The JavaScript Object.values() method retrieves a list of the values in an object. You can iterate over the result of the values() method to see the contents of each value in the object.
Objects contain keys and values. Sometimes, you will only want to retrieve the values in the object. Say we have an object which stores the names of all the people on a leaderboard. We may only want to retrieve their names from the object rather than the keys associated with their names (i.e. their positions).
That’s where the Object.values() method comes in. This method lets you see all the values in an object. In this guide, we’re going to discuss how to use the Object.values() method. We’ll refer to a detailed example to help you get started.
JavaScript Object.values()
The JavaScript Object.values() method takes in the object and returns its enumerable properties in an array. Values are ordered as if you were to loop over the object manually and push them into an array.
Let’s take a look at the syntax for this method:
let players = { a: "lucy", b: "harry" } console.log(Object.values(players))
The Object.values() method accepts one argument: the name of the object whose values you want to see. In this syntax, we print the result of the method to the console.
This code returns the names of the players in our JavaScript object: lucy and harry. These names are returned in a list, which we print to the JavaScript console:
["lucy", "harry"]
Notice that the values are in the same order as they were in the dictionary.
The Object.values() method is an easy way to retrieve values from an object. The alternative is to create a new list and iterate over the object with a for loop, adding each value to the new list. This method takes more time and more code to write.
Let’s look at an example of this method in action.
Object.values() JavaScript Example
Suppose we have an object that contains information about San Jose, a city in California. We want to retrieve all the information about this city without retrieving the labels associated with each piece of data.
Consider this key-value pair:
city: "San Jose",
We only want to retrieve the city name, rather than the label city.
Let’s take a look at a program that will let us do this:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width"> <title></title> </head> <body> <div id="root"></div> <script async defer> const cityData = { city: "San Jose", state: "California", area: 181.36, land: 178.24, water: 3.12, urban: 342.27, metro: 2694.61, elevation: 82, population: 1021795, timezone: "Los_Angeles/Pacific", website: "www.sanjoseca.gov" } let arr = Object.values(cityData); let root = document.getElementById('root'); root.innerHTML = JSON.stringify(arr); </script> </body> </html>
First, we set up a standard HTML page with a <head> tag, a <body> tag, and some basic meta information in the <head> tag.
We use a <script> tag in which we write our JavaScript code. Within this tag, we declare a JavaScript object. This object is called cityData.
Next, we retrieve the values in this object using the Object.values() method. We then select a HTML <div> tag with the id root in our code. This is the use case for the JavaScript getElementById method above.
After we select this <div> tag, we replace the contents of the <div> tag with the result of the Object.values() method.
Our code returns a list:
["San Jose","California", 181.36, 178.24, 3.12, 342.27, 2694.61, 82, 1021795, "Los_Angeles/Pacific", "www.sanjoseca.gov"]
We can see all of the values in our original object. These values are stored as a list.
Conclusion
The JavaScript Object.values() method returns a list of the values in an object. This method accepts one argument: the name of the object from which you want to retrieve values.
Do you want to learn more about coding in JavaScript? Check out our How to Learn JavaScript guide. You’ll find top learning tips and a list of courses for beginner and intermediate-level developers.
Check out these tutorials if you want to learn more about JavaScript objects:
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
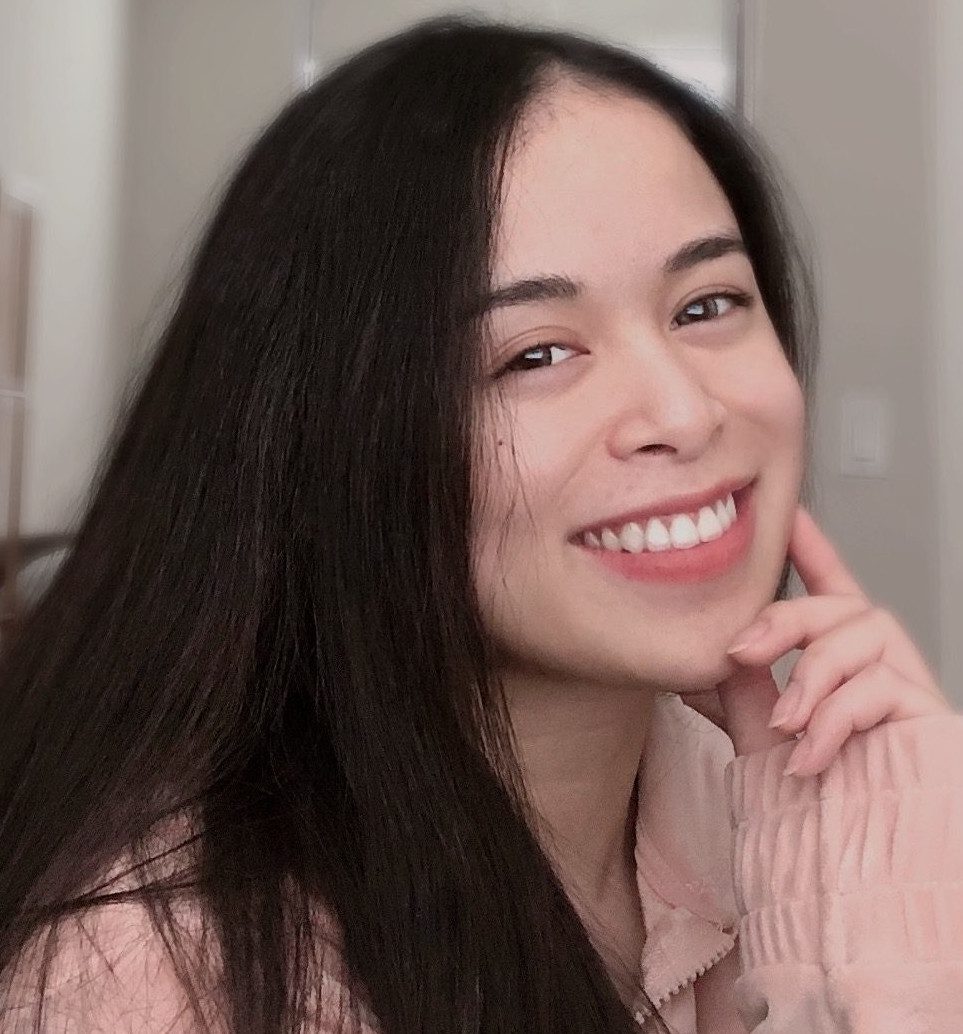
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot