Once upon a time, working as a web developer just meant opening up a text editor on your desktop computer and typing some HTML. Today it’s much more involved and the jobs require very specific language skill sets. One of those skills that’s in high demand is working with JQuery, a Javascript library that’s used to create complex web front-ends. Having strong skills in JQuery will put you in a good position for a number of web development jobs.
If you’re applying to jobs that require JQuery skills, you’ll want to be prepared for anything that is thrown at you in the interview. Maybe you’ve never had a software-related job interview before, or maybe it’s been a while since you’ve interviewed for a position. The list below is a selection of questions that might come up during your interview. While these are sample questions that aren’t from any one particular employer, they will give you a good sense of what could be asked–and what you need to be knowledgeable about.
So, What is JQuery, Anyway?
It’s certainly not impossible for you to be asked something as basic as this. This isn’t to see if you actually know what the interview is for (but that’s certainly worth checking for), but more to see if you understand the underlying principles of a language you use.
In short, JQuery is a lightweight JavaScript library designed to help create front-ends faster. Its motto is, “write less, do more.” On a technical level, it simplifies traversing the DOM in HTML, manages event handling based on user actions, and makes working with AJAX easier.
JQuery makes it much easier to:
- Have websites that change their design or information according to user actions or incoming data.
- Have multiple displays of data that are continuously updating with bogging down the site or requiring frequent reloads of the page
- Provide interactivity with the user in a way that’s closer to a native app rather than a webpage.
Why Use It? What Are Some of the Advantages of JQuery?
There are as many Javascript libraries as there are Javascript methods. It’s good to be able to explain to an interviewer why you took the time to learn and use JQuery over other libraries. They may not need to be sold on it, but they want to know it’s important to you. Some reasons for doing so are:
- If you already know JQuery, you know pretty much everything you need to work with JQuery. It follows the same structure and syntax so you can find your way pretty easily.
- JQuery does its work while keeping the code clear and simple. This means the time spent coding is reduced so that you don’t have to reinvent the wheel. This helps when reusing code as well.
- There is extensive cross-browser support for JQuery. You don’t have to do a lot of bending over backwards to get it to work. As time goes on, this is less of a concern, but there are still a number of sites with legacy browser support (and concerns about accessibility).
- It has sophisticated event detection and handling methods, something that regular Javascript requires a lot of work to make work.
- JQuery is very lightweight, especially when zipped. This is not a bloated library that will slow your site down.
- Lots of plug-ins are available for JQuery, meaning it will continue to grow in what it can do.
What Are Some of the Features of JQuery?
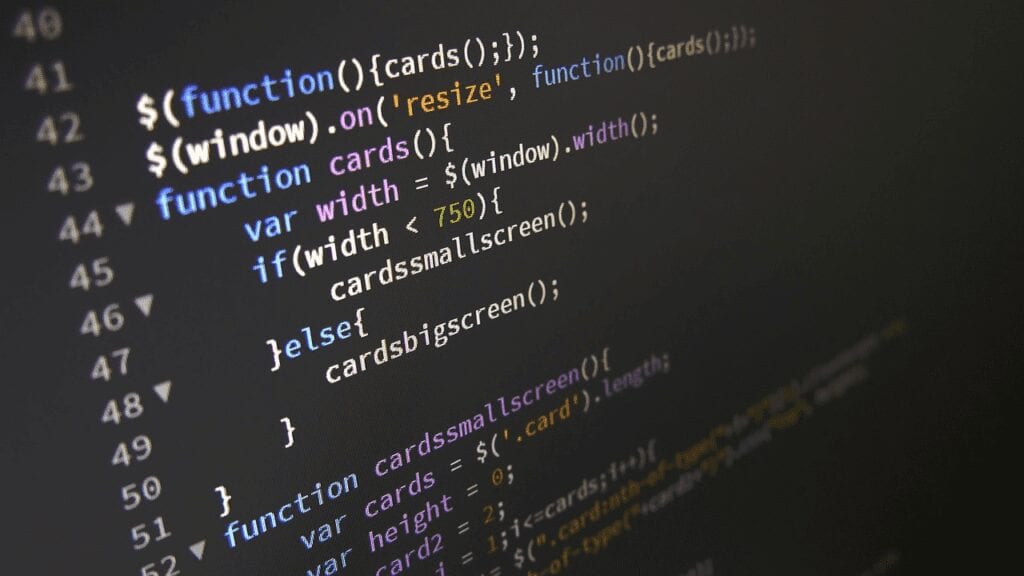
There are tons of JavaScript libraries out there. Why use JQuery?
You might not need to sell an employer on JQuery, but it’s good for you to know some of its important features. This is pretty open ended, but a few things of note worth mentioning are:
DOM manipulation: JQuery is probably the most popular tool for navigating the DOM of a website. The DOM is the Document Object Model, a way of representing the HTML of a page so that it can be manipulated and navigated. It connects all the various parts in a sort of hierarchy.
Event handling: JQuery makes monitoring, capturing, and responding to any user action (such as clicking a mouse) easier and cleaner by not keeping the code for this in the HTML itself.
AJAX features: AJAX stands for “Asynchronous JavaScript and XML” and uses existing web tools (like JavaScript and XML) to update web pages without reloading the whole site. This enables, say, one panel of data to be up to date while the rest of a page is unchanged. It can make these updates in response to user actions among other things.
Animation features: There are plenty of ways to animate user interactions on websites. JQuery makes the process of creating these animations simpler and cleaner. But what are some of those animations?
Speaking of Animation, What Are Some of the Effects Methods That JQuery Uses?
If you’re going to bring it up, an interview might dig a little deeper and want to know that you’ve got the hands on experience with a feature like animation. The animation of web interface elements is one useful tool in JQuery’s toolbox that websites use a lot. There are five main effects methods you can call with JQuery. They all provide some sort of animation effect.
- show(): To display some selected element when it isn’t already visible.
- hide(): You guessed it – to hide a selected element.
- toggle(): This enables you to switch back and forth between show and hide.
- fadeIn() and fadeOut(): Brings a selected element in and out of opacity, making it visible or invisible.
What Are the Selectors you Can Use in JQuery?
Selectors are the hooks that connect JQuery to a website. Showing your knowledge on this shows you have working knowledge of the library. Usually you will use HTML or CSS elements as selectors for any JQuery action. All JQuery selectors start with a dollar sign and parentheses: $()
Some of the ways in which you might find them with the page are: name, ID, class, or universal (for all elements within the DOM). You can then use expressions and logic to match to these selectors. So for selecting by tag, you’d use:
$(div)
To select by ID:
$(“#thisIsTheID”)
..and by class:
$(“.thisIsTheClassName”)
What Are the Events in JQuery?
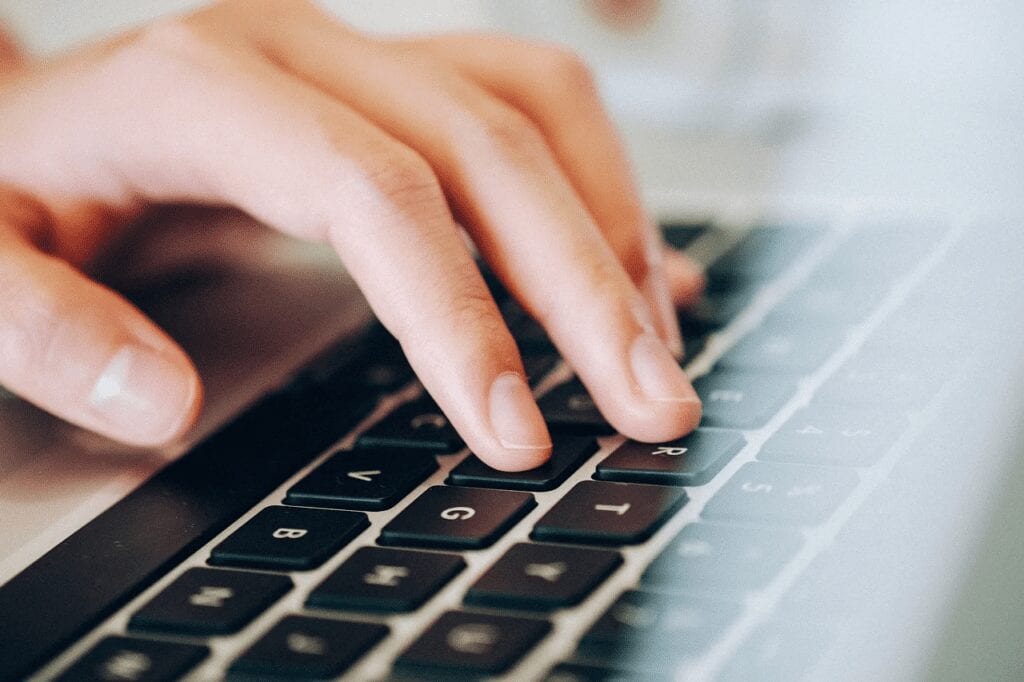
Any time a web page responds to a user’s actions, that’s called an event. One advantage of JQuery is that it makes it easy (or at least easier than with standard JavaScript) to add event handlers to code. This means you can have any user event trigger nearly any action like an animation, a data call, or a change in styling.
The DOM has five main events that can trigger actions: form submission, keyboard input, mouse action, browser, and document loading.
What’s the First Thing You Do With a Web Page to Make It Ready to Use JQuery Functionality?
A question like this demonstrates that you’re aware of what to do when incorporating the JQuery library and that you won’t make this rookie mistake. You need to make sure that the DOM is ready for what you’re going to throw at it. You do this by using this function:
$(document).ready() function
….and now your functionality will be available.
How Do You Access AJAX Functionality With JQuery?
Being able to use AJAX functionality quickly and easily is one of the main perks of JQuery. Being able to talk through that process will show the interviewer that you’re familiar with it and able to work with those functions. Some functions of note are:
- $.ajaxSetup(): Use this function to define and set your options for the calls you’ll be making on the page.
- $.ajax(): This is how you send data requests at the most basic level.
- $.getJSON(): This enables you to get data from a JSON formatted stream.
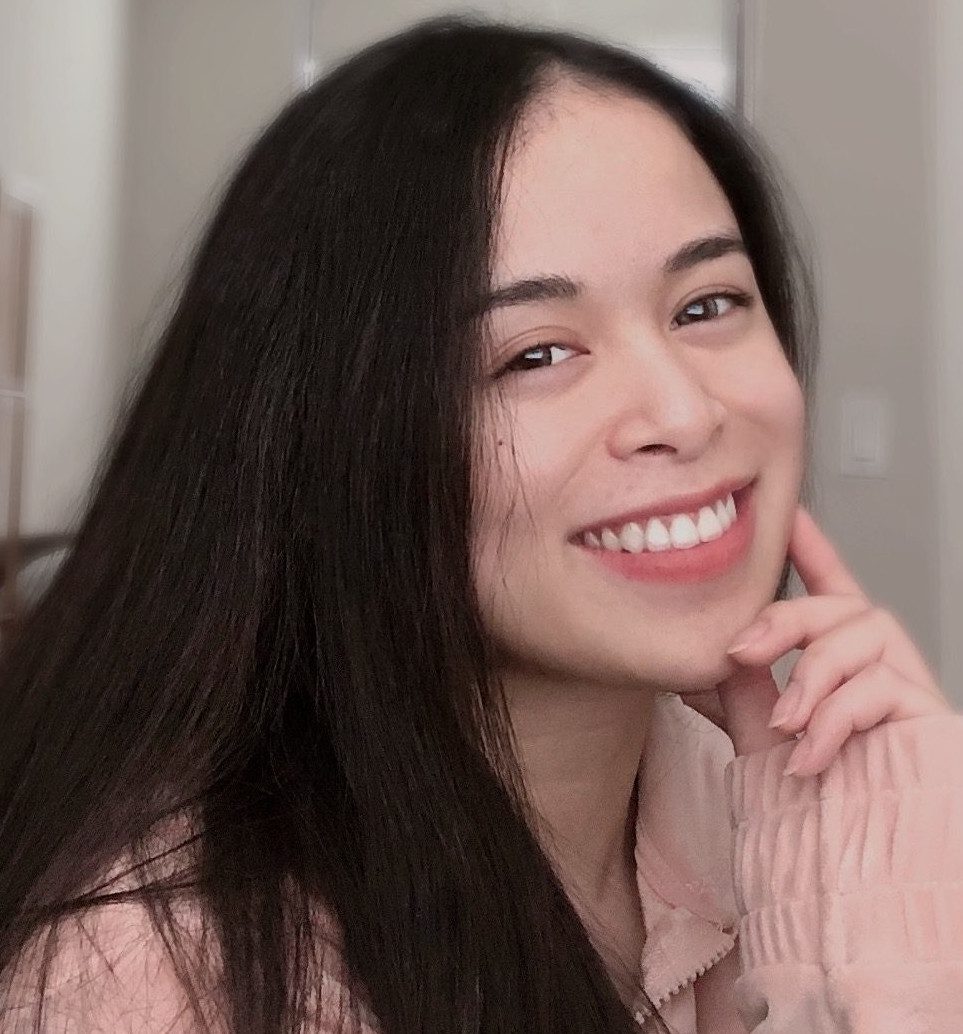
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Knock ‘em dead
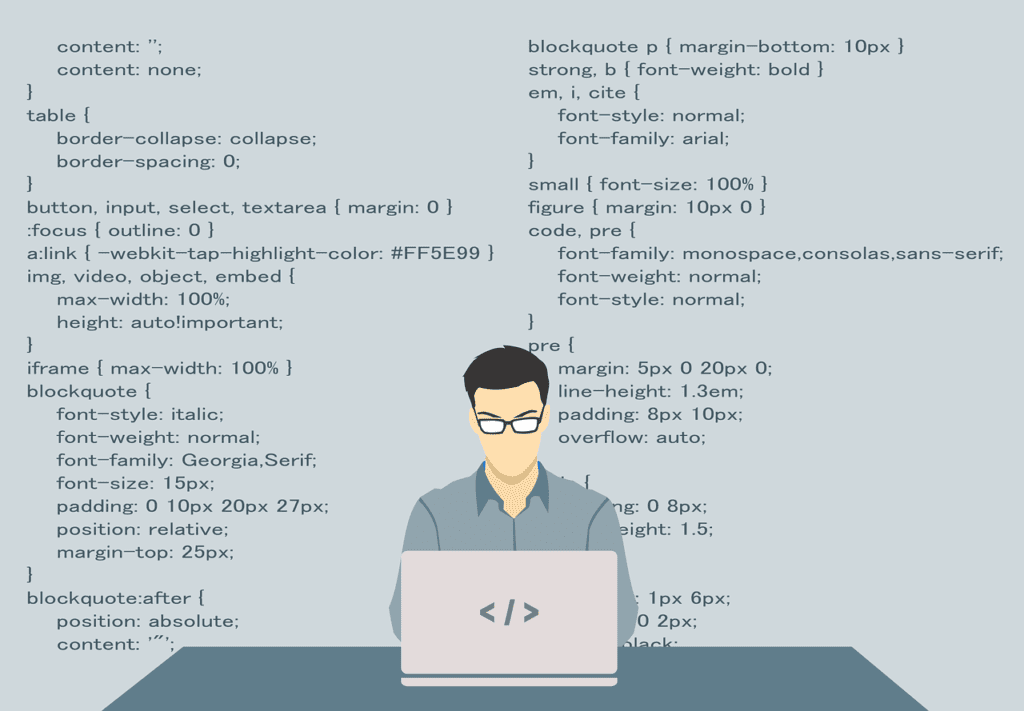
Congratulations on landing that JQuery developer job interview! You’ve worked hard at developing your skills and becoming knowledgeable in this leading JavaScript library. You’ll want to make sure you’re showing your best side in an interview. Review the questions above and make sure you feel comfortable with them and giving your own answers.
And if these questions spark some questions of your own, follow them to their answers to help you become even more prepared. If you’re not sure about your JavaScript or JQuery knowledge now, enroll in a coding bootcamp. Any boot camp near you will have classes in JavaScript that will help you build your skills and get you that much closer to acing that interview.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.