How to Learn Writing Algorithms
Writing algorithms can be overwhelming for even the most seasoned developer. Many of them memorize all of the different approaches to a particular problem. However, what if we instead took a look at a guided outline of how to look at a problem so we can solve the problem?
This article takes a look at algorithms: what they are, what they are used for, resources, and how to get started to learn how to write algorithms.
What is an Algorithm?
An algorithm is a set of instructions. If you compare an algorithm to a recipe, you can see what an algorithm is like:
Recipe for Burgers
Prep Time: 10 min
Input:
Ingredients[] — a list of objects that contain the name of the object and the amount needed
Ex: [{name: “beef, 85/15”, amount: “2 lb”}, {name: “American cheese, amount: “8 slices”}, etc.]
Directions[] — a list of strings that outline steps to finish and present burgers to hungry eaters
Ex: [“Unwrap meat and separate into 8 different sections”, “Roll up sections into a ball”, “Flatten each ball into a disc shape. All discs should be of an equal size”, etc.]
Output: Yields 8 servings
An algorithm, when written in code, assumes that you know about at least one programming language and its popular methods, and data structures and how to work with them. The concept is similar to how a recipe assumes you know about certain cooking methods and how to use kitchen supplies.
An algorithm has an input and an output. The output (solving the big problem) comes from solving smaller problems along the way.
What is an Algorithm Used for?
Algorithms are in use everywhere because they solve problems. In computer science and web development, they are used when writing code to instruct the computer to do something.
If we had a list of names, for instance, we can use a sorting algorithm like selection sort or bubble sort to sort the names by first name or by last name. We can also use a binary search algorithm to search for a name in this same list. These are just some of the standard algorithms that exist for you to take advantage of.
Know that you’re never truly done learning about how to write algorithms after finishing a course – you just get better at doing it. We’ll take a look at one way you can approach writing algorithms in the next section.
How to Learn to Write An Algorithm: Step-by-Step
Here are the recommended steps to learning how to write algorithms.
1. Learn a programming language
Java, JavaScript, and Python are all popular languages used for writing computer algorithms. Choose a language and learn it inside and out.
Make these concepts a part of your understanding of the language you choose:
- Data Types – Number, Boolean, Strings
- Variables
- Conditionals – if-else statements, ternary statements
- Functions – anonymous, named, and higher order functions
- Objects – be able to create objects, manipulate objects, and use object methods
- Arrays or Lists – be able to create arrays/lists, manipulate them, and use their associated methods
- Loops – both traditional for loops and advanced array/list methods
- Classes
2. Data Structures
You need to have a good handle on data structures to make your algorithms more efficient. Be sure to know what each data structure is used for and how it works.
- Arrays/Lists. Know how to manipulate an array/list and what the Big O is of the different insertion, deletion, and get methods. It’s important to know how arrays/lists work under the hood so you can figure out the time and space complexity of an algorithm.
- Linked List. Be able to explain the purpose of a linked list, and how it is different from an array. Also you should know the Big O of its common methods.
- Hash Table. Understand how a hash table works and what the Big O is when working with one.
- Stacks/Queues. Be able to discuss the differences and similarities between a stack and a queue and what data structures can be used for this special abstract data type.
- Trees. Using trees to cut down the time an algorithm takes to run is a good method. Binary trees are a very popular tool in algorithm manipulation.
- Graphs. What graphs could represent and why graphs might be used in constructing an algorithm.
3. Writing Algorithms: The Problem-Solving Process
You’ve learned a basic programming language and enough data structures to get started. Congratulations! The fun is getting started.
Start small. It’s important to understand the four concepts from George Pólya’s problem solving treatise (see the Resources section). A couple more steps were added here to breakdown his general approach even further:
George Pólya’s Guide to Solving Problems
- Understand the Problem
- What is it that you’re looking for?
- Take the problem and put it into your own words – try to explain it to someone who is not technically savvy.
- Grab keywords from the problem to help you construct a basic plan – no code. Just steps needed to get to an answer.
- Iterate on that plan to create a code solution.
