Computers are really good at math. It’s in their metaphorical blood, considering computers are powered by the 1s and 0s that we call binary. When you’re coding, there’s probably going to be a time when you want to perform some mathematical operation.
In JavaScript, math comes up all the time. You can use math to calculate the size of a window. You can use it to calculate whether a user is old enough to use your site. You can use math to add together two numbers provided by the user.
In this guide, we’re going to talk about how to perform mathematical operations in JavaScript using the five main mathematical operators.
Mathematical Operators: A Refresher
JavaScript is made up of a number of operators. Some operators allow you to work with strings, whereas others help you perform JS math functions. In this guide, we’re going to focus on a special type of operator called an arithmetic operator.
Arithmetic operators are the symbols used to perform mathematical tasks. These JS operators are somewhat similar to the ones that you learned in school. For this article, we’re going to focus on six operators:
- Addition (+)
- Subtraction (-)
- Division (/)
- Multiplication (*)
- Modulo (%)
- Power (**)
Let’s get started and delve into each of these.
Before we begin, it’s worth noting that all JavaScript numbers are stored as numbers. There is no separate data type for floating-point (decimal) numbers or whole numbers. We simply refer to them all as “numbers.”
Adding and Subtracting
The JavaScript symbols for adding and subtracting JS numbers are the same as we use in our day-to-day lives for math. How convenient! We can add numbers together by stating the numbers we want to add, separated by a plus sign:
console.log(5 + 9);
Our code returns: 14. We could perform a subtraction sum by substituting our plus for a minus:
console.log(5 - 9);
Our code returns: -4.
JavaScript math is capable of working with both positive and negative numbers.
When you’re working with a math sum, you are probably not going to just print it to the console. That’s where variables come in. You can assign the numbers in your math problems to store all the numbers with which you are working:
var a = 10; var b = 20; var c = a + b; console.log(c);
Our code returns: 30
. We’ve assigned the value 10
to a
and the value 20
to b
. Then, we’ve used the variable c
to add these two numbers together. It’s that simple!
Multiply and Divide
Let’s go up a level and talk about multiplication and division. Unlike the previous examples, we’re going to have to learn two new operators:
- The asterisk (*) represents multiplication.
- The forward-slash (/) represents division.
Let’s say that we own a cookie store and we want to figure out how many kilograms of flour we need. Each batch of cookies requires 2kg of flour, and we want to bake five batches. We could calculate how many kilograms of flour we need using this code:
var batches = 5; var flourQuantity = 2; var flourNeeded = batches * flourQuantity; console.log("You need ", flourNeeded, "kgs of flour.");
Our code returns: You need 10kgs of flour
.
You can use the forward slash to perform division operations.
Let’s say that each batch contains 40 cookies and you want to divide them into packs. Each pack contains 5 cookies. Now, you want to find out how many packs you could make. This could be done using the following code:
var batchQuantity = 40; var pack = 5; var packsMade = batchQuantity / pack; console.log("You can make ", packsMade, "packs of cookies.");
Our code returns: You can make 8 packs of cookies
.
Modulo
The modulo operator may not be as commonly used as the others but it’s still an important part of JavaScript. The modulo operator calculates the remainder of a number after it’s been divided.
In JavaScript, the modulo operation is represented using a percentage sign. Our last example showed us dividing numbers that could be split up evenly. As you know, not all math problems go this way; some return remainders.
Let’s go back to our cookie example from earlier. Suppose we’ve overestimated how many cookies we can bake in each batch. It turns out each batch will only yield 37 cookies. To find out how many packs we can make from our batch, we could use this code:
var batchQuantity = 37; var pack = 5; var packsMade = batchQuantity / pack; console.log("You can make ", packsMade, "packs of cookies.");
The trouble is that we’ve got a decimal number! That’s where the modulo comes in. We could update our code to calculate the remainder of the cookies available:
var batchQuantity = 37; var pack = 5; var packsMade = batchQuantity / pack; console.log("You can make ", Math.floor(packsMade), "packs of cookies."); var remainder = batchQuantity % pack; console.log("You'll have ", remainder, " cookies left over.");
Our code returns:
You can make 7 packs of cookies. You'll have 2 cookies left over.
We’ve made a couple of modifications to our code. First, we have rounded the value of packsMade
down to the nearest whole number. We used Math.floor()
for this purpose. This allows us to see that we can make 7 full packs of cookies.
Then we used the modulo operator to calculate the remaining cookies that would be left over. This tells us that, after dividing our packs, we will have two cookies remaining.
