Countdown timers are used all over the Internet by people who want to count down to a major event.
You could use a countdown timer to count down to the launch of your new website. A countdown timer could mark when you are going to make a product announcement. There are so many possibilities for you to explore.
In this guide, we’ll show you how to create a countdown timer in JavaScript using three technologies: HTML, CSS and JavaScript. Let’s begin!
Setting Up Our Webpage
The first step is to set up our basic web page. In this guide, we’re not going to worry about using any external libraries. We’ll keep it simple and use plain old HTML, CSS and JavaScript.
Let’s start by creating our HTML file:
<!DOCTYPE html> <html> <script> // We'll store our JavaScript code here </script> <style> // We'll write our CSS styles here </style> <body> <h1>It's almost time…</h1> <div id="timer"></div> </body> </html>
Our code doesn’t return anything special right now, see below:
It appears this way because we haven’t added any styles to our code yet or created our JavaScript timer. Let’s move on to creating the JavaScript that will power our timer.
Creating our Timer
JavaScript has a handy object called Date which we can use to work with dates and times. We’re going to use this object for two purposes: to get a timestamp of the time to which we want to count down, and to get the current date.
In this tutorial, we’re going to write all of our scripts in a <script></script> tag. We’re not going to be using any external scripts.
Subtracting the date to which we are counting down from our current date will give us the difference between these two dates:
var timeLeft = +new Date("2021-05-04") - +new Date();
This code will calculate how many days are left until the next Star Wars Day, which is May 4th, 2021.
While we now know the difference between these two days, there’s a few problems. First, we don’t have a timer that counts down yet. Second, our date isn’t stored in a format easily readable by humans. It’s stored in milliseconds, which isn’t exactly convenient for us. We need to convert the time into days, hours and minutes.
To calculate the time remaining in a more readable format, we are going to use the following code:
var daysLeft = Math.floor(timeLeft / (1000 * 60 * 60 * 24)); var hoursLeft = Math.floor((timeLeft / (1000 * 60 * 60)) % 24); var minutesLeft = Math.floor((timeLeft / 1000) / 60 % 60);
We can format the values into a string using this code:
const timeLeftText = `${daysLeft} days ${hoursLeft} hours ${minutesLeft} minutes.`;
In this code, we use string interpolation to substitute in the values we calculated earlier. This will give us a timestamp like: 314 days 13 hours 52 minutes.
Now that we’ve generated our timestamp, we can place it on our webpage:
document.getElementById("timer").innerHTML = timeLeftText;
We can move all of our code into a function:
function countdown() { var daysLeft = Math.floor(timeLeft / (1000 * 60 * 60 * 24)); var hoursLeft = Math.floor((timeLeft / (1000 * 60 * 60)) % 24); var minutesLeft = Math.floor((timeLeft / 1000) / 60 % 60); const timeLeftText = `${daysLeft} days ${hoursLeft} hours ${minutesLeft} minutes.`; document.getElementById("timer").innerHTML = timeLeftText; } countdown();
When we load our page, the following is returned:
Now we have our timer. We’re done for the day, right? Nope; not so fast. If you wait a minute, you’ll see our timer doesn’t actually count down. That’s because we need to tell our browser to update every minute. We do so using this code:
setInterval(countdown, 60000);
This code should be added after our countdown() function is declared. This will refresh our page every 60,000 milliseconds. This is equivalent to every 60 seconds.
Now when we update our code you’ll see that it changes every minute!
Adding Some Styles
Our countdown timer may be functional, but it’s not exactly aesthetically pleasing. Let’s create a few styles to make our page more attractive:
<style> body { padding-top: 20%; height: 100vh; background: linear-gradient(to bottom right, #CAA89C, #434EFC); } h1, p { text-align: center; color: white; } </style>
Our webpage now looks like this:
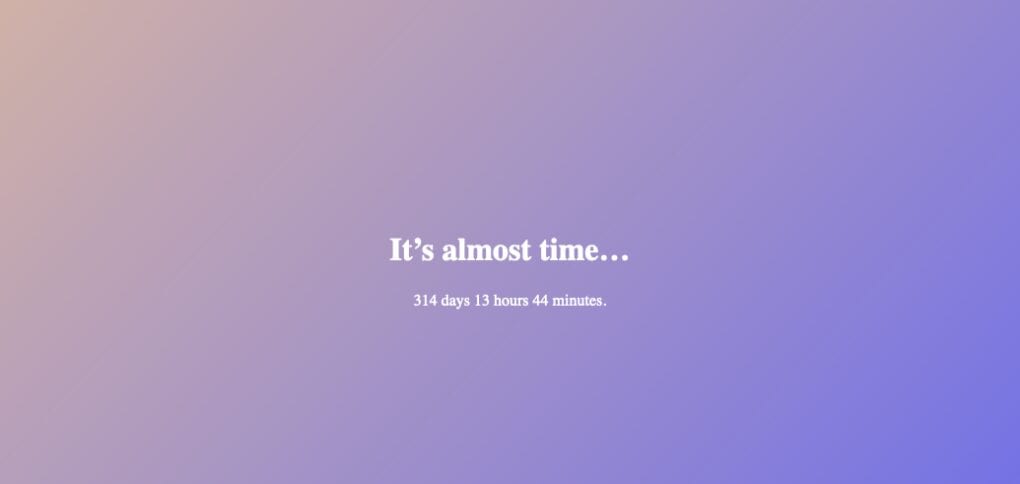
As you can see, our page now has a gradient background, our text is aligned to the center and our text appears in white. It looks great!
