Writing code, while entertaining, can also become quite tedious.
There are days when you start writing code, realize something is not running, and get very frustrated. Even more annoying is when your code runs, but you can’t figure out why it’s not working correctly; when there’s something in your code you need to fix.
That’s where the JavaScript console can be useful. The console allows you to keep track of what is going on in your code. This makes it easy to figure out what’s wrong in your code.
In this guide, we’re going to discuss how to use the JavaScript console. We’ll also provide an example of an application using the console so you can quickly master the JavaScript console.
The JavaScript Console
Developer, meet the console. You’ll be spending a lot of time in the console during your time as a developer, so it’s something with which you’ll need to familiarize yourself.
The console is a place where you can display messages as your application is running and where you can manipulate the contents of a web page.
You can tell your code to log messages to the console and they will appear when you have instructed them to. You can also use the console to modify variables, values and inspect the values stored in a variable.
To display your console, you’ve got to open up developer tools. In Chrome, you can do this using Ctrl + Shift + I on Windows or Cmd + Alt + I on Mac. In Firefox, you can open developer tools using Command + Option + K.
When you do, a window like this will open:
Every modern browser has its own developer tools suite and there are a number of common features across all browsers. For this tutorial, we’re just going to focus on the console.
Getting Started with the Console
We’re going to start by displaying a message to the console. We can do this without even having a web page open. All you have to do is open your browser console and write the JavaScript statements you want to execute.
There are three main methods you’ll use in the console:
- console.log(): Displays a message to the console.
- console.warn(): Displays a warning in the console.
- console.error(): Displays an error in the console.
Before we continue, you shouldn’t paste anything into your console without first knowing what the code does. Many modern sites will warn you against this as there are scams which use the console to access sensitive user information.
With that said, let’s begin! To display a message to the console, we can use this code:
console.log("Career Karma is logging stuff to the console!");
As soon as you execute this command, the following will be returned:
You can also display errors and warnings to the console. The difference between errors and warnings and a traditional log()
statement is that errors and warnings appear in orange and red, respectively:
console.warn("Hey, something is going on!"); console.error("Hey, your code has stopped working!");
Our code returns:
Using Console in an Application
We’re going to build an app modelled after cookie clicker to showcase the console object in action. This app will display a counter which shows us how many times an image of a cup of tea has been clicked. We’ll log every click to the console so we can keep track of when they occur.
Developing the Front-End
Our first step is to develop the front-end of our application. Create a file called “index.html” and paste in the following code:
<!DOCTYPE html> <html> <head> <title>Tea Clicker</title> <link rel="stylesheet" href="./styles.css"> </head> <body> <div class="container"> <h2>Tea Clicker</h2> <p>Click the tea cup as many times as you can!</p> <img src="http://images.all-free-download.com/images/graphiclarge/tea_cup_with_teabag_311679.jpg" height="100" width="100" onclick="createClick()" /> <p>You have clicked the tea cup <span>0</span> times.</p> </div> <script src="./scripts.js"></script> </body> </html>
This code displays four items on our web page: a title, a description of the game, an image of a cup of tea and a message informing the user how many times they have clicked the cup of tea.
Let’s also add some styles to our code to make it look attractive. Create a file called “styles.css” and paste in this code:
.container { background-color: lightblue; margin: auto; width: 50%; padding: 40px; text-align: center; } span { color: yellow; } img { border-radius: 50px; }
These styles create a box for our tea clicker game, set the color of the click counter to yellow and create rounded corners for our image. Here’s what our webpage looks like now:
When you click the tea cup, nothing happens. That’s because we haven’t added our JavaScript code yet. Let’s write the JavaScript code for our application.
Adding in Functionality Using JavaScript
When the user clicks on the cup of tea, the counter should increment by one. Before we can increment our counter, we need to select the DOM elements we’re going to be working with: the image and the counter. DOM, or Document Object Model, elements are tags on our webpage.
var clickCounter = document.querySelector("span"); var teaCup = document.querySelector("img");
We’re also going to set up a variable which tracks how many times the cup of tea has been clicked:
var clicks = 0;
Our next step is to create a function which logs when our button has been clicked and increments our counter by one. We can do this using the following code:
function createClick() { console.log("The button has been clicked") clicks += 1; clickCounter.innerText = clicks; }
The first line will print the console message “The button has been clicked” to the console. Next, we add 1 to our “clicks” counter. We then change the contents of the text in our <span> tag to reflect the updated click count using the innerText method.
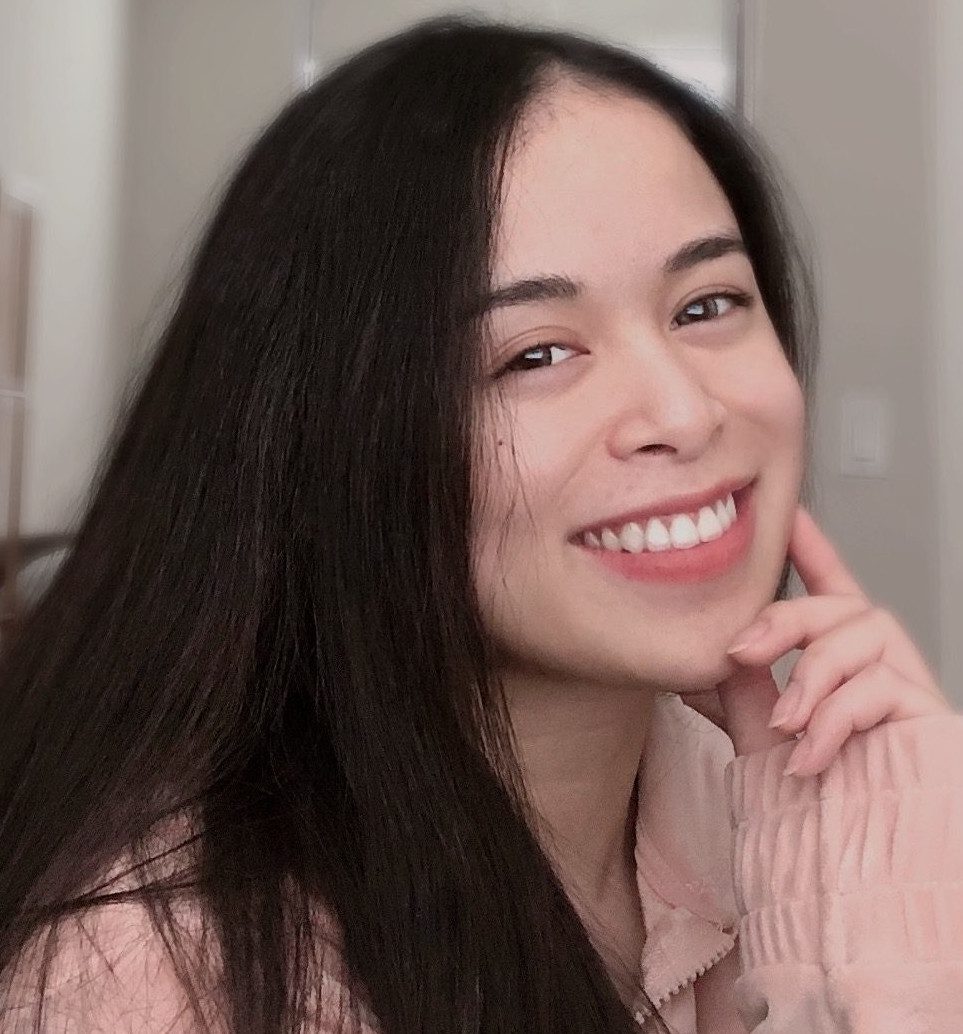
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Let’s view our web page and click on the image of the cup of tea:
As seen, when we click the cup of tea our counter increments by one. In addition, the message we specified in our code is printed to the console. This helps us understand when the user clicks on the cup of tea.
While this is a very basic implementation of the console.log()
statement, in a larger application you may see that logging is used widely. For the most part, logs are only kept in development versions of applications. This is because users don’t need to see what’s going on behind the scenes. With that said, logging is still an incredibly valuable tool for developers.
Conclusion
The console is a tool you can use to learn how your code works. You can use it to display debugging messages as your code runs or to keep track of warnings and errors.
You should only use logging statements if you are developing your application, or if you want to create custom error messages for your code you can use for debugging. You shouldn’t keep too many logs in a web application that’s available online.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
Testing would have revealed these errors:
1. There is no event listener in the HTML. In the tag, there needs to be onclick=”createClick()” attribute/value pair. Solution:
2. The and tags are invalid in a CSS file. This entirely disables the .container rule, since it directly follows this error. Solution: remove both tags.
Hello there,
Thank you for your comment. It appears as if we did miss the onclick event in the
tag. I have added one to the article. I also removed the