You can use the JavaScript sort() method to sort an array. The sort() method accepts an array as an argument and sorts its values in ascending order. Arrays are sorted in place which means the original array is modified. A new array is not created.
You may decide that you want to sort your array in a particular order. For instance, you may have a list of names that you want to display to the user in alphabetical order.
Depending on how you want to sort the elements in an array, there are built-in JavaScript functions that can help. For example, you can use the sort() function to sort an array in alphabetical order, the reverse() function to sort an array in reverse order, and the sort() function with a nested function to create your own custom sorts.
In this tutorial, we are going to break down how to use sort() and reverse() to perform a sort array function in JavaScript.
JavaScript sort() Method
The JavaScript sort() method reads the values in an array and returns those values in either ascending or descending order. A string containing either numbers or strings can be sorted.
Consider the following syntax:
const values = [1, 2, 8, 9, 3]; values.sort();
This code sorts our “values” list. The list is sorted in place which means our original list is modified. sort() does not create a new version of a list.
It’s worth noting that the sort() method modifies the original array and changes its order. If we want to keep our original array the same, we can run the sort() function and store its value in a new variable.
If you assign the contents of the sort() method to a new variable, the original list will still be changed.
JavaScript Sort Array Example
To sort an array in JavaScript, we use the built-in default sort() method. In its most simple form, the sort() method sorts an array in ascending alphabetical order. Here’s an example of the sort() method in action:
var students = ['Alex', 'Cathy', 'Lincoln', 'Jeff']; var sorted_students = students.sort(); console.log(sorted_students);
Our code returns the following:
["Alex", "Cathy", "Jeff", "Lincoln"]
Our array has been sorted in ascending order. We use the sort() method to sort our JavaScript variable called “students”. Then, we print the value of “students” to the JavaScript console.
JavaScript Array sort(): Order a Numeric Array
We can use the sort() method to sort the values in a numerical list in ascending order.
Say we have a list of student grades that we want to sort in ascending order. We could sort them using the sort() method:
var grades = [67, 75, 62, 78]; grades.sort(); console.log(grades);
The output from our code is as follows:
[62, 67, 75, 78]
Our array has been sorted in ascending order! When our program starts the sort() operation, the elements within our array are sorted based on their values/.
Because sort() sorts a list in place, we do not need to assign the result of sort() to a new variable. Our code prints out the newly-sorted “grades” array to the console.
JavaScript Sort Array of Objects
The sort() method can be used to sort an array of objects. For example, we may have an array of JSON objects that store both a student’s name and age.
Here’s an example of a sort() function that would sort such an array by the ages of our students:
var students = [ { name: "Alex", age: 16 }, { name: "Cathy", age: 14 }, { name: "Lincoln", age: 14 }, { name: "Jeff", age: 15 } ]; var sorted_students = students.sort(function(a, b) { return a.age - b.age; }); console.log(sorted_students);
Our code returns the following:
[ { "name": "Cathy", "age": 14}, { "name": "Lincoln", "age": 14}, { "name": "Jeff", "age": 15}, { "name": "Alex", "age": 16} ]
Our code returned our array of JSON objects in order of student ages.
On the first line, we declare our “students” variable with four students. We then create a function that sorts our students variable based on the “age” value in each JSON object by comparing ages. Finally, our program prints out our sorted list of students.
JavaScript Array sort(): Sort in Reverse Order
There is also a built-in JavaScript function that allows you to sort an array in reverse order: reverse().
The reverse() method reverses an array so that the first element becomes the last, and the last element becomes the first. Thus, this array does not order an array in alphabetical descending order.
If we sort a list first using the sort() method, we can use reverse() to see our array in descending order.
