How to Use Lambda Expressions in Java
Have you ever thought that some methods could fit on one line, if only it weren’t for the verbose Java syntax that you’ve got to use to declare a method? You’re not the only one.
Methods are an incredibly useful feature in programming.
A method is a block of code that does something specific in your program. They’re used because once you define a method, you can call it multiple times. This helps reduce redundancy in your code, which in turn makes it easier to maintain your code.
In this guide, we’re going to talk about lambda expressions in Java. These are a special type of method that you can use called a lambda expression, which implements a functional interface. We’ll talk about how they work and why they are used.
What is a Functional Interface?
Before we go on to talk about lambda expressions, you’ve got to understand functional interfaces. These are interfaces that only contain one abstract method. The one abstract method contained within a functional interface states the purpose of the interface.
Consider this example:
interface CalculateThree { double multiply_by_three(String day); }
We’ve created an interface called CalculateThree. This interface only has one method, multiply_by_three
, which means that it is a functional interface.
What is a Java Lambda Expression?
A lambda expression is an unnamed method. It is used to implement a method that has been defined within a functional interface. Lambda expressions are sometimes called anonymous methods on account of how they don’t have a name.
Lambda expressions make use of the arrow operator, which divides them into two sections:
(parameter list) -> lambda body
The left side contains the parameters that the expression uses; the right side contains the code that will be run when the Lambda expression is executed. Lambda functions can accept a single parameter or multiple parameters.
How to Use a Lambda Expression
Let’s create a program that takes in a number inserted by a user and multiplies it by three. We’ll start by writing the code that accepts a number from a user:
import java.util.Scanner; class Main { public static void main(String args[]) { Scanner user_input = new Scanner(System.in); System.out.println("Insert a number to multiply by three: "); double user_number = user_input.nextDouble(); } }
When we run this code, our user will be prompted to insert a number to multiply. Our program will read that number into the variable “user_number”. You can learn more about how the Scanner class works by reading our tutorial on Java Scanner.
Next, we’re going to define a lambda expression to multiply the number by three. Paste in the following code above your main
method:
interface CalculateThree { double multiply_by_three(double number); }
This code defines the interface to which our lambda expression will refer. Then add the following code at the bottom of the main method in the class:
CalculateThree multiply = (number) -> number * 3; double answer = multiply.multiply_by_three(user_number); System.out.println(user_number + " multiplied by three is " + answer);
We’ve used the CalculateThree interface to declare a variable called “multiply”. This stores the code for our lambda expression. The lambda expression is associated with multiply_by_three
in our CalculateThree interface because multiply_by_three
is the only function in the interface.
We’ve then used dot notation to call this function:
multiply.multiply_by_three(user_number);
Dot notation is where you specify the name of the class or interface you want to reference, followed by a dot, then the name of the method that you want to access. In this case, we want to access the multiply_by_three
method inside the “multiply” interface.
Our final code looks like this:
import java.util.Scanner; interface CalculateThree { double multiply_by_three(double number); } class Main { public static void main(String args[]) { Scanner user_input = new Scanner(System.in); System.out.println("Insert a number to multiply by three: "); double user_number = user_input.nextDouble(); CalculateThree multiply = (number) -> number * 3; double answer = multiply.multiply_by_three(user_number); System.out.println(user_number + " multiplied by three is " + answer); } }
Let’s run our code and insert the number 3 to multiply:
Insert a number to multiply by three: 3 3.0 multiplied by three is 9.0
Our code has successfully multiplied the number we inserted by 3. This action was performed using the lambda expression that we defined.
How to Use a Block Lambda Expression
Lambda expressions can appear in two forms: using single expressions, or using blocks. The block lambda syntax is used when the code on the right hand side of the arrow will take up multiple lines.
The block syntax is where you surround the code in the right hand side of a lambda expression with curly braces ({}).
