The JavaScript forEach
loop is an Array method that executes a custom callback function on each item in an array. The forEach
loop can only be used on Arrays, Sets, and Maps.
If you’ve spent any time around a programming language, you should have seen a “for loop.” Using a for loop, you can run through a set of data or a function for a certain number of times. For example, you could have a list of names, also known as an array, and a for loop will go through 100 of those names.
There is a more efficient way to write a for loop if you are working with a collection, like a list or a set. That’s where JavaScript forEach loops come in. A forEach loop will run a JavaScript callback function for each item in a list. Then, the loop stops.
JavaScript for Loop Refresher
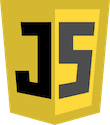
The JavaScript for loop executes a function a predefined number of times. For loops are useful if you need to run the same block of code multiple times. Using a for loop instead of copying your code helps reduce redundancy. This improves the readability of a code base.
Let’s write a for loop that prints a value to the console ten times:
for (i = 0; i <10; i++) { console.log(“test”) }
This loop will execute the console.log(“test”)
line of code ten times, once for each time the loop is executed.
How does this work? The for loop is split up into three components.
The first component is i = 0. This is where we define a JavaScript variable that keeps track of how many times our loop has been run.
Next, the i < 10 code defines how many times the loop should be run (in this case, 10). The i++ component executes after each iteration. This component adds one to the “i” variable counter.
The JavaScript forEach
Loop
forEach is a JavaScript Array method. It is used to execute a function on each item in an array. Lists, sets, and all other list-like objects support the forEach method.
We’re going to write a loop that displays a list of companies to the console. Instead of using a for loop, we’re going to use a forEach loop. This is because we are working with a list of companies.
The following code prints each value from a list of companies to the console:
const companies = ["Apple", "Google", "Facebook"]; companies.forEach(company => { console.log(company); });
Our output for our code is as follows:
Apple Google Facebook
For each item in our “companies” list, we print out the item to the console. This is different to how a regular for loop works.
JavaScript forEach vs for Loop
In a traditional for loop, you would have to access each item in the “companies” list by list indexing. A forEach loop gives you direct access to each item in an array.
Indexing is where you specify the index number of the value you want to access in a list. This index number can be retrieved from the counter that a for loop contains. We discussed this counter earlier.
With a forEach loop, we can access each item in our list individually. In our last example, we created the variable “company”. This variable represented an individual company over which our forEach loop was iterating.
forEach loops accept a callback function whereas for loops do not. In a for loop, all of your code is enclosed in the main body of the loop. In a forEach loop, you must write a function which will be executed for each item in the list over which you are iterating.
JavaScript forEach Callbacks
The forEach method accepts a callback function. This is a function passed into another function as an argument. Callback functions are executed inside the function in which they appear.
Let’s go back to our last example:
companies.forEach(company => { console.log(company); });
Our callback function comes after the arrow (=>). This arrow denotes an arrow function. But, callback functions do not need to be arrow functions.
We can define the function that we want to execute elsewhere:
const companies = ["Apple", "Google", "Facebook"]; function printValue(company) { console.log(company); }); companies.forEach(printValue);
In this code, we have defined a new function called printValue. We have passed this function into our forEach loop as an argument.
This function is defined independently of our forEach loop. Our code works because the forEach loop accepts any type of function. We do not need to specify an arrow function.
The advantage of this approach is that our code is easier to read. It is clear that the printValue() function is executed for each item in the “companies” list.
Tracking the Current Index
We can track the index value of the list item we are viewing with a forEach method. We can do this by specifying an “index” variable in our callback function.
We’ve decided that we want to see both the index of the company and the contents of the item we are viewing. This can be achieved by adding an “index” variable:
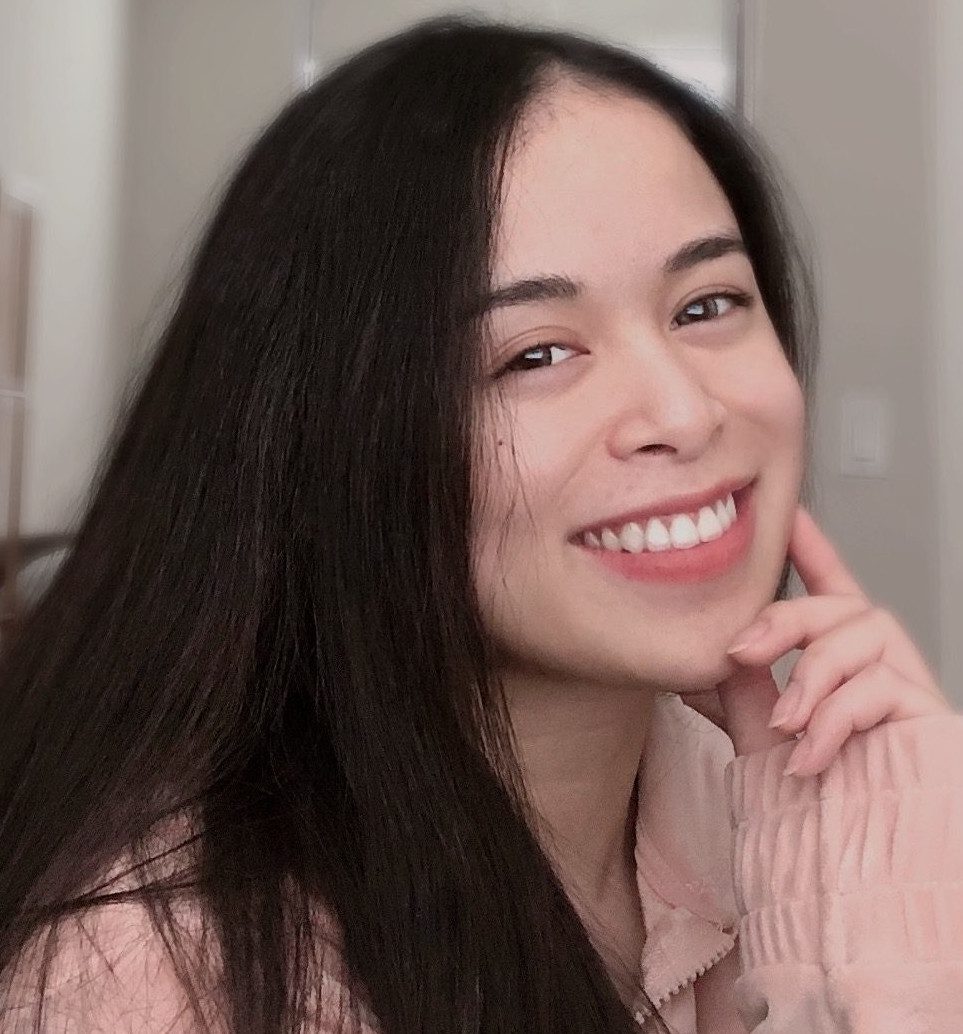
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
const companies = ["Apple", "Google", "Facebook"]; function printValue(company, index) { console.log(`${index}. ${company}); }); companies.forEach(printValue);
Let’s run our code and see what happens:
0. Apple
1. Google
2. Facebook
We can see both the name of each company and its index value. The “index” variable represents each index value.
“index” can be named whatever you want, as long as it comes after the first variable in your callback. The first variable is reserved to track the item over which the loop is iterating.
Use Cases of JavaScript forEach
You should use the forEach method if you want to iterate over array items. The map() and reduce() methods are more effective if you need to calculate a result depending on the values of a list.
JavaScript forEach loops are most useful when you need to do something with every item in an array in JavaScript, not just a few.
The same applies to for…in loops. If you’re looking to iterate through every object in an array, a for…in loop would work.
On the other hand, if you need to write something more customizable—perhaps with more rules—a “for loop” may be better.
In addition, forEach calls are part of JavaScript 1.6. This means that the forEach method it has browser support across most of the main browsers.
And there you have it: JavaScript forEach loops in a nutshell!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.