Here’s an example of the reverse() function in action:
var students = ['Alex', 'Cathy', 'Lincoln', 'Jeff']; var reversed_students = students.reverse(); console.log(reversed_students);
Our code returns the following:
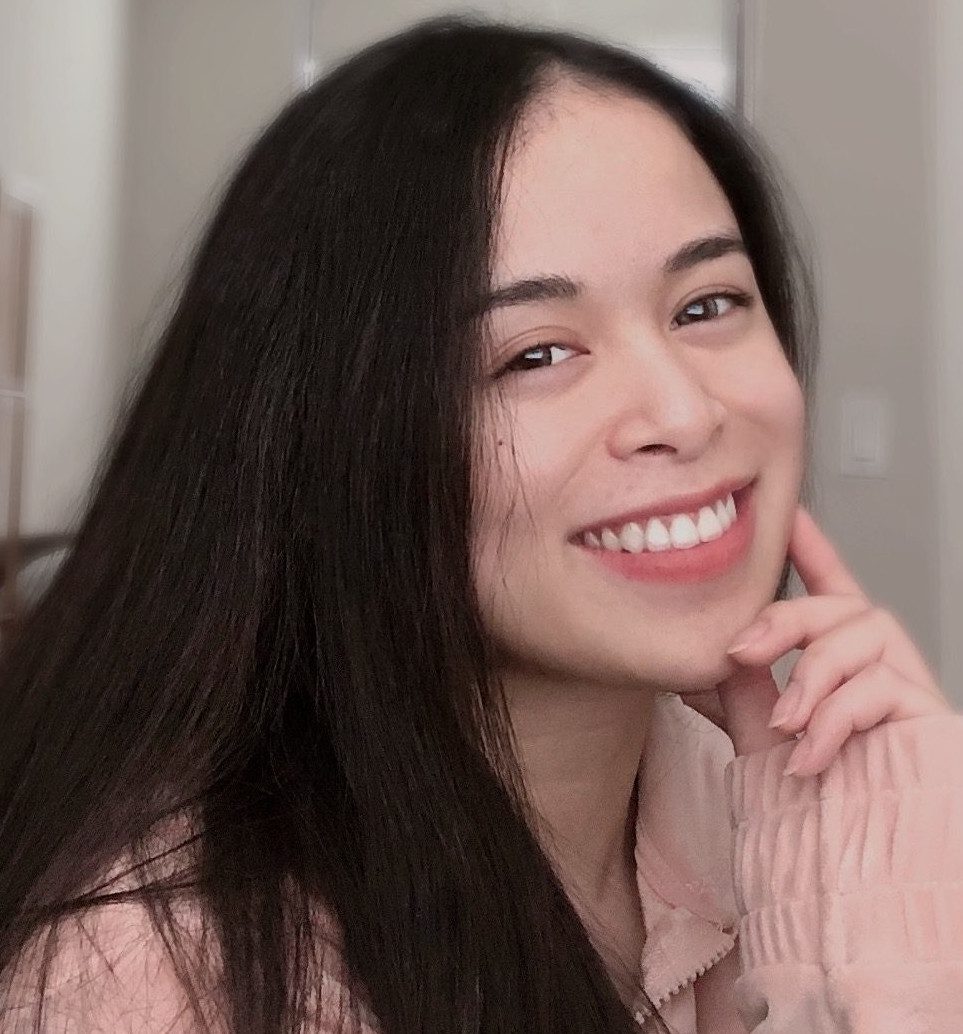
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
[“Jeff”, “Lincoln”, “Cathy”, “Alex”]
We first sort our list in ascending order using sort(). Then, we reverse the list using reverse(). Our list now shows our names in descending order alphametically.
The reverse() function, like the sort() function, modifies the order of our list. We cannot assign the result of reverse() to a new variable without modifying the original array. We talked about this earlier with the sort() method.
Conclusion
The JavaScript sort() method orders the contents of a list in either ascending or descending order. sort() modifies a list in place which means the method does not create a new list. reverse(), when used after sort(), sorts a list in descending order.
In this tutorial, we have broken down how to sort an array in JavaScript using sort(). We discussed sorting a numerical array, how to sort an array of objects, and using reverse() to reverse the contents of an array.
For advice on top JavaScript learning resources and online courses, check out our How to Learn JavaScript article.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.