Adding interactive features to a website is where JavaScript shines. Whereas HTML and CSS are used to set the structure and styles of a web page, respectively, you can use JavaScript to make your site more dynamic.
In JavaScript, events are used to make a site interactive. In this guide, we’re going to talk about JavaScript events. We’ll explore where events may be used, how to define an event, and how to call an event. Let’s get started!
What Is a JavaScript Event?
Events can either be triggered by the browser or the user and are capable of modifying the state of the web page. When you click a button on a web page, a JavaScript event is created. When you click on a dropdown menu that expands, a JavaScript event is used.
JavaScript events have a wide range of uses, which include:
- Checking whether a form has been filled out
- Navigating a user to another page when they have filled out a form
- Making dropdown menus interactive
- Allowing a user to zoom in and out of images
To work with events in JavaScript, you need to know about two concepts: event handlers and event listeners.
An event handler is a function that is run when an event is triggered on a web page. Event listeners connect an event function to an HTML element, so when that function is executed, the web element is changed.
Event handlers can be defined in one of three ways: using either an inline event handler, an event property, or an event listener.
Inline Event Handlers
The easiest way to get started with events in JavaScript is to use an inline event handler. This means that you’ll define your event in your HTML file.
For this example, let’s suppose that we are designing a website for a bakery called JJ Smith’s Bakery. We want a user to be prompted with the text “Response received.” when they submit a form.
Consider the following code:
<!DOCTYPE html> <html> <head> <title>JJ Smith's Bakery</title> </head> <script src="main.js"></script> <body> <p id="submitted"></p> <button onclick="submitForm()">Submit form</button> </body> </html>
This code renders a web page which looks like this:
So far, not much is going on. Our web page contains a button that should do something when we click our button. When we click our button, nothing happens. This is because we have yet to write our event handler.
In the file main.js, paste in the following code:
const submitForm = () => { const text = document.getElementById("submitted"); text.textContent = "Form submitted." }
This code will find the element with the ID “submitted”, and will modify its content to be “Form submitted.” This code will only run when our submitForm()
function is called. This is scheduled to happen when the user clicks on our button.
When we click our button, the following happens:
As you can see, our prompt appears. We’ve used an inline event handler to make our web page interactive. But inline event handlers are not the only way to use events in JavaScript. Let’s discuss how to use the event handler property in our code.
Events with Handler Properties
In an inline handler, you need to specify which function you want to execute in your HTML file. In our HTML code from earlier, this line is where we defined the function we wanted to call when our button was pressed:
<button onclick="submitForm()">Submit form</button>
Using handler properties, we can remove the “onclick” event from our code. This allows us to move all of the JavaScript that makes our web page interactive into its own file. For this example, we’re going to assign our button the ID “button”, so that we can identify our button in our JavaScript code. Let’s remove the onclick event and assign our button an ID:
<button id="button">Submit form</button>
In order to get our event to fire when our button is pressed, we are going to write an event with a handler property inside our “main.js” file. Here’s the code that we will use:
const submitForm = () => { const text = document.getElementById("submitted"); text.textContent = "Form submitted." } const button = document.getElementById("button") button.onclick = submitForm;
When you run this code and click on the button, the following is returned:
Our button functions in the same way as earlier, but our code is written in a different way. Rather than writing our event handlers inline, we moved them to our main.js file. This helps us write more maintainable code because we don’t need to read through all our HTML code to find out where our inline events are triggered.
Event Listener
Event listeners can be used to declare an event in JavaScript. Listeners are always looking for when the state of an element is changed, and if that element is changed, the code in the listener will be executed.
For instance, when you click on a button that’s attached to an event listener, the listener will “hear” that a mouse event has been triggered. Then it will execute the code you have associated with that particular event listener.
Our HTML code for this example is the same as above, but we’re going to make a minor change to our “main.js” file:
const submitForm = () => { const text = document.getElementById("submitted"); text.textContent = "Form submitted." } const button = document.getElementById("button") button.addEventListener("click", submitForm);
In this code, we are using addEventListener
to create an event listener. This is instead of binding our submitForm()
JavaScript function to an onclick event as we did in our last two examples.
