JSON, short for JavaScript Object Notation, is a way of storing data. In JSON objects, values are mapped to labels called keys. JSON objects can store any data type, including strings, integers, and other JSON values.
JSON is a standard file format that allows you to store and share data. Programming languages like JavaScript, Python, and PHP all support manipulating and reading JSON data.
In this guide, we’re going to explore how to use JavaScript JSON. We’ll explore the basics of the JSON format and how a JSON object compares to a JavaScript object.
What is JSON?
JSON is a method of storing and sharing data. It is based on the JavaScript object syntax. The difference is that JSON is based entirely on text, whereas objects are not. JSON stands for JavaScript Object Notation.
While JSON is based on principles from the JavaScript programming language, it is used in many other languages like PHP, Java and Ruby.
You can store JSON data as a file or as a value in a program. For instance, a program may include a file called mtg_cards.json which stores a list of Magic the Gathering cards. We could store that data in a JavaScript variable called mtg_cards.
Here’s the structure for a piece of data stored in JSON:
{ "card_name": "Black Lotus", "status": "banned", "card_type": "Mono Artifact" "mana": "0" }
JSON stores data using a structure called key-value pairs. Key-value pairs are pieces of data which use the following structure: “key”: “value”.
card_name is an example of a key. Values are on the right-hand side of a colon. In JSON, you store key names as strings, but values can be any data type such as a boolean or an integer.
JSON is often APIs to send data. For example, say you use the Airtable or Fitbit APIs. You’ll need to send data and read data in the JSON data format.
How to Access JavaScript JSON Data
To access JSON data in JavaScript, you can use either square brackets or dot notation. The square bracket notation is similar to how you would retrieve a value from a JavaScript array. Instead of specifying an index position, you specify the name of the key whose value you want to retrieve.
Consider our object from earlier, now formatted as a JSON object:
var black_lotus = { "card_name": "Black Lotus", "status": "banned", "card_type": "Mono Artifact", "mana": "0" }
In this example, we’ve assigned our JSON object to the variable black_lotus. Let’s suppose you want to find out the card type for Black Lotus. This is a banned card in the trading card game Magic the Gathering.
Square Brackets
Using square bracket notation, we can find out the card type of the Black Lotus card.
To use this notation, we need to specify the name of the object from which we want to retrieve a value. Then, we specify the name of the key associated with that value. You must enclose the key name in quotation marks and square brackets.
The following code retrieves data on the Black Lotus’ card type:
console.log(black_lotus["card_type"])
Our code returns: Mono Artifact. We’ve double-quoted our key name, surrounded it by square brackets, then we appended it to the end of the black_lotus variable. This allows us to access data stored with the name card_type.
Dot Notation
Dot notation is where you access an object by specifying an object name, followed by a dot. You then specify the name of the key whose value you want to access.
To access the card type of Black Lotus using dot notation, you could use this code:
console.log(black_lotus.card_type)
Our code returns: “Mono Artifact”.
We have specified the name of our object (“black_lotus”), followed by the key whose value we want to access (“card_type”).
JSON Conversion
Often, when you’re working with an object, you’ll want to convert it into a JSON string, or vice versa. This is common practice if you want to send data across a web server.
JSON is easier to manipulate in technologies like JavaScript. It would be very difficult to extract all the values from a plain string.
At first, you’ll want to convert an object to a string so you can send it over a server. Then you’ll want to convert it back to an object so you can treat the data like an object.
To convert an object to a JSON string, you can use the JSON.stringify() function. Suppose we want to convert our Black Lotus object from earlier into a string. We could do so using this code:
var black_lotus = { "card_name": "Black Lotus", "status": "banned", "card_type": "Mono Artifact", "mana": "0" } var string_black_lotus = JSON.stringify(black_lotus) console.log(string_black_lotus)
Our code returns the following JSON text:
“{“card_name”:”Black Lotus”,”status”:”banned”,”card_type”:”Mono Artifact”,”mana”:”0″}”
As you can see, our JSON data is a string. You can use the JSON.parse() method to convert this string back into a JSON object:
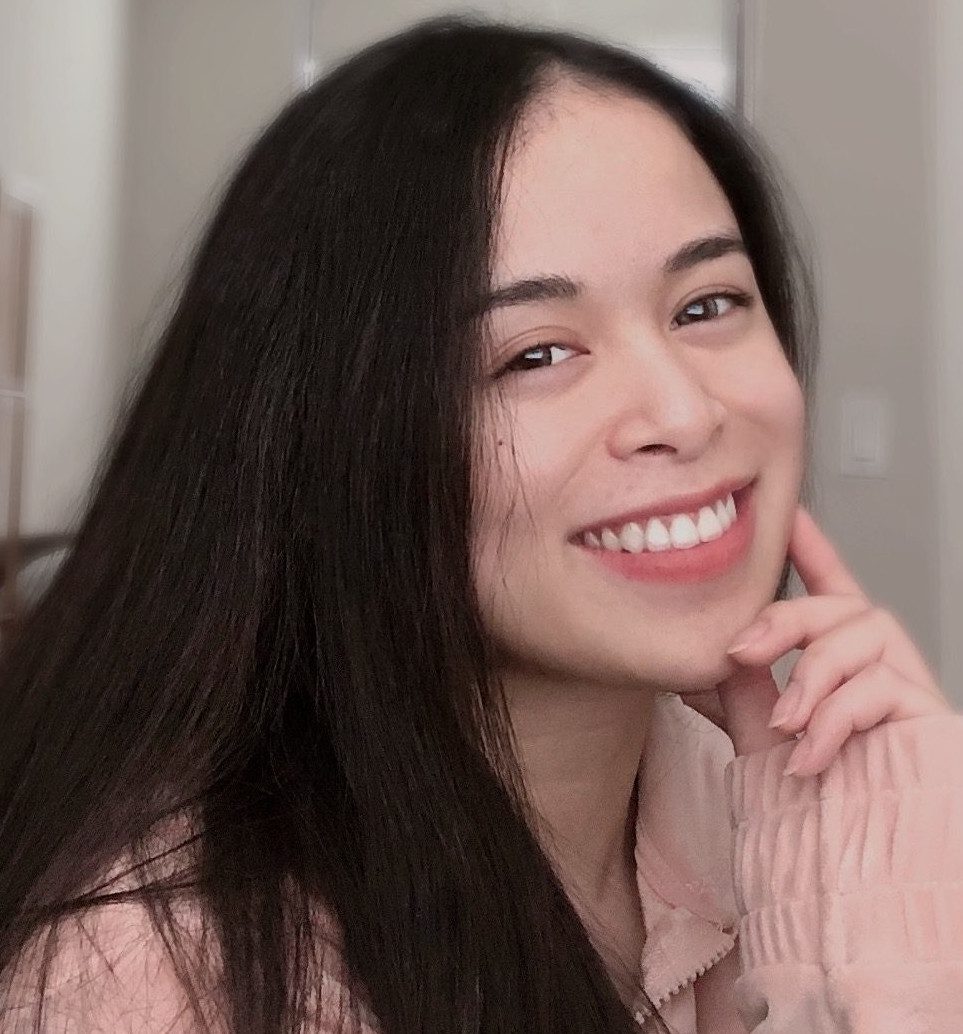
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
var black_lotus_object = JSON.parse(string_black_lotus) console.log(black_lotus_object)
This code returns:
{ "card_name": "Black Lotus", "status": "banned", "card_type": "Mono Artifact", "mana": "0" }
As you can see, our data is now an object.
JSON vs JavaScript Object
The JSON notation was based on JavaScript objects.
Because JSON was designed to be supported in multiple programming languages, it is not exactly the same as a JavaScript object. For instance, JSON is supported in PHP and Python. Each programming language that supports JSON has its own unique implementation.
Whereas keys in JavaScript objects are not stored in quotation marks, keys in JSON data must be stored in quotation marks.
If you look in a JSON file, you’ll see all the keys are surrounded in quotation marks. If you look at a JavaScript object, you’ll see the keys may not be surrounded in quotation marks.
Both JSON and JavaScript objects store data in a key-value structure.
Conclusion
JavaScript supports storing data in JSON. JSON, short for JavaScript Object Notation, is a way to store related data in one object. Each value in the object has its own label, also called a key.
Now you’re equipped with the knowledge you need to use JSON in JavaScript like a pro!
Do you want to learn more about JavaScript? Check out our How to Learn JavaScript guide. You’ll find top tips on how to learn JavaScript and a list of learning resources for beginner and intermediate developers.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.