To replace text in a JavaScript string the replace()
function is used. The replace()
function takes two arguments, the substring to be replaced and the new string that will take its place. Regex(p) can also be used to replace text in a string.
Replacing text within a string is a common operation in any programming language. For example, you may want to remove certain symbols from a string to make sure your program will work.
Whatever the reason, knowing how to replace text in a JavaScript string can be useful.
In this guide, we’re going to break down the JavaScript string replace() function and explore how it can be used to amend the text.
JavaScript Replace() Syntax
The JavaScript replace() method searches a string for a pattern or regular expression. If that pattern is found in the string, it is replaced with a specified value. replace() returns a new string. The original string is not changed.
The replace() method accepts two arguments:
- The pattern or regular expression for which replace() should search; and
- A string which should replace any instances of the pattern you specified in the first argument.
Let’s take a look at this syntax:
var name = "Pieter"; console.log(name.replace("Pi", "P"));
Our first argument, “Pi”, is the pattern whose contents we want to replace. The second argument, “P”, is the text we want to replace our pattern with. So, our code replaces “Pi” with “P”.
Our code prints “Peter” to the JavaScript console. Our output is a new string because strings cannot be modified in JavaScript. They are immutable.
The replace() method is added to the end of our string. You should not specify the string from which you want to replace values. Only the pattern for which you want to search and the text which should replace any instances of that pattern are accepted arguments.
replace() JavaScript Example
Let’s say, for example, you want to replace the word interesting with intriguing in a string. How would you do that? We could employ the use of the replace() method:
var ourInterestingString = "This string is interesting."; var ourIntriguingString = ourInterestingString.replace("interesting", "intriguing"); console.log(ourIntriguingString);
Our code returns a new string which is as follows:
"This string is intriguing."
On the first line, we declare a JavaScript variable called ourInterestingString. This variable contains the value This string is interesting. On the next line, we replace the word interesting with intriguing, using the JavaScript string replace() method.
We can see that the word “interesting” has been replaced with “intriguing” in our string.
JavaScript Replace Multiple Substrings
If we want to replace multiple parts of our string, we can do that by chaining our replace() functions. Chaining means that we place multiple replace() statements after one another. Here’s an example:
var ourInterestingString = "This string is interesting."; var ourNewString = ourInterestingString .replace("This", "Our") .replace("string", "code") .replace("interesting.", "amazing!"); console.log(ourNewString);
Our code returns the following:
"Our code is amazing!"
Our code replaces all occurrences of the words “This”, “string”, and “interesting”, with the words “Our”, “code”, “amazing!”, respectively. Then it substitutes three words with three others in our string using the replace() function.
JavaScript String replace() Using Regex
Regular expression, or regex(p), defines a search pattern. When you’re looking to find or replace characters within a string, using regex can be useful. Regexp objects give us more control over our replace() function, so we can do more exciting replacements.
Here’s an example of using regex to replace the letter e with the letter f:
var ourInterestingString = “This string is interesting.”; var ourNewString = ourInterestingString .replace(“This”, “Our”) .replace(“string”, “code”) .replace(“interesting.”, “amazing!); console.log(ourNewString);
The output for our code is as follows:
"This string is interesting."
Our code first declares the variable ourInterestingString, and assigns it the value This string is interesting. Then, our code replaces every e throughout the string with the letter f, and the function returns our new string.
We use the /e/g/ regex expression to do this. This pattern will replace every e that comes up throughout the whole string (which is what the g signifies).
If you’re looking to learn more about regex, check out Regexr, which allows you to easily try out different expressions.
Summary
The JavaScript replace() function allows us to replace one part of a string with another and return the newly modified string. The Regex() function allows us to do the same thing but with greater control.
In this tutorial, we broke down how to use the JavaScript replace() function. We also discussed how regex could be used to perform more advanced replace functions on a string.
To learn more about how to code in the JavaScript programming language, read our How to Learn JavaScript guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
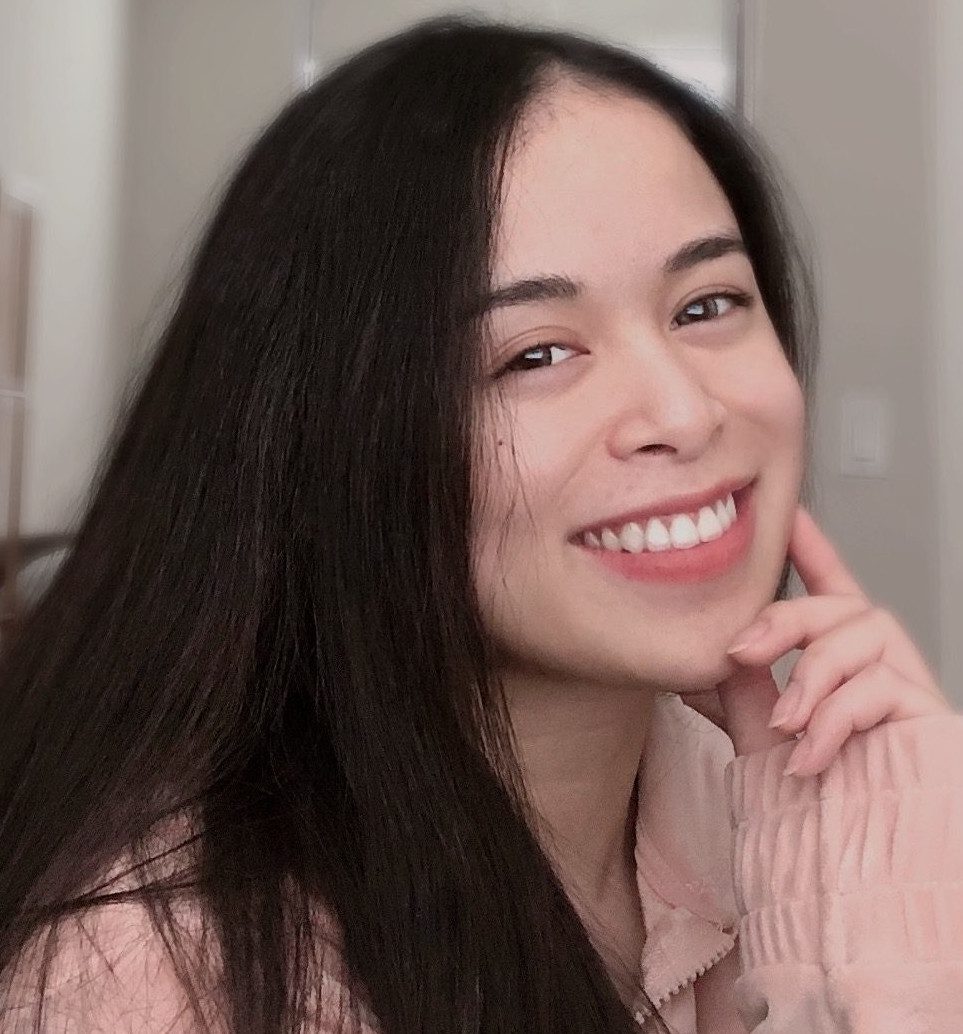
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot