avaScript spread operator expands an array in syntax that accepts arguments. The spread operator is commonly used to duplicate objects, merge arrays or pass the contents of a list into a function.
The spread operator. No, it’s got nothing to do with toast. In JavaScript, the spread operator has a specific meaning: it’s a way to access the contents of an iterable object. While this may not sound as fun as pasting a spread on toast, it’s an incredibly useful tool to know about.
In this guide, we’re going to talk about the JavaScript spread operator and how it works. We’ll walk through a few examples of common use cases to help you get started.
What is the JavaScript Spread Operator?
A JavaScript spread operator lets you access the contents of an iterable object. The spread operator is a set of three dots (an ellipsis) followed by the name of the iterable you want to access. This operator was introduced in JavaScript ES6.
The three types of iterable objects are arrays, object literals, and strings. Using a for loop, you can loop through all of these types of data and run a common process on them.
Iterable objects are useful because you can execute the same process on them multiple times. You can loop through a string and replace certain characters. You can loop through an array and create a total of all the values stored in the array.
The syntax for the spread operator is:
var names = ["John", "Lisa", "Harry"]; var new_names = [...names, "Leslie"]; console.log(new_names);
In this syntax, we use …names to pass the contents of our “names” list into a list called “new_names”. The “new_names” list contains all the items in the “names” list, as well as a new name: Leslie.
Three common use cases for the spread operator are to:
- Create a copy of an iterable.
- Add items to a new iterable.
- Pass a list of arguments into a function.
The spread syntax represents all the individual elements in a list.
Spread Operator JavaScript: Create a Copy of an Iterable
The spread operator is an effective method of duplicating an iterable. While there are other ways to approach this problem, the spread operator is really easy to use. To create a copy of an iterable, specify three dots and the name of the array you want to create.
In our last example, we showed how this works with an array. We can also use the spread operator to duplicate JavaScript objects:
const lemon_drizzle = { name: "Lemon Drizzle", price: 1.95, vegan: true } const new_lemon_drizzle = {...lemon_drizzle}; console.log(new_lemon_drizzle);
Our code prints the following to the JavaScript console:
{ name: "Lemon Drizzle", price: 1.95, vegan: true }
There are a few important distinctions we need to make in this example. We are creating a copy of a JavaScript object. This means that we need to use curly braces ({}) instead of square brackets ([]).
Second, instead of specifying a single array, we have specified an object. This object contains three keys and values, each of which is related to our “Lemon Drizzle” cupcake.
Spread JavaScript: Add Items to a New Iterable
The use cases of the spread operator don’t stop with copying iterables! There’s more to explore. The spread operator is commonly used to add items from one iterable to the next. Consider the following example:
const cupcakes = [ 'Lemon Drizzle', 'Chocolate Chip', 'Vanilla Iced' ]; const new_cupcakes = [...cupcakes, 'Red Velvet', 'Raspberry Dark Chocolate']; console.log(new_cupcakes);
Our code returns:
["Lemon Drizzle", "Chocolate Chip", "Vanilla Iced", "Red Velvet", "Raspberry Dark Chocolate"]
We’ve created a copy of our original JavaScript array called “new_cupcakes”, which also includes a few additional values that we’ve created.
You could use the same syntax to merge two iterables together as well. All you would need to do is specify the two iterables inside square brackets using the spread operator:
const old_menu = [ 'Lemon Drizzle', 'Chocolate Chip', 'Vanilla Iced' ]; const new_menu = [ 'Red Velvet', 'Raspberry Dark Chocolate' ] const final_menu = [...old_menu, ...new_menu]; console.log(final_menu);
Our code returns:
["Lemon Drizzle", "Chocolate Chip", "Vanilla Iced", "Red Velvet", "Raspberry Dark Chocolate"]
The same result is returned as from earlier but this time we’ve merged two arrays together.
Spread JavaScript: Passing Arguments to a Function
When you’re passing multiple arguments into a JavaScript function, it can be helpful to use the spread operator. Consider this example:
function placeOrder(name, order, price) { console.log('Name: ' + name); console.log('Order: ' + order); console.log('Price: ' + price); } placeOrder('Geoff', 'Red Velvet', 1.85);
Our code returns:
Name: Geoff Order: Red Velvet Price 1.85
We’ve declared a function called placeOrder() that takes in three arguments and prints them to the console. Each value is preceded by a label describing what each argument contains.
While this code is functional, we could specify our arguments in an array and use the spread operator to pass them into our function:
function placeOrder(name, order, price) { console.log('Name: ' + name); console.log('Order: ' + order); console.log('Price: ' + price); } const order = ['Geoff', 'Red Velvet', 1.85]; placeOrder(...order);
Our code returns:
Name: Geoff Order: Red Velvet Price 1.85
The output of this code is the same, but the way our code works is different. Instead of directly passing values to our function, we specify those values in a list. We then use the spread operator to pass those values into our function.
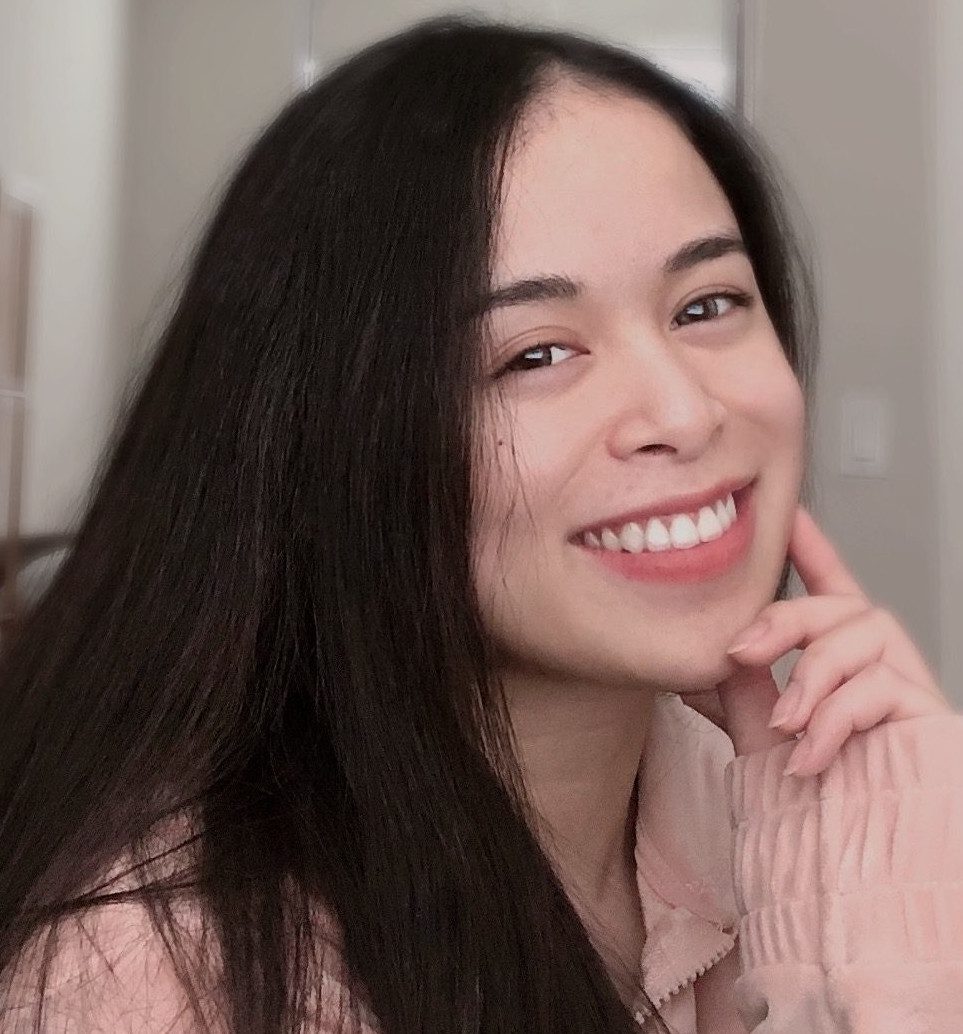
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
The spread operator is widely used throughout JavaScript. It makes it easy to copy iterable objects, add items to a new iterable, and pass arguments to a function. The syntax for the spread operator is three dots, followed by the iterable object which you want to access.
Now you’re ready to start using the JavaScript spread operator like a pro!
For advice on top JavaScript learning resources, books, and courses, check out our How to Learn JavaScript article.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.