Cookies are both a delicious snack and a crucial part of modern websites.
Cookies allow you to store data about a user in the web browser. Cookies often store information like authentication sessions so the browser remembers you have logged in to a web page.
In this guide, we’re going to talk about why cookies exist, what types of cookies there are and how to use cookies in JavaScript. Let’s begin!
What are Browser Cookies?
Cookies are strings of text which store information about a user in their browser. The idea was pioneered by Netscape, which developed one of the most popular browsers in the world in the 1990s. Netscape developed cookies to track whether a user had already visited their site. If they had, it meant the user probably knew something about Netscape.
Cookies must contain at least a name and a value field. The name field is used to identify the contents of the value field. A cookie can store additional attributes such as a file path and when it expires, depending on how you are using cookies in your applications.
Here’s an example of a cookie:
Name: Authentication Value: AUTHENTICATION_KEY Domain: careerkarma.com
Cookies are stored in key:value pairs. When you view this cookie, you’d see the following:
Authentication=AUTHENTICATION_KEY
This cookie would store the value AUTHENTICATION_KEY in your browser. Every time you access the domain careerkarma.com, that cookie would be made available to the site.
There’s no limit to how many cookies you can set for a site, but what you’ll find is that most applications only need to set a few, if any. Often, cookies are used to keep track of a user’s session.
Retrieve Browser Cookies
JavaScript has an object called document.cookie which stores information about all the cookies available to a site. To set, update and delete cookies, we need to manipulate this object. To retrieve cookies, we can just call this object:
console.log(document.cookie);
This code will return a list of all the cookies set in the browser. There’s no way to retrieve an individual cookie from this list without writing a custom function to do so.
How to Set a Browser Cookie
How do I set a browser cookie? Well, there’s one thing you should know upfront: it’s arguably easier than baking your own cookies.
Let’s say we want to store a user’s email in a cookie. We could do so using this JavaScript code:
document.cookie = "email=user@email.com"; console.log(document.cookie);
This code creates a cookie with the name “email” and the value “user@email.com.” Our console.log()
statement returns the following:
email=user@email.com;
You can also specify a path on which a cookie is available:
document.cookie = "email=user@email.com; path=/dashboard";
This code would make the “email” cookie available on all paths that begin with “/dashboard”.
Cookie Expiry Dates
By default, cookies are deleted when the browser is closed. When you’re setting a cookie, you can optionally add an expiry date which will override this setting:
document.cookie = "email=user@email.com; expires=Wed, 24 Jun 2019 12:00:00 UTC";
This will create a cookie that expires on June 24, 2020 at the start of the day. Your expiry date should be specified in UTC time.
One common approach to setting an expiration date is to use the JavaScript Date object, which returns a UTC timestamp. A cookie set to expire on June 30, 2020 would use this code:
const expiryDate = new Date(2020, 6, 30); document.cookie = "email=user@email.com; expires=" + expiryDate + ";";
The document.cookie object supports setting a maximum age of a cookie, or Max-Age, which states how long a cookie should be available before expiring. While it is not supported by every browser, it makes it easy for you to create cookies that expire depending on when the user initiated the creation of the cookie:
const expiryDate= 24 * 60 * 60 * 2; document.cookie = "email=user@email.com; max-age=" + expiryDate + ";";
This will create a cookie that expires after two days.
Updating and Deleting Cookies
There are no functions used to update or delete cookies. Instead, you make a change to the “document.cookie” object.
You can update a cookie in the same way as you created it:
document.cookie = "email=user@email.app;"
This changes the value of “email” from “user@email.com” to “user@email.app”.
Deleting a cookie uses a similar approach. All you have to do is use the same syntax as used to create or update a cookie, but remove the value assigned to the cookie:
document.cookie = "email=; expires=Wed, 24 Jun 2019 12:00:00 UTC; path/;"
You should specify a path to make sure that you delete the right cookie.
Types of Cookies
Raspberry. Chocolate Chip. Fudge. No, not those types of cookies! In the browser, there are three main types of cookies: session, third-party and persistent.
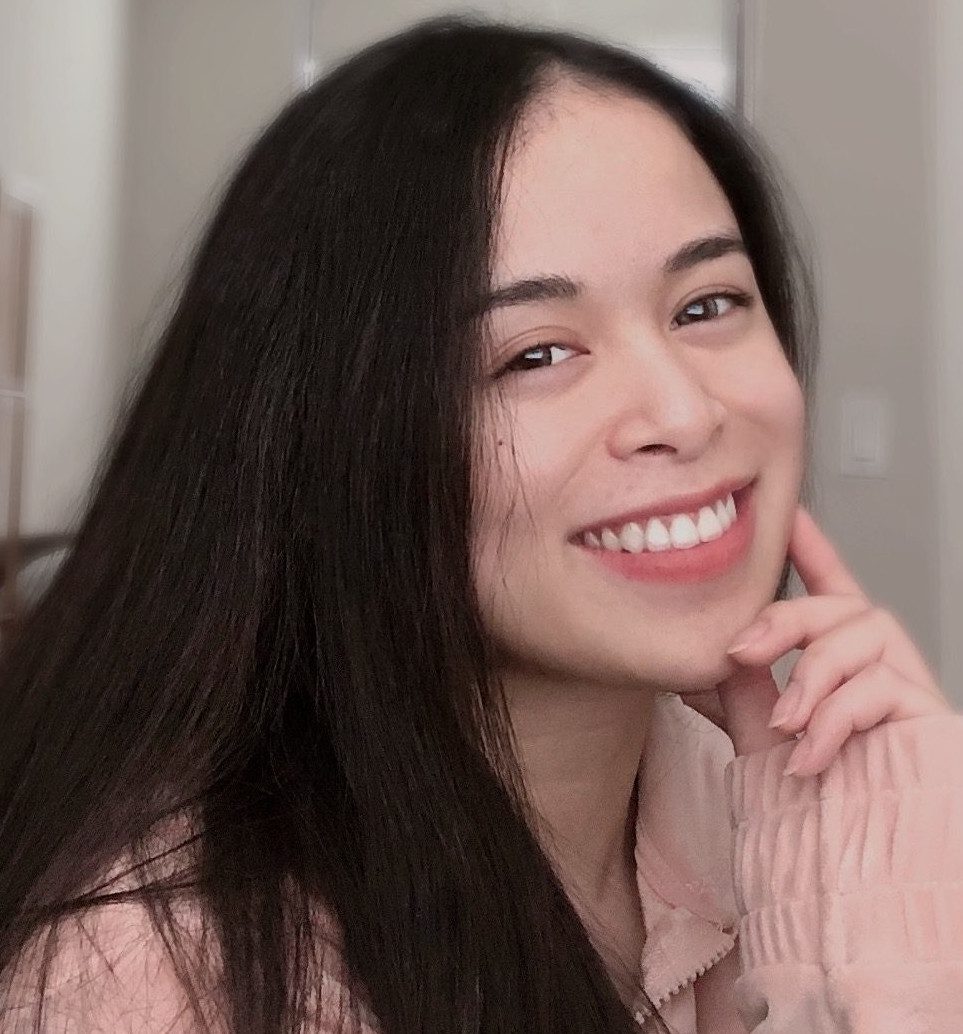
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Session cookies are cookies that exist until the browser is closed. These are cookies that are set without an expiry date, like the one in our first example. It’s worth noting that some developers use the term “session cookie” to refer to any cookie that authenticates a user, but session cookies are not the only cookies you can use for authentication.
Persistent cookies are cookies that exist even once the browser has closed. These are cookies with a set expiration date.
Third-party cookies are cookies that have been created by other websites. An example of this is Google Analytics cookies. If you install Google Analytics on your site, the extension will be able to set cookies to keep track of users.
Conclusion
Cookies are a handy way of storing user information in the browser. They are often used to track whether a user has visited a site before and to store authentication cookies.
There are three main types of cookies: session, persistent and third-party. For your own applications, you’ll mainly be focused on using session and persistent cookies.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.