Power
Raise x to the power of y. It sounds quite complicated, especially when you start using words like exponentiation. But it doesn’t have to be difficult.
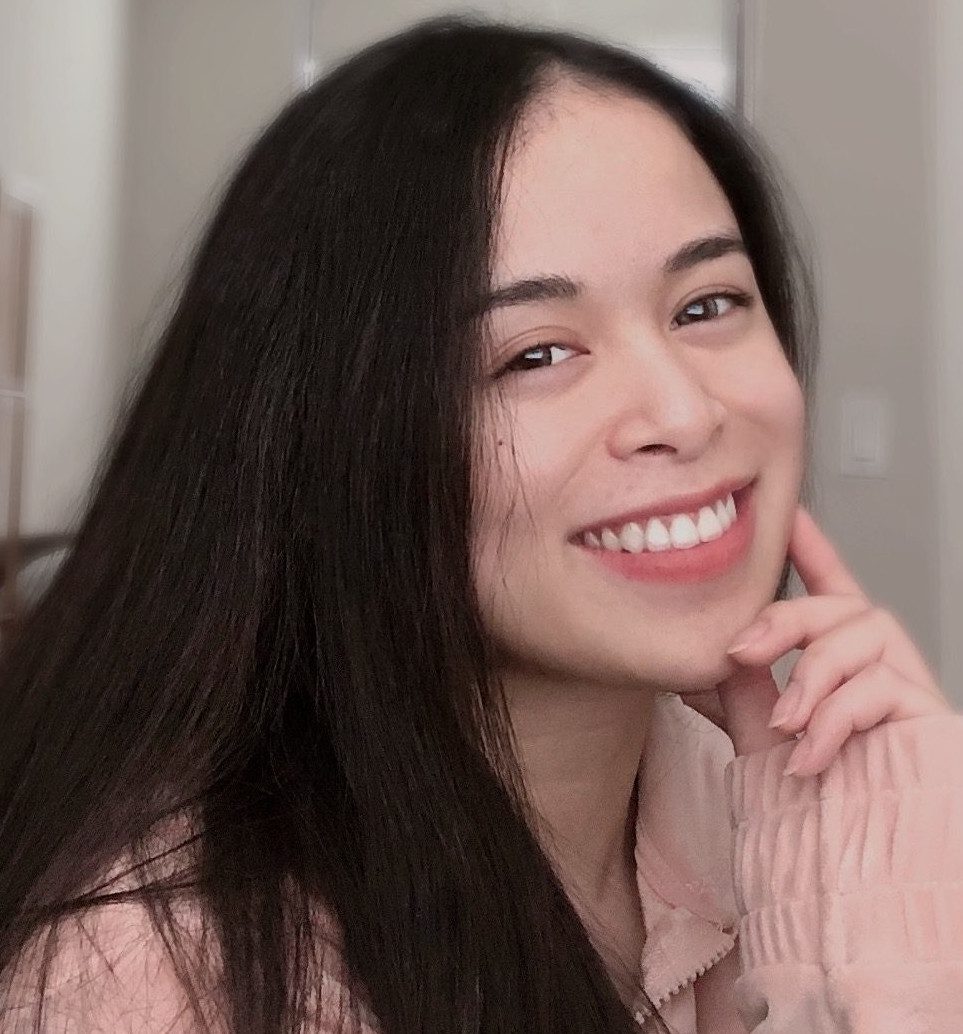
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
In JavaScript, two asterisks (**) represents raising a number to a power:
console.log(7 ** 3);
This code returns: 343
.
This is another way of writing 7 * 7 * 7, but it is shorter and easier to read.
Order of Operations
Math has a specific order of operations. This describes what operations you should perform in what order when you’re presented with a math problem.
One of the most common ways to remember this order is BODMAS:
- Brackets (())
- Order (power)
- Division
- Multiplication
- Addition
- Subtraction
This tells us that we should do everything in brackets first, then all the power calculations, then division, and so on. Consider the following problem:
var problem = (5 * 3) + 2; console.log(problem);
The answer to this problem is: 17. JavaScript first calculates 5 * 3 because it is in brackets. Then it adds two to the result of that calculation.
Conclusion
JavaScript offers a range of arithmetic operators that you can use to perform mathematical operations. Some of these operators, like addition, are the same as what we would use when evaluating a math problem; others have their own symbols.
Remember that when you evaluate a math problem you need to consider BODMAS, the order of operations in which the problem will be read.
Now you’re ready to start doing math in JavaScript like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.