A JavaScript for loop executes a block of code as long as a specified condition is true. JavaScript for loops take three arguments: initialization, condition, and increment. The condition expression is evaluated on every loop. A loop continues to run if the expression returns true.
Loops repeat the same block of code until a certain condition is met. They are useful for many repetitive programming tasks.
For example, you may have two lists of names—first names and surnames—that you want to merge into a list of full names. You could write a line of code for every name, but that could require hundreds of lines of code if the list is long. In addition, what if you aren’t sure how long the list will be? Here you could use a loop that repeats a similar block of code merging each list item.
There are two main types of loops used in JavaScript: while loops and for loops. While loops are based on a condition and run while that condition is equal to true. For loops run while a certain set of conditions are true. They can count the number of iterations the loop makes.
In this guide, we are going to explore the basics of JavaScript for loops. We’ll also discuss the for… in and for…of JavaScript loops, and use a few examples to illustrate how these loops work in JavaScript.
JavaScript for Loops
A for loop runs the same code repeatedly as long as a condition is met. Each iteration of a loop runs the same code with a different value. The syntax for a for loop is as follows:
for (initialization; condition; increment) { // Execute code }
This syntax is rather complex, so let’s break it down and define each term we have used.
Initialization is used to declare a counter variable. This variable that we keeps track of how many times our loop has been executed.
Condition is the condition that is evaluated before each loop starts. If the condition is equal to true, the code within the for loop will run. If the condition becomes false, the loop will stop running.
Increment updates the loop counter each time the loop executed.
Let’s use a basic example to show how a for loop works in JavaScript. Here’s a loop that prints out the numbers between 0 and 5:
for (let i = 0; i < 5; i++) { console.log(i); };
Our code returns the following:
0 1 2 3 4
You can see that our code has been executed repeatedly until our loop condition has been met. When the number 4 is printed to the JavaScript console, our loop stops. This is because we instructed our loop to print out numbers in the range of 0 and 5.
Components of a For Loop in JavaScript
Let’s break our example down in terms of the components of a for loop:
Initialization
Our loop’s initialization declares a variable that keeps track of how many times our loop has executed. In the above example, we used let i = 0;. This tells our program to start a counter called i that has the initial value 0.
Typically, initialization variables have the name i. But, you can use any variable name you want.
Condition
Our loop uses the i < 5 condition. This tells our program that our loop should only run when the i variable is less than 5.
If i is equal to or greater than 5, our loop will stop.
Increment
At the end of our for loop, our code uses i++, as an increment. This feature tells our program to add 1 to the variable i each time the loop is executed.
For Loop JavaScript: How it Works
Now that we’ve broken down our loop, we can discuss how the loop works as a whole. Here’s the code from the loop we used above:
for (let i = 0; i < 5; i++) { console.log(i); };
To start, we declare a for loop. This loop has an initializing variable set to 0. So, our counter starts at 0.
Then, we state that our loop should run when i is less than 5.
Our code increments the i counter by one every time our loop is run. If our condition is met, the code within our loop is run, which gives us the result we saw above. So, our code loops through a block of code until i is equal to or greater than five, then our code stops.
If we created a situation where our condition always evaluates to true, the loop would become infinite. Infinite loops continue indefinitely unless we add a break statement.
