There are four ways to compare equality in JavaScript. This article talks about the unique operators used to determine equality, what type coercion is, and some of the idiosyncrasies encountered when evaluating for a truthy or falsy value in the JavaScript language.
Strict Equality Comparison
The strict equality operator is represented by a triple equals sign (===). The purpose of this operator is to compare not only the value, but also its type.
const strictComparison = (a, b) => { console.log(typeof a); console.log(typeof b); return a === b; } strictComparison(8, '8'); //false
The strictComparison
function in the example above takes in two values and returns whether or not the two values are strictly equal to one another.
At first glance, the values look the same because they are both of value 8
. However, the strict equality operator also looks at the type. If we were to look at a
in this instance, and look at
typeof a
,
it will return ‘number’
. If we were to do the same to b
, with typeof b
,
it would return ‘string’
.
Because the types are not the same, it will return false
.
Unusual Comparisons With ===
There are some unusual comparisons that can be made with the strict equal operator in JavaScript. Here are some that are commonly asked about in interview situations:
Operand1 | typeof Operand1 | Operand2 | typeof Operand2 | Return Value |
null | object | null | object | true |
undefined | undefined | undefined | undefined | true |
null | object | undefined | undefined | false |
NaN | number | NaN | number | false |
false | boolean | “false” | string | false |
false | boolean | false | boolean | true |
“” | string | “” | string | true |
“” | string | undefined | undefined | false |
One other thing to note is this will not do a comparison with data structures. You need to use a more sophisticated statement to compare arrays or objects.
Most of the entries here evaluate the way they do because the types match or don’t match. The one exception is NaN
– this evaluates to false
due to the fact that NaN
can theoretically be anything – so there is no indication whether or not it could be equal, therefore evaluating to false.
Strict Inequality Comparison
The strict inequality operator is represented by an exclamation point and two equal signs (!==). It will evaluate whether or not the two values are NOT equal in value and type.
const strictInequalityComparison = (a, b) => { console.log(typeof a); console.log(typeof b); return a !== b; } strictInequalityComparison("8", 8); //true
The strictInequalityComparison
function in the example above takes in two values and returns whether or not the two values are strictly not equal to one another.
At first glance, the values look equal (and not unequal) because both have the same value. However, the strict inequality operator, like the strict equality operator, also looks at the type.
If we were to look at a
in this instance, and look at typeof a
, it will return ‘string’
. If we were to do the same to b
, with typeof b
, it would return ‘number’
. Because the types are not the same, it will return
true
.
Unusual Comparisons With !==
There are some unusual comparisons that can be made with the strict inequality operator in JavaScript. Here are some commonly asked about in interview situations:
Operand1 | typeof Operand1 | Operand2 | typeof Operand2 | Return Value |
null | object | null | object | false |
undefined | undefined | undefined | undefined | false |
null | object | undefined | undefined | true |
NaN | number | NaN | number | true |
false | boolean | “false” | string | true |
false | boolean | false | boolean | false |
“” | string | “” | string | false |
“” | string | undefined | undefined | true |
Just like with the strict equality operator, comparisons cannot be made between objects or arrays.
One More Thing…
In most situations in your JavaScript development career, these two operators, the === and the !==, will be the ones that you will be writing your conditional logic with.
Loose Equality Comparison
The loose equality operator is represented by a double equals sign (==). The purpose of this operator is to coerce both values to a common type before evaluating whether or not it is equal. This is called type coercion or type conversion.
const looseComparison = (a, b) => { console.log(typeof a); console.log(typeof b); return a == b; } strictComparison(8, '8'); //true
The looseComparison
function in the example above takes in two values and returns whether or not the two values are loosely equal to one another.
At first glance, the values don’t look the same because one is a number and one is a string. However, the loose equality operator doesn’t look at the type. The statement will attempt to coerce the types to be the same before comparing the value — so the example will return true because the second operand is converted to a number and then compared.
Unusual Comparisons With ==
There are some unusual comparisons that can be made with the strict equal operator in JavaScript. Here are some commonly asked about in interview situations:
Operand1 | typeof Operand1 | Operand2 | typeof Operand2 | Return Value |
null | object | null | object | true |
undefined | undefined | undefined | undefined | true |
null | object | undefined | undefined | true |
NaN | number | NaN | number | false |
false | boolean | “false” | string | false |
false | boolean | false | boolean | true |
“” | string | “” | string | true |
“” | string | undefined | undefined | false |
Most of the entries here evaluate the same as they did in the first section on strict equality. The notable exception is that null and undefined loosely compared evaluates to true.
