jQuery each()
is a concise way to iterate over DOM elements. Each()
method is designed to be called on the target jQuery object. A jQuery object is an object that contains one or more DOM elements and has access to jQuery methods. Not only is each()
less prone to errors, it simplifies manipulating multiple DOM elements.
jQuery each()
requires a callback function — a function passed to another function that will be executed later. Inside of the callback function is access to the index number of the element and the element itself. A more comprehensive review of callback functions can be found here.
The application of each()
is as straightforward as any JavaScript loops, but there are some places for potential confusion. We will be clearing up these points as well as looking at syntax. At the end of this guide will be step-by-step code examples to see different ways to apply jQuery each()
.
What is jQuery each()?
jQuery each is a loop method for the jQuery library. It works like a JavaScript loop by iterating over DOM elements. jQuery each()
requires a callback function, and inside the callback function is where the DOM elements can be manipulated.
While inside the body of the callback function, conditional statements can be used to change some of the selected elements. Conditional statements are if…then statements in JavaScript. This provides a deeper level of control over how exactly the elements are changed.
jQuery each() Syntax
jQuery each can be invoked in two ways. First, as a method called on a selected element. The callback function accepts up to two optional arguments: index and value. Index is the index number of the current element. If the current element was the first in the list, the index number would return 0.
The value argument refers to the current element. It is important to note that to chain a method to the value requires wrapping value in a jQuery selector. Let’s put this all into some abstract code so we can break down the syntax.
$('div').each((index, value) => { console.log(`${index}: ${$(value).text()}`) })
Here, we are selecting each <div> on the page and looping through it. At each iteration, we have access to the index position and value of the current element. Returning simply $(value) gives us the dreaded [object Object] value.
Remember that [object Object] is a string representation of an object. This means we need to call a method to extract the exact value we want. In the case above, we are using the text()
method to reveal the text attribute of the object. Read more about [object Object] to get a sense of what exactly is being returned.
The above example will log the index number followed by the text attribute for each <div>. If we did not need access to the index, we could refactor the code as such:
$('div').each(function() { alert($(this).text()) })
Writing it like this logs the text of the current <div> element as well. In the scope of this callback function, the keyword this also refers to the element previously referred to by the value variable. Note that you cannot access the keyword this in an arrow function.
Although arrow functions are a compact alternative to writing a function, it does not bind to the keyword this. In other words, the object returned by using this in an arrow function is not what is returned by this when written in functional notation.
This guide covers the ins and outs of arrow functions.
jQuery each() Examples
Let’s expand on the above syntax examples and add some attributes. We start off with a basic HTML rendering of a list of colors belonging to various classes.
<div class="colorSelect">Red</div> <div>Pink</div> <div class="color">Orange</div> <div class="colorSelect">Purple</div> <div class="colorSelect">Blue</div>
This renders each text element in the DOM. First, let’s begin by simply logging the text in the console using both arrow functions and a traditional function.
$('.colorSelect').each((index, value) => { console.log($(value).text()) })
By selecting only the <div>s with a class name of colorSelect, we log the text Red, Purple, and Blue. Notice how we are wrapping value in a new jQuery instance to call the jQuery text()
method. Doing so returns just the text of the selected <div> elements.
Let’s return the same results, but by using the keyword this.
$('.colorSelect').each(function() { console.log($(this).text()) })
We can use an anonymous function — a function without a name, as the callback. Again, it returns the text Red, Purple, and Blue. It is worth mentioning that although arrow functions do have some functionality in jQuery, it’s best practice to use traditional functional notation for jQuery callbacks.
We now know how to iterate over a collection of elements. Now, let’s have some fun and change the color!
<div class="colorSelect">Red</div> <div>Pink</div> <div class="color">Orange</div> <div class="colorSelect">Purple</div> <div class="colorSelect">Blue</div> <div class='result'> </div> <style> .colorSelect { color: blue; } .green { color: green; } </style>
Keeping with the color list page, we now have added some classes to the <style> element.
In this example, we have assigned the <div>s with the class colorSelect the color blue. We can get a little fancy and use a conditional statement to only change two of the three selected to the color green.
$('.colorSelect').each(function() { if ($(this).text() !== "Purple") { $(this).toggleClass('green') } })
We called each()
on the <div>s that match our class selector. Then we used a conditional statement to check each selected element to see if the text property was “Purple.” In the body of the conditional statement, we have another instance of this.
What this in the body of the conditional statement is referring to are the text attributes themselves that satisfy the condition. Based on our output, we know that this in the body of the conditional referred to text attributes “Red” and “Blue.”
Since “Red” and “Blue” do not equal “Purple,” we used the jQuery toggleClass method to change the original class to the class green. To learn more about jQuery toggleClass()
, I recommend reading this article.
Conclusion
We covered a lot of ground in this guide. First, we learned that jQuery each()
works a lot like any other JavaScript iterators, just for jQuery objects. Then we covered some syntax best practices and some potential problems in writing arrow functions and the traditional function notation.
In our examples, we saw how arrow functions are useful, but limited. Here’s a reminder to save yourself the headache and use traditional functional notation for all jQuery callbacks.
We then had some fun and changed colors of text attributes. By using a conditional statement in the body of the each()
loop, we saw how we can select and change only the elements that satisfy our condition.
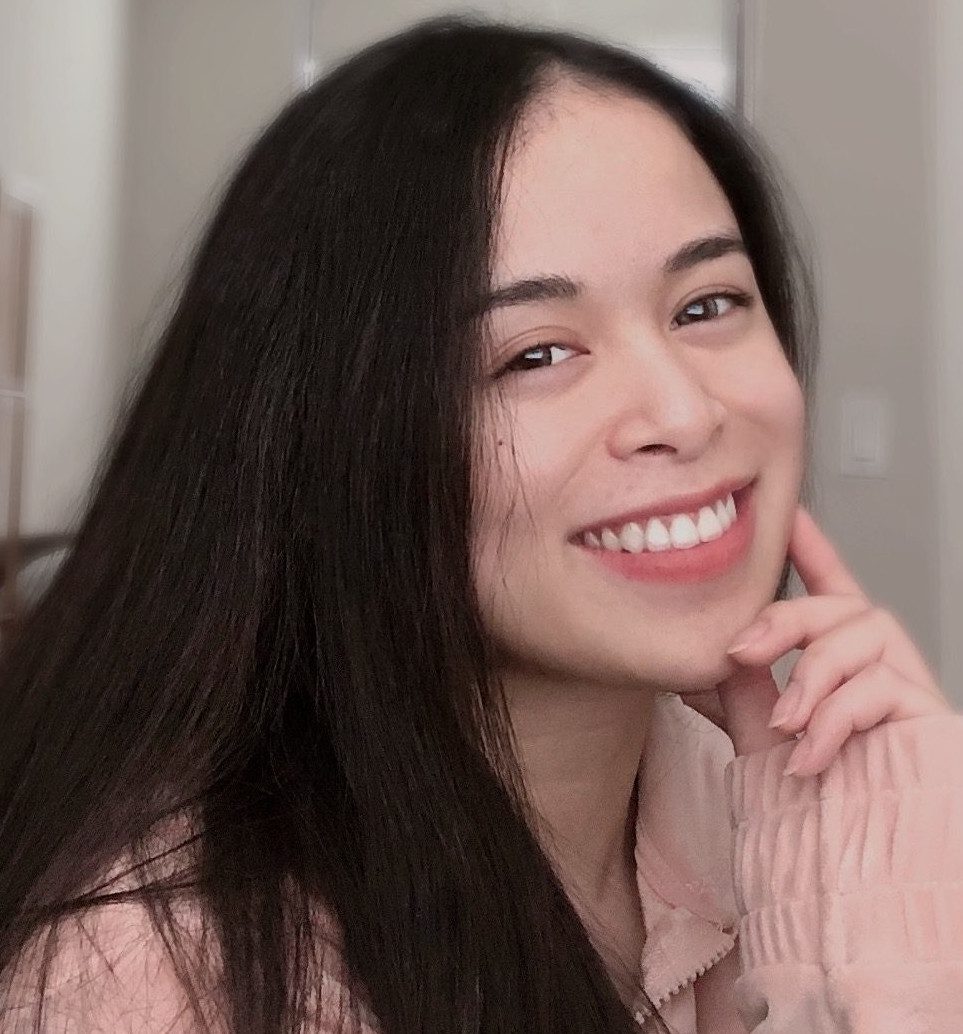
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Now, it’s up to you to practice your new found jQuery looping patterns. Start small then build up to seeing what all jQuery each()
is capable of.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.