- If you can’t figure out a solution, go back to #1 to ensure you are properly understanding the problem. Are you returning the correct output? Is there something you may have missed in the coding prompt?
- What is the Big O notation of your solution? Is there anything you can do to make the solution a more efficient algorithm in reference to space complexity or time complexity?
4. Start Small
Start with a basic searching algorithm that loops over a string or an array to search for an input. If the input can’t be found, return false. If it is found, return true.
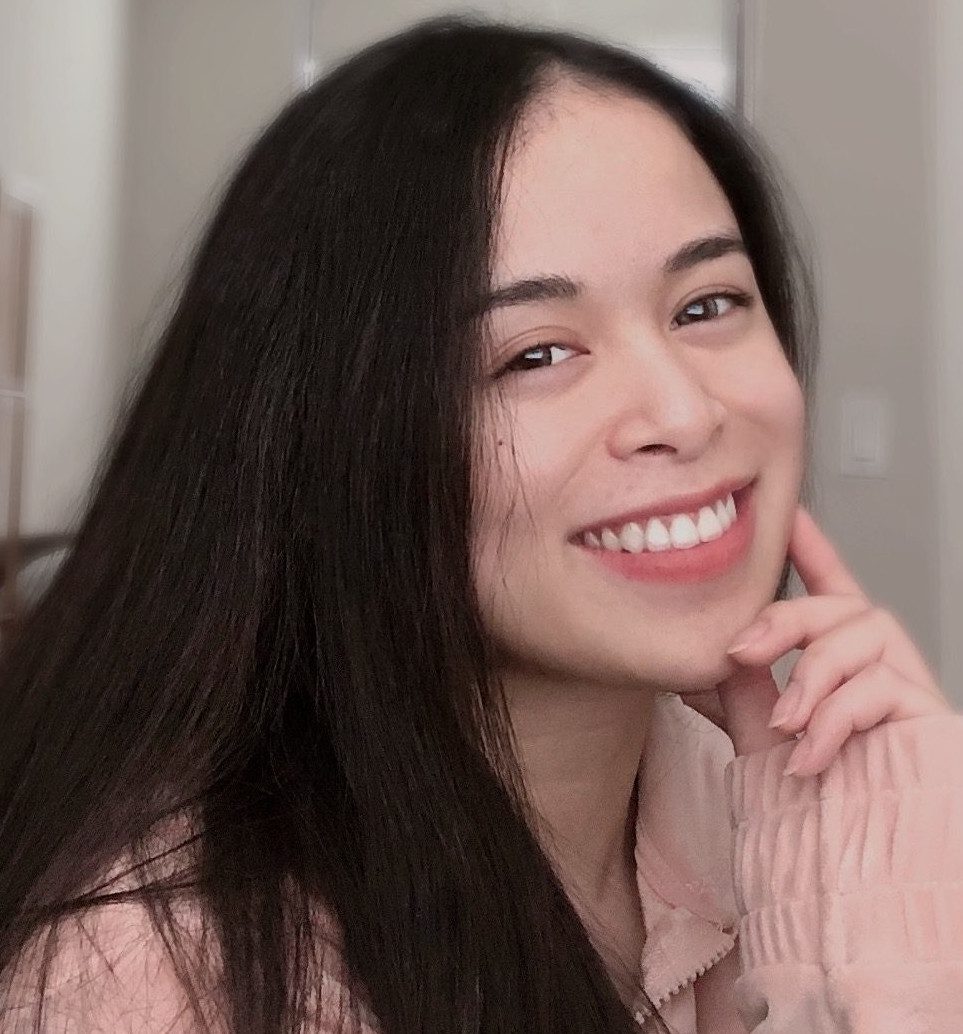
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Expand on this beginner algorithm and use other data structures to see how others might need a different process. Try sorting the input to use more efficient search algorithms.
Use algorithm practicing sites like the ones listed in the resource section to advance your skills.
5. Advance to More Difficult Algorithms
After several iterations, you’ll be able to advance to more difficult algorithms. At this point, you should be able to recreate some of the sorting algorithms, including Bubble Sort and Merge Sort. Binary Search should also be a part of your everyday coding experience.
