setTimeout() and setInterval() are JavaScript timing events. The JavaScript setTimeout() method executes a function after a period of milliseconds. JavaScript setInterval() executes a function continuously after a certain period of milliseconds have passed.
JavaScript runs line-by-line. As soon as one line has executed, the next line begins. For most websites, this structure makes sense: you need your code to execute in an order. What if you want to introduce a break between operations?
That’s where the setTimeout and setInterval methods come in handy. setTimeout calls a function after a specified delay. setInterval calls a function continuously at a specified delay.
In this guide, we’re going to talk about what setTimeout and setInterval are. We’ll show how they work by referring to a few examples so you can get started using these methods. Let’s begin!
What is JavaScript setTimeout?
JavaScript setTimeout() calls a method after a certain period of milliseconds have passed. The method is only called once. The setTimeout() method lets you introduce delays into your code.
Let’s take a look at the syntax for this method:
window.setTimeout(function, time);
The two parameters accepted by setTimeout are:
- function: The function you want to execute after a period of time has passed.
- time: The amount of time your program should wait before executing a function.
Start a setTimeout JavaScript Timer
Let’s create a timer which prints “Career Karma!” to the console using an arrow function:
setTimeout(() => { console.log("Career Karma!"); }, 2000);
This timer has two arguments. The first argument is the JavaScript function that we want to execute inside our window object. The second argument is the period of time in milliseconds that we want to elapse before the code inside our function should execute.
After waiting two seconds, our code prints “Career Karma!” to the JavaScript console.
The function you want to call does not need to be defined within the setTimeout function. We can refactor our code to make it easier to read:
function printCareerKarma() { console.log("Career Karma!"); } setTimeout(printCareerKarma, 2000);
The function that was previously an argument is now in its own function called printCareerKarma. We reference that function inside the setTimeout method. This code is easier to read than our original example.
How to Cancel a setTimeout Timer
You can cancel a timer using the clearTimeout() method. This will stop a timer from running and the code inside a timer will not be executed. Consider the following code:
function printCareerKarma() { console.log("Career Karma!"); } var setTimer = setTimeout(printCareerKarma, 2000); setTimeout(() => { clearTimeout(setTimer); }, 100);
This code will not print “Career Karma!” to the console. This is because we cancel our timer using clearTimeout before the setTimer timer has the chance to execute.
What is JavaScript setInterval?
The JavaScript setInterval() method calls a function repeatedly. A specified delay is set between each time a function is called.
setInterval() uses a similar syntax to setTimeout():
window.setInterval(function, time);
You must specify two parameters to use setInterval:
- function: The function that will be executed.
- time: The time between each call of the function you have specified.
Start a setInterval JavaScript Timer
Whereas setTimeout only executes a function once, setInterval will keep executing a function over again. It uses the same syntax as the setTimeout method.
Let’s create a function which prints out the contents of an array of coffees to the console:
let i = 0; let coffees = ["Cortado", "Macchiato", "Mocha", "Latte"]; function iterateOverArray() { console.log(coffees[i]); i++; } setInterval(iterateOverArray, 1000);
This code execute sthe iterateOverArray function repeatedly. There is a one second (1000 millisecond) delay between each call of this function.
Let’s run our code:
Cortado Macchiato Mocha Latte undefined undefined ...
The trouble with this code is that it keeps going even after it has iterated over every item in our list. When all items in our list have been printed to the console, “undefined” values are printed indefinitely.
The setInterval function will keep executing because it repeats until it has been instructed to stop. Let’s fix this problem.
How to Cancel a setInterval Timer
The clearInterval method stops a setInterval method from executing further.
Let’s cancel our timer once all the coffees on the menu are printed to the console. To start, we’ll calculate how many items are in our coffees list. This will allow us to identify when we should cancel our timer.
Next, we’ll add a function to the end of our code. This function stops our setInterval method after all our coffees have been printed to the console:
let numberOfCoffees = coffees.length;
Next, we’ll add a function to the end of our code. This function stops our setInterval method after all our coffees have been printed to the console:
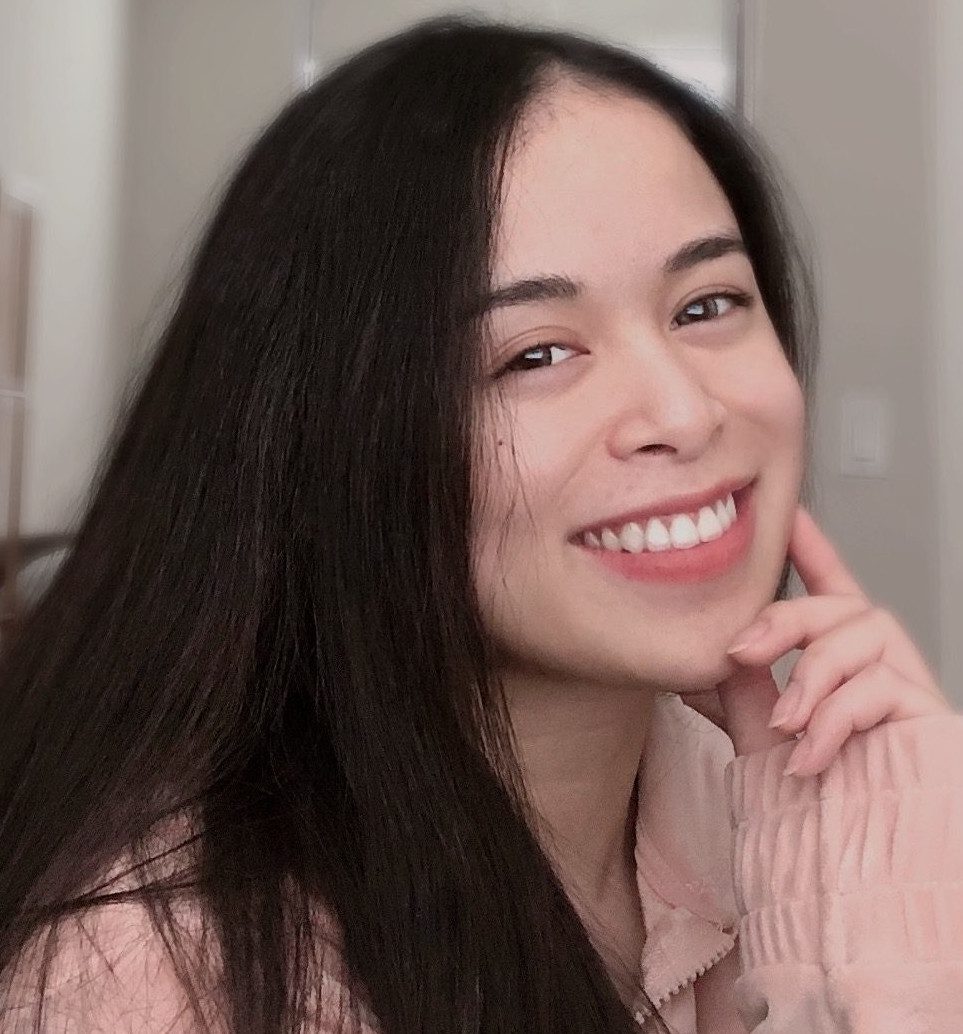
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
let i = 0; let coffees = ["Cortado", "Macchiato", "Mocha", "Latte"]; let numberOfCoffees = coffees.length +1; function iterateOverArray() { console.log(coffees[i]); i++; } var printCoffees = setInterval(iterateOverArray, 1000); setTimeout(() => { clearInterval(printCoffees); }, numberOfCoffees * 1001);
We have assigned our setInterval() method to its own variable called printCoffees. We have then declared a setTimeout() method which cancels the printCoffees time interval. This method will execute after every coffee in our list has been iterated over.
We calculate how many seconds the setTimeout() method should wait by multiplying the number of coffees to print out by 1001. Four coffees are stored in our list. Four multiplied by 1000 is 4000, which is four seconds.
We have chosen the number 1001 so that our timer stops after the last array item has been printed to the console. If we chose the number 4000, our printCoffees method would halt before it printed the last item in our “coffees” array to the console.
Let’s run our code:
Cortado Macchiato Mocha Latte
Our code stops after all the items in our list have been printed to the console. It no longer continues to execute indefinitely. This is because we have used the clearInterval() method to stop the setInterval() method.
setInterval: A Visual Example
Let’s use some HTML to show off how the setInterval function works. We’re going to create a simple website which shows our list of coffees. These coffees will be added one-by-one to the site. We’ll begin by creating a simple front-end for the project in a file called index.html:
<!DOCTYPE html> <html> <head> <title>Coffee List</title> </head> <body> <h1>Coffee List</h1> <ul id="coffees"> </ul> </body> <script src="scripts.js"></script> </html>
Our interface looks like this:
So far, there is not much going on. That’s because we need to write some JavaScript to make our coffees appear on the web page.
We’ll start our JavaScript by selecting our list. Open up a file called scripts.js and paste in this code:
var ul = document.getElementById("coffees");
This code selects our list element. You can learn more about getElementById in our tutorial on JavaScript getElementById. Then, we’ll use our code from earlier, with a few changes, to display the coffees on the web page:
var ul = document.getElementById("coffees"); let i = 0; let coffees = ["Cortado", "Macchiato", "Mocha", "Latte"]; let numberOfCoffees = coffees.length +1; function iterateOverArray() { var li = document.createElement("li"); li.innerText = coffees[i]; ul.appendChild(li); i++; } var printCoffees = setInterval(iterateOverArray, 1000); setTimeout(() => { clearInterval(printCoffees); }, numberOfCoffees * 1001);
In the iterateOverArray function, we have added in some new code. We have first used createElement() to create a new li element. We have then set the contents of that li element to be equal to the name of a coffee in our list.
Our code adds the li element to the ul element in our list. This process repeats until every item in our coffees list has been added to the web page, like in our last example.
Let’s run our code and see what happens. After four seconds, our code returns:
There is a one second gap between each item in the list being added to the web page.
Conclusion (and Challenge)
setTimeout and setInterval are JavaScript timing events. The setTimeout method executes a function after a delay. setInterval calls a function repeatedly with a delay between each call.
Are you looking to level up your skills with these methods? Try to create a visual interface using HTML and JavaScript for a memory game.
The game should show a series of five words for a user to remember. After the words have been displayed, the user should be asked which ones they remember. If you are looking for more help learning JavaScript, read our guide on the best tutorials for JavaScript beginners.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.