JavaScript is a popular programming language that is one of the core technologies of the Internet. It is a code editor used for client-side web behavior, as well as desktop apps and mobile app development. It is a cross-platform, object-oriented scripting language used client-side and server-side for web page interaction. If you’re new, it’s easy to get help with JavaScript.
JavaScript (JS) is one of the easiest programming languages to learn, which makes it one of the go-to languages for coding beginners. One of the best ways to learn, practice, and enhance your skills with this code editor is through basic exercises and practice projects. These will allow you to understand the language better. In this article, we’ve curated a list of basic JavaScript exercises as well as additional resources for you to work on your skills. If you’ve ever asked, “Where can I practice JavaScript?” then read on.
JavaScript Exercises to Help You Learn JavaScript
Many coding platforms offer exercises, interactive courses, and project ideas to help beginners and advanced learners practice coding and learn how to use JavaScript. You can find JS exercises on many of these platforms to help build and enhance your skills. These practice challenges tackle different JavaScript tasks essential to your career’s progress.
10 JavaScript Exercises and Practice Problems (With Solutions)
1. JS Basic
This exercise is an introduction to JavaScript through simple scripts to help you understand how JavaScript works and what you can do with the program. An example of beginner-level knowledge is how to write a JavaScript program to tell the current time and date in a particular format. This is an introductory exercise that is relatively easy to complete and execute.
Solution: The solution is to input your variable as the current day and assign the new date to it.
2. JS Variables
Variables are an essential part of JavaScript. They are used to identify and store data values that can be changed later. Variables are assigned to a value using the “=” sign when, before, and after declaring and accessing it. This exercise will teach you how to use variables, assign data values, declare, and access object properties. This exercise will help you get started with JavaScript coding.
Solution: The solution is to create a value to a variable “var” and assign a value to it. Next, you will learn to display values using variables. In this particular example, you will create a variable car name with the term “Volvo.”
3. JS Operators
In JavaScript, an operator can be used to compare values, perform arithmetic operations, or manipulate a specific value. In a JS operators exercise, you will learn to multiply and divide data values, alert results, alert remainders, and use assignment operators. This exercise involves learning to add up data values and use variables better.
Solution: This exercise will require you to multiply data values 10 and 5 and alert the answer. Next is to divide two values 10 and 2 and alert the result. Afterward, you have to get the remainder of another set of values, when 15 is divided by 9. Lastly, choose the assignment operator that will result in two values being added up.
4. JS Functions
JavaScript functions are a group of statements that perform a task or calculate a value, like a basic fetch function. Functions allow you to define, name, and execute a block of code multiple times. The most common example of a JS function is “Hello World!” This exercise will teach you how to name and execute JS functions efficiently. You will learn to create and name a function and make the function display.
Solution: Your solution is to learn to create, name, and execute a function using ‘myFunction’ to alert “Hello World!” Then, you will make the function return the “Hello” and lastly, make the function display “Hello.”
5. JS Events
JS events happen when a user or browser manipulates a page and the page loads. Every other thing that occurs when the user is on that page is also an event. For instance, when the user clicks a button, closes a window, or presses any key. This exercise teaches you how to create a button that triggers a response when someone clicks on it.
Solution: Create a button element that does something when someone tries to click on it, for example, “onclick.” Then, create a function that should be executed when the button is clicked and name it. Lastly, create a color that appears when the user clicks on the button.
6. JS Strings
Strings in JavaScript are primitive data types and are immutable, that is, they cannot be changed or moved. Strings must be closed in single or double quotation marks. While a string can’t be changed or moved, the variable name can be attached to a new string. This exercise is on writing a JavaScript function to split a string and convert it into an array of words. Once you master these, you’ll be able to learn about template strings.
Solution: Create a string to array function, use the length property to alert the length of the function, use escape characters to write the string, then split the string to get the output. For example, [‘Hello, World’] or [“Hello, World”].
7. JS Comparisons
JavaScript comparison operators compare two values and give back a boolean value, either true or false. They are used to make decisions and loops. This exercise involves you learning to compare in JavaScript. You will have to choose the correct comparison operator to alert a boolean value.
Solution: Choose the correct comparison operator to alert true when x is greater than y. That is, “x=10; y=5; alert(x>y).” Another is to choose the comparison operator to alert when x is not greater than “y x=5; y=10; alert(x!>y).”
8. JS Arrays
An array in JavaScript is an ordered list of values. It uses a single variable to store multiple elements or values at once. It is best used when you have a list of elements that you want to access easily with a single variable. This exercise includes writing a JavaScript function to determine if an “input” is an array or not.
Solution: First, you have to create your variable, input the function, and then set the program to return a boolean value. Afterward, you have to alert the number of items in an array using the correct array property.
9. JS Conditions
In JavaScript, a conditional statement controls behavior and determines if pieces of code can run. JS has three types, including “if”, “else”, and “else if” statements. This exercise requires fixing the if statement to alert “Hello World” if x is greater than y and to otherwise alert “Goodbye.”
Solution: What you have to do here is to include “if” at the beginning of the variables x and y to alert the function and “else” at the beginning of the alert.
10. JS Math
Math in JavaScript is an inbuilt object with properties and methods that allow you to perform mathematical tasks on numbers. JS math practice exercises will enable you to learn to run numbers using math methods. An example is to use the correct Math method to get the square root of 9.
Solution: The solution is to let x = math.sqrt(9).
How to Get Help with JavaScript
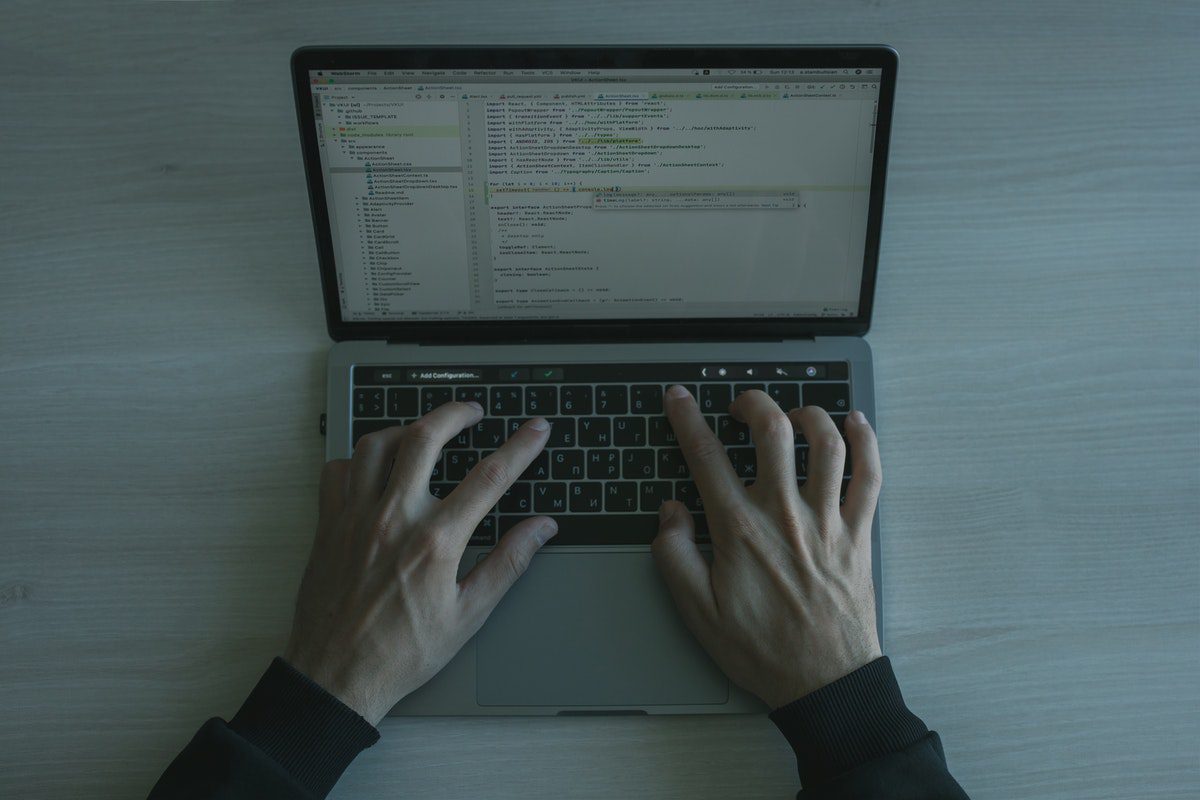
JavaScript is one of the most popular programming languages available today. There are many options for getting help learning JavaScript. You could go to a coding bootcamp, visit an online learning platform, practice JavaScript exercises on a website, practice with simple and complex projects, read a blog, or join a JavaScript forum.
JavaScript Exercises
JavaScript exercises are coding exercises that help you practice the language. Several websites offer multiple coding exercises for you to practice with for you to develop your skills. They typically include solutions to help you figure out the right way to work through it and check your progress.
JavaScript Projects
Working on real-life projects is an excellent way to learn any programming language. You can start by researching beginner JavaScript projects and doing them multiple times. This will help you learn practical skills, improve your speed, and build a solid foundation for your portfolio. A JavaScript calculator, an interactive landing page, or a tic-tac-toe game are great practice projects.
JavaScript Quizzes
Quizzes are short tests that include typical questions for testing your knowledge and skills in a field. There are numerous websites online that offer quizzes on JavaScript. You can take these tests to learn and practice your JS skills. You can track your progress to determine how much you’ve learned and still need to learn. Some websites that can help are W3Schools, Skillvalue, and Code Conquest.
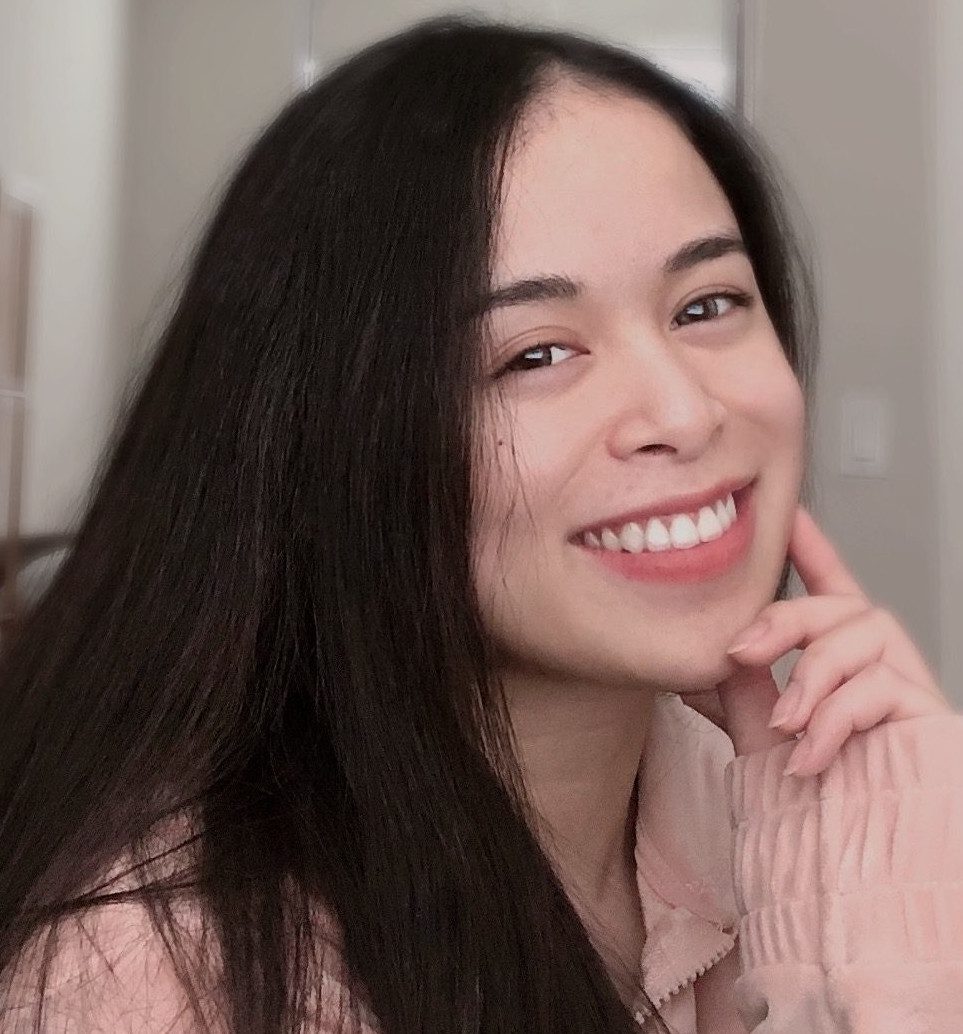
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
JavaScript Forums and Blogs
Joining a forum can help you stay updated on industry trends, learn more, improve your skills, and gain awareness of job openings. Some blogs have a focus on teaching beginners about JavaScript, while others are for coders with more advanced coding knowledge. They typically include step-by-step guides or tutorials to help you learn the language.
Where Can I Practice JavaScript?
There are many websites where you can practice using JavaScript. They typically include amazing content such as tutorials, step-by-step guides, and simple exercises to make learning easier and more efficient. You can find some of them below.
Websites to Practice JavaScript
- W3Schools. W3Schools is a coding website that allows new and experienced web developers to learn and practice their skills. The website offers tutorials on all web development programming languages, including JavaScript. You can learn all you need to know about JS and its syntax on this website.
- W3Resource. W3Resource is a website that offers a wide range of tutorials and quizzes on web development programming languages, including JavaScript. This is a helpful website to use as a beginner and work on your professional skills. You can practice JS exercises multiple times and use the quizzes to monitor your progress.
- Practity. Practity is a website that allows you to work on projects, programming exercises, and quizzes in several languages, including JavaScript. This website is a great version for those just starting, as the website includes lots of beginner-level JavaScript practice exercises.
- Exercism. This is one of the best online learning platforms for coding as it offers practice challenges for 50 different programming languages. Exercism is free to use and is designed to be beginner-friendly and has fun JavaScript exercises. You can learn any programming language on the platform regardless of your skill level.
- Code Wars. Code Wars is an online coding platform that allows you to learn and practice coding. This platform has challenge libraries that allow programmers who are a part of this community to participate in coding challenges and build their knowledge and skills.
What’s the Best Way to Learn JavaScript?
The best way to learn JavaScript is to use the resources at your disposal and practice regularly. JavaScript is one of the most popular programming languages today, and it is simple to learn on any of the many websites available on the Internet. You can begin by taking online courses or enrolling in a coding bootcamp. These options can give you the boost you need to become a professional, but you’ll need to practice regularly to make the most of your new skills.
JavaScript Exercises FAQ
To practice JavaScript, you should use practice exercises, build projects, improve existing projects, use practice challenges, take JavaScript quizzes, and join a JavaScript forum or community.
You can practice JavaScript daily by researching project ideas, building complex projects, working on exercise questions, taking quizzes, participating in coding challenges, and watching tutorial videos.
You can practice JavaScript online on websites such as freecodecamp, W3resources, W3schools, Code Wars, Practity, and Exercism.
Yes, you can. However, you will learn Node JS and other JavaScript topics that can be executed independently in the JS compiler, like declarations and closures.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.