Conclusion
Countdown timers are an important feature of many sites. You’ll see countdowns on both personal and professional web pages. A person’s blog may feature a countdown to their wedding. A company website may feature a countdown to a product launch.
Now you’re ready to create your own JavaScript countdown timer like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
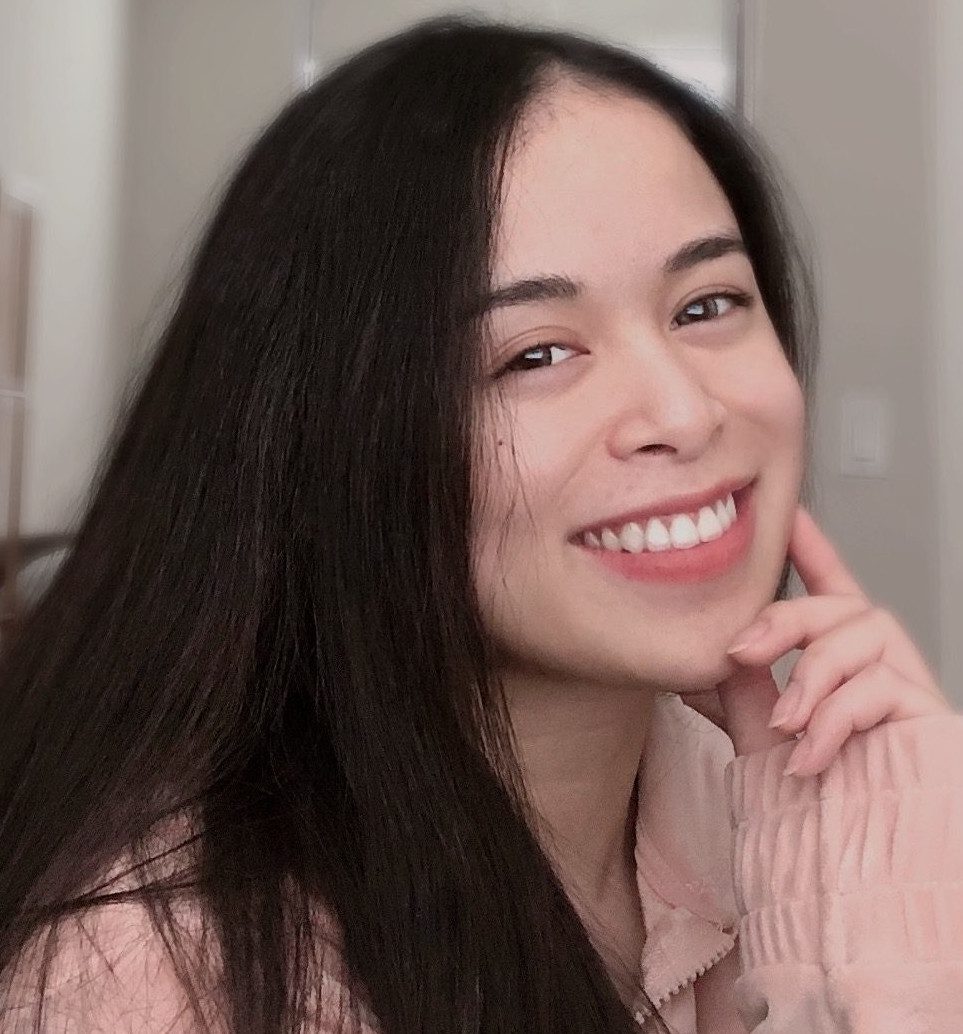
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot