JavaScript try catch blocks are error handlers. The “try” block contains the code you want to test. “catch” contains code that will run if the code in your “try” block cannot execute successfully.
When developers are testing their programs, it’s common to write code that handles errors in a certain way. This is more graceful than allowing the program to crash, which would cause a programming error to appear in their program. For example, you may want the user to see a message, “Please try again later,” rather than a long error log.
That’s where the try/catch blocks come in. Try/catch blocks allow you to handle errors gracefully so that coding problems don’t crash the entire program. In this guide, we’ll demonstrate how to use try/catch blocks and discuss how they’re useful.
Error Handling Refresher
Every developer makes mistakes in their code because there are so many ways in which a program can break.
When an error is encountered in JavaScript, the program usually stops and prints the error to the console. This is useful because you can see what’s wrong, but users who encounter the error aren’t likely to understand it.
That’s why it’s important to include error handling procedures in your code. By including an error handler, you can customize how your program responds if an error is encountered. Thus, error handlers allow you to decide what messages users see when an error is encountered, or how you are notified of a problem.
JavaScript try catch
The JavaScript try catch block identifies errors in a specified block of code. The contents of the “catch” block run if there is an error in the code in your “try” block. You can also use a “finally” block to run code whether your program executes successfully.
Here is the syntax for a try/catch procedure:
try { // Your code here console.log(“The code works!”); } catch (e) { console.log(“There is a problem in my code!”); }
There are two statements in our block:
- try {}.
- catch (e) {}.
The code enclosed in the “try” statement is what the program will attempt to run. In this case, our program attempts to print “This code works” to the console. The code enclosed in the catch statement runs if and when an error is returned in your try statement.
The (e) value in the catch statement represents the error that caused the code in your “try” statement to fail. You may decide to print out the value of “e” so you can see the root cause of the error that stopped your code.
try catch JavaScript Example
To illustrate the try/catch blocks in action, let’s use an example. In the example below, we misspelled a JavaScript variable name in our code.
let ourVariable = “Test”; console.log(ourVarible);
Our code returns:
ReferenceError: ourVarible is not defined
This is the default error returned when a variable cannot be found. But what if the user were to see this error? They would likely be confused. Now let’s try our code using a try/catch block:
let ourVariable = “Test”; try { console.log(ourVarible); } catch (e) { console.log(“There is a problem!”) }
Our code returns:
There is a problem!
Our code contains the same ReferenceError as we have seen above. But instead of returning the lengthy and complex default error, our program returns what we specified in the catch block. Our code logs the error, but the program returns our custom error instead.
The catch block keeps track of our error in the argument “e,” If we wanted, we can still access the error that was returned. Here’s an example of a program that returns the value “e”:
let ourVariable = “Test”; try { console.log(ourVarible); } catch (e) { console.log(“There is a problem! Here is the error message from the code:”, e) }
Our code returns the following:
There is a problem! Here is the error message from the code: ReferenceError: ourVarible is not defined.
Now our code reports an error to us and also shows the error message thrown by the program.
It’s worth noting that catch clauses are optional. If you want your program to do nothing if an error is encountered, you only need to enclose your code in a try block.
JavaScript try catch finally Clause
There is one more clause we can add to our code: finally. Finally, is an optional clause like catch, and allows us to run code even when an error is encountered. Here’s an example of a try/catch/finally clause in action:
let ourVariable = "Test"; try { console.log(ourVarible); } catch (e) { console.log("There is a problem! Here is the error message from the code:", e) } finally { console.log("The code has been run!") }
Here is what our program returns:
TypeError: Your variable is not a string! The code has been run!
Our code encounters an error so the code within our catch clause is run. This returns the TypeError that we see above. After the error has been handled, the code in our finally block is run, which prints out This code has been run!
Conclusion
You can use JavaScript try/catch clauses to test out your code. The contents of a catch statement will run if your try statement cannot execute successfully. A finally statement executes immediately after either the try or catch statements.
If you are developing a site that users will see, having custom errors can be useful. Custom errors will make sure users don’t get confused when something goes wrong.
Do you want to learn more about coding in JavaScript? Read our How to Learn JavaScript guide. This guide contains top advice on how to learn JavaScript.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
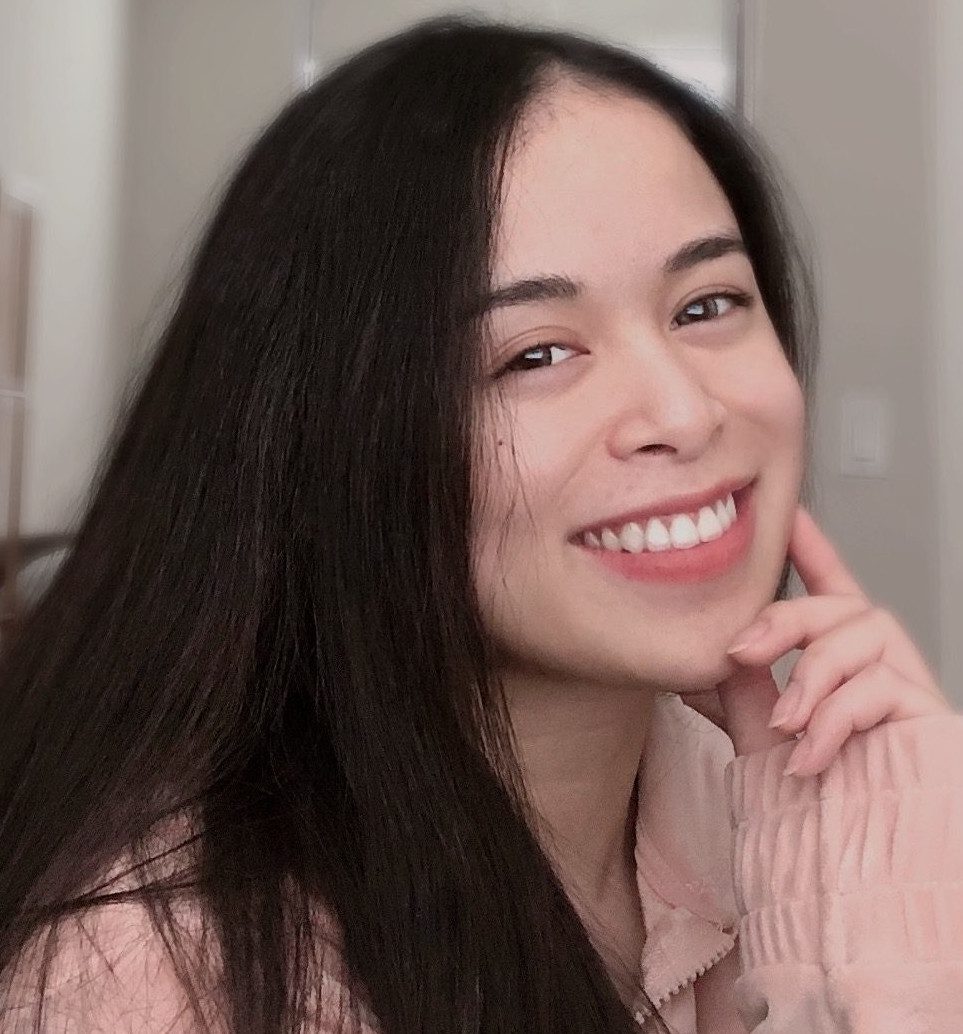
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot