JavaScript shift() removes an element at a specified position and shifts the remaining elements up. The JavaScript unshift() function does the opposite. unshift() adds a new element at a particular position and shifts the remaining elements down the list.
You may decide that you want to add or remove an item at the start of a list. Let’s say you make a list of top salespeople. You would want to insert the number-one top seller at the start of the list.
That’s where the JavaScript shift() and unshift() functions come in. shift() and unshift() are used to remove and add items to the start of a list, respectively. These functions are similar to the push() function and pop() function, although shift() and unshift() only support removing and adding items to the start of a list.
This tutorial will explore how to use shift() and unshift() to remove and add items to the start of an array. In addition, we’ll walk through an example of each of these functions in a JavaScript program.
JavaScript shift
shift() is a built-in JavaScript function that removes the first element from an array. The shift() function directly modifies the JavaScript array with which you are working. shift() returns the item you have removed from the array.
The shift() function removes the item at index position 0 and shifts the values at future index numbers down by one. If you are struggling to remember the purpose of the shift() function, tell yourself this:
shift() shifts the index values in an array down by one.
shift() returns undefined if you use the shift() function on an empty list.
Here’s the syntax for the JavaScript shift() function:
array_name.shift()
The JavaScript array shift() function takes in no parameters. This is because shift() only performs one function. shift() removes an item from the end of an array. If shift() let you remove an item from any position, a parameter would be needed.
Shift JavaScript Example
Let’s walk through an example to explain how the shift() method works.
Suppose you are the manager of a pharmaceutical sales team. You want to compose a list of this month’s top salespeople. To start with, you have a list of last month’s top salespeople. You want to remove Mark from the start of the list. Mark did not make the list last month because he was on vacation for two weeks.
We could use the shift() function to remove Mark’s name to the start of the list. Here’s an example of shift() being used to add Heather to the start of the top salespeople list:
let top_salespeople = ['Mark', 'Lucy', 'Graham', 'Carol', 'Ann']; top_salespeople.shift(); console.log(top_salespeople);
Our code returns:
['Lucy', 'Graham', 'Carol', 'Ann']
Let’s explain what is happening in our code. On the first line, we declare an array called “top_salespeople” which stores the top salespeople in the pharmaceutical sales team. Then, we use the shift() function to remove the first value from the list. Finally, we use console.log() to print to the console the revised list.
As you can see, the shift() function removed Mark from the list, who appeared in the first position.
The top_salespeople array no longer contains Mark. This is because the shift() function changes the original array. It does not create a new array with the modifications we have made.
JavaScript unshift
The JavaScript array unshift() function adds one or more elements to the start of an array. The unshift() function changes the array and returns the length of the new array.
Here’s the syntax for the unshift() function:
array_name.unshift(item_one, item_two, …)
The unshift() function takes in as many parameters as you specify. These parameters will all be added to the start of the array.
Unshift JavaScript Example
Let’s walk through an example and discuss how the unshift() function works.
An employee called Hannah had an amazing month on our sales team. She advanced to 1st position on the top salespeople list. We want to add Hannah to the 1st position in our top_salespeople array. We could use the following code to accomplish this task:
let top_salespeople = ['Lucy', 'Graham', 'Carol', 'Ann']; top_salespeople.unshift('Hannah'); console.log(top_salespeople);
When we run our code, the following response is returned to the console:
['Hannah', 'Lucy', 'Graham', 'Carol', 'Ann']
As you can see, Hannah’s name was added to the start of our list. The unshift() function works in the same way as the shift() function but with two differences: unshift() adds an item to the start of a list, and accepts that item as a parameter.
Our sales team has been expanding. Two of our new employees have managed to advance to the top of the sales team in terms of sales in only one month. We want to add these employees to our top_salespeople list. Geoff should appear at the start of the list, and Peter should appear second.
