The JavaScript find()
function returns the first element in a list that meets a given requirement. The function is useful for finding specific values in an array.
When working with JavaScript, you may want to retrieve a specific value from an array. That’s where the JavaScript find()
function comes in. This function searches through an array and returns the first element that meets a certain criterion.
In this tutorial, we will discuss the basics of arrays in JavaScript. Then we’ll explore how you can use the JavaScript find()
function to get the value of the first item that meets a certain condition in an array.
JavaScript Array Refresher
Arrays are ordered lists of items that are used to store data. Arrays can contain zero or more items and are useful if you want to store multiple common values in a single variable. For example, you could use an array to store a list of shipments received at a warehouse or a list of books sold at a bookshop.
Arrays can contain any data type, including numbers, objects, and strings. In JavaScript, arrays are declared as a list of comma-separated values and are enclosed within square brackets. Here’s an example of an array that stores a list of colors in JavaScript:
var colors = ['Red', 'Orange', 'Yellow', 'Green'];
The above variable (colors
) was assigned an array of colors. Our colors
array contains four values. Having declared this array, we can retrieve individual items from it.
In JavaScript, items in an array are assigned an index value, starting with 0
. We can use these index values to retrieve an item or set of items. Here are the index values that JavaScript automatically assigns to items in the array we declared above:
Red | Orange | Yellow | Green |
0 | 1 | 2 | 3 |
Now, if we want to retrieve the value Orange
from our array, we can do so by referencing its index number. Here’s the code we would use to do this:
console.log(colors[1]);
Our code returns:
Orange
As you can see, our program returned the value at the index position 1
in our array.
JavaScript Find
You can use the JavaScript find()
method to locate a specific value within an array. The program will return the first item that meets the criterion you define. If more than one item meets that criterion, the program will return only the first item.
The find()
method takes in three arguments:
- element: what the
find()
function processes - index: the index value of the item that the
find()
function processes - array: the array on which the
find()
function is executed
Here’s the syntax for the find()
method:
array.find(function(element), index);
If the value you are searching for is not found within the array you specify, the find()
method will return: undefined
.
Let’s use an example to explain how the find()
method works in JavaScript. Let’s say that we are the owners of a bakery, and we want to check whether or not our product listing includes brownies, which we just started baking. We could use the following code to perform this action:
var products = ['Coffee Cake', 'Custard Slice', 'Millionaire Shortcake', 'Apple Pie', 'Carrot Cake', 'Brownie'];
var check_for_brownies = products.find(function(product) {
return product === 'Brownie';
});
console.log(check_for_brownies);
Our program returns the following: Brownie
.
There’s a lot going on in our code, so let’s break it down. On the first line, we declare an array called products
, which stores six different products offered by our bakery.
On the next line, we use the find()
method to check whether our array contains Brownie
. We do so by creating a function within the find()
method that checks for whether each product is equal to Brownie
(return product === ‘Brownie’
is the code we use above).
Then, on the final line, we print out our check_for_brownies
variable to see whether our program identified the word Brownie
in our array. Because Brownie
was in our products
array and we satisfied the condition, our program returns the value.
In addition, we can use a JavaScript arrow function to make our code more efficient. Here’s an example of an arrow function that performs the same action as above but in fewer lines of code:
var products = ['Coffee Cake', 'Custard Slice', 'Millionaire Shortcake', 'Apple Pie', 'Carrot Cake', 'Brownie'];
var check_for_brownies = products.find(function(product) {
return product === 'Brownie';
});
console.log(check_for_brownies);
Our find() method returns: Brownie
.
In addition, we can use the find()
function to search for an item in a list of numbers.
JavaScript Find Object in Array
In the above example, we used find()
to determine whether our bakery product listings included Brownie
. The array we were searching through was a simple list of strings.
But what if you want to search through an array of objects? You can use the find()
method to do this, too. Let’s say that you have a list of product names and prices stored as an array of objects, and you want to search this list. Specifically, you are looking to see whether Apple Pie
is in the array of objects.