We specify “click” to tell our code to listen for when our button is clicked with the mouse pointer. There are other event types aside from the click event—such as keyboard events—but we’ll focus on the mouse click event in this tutorial.
Let’s run our code again and click our button.
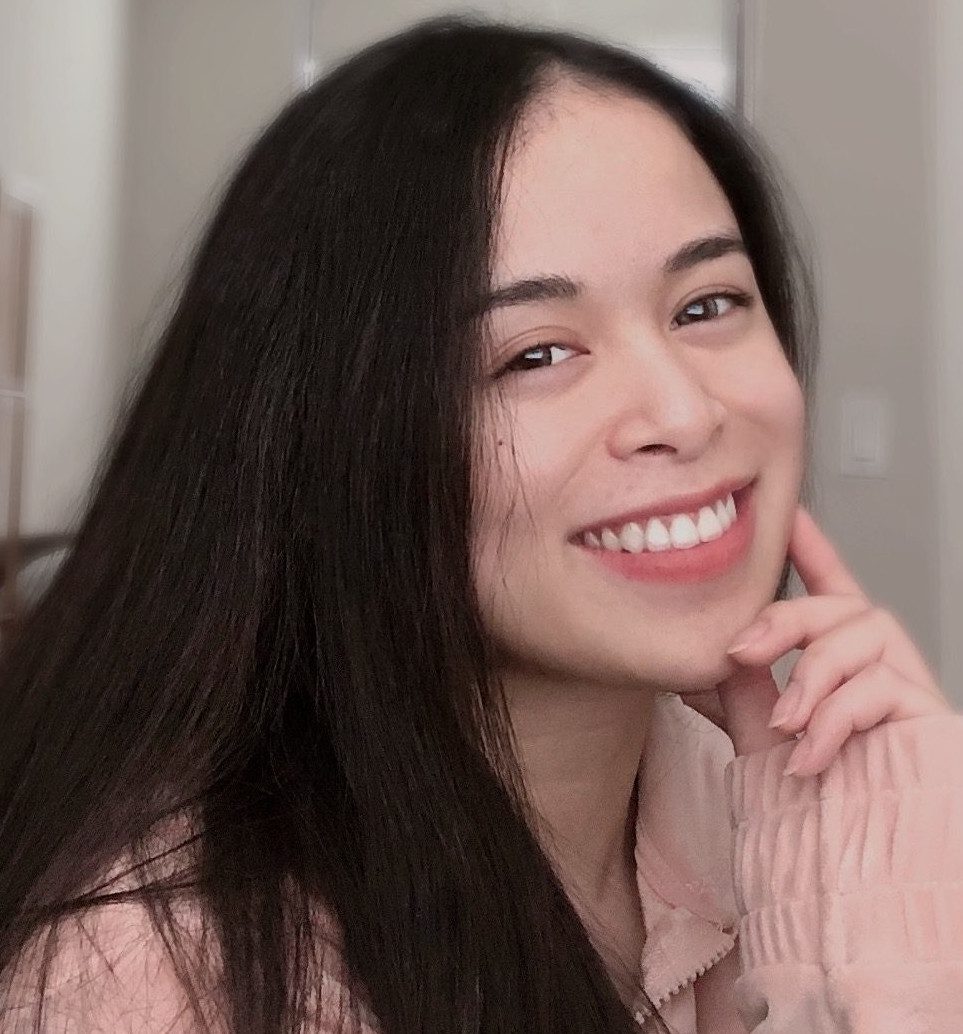
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The output is the same as we’ve seen in our last two examples, but this time we’re using an HTML event listener. Event listeners are the newest way to declare events in JavaScript, and they are perhaps the most common method used.
Conclusion
JavaScript events allow you to make your web page interactive. An event could be triggered when you press a button, submit a form, or hover over some text. There are three ways to declare an event in JavaScript: inline, using a property, or using a listener.
Now you’re ready to start creating events in JavaScript like a professional web developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.