Loose Inequality Comparison
The loose inequality operator is represented by an exclamation point and one equal sign (!=). It will evaluate whether or not the two values are NOT equal only in value. It does this by attempting to convert both arguments to be of the same type.
const looseInequalityComparison = (a, b) => { console.log(typeof a); console.log(typeof b); return a != b; } looseInequalityComparison("8", 8); //false
The looseInequalityComparison
function in the example above takes in two values and returns whether or not the two values are loosely not equal to one another.
At first glance, the values look equal because they both have the same value. However, the loose inequality operator, like the loose equality operator, coerces the type to be the same on both operands before comparison. Because the values are the same after coercion, it will return false
.
Unusual Comparisons With !=
There are some unusual comparisons that can be made with the loose inequality operator in JavaScript. Here are some commonly asked about in interview situations:
Operand1 | typeof Operand1 | Operand2 | typeof Operand2 | Return Value |
null | object | null | object | false |
undefined | undefined | undefined | undefined | false |
null | object | undefined | undefined | false |
NaN | number | NaN | number | true |
false | boolean | “false” | string | true |
false | boolean | false | boolean | false |
“” | string | “” | string | false |
“” | string | undefined | undefined | true |
Just like with the strict equality operator, comparisons cannot be made between objects or arrays.
Most of the entries here evaluate the same as they did in the second section on strict inequality. The notable exception is that null and undefined loosely compared evaluates to false.
Conclusion
This article has looked at the four ways to compare equality in JavaScript. The main thing to remember is triple equals (===) and double equals (==) are not the same operator. One evaluates strictly on type and value and the other only evaluates the value after trying to coerce both to be of the same type.
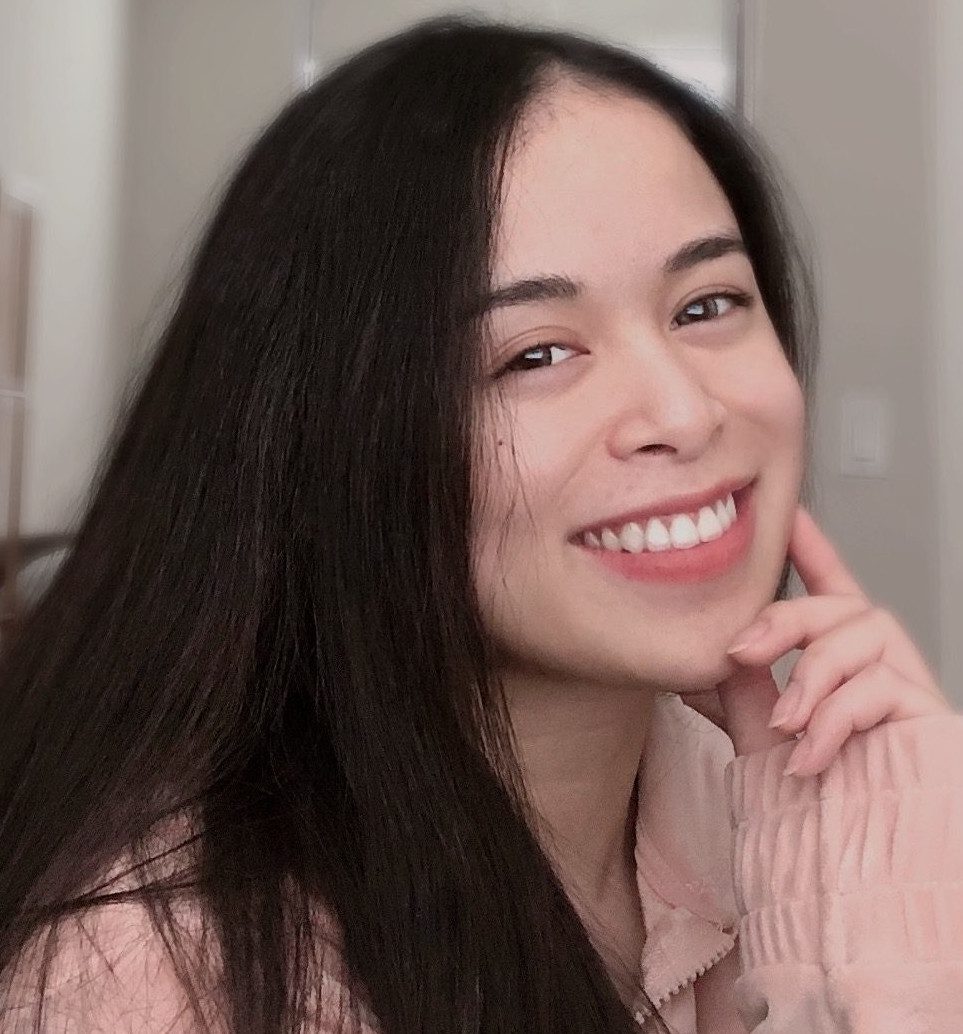
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.