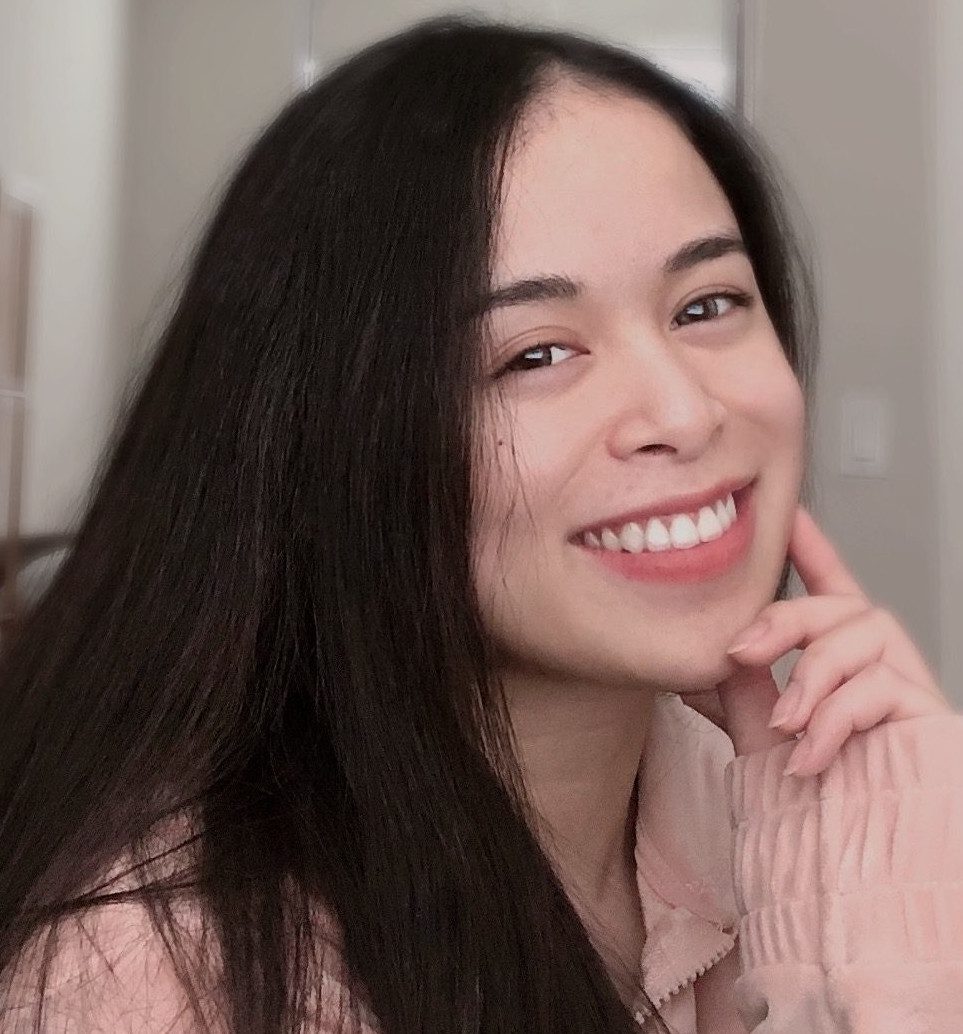
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our code then prints out i when the for statement is executed.
It’s worth noting that every argument in a for loop is optional. For example, if we have already declared an initialization variable, we don’t need to declare a new one in our for loop. Below is an example of a for loop being used with two arguments: a condition and an increment.
let i = 0; for (; i < 5; i++) { console.log(i); };
Our code returns:
0 1 2 3 4
We have to include the semicolon even though we have not specified an initializing variable for our loop. This is because semicolon is used to tell the program where the initialization, condition, and increment appear. Without a semicolon, JavaScript cannot interpret our loop.
JavaScript For… In Loops
for…in loops can be used to iterate over the items within an iterable object. Here’s the syntax for a for…in loop in JavaScript:
for (variable in object) { // Execute code }
for…in loops are useful if we want to run a certain block of code based on the number of attributes in an object. For example, we may have a student object whose properties we want to print out to the console.
Here’s an example of a for…in loop that will loop through every item in a student object and print out each attribute:
const student = { name: "Mark Redmond", gpa: 3.7, age: 17 }; for (item in student) { console.log(item); }
Our program returns the following:
name gpa age
As you can see, our program has iterated through each item in the student object and printed out its property name. If we wanted to print out the value of each item, we could use this code:
for (item in student) { console.log(student[item]); }
Our code returns the following:
Mark Redmond 3.7 17
We used the student[item] code to get the value of each item in our iterable object. Then, we printed the item to the console using console.log().
JavaScript For…Of Loops
For…in loops are useful when you want to iterate over properties in an object. You should use a for…of loop if But if you want to iterate over items in an object.
Let’s iterate through an array of students and print their names to the console:
let students = ["Paul", "Andy", "Reba", "Erin"]; for (let student of students) { console.log(student); };
Our code returns the following:
Paul Andy Reba Erin
As you can see, our for…of loop has iterated through every item in the students array. Each item is printed to the console.
For…of loops are more concise than a regular for loop. This makes a for…of loop easier to read. The general rule of programming is to use the right tools for the job. For…of loops are easier to understand if you want to iterate over a list. So, you should use for…of loops when possible.
Similarly, we can use for…of on a string to iterate through its component characters. Here’s an example of this in action:
let name = "Paul"; for (let character of name) { console.log(character) };
The result of our code is:
P a u l
Our program has iterated through each letter in the name variable, then printed them out individually to the console.
This could be useful if you want to check whether a character appears in a string, for instance.
Conclusion
For loops are a useful feature in JavaScript that allow you to run a block of code while a certain condition evaluates to true.
In this article, we have broken down how for loops work, and how you can use them in JavaScript. We also discussed how to use the for…in and for…of loops in JavaScript. Now you’re ready to start automating repetitive tasks using for loops like a JavaScript master!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.