You could use the following code to check if Apple Pie
appears in your list:
var products = [
{ name: 'Coffee Cake', price: 2.50 },
{ name: 'Apple Pie', price: 2.25 },
{ name: 'Brownie', price: 1.75 }
];
var check_for_apple_pie = products.find(product => product.name === "Apple Pie");
console.log(check_for_apple_pie);
Our code returns:
{ name: 'Apple Pie', price: 2.25 }
As you can see, our program searched through our list of objects and returned the one with the product name equal to Apple Pie
. This code works in the same way as it did in our previous example where we searched for brownies; the main difference is that instead of searching through an array of strings, we searched through an array of objects.
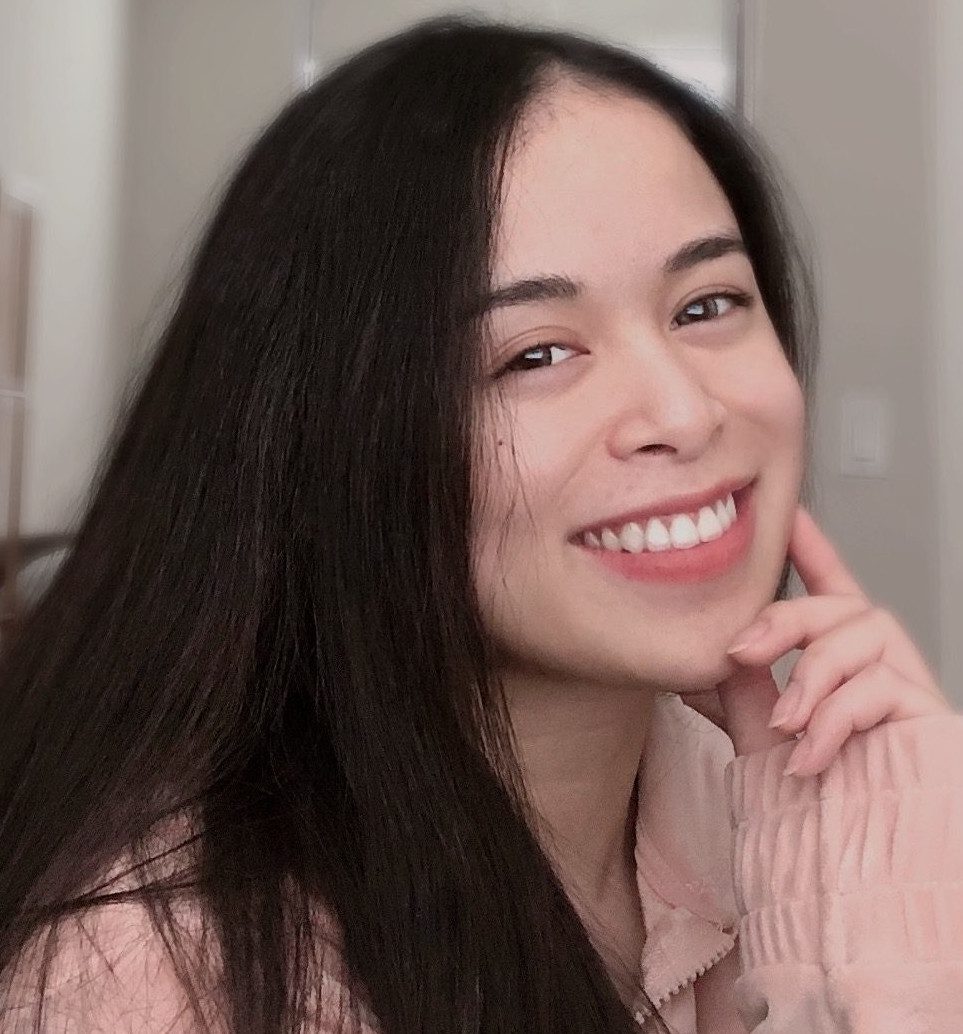
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
You can use the JavaScript find()
function to determine whether an array contains a particular value. If the array does contain the value for which you are searching, your program will return the first instance of that value; if the array does not contain the value, your program will return a null value.
In this tutorial, we explored the basics of arrays in JavaScript, and we discussed how to use the find()
method to determine whether an array contains a specific value. We also discussed how to use the find()
method with an array of objects.
Now you’re ready to start using the JavaScript find()
method like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.