeJS is a comprehensive look at how to construct a website using a model-view-controller paradigm. You only need a basic understanding of JavaScript to get started. Maximillian does a thorough job in teaching the fundamentals you need to get started.
ASP.NET MVC 5 Path: Beginner Courses
- PluralSight
- 2 courses:
- ASP.NET MVC 5 Fundamentals
- The MVC Request Life Cycle
- Prerequisites: Basic web development, familiarity with the C# language and ASP.NET framework
- Requires membership to PluralSight (Watch out for free preview weekends!)
These courses by PluralSight will give you an introduction to the ASP.NET framework and the MVC Design Pattern. In addition, PluralSight offers more advanced courses that build on these foundational ones.
PHP: The Complete PHP MVC Course
- Udemy
- 24.5 h of on-demand video
- Prerequisites: Basic Understanding of PHP OOP
- $9.99–$94.99 (Find it on sale!)
This course, taught by Terry Osayawe, teaches you how to master modern PHP MVC core development step-by-step by building your first e-commerce store.
Free MVC Courses
PHP MVC Framework Tutorial
- Guru99
- Self-paced
- Prerequisites: Basic PHP
- FREE
This is a free tutorial in PHP from Guru99. The tutorial covers the basics you need to know about MVC as you create a CodeIgniter application — a popular PHP MVC Framework.
Struts 2 Tutorial
- Tutorialspoint
- Self-paced Walkthrough
- Prerequisites: basic understanding of Java
- FREE
Apache Struts 2 is an elegant, extensible framework for creating enterprise-ready Java web applications. This tutorial is an explanation of what the MVC design pattern is and how to construct a web application around that idea.
How to Build and Structure a NodeJS MVC Application
- Sitepoint
- Self-paced Walkthrough
- Prerequisites: basic understanding of JavaScript
- FREE
Sitepoint walks you through a free tutorial on how to implement an MVC application structure on a NodeJS application. It is complete with code examples and explanations.
MVC Books
There are several books to assist in your MVC adventure as well.
‘Pro ASP.NET MVC 5
This guide on ASP.NET MVC 5 teaches you about the model-view-control pattern before progressing to more advanced topics. Freeman describes everything you need to know to use ASP.NET MVC 5 in good detail and puts the topic to practice by prompting you to create an e-commerce site.
‘CodeIgniter: Learn CodeIgniter in One Day’
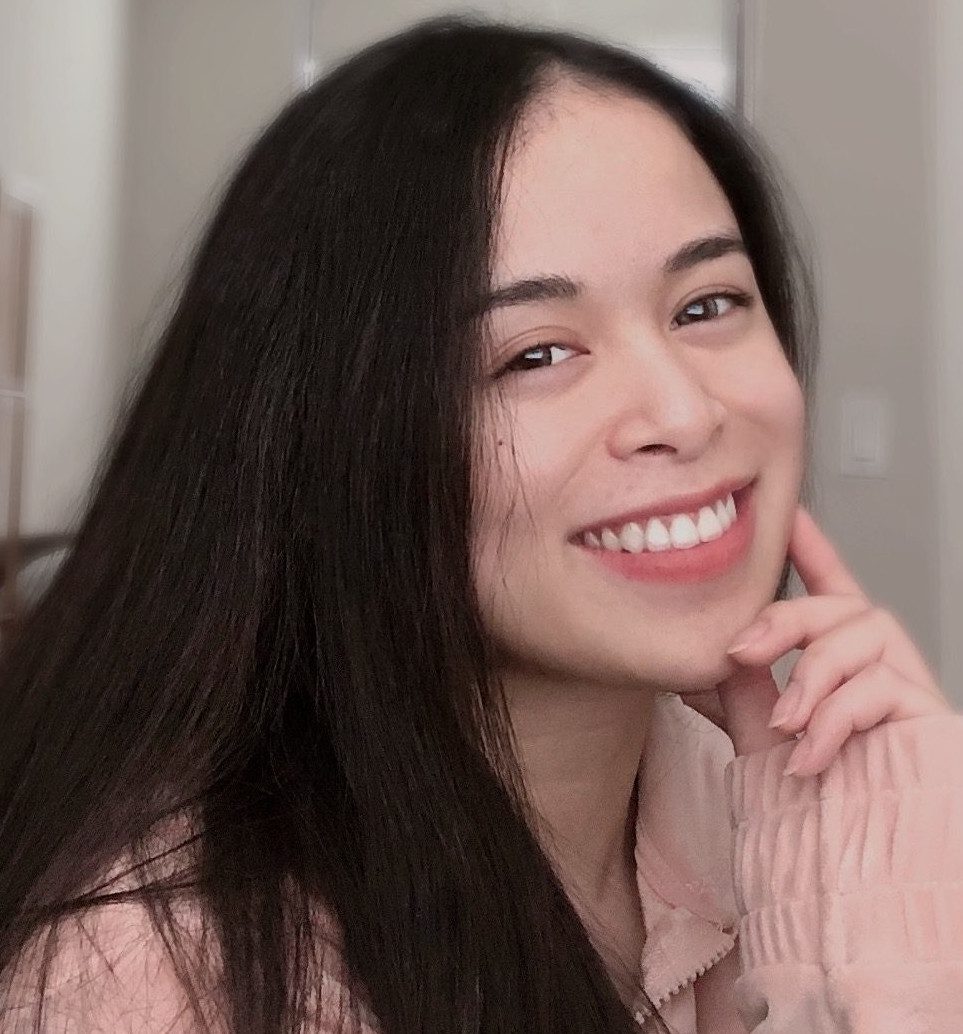
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
This is a step-by-step guide to using CodeIgniter — an MVC framework — to create PHP applications. The author takes the time to describe in detail what CodeIgniter is, what its purpose is, and how it intertwines with the MVC architecture to create a web application.
Online MVC Resources
This is a list of some of the decent resources that are available to you to learn MVC or to refer to later as you gain more experience.
Understanding the MVC Pattern in Django
Django is a Python framework that builds on the idea of the MVC user paradigm and expands on it to create its own pattern called the model-template-view. This resource goes over the MVC pattern and how it relates to Django.
ASP.NET MVC Patterns
You as a learner must refer to documentations on new topics when first getting started. Even if you don’t understand the language here, Microsoft does a pretty good job explaining what the purpose of the MVC pattern is.
Why Should You Study MVC?
Studying the model-view-controller pattern will benefit you if you plan to become a web developer or want to work for a company that uses this paradigm as a part of its solution stack. You should learn the basic concepts of the MVC user paradigm to become a better developer.
Good luck on your journey to become a web developer or software engineer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.