As you learn how to build web forms, one of the most common tasks is to get values from dropdowns. In this article we’ll learn how to get such values with JavaScript.
Dropdown HTML Element
Let’s have a quick recap as to how to build a dropdown element using HTML. Do follow my Codepen if you get lost.
In order to build our dropdown element we need to use the select
tag and use option
children nested under it. The select element creates the dropdown and with the option tags we define those options that will live the dropdown.
Let’s create a dropdown of our favorite fruits (that have emojis).
<select name="fruits" id="fruits"> <option value="">--select--</option> <option value="avocado">Avocado🥑</option> <option value="watermelon">Watermelon🍉</option> <option value="kiwi">Kiwi🥝</option> <option value="tomato">Tomato🍅</option> </select> <h2 id="pick"></h2>
We added an empty h2 that we will populate with JavaScript later. At this point you can pick your favorite fruit:
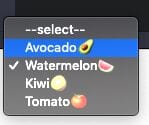
Notice the importance of the value property. This property gives us non-stylized data that we can easily play with JavaScript. On the other hand the text inside the option tag can be pretty much anything, so it is not reliable. The value property is what is important here.
Selecting and Displaying Dropdown Values with JavaScript
First of all we need to select our fruits dropdown by using getElementById
on the select tag.
const element = document.getElementById("fruits");
Now that our dropdown is selected we can get the value of the currently selected option. This by default is the first option. Let’s try that:
const checkValue = element.options[element.selectedIndex].value; const checkText = element.options[element.selectedIndex].text;
Here we are accessing the elements options array attribute. Then we select the index of the currently selected option and access its value or text.
Now try to console.log both variables. What happens? Nothing. Why? Because our first element has an empty value (it’s just a placeholder). Comment out the first option and try again. Yay! We get both the value and text with javascript.
Listening for Dropdown Changes with JavaScript
We should make our dropdown dynamic since JavaScript is designed to react for changes in your page. With that in mind we will assign an eventListener
to our fruit element.
element.addEventListener("change", (e) => { const value = e.target.value; const text = element.options[element.selectedIndex].text; if (value) { document.getElementById("pick").textContent = `Value Selected: ${value}`; } else { document.getElementById("pick").textContent = ""; } });
With our eventListener
we are listening for a change in our dropdown. Note how you can also get the value of the selected option by doing e.target.value
, this you’ve probably seen in another tutorial about forms.
We’ve arrived. Now you can select any fruit option and JavaScript is reacting to changes in our dropdown and updating our h2 accordingly. Very fruitful right!
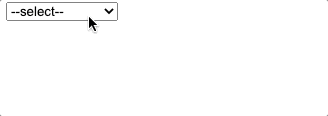
Conclusion
Getting dropdown values is a crucial skill as you learn web development. The values we get are important if we want to update a database, show the selection to the user or just ‘react’ to DOM changes. With JavaScript we can get such values in no time.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.