Continue to use algorithm practicing sites to skill up. Try your hand at problems that are labeled a little more difficult to see how you do.
The Best Algorithm Courses and Trainings
Everyone learns a little bit differently. Compiled here are some of the best courses and training on algorithms available. Some have a fee, but there are free ones as well. This list is in no particular order.
Online Algorithm Courses
Here are some of the best algorithm courses available online:
Algorithms Specialization, Stanford University
- Coursera
- 4 courses:
- Divide and Conquer, Sorting and Searching, and Randomized Algorithms
- Graph Search, Shortest Paths, and Data Structures
- Greedy Algorithms, Minimum Spanning Trees, and Dynamic Programming
- Shortest Paths Revisited, NP-Complete Problems and What To Do About Them
- 4 months
- Prerequisites: The course is language agnostic, but assumes you know at least one programming language, basic data structures, and enough mathematics to know what proofs are.
- Certificate: $49/month until finished; Audit: FREE
Professor Tim Roughgarden of Stanford University teaches the Algorithms Specialization courses on Coursera. These courses will cover just about everything you need to touch upon to thoroughly understand algorithms so that you will do well in technical interviews.
Algorithms, IIT Bombay
- edX
- 6 weeks, 6-8 hrs/week
- Prerequisites: Basic understanding of data structures
- Verified Certificate: $149; No Certificate: FREE
This course is part of the IIT Bombay Fundamentals of Computer Science XSeries Program. Topics covered here include Sorting and Searching, Numerical Algorithms, String Algorithms, Geometric Algorithms, and Graph Algorithms.
Free Algorithm Courses
Intro to Algorithms
- Udacity
- Approx. 4 months, self-paced learning
- Prerequisites: At least one programming language; Course uses Python in examples
- FREE
This is a free crash course in algorithms from Udacity’s Michael Littman. He uses the six degrees of Kevin Bacon phenomenon to show how algorithms work. Think of the six degrees of separation – it’s been said that everyone is six or fewer social connections away from the other. He uses the same concept but with Kevin Bacon movies and actors.
FreeCodeCamp, Basic Algorithm Scripting
- FreeCodeCamp
- Self-paced
- Prerequisites: basic understanding of JavaScript and data structures
- FREE
This set of exercises by FreeCodeCamp will set you up for success through solving smaller problems before graduating to more difficult ones. Self-paced exercises use FreeCodeCamp’s learning platform to test your code.
A Visual Introduction to Algorithms
- Educative
- Self-paced, mainly text-based and examples
- Prerequisites: JavaScript, Python, C++, or Java
- FREE
In this free course, visual aids and videos are used to illustrate how algorithms work. Built-in code environments make the user experience fairly good. Potential solutions included.
Algorithm Books
There are several books to assist you in your journey to become a better algorithm scriptwriter. Most of them are available at a local bookstore and on Amazon.
‘Grokking Algorithms’
‘An Illustrated Guide for Programmers and Other Curious People’
Grokking Algorithms is one of the best books on algorithms in print. Created by an artist-turned-software developer, Bhargava takes an illustrated approach to help you intuitively understand, or grok, algorithms. Exercises at the end of each chapter help solidify the material in the book.
‘Algorithms in a Nutshell, 2nd ed.’
‘A Practical Guide’
By George T. Heineman, Gary Pollice, Stanley Selkow
This book takes a design pattern approach for you to learn when to implement certain algorithms. You will learn how to code out solutions then improve on those solutions by using advanced data structures. Solutions are in Ruby, Java, C++, and C, but are easily translated to preferred languages.
‘Algorithms, 4th ed.’
By Robert Sedgwick and Kevin Wayne
The fourth edition of Sedgewick and Wayne’s Algorithms text is a fantastic introduction to the algorithm world. The authors go over 50 of the most important algorithms for you to know. The Princeton educators have developed an online course that complements this text well.
Online Algorithm Resources
This is a list of some of the decent resources available to you to learn, practice, or get assistance with algorithms. No official ranking or order:
Cracking the Coding Interview
Cracking the Coding Interview by Gayle Laakman is one of the most popular technical interview resources out there.
Laakman writes about what it’s like to go through the interview process at FAANG companies like Facebook and Google, tips for the process, and code challenges to prepare you for the technical interview. Solutions are included.
Big O Cheat Sheet
Time and space complexity can be an overwhelming and sometimes confusing part of learning algorithms. Knowing the Big O of an algorithm can hint at whether or not you are able to refactor your code to make it more efficient.
This Big O Cheat Sheet was created when an engineer was trying to prepare for interviews at Big Tech and other larger companies that required data structures and algorithms as part of the interview process.
When in an interview, time and space complexity, the “Big O”, was often asked, and keeping everything straight in the engineer’s mind was difficult. Creating this cheat sheet was the solution they needed to nail the interview.
Pólya’s Problem Solving Techniques
In the 1940s, Stanford professor George Pólya, who was known for his techniques and teachings on problems solving, wrote a book on the subject.
He outlines a specific approach to create a plan for solving any problem you might come across. The main reason for the creation of this problem solving method was because he was tired of memorizing information he could deduce from taking a look at the problem!
This was the problem solving method that helped me understand how to approach solving code challenges by planning out my solution step-by-step. I highly recommend you at least giving it a try.
Sites to Help With Algorithm Practice
There are several websites that can assist in your practice before technical interviews. Here are just a few:
- Hackerrank
Hackerrank provides access to hundreds of coding challenges to help you practice your problem solving skills. Each problem offers a sample approach or hints to assist in thinking about an approach.
Challenges come with their own test cases, so it helps when it comes to learning how to think of trickier edge cases. Many companies use the Hackerrank platform to issue their own code challenges or assessments, so I would at least become familiar with the environment to prepare for technical challenges. - InterviewCake
The InterviewCake platform helps you to break down problems by their constituent steps if you get stuck. The platform is great for advancing your ability to think about more difficult coding challenges because it walks you through a problem step-by-step if you get stuck. This one costs money to access, but you can find it on sale. - LeetCode
LeetCode is similar to Hackerrank in providing access to hundreds, even thousands, of code challenges to help you practice your problem solving skills. A premium option gives you the ability to group questions by the company that has been known to ask them. This helps if you are preparing for an interview at a particular company. - CodeWars
If you are looking for a site that will help you skill up, look no further than CodeWars. The site uses a kata-based martial arts style system that assists in leveling up your skills. - AlgoExpert
This site is geared more for folks who are hardcore studying for technical interviews and want to get a highly competitive job at one of the top companies in the field. Likely, the typical user already has several years experience or a background in software engineering and just needs a competitive edge.
Videos and explanations are given by a top “AlgoExpert” from Facebook and Google whose goal in creating the website is to help engineers level up fast. - Project Euler
Leonhard Euler is credited as being one of the greatest mathematicians who ever lived. His research and specialty in how to solve analytic number problems did much for his industry.
This site is geared toward learning how to use mathematics in combination with computer programming to solve problems. The site has a bit of an old school feel, is not as complex in its judging system as other sites, and doesn’t have a built-in IDE. However, it asks questions that are not seen in other sites because it focuses more on mathematics. - Exercism.io
Exercism.io is a little different than other coding sites as it has you turn in your code solutions so a mentor can go over it with you. You can use your favorite IDE to create your solutions to coding challenges, turn it in using the command line or terminal, and then wait for feedback from a professional in the field. Many times it has been my experience that the mentor will go over how to improve your solution.
Most of these are free to use, but some are either premium or have a premium option to give a more customized experience.
Should You Study Algorithms?
The short answer is a resounding YES. This is the most important aspect of what makes one a software engineer.
The long answer: Studying and practicing algorithms will make you a better problem solver and a better software engineer. Being an algorithms expert makes you more desirable to companies as you are able to make their applications more efficient. By using your algorithm skills, you are able to pinpoint where any bottlenecks are in the code that may come up as a result of a runtime issue or a loading issue.
The learning never really stops with studying algorithms – just like music, practicing when you can will make you a better coder, interviewer, and employee.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.