This code updates the list of top salespeople:
let top_salespeople = ['Hannah', 'Lucy', 'Graham', 'Carol', 'Ann']; top_salespeople.unshift('Geoff', 'Peter'); console.log(top_salespeople);
Our code returns:
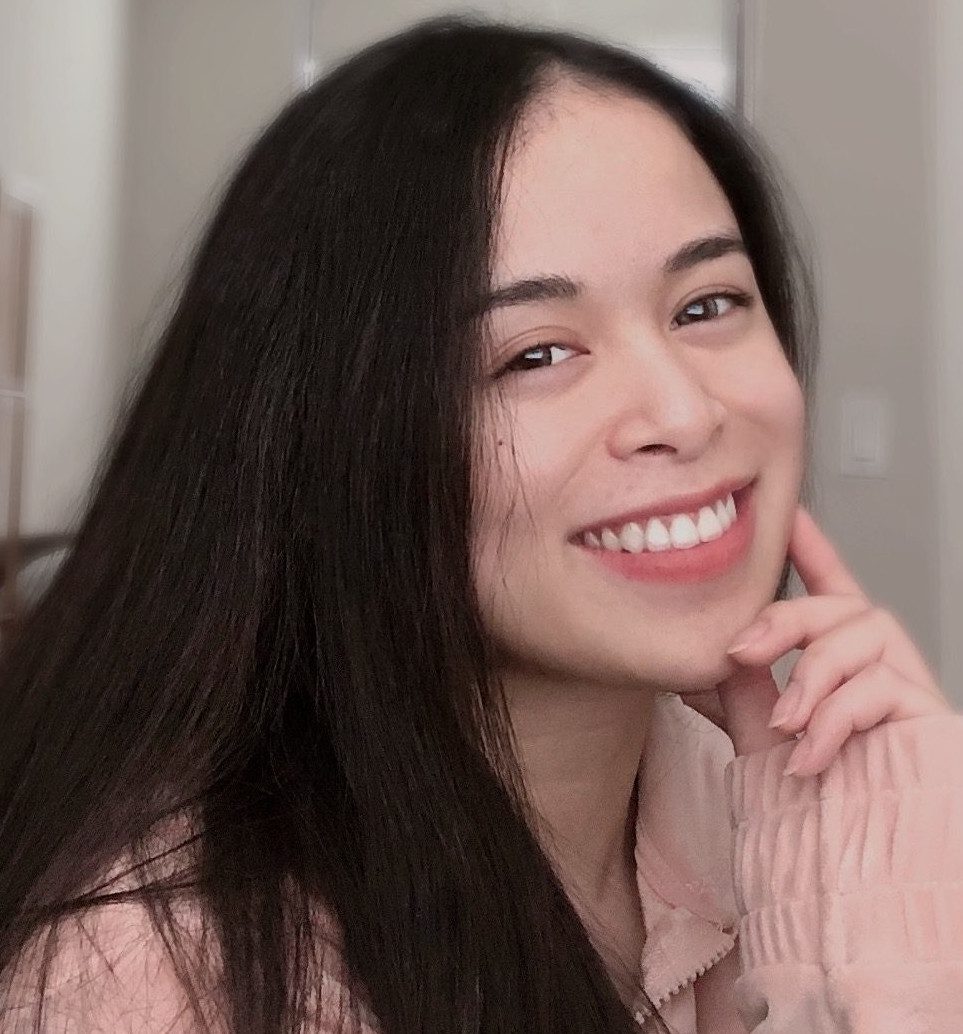
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
['Geoff', 'Peter', 'Hannah', 'Lucy', 'Graham', 'Carol', 'Ann']
This second example code works in the same way as our first example. There is one difference. In the second example, we have specified two parameters in the unshift() function instead of one.
As you can see, these parameters have been added to the start of our list in the order we specified. Geoff appears first and Peter appears second.
Conclusion
The shift() function lets you remove an item from the start of an array. The the unshift() function adds one or more items to the start of an array.
This tutorial discussed how to use both the shift() and unshift() functions to manipulate arrays in JavaScript. We walked through JavaScript exercises for each of these functions in action.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.