Consider this example:
interface GreetUser { String welcome_user(String name); } class Main { public static void main(String args[]) { GreetUser send_greeting = (name) -> { System.out.println("Good morning!"); System.out.println("Welcome, " + name); }; System.out.println(send_greeting.welcome_user("Brad")); } }
We’ve declared a lambda expression called send_greeting
. This expression refers to the interface GreetUser that we defined at the start of our program. Our lambda expression performs two actions. It:
- Prints “Good morning!” to the console
- Prints “Welcome, ”, followed by the user’s name, to the console
Because this takes up two lines of code, we’ve used the block syntax. The code on the right hand side of our lambda expression is enclosed within curly braces.
Conclusion
Lambda expressions are unnamed methods that implement a functional interface. These functions are sometimes called anonymous classes or functions because they don’t have a name and they do not execute on their own.
Are you up for a challenge? Write a lambda expression that checks whether or not a number is even. If it is, “X is even” should be printed to the console, where X is the number being tested; otherwise, “X is odd” should be printed to the console.
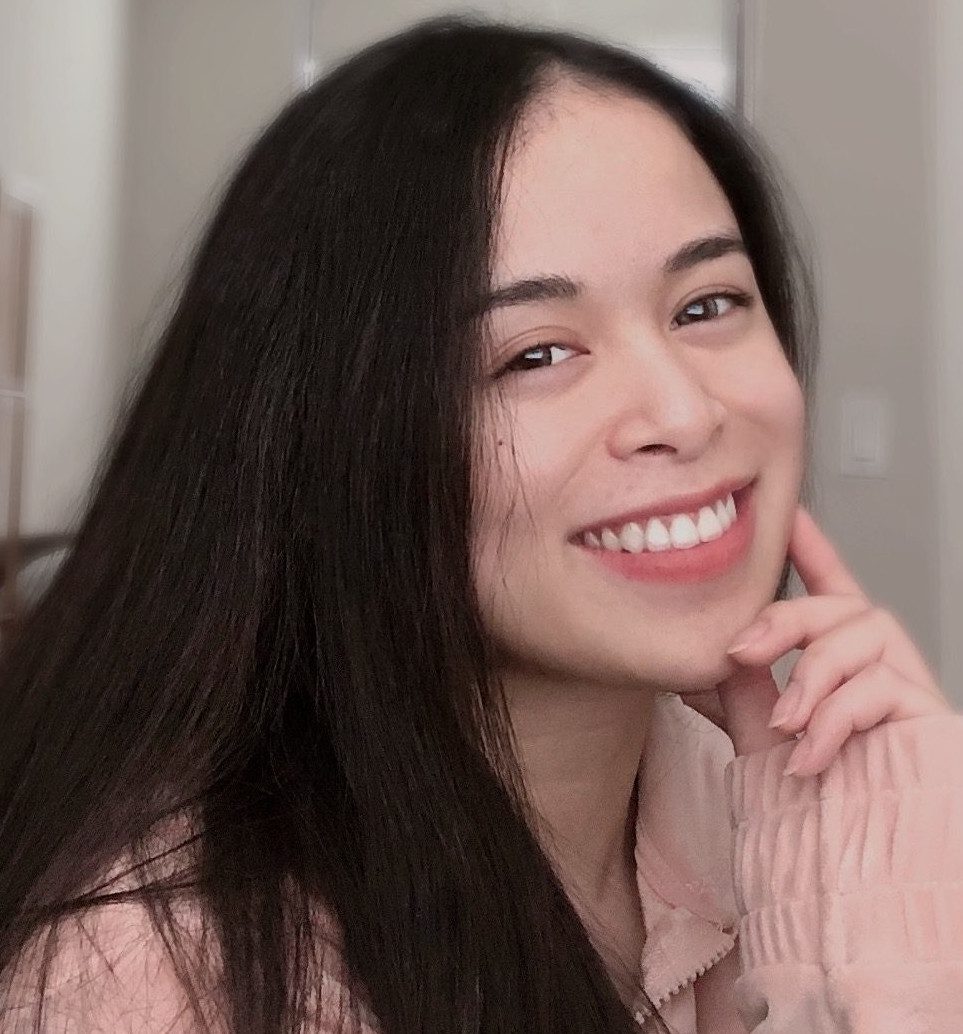
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Now you’re ready to start working with lambda expressions in Java like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.