The JavaScript includes() method searches an array for an item. This method returns True if the element in the array exists. The filter() method lets you find an item in a list. Unlike includes(), the filter() method returns the item for which you have been searching.
You may want to find out whether that array contains a particular value. For example, you may have a list of product orders and want to check if anyone has ordered a bedside cabinet from your store.
JavaScript Array Contains
There are two ways to determine if a JavaScript array contains an item:
- includes().
- filter().
The includes() method checks whether an item exists in array and returns true or false. filter() finds an item in an array and returns that item.
In this tutorial, we are going to discuss using the array includes() method in JavaScript to determine whether an array contains a particular element. We will also discuss how to use filter() to check if an array of objects contains a value.
Array Contains JavaScript: includes()
The JavaScript includes() method determines whether an array contains a particular value. The includes() method returns true if the specified item is found and false if the specified item
array_name.includes(element, start_position);
The includes() method accepts two arguments:
- element: The value for which we are searching. (required)
- start_position: The index position in the array at which the search should begin. (optional)
The includes() method can return one of two values: true and false. These values are called JavaScript Booleans.
Array.includes() works in the same way as String.includes(). But, Array.includes() searches through an array of objects.
includes() JavaScript Example
Say that we are running a coffee shop. We want to check whether anyone has ordered any espressos today. We could use the following code to check today’s order list to see if an order for an espresso has been placed:
var todays_orders = ['Latte', 'Americano', 'Latte', 'Latte', 'Mocha', 'Cortado']; console.log(todays_orders.includes('Espresso'));
Our code returns: false.
The array todays_orders does not include the value Espresso. So, when our includes() function is executed, it returns the value false. If we were to search through an array where the element did exist, includes() would return true.
Now, let’s say that we want to see whether anyone has ordered a latte in the last three orders that have been placed. We could use the includes() method with a start_position argument to do so.
Here’s the code we would use:
var todays_orders = ['Latte', 'Americano', 'Latte', 'Latte', 'Mocha', 'Cortado']; console.log(todays_orders.includes('Latte', 3));
Our program returns: true. Because a latte was ordered after the index value 3 in our array, our includes() function has returned a true value.
Array Contains JavaScript: filter()
In the above example, we used includes() to check if an array of strings included a particular value. But what if we want to check if an array of objects contains a specific value? We can use the filter() function to perform that action.
The filter() function iterates through an array of objects and search for a particular value. filter() takes in one argument: a function that is used to search for a value. Here’s the syntax of the JavaScript filter() function:
var filterArray = array_name.filter(function(item) { return item; });
filter() JavaScript Example
We have an array of coffee shop order objects that contain both the name of the coffee ordered and the customer’s name.
We want to check whether anyone by the name of John Appleseed ordered a coffee today. John is a loyalty customer and was supposed to get a discount on his next coffee.
The following code checks if John Appleseed has placed an order:
var orders = [ { drink: 'Cappuccino', name: 'John Appleseed' }, { drink: 'Espresso', name: 'Mya Stuart' }, { drink: 'Cappuccino', name: 'Emma Berry' } ]; var check_orders = orders.filter(order => (order.name === "John Appleseed")); console.log(check_orders);
Our program returns:
[{ drink: 'Cappuccino', name: 'John Appleseed' }]
We first declare an array called orders. This stores a list of the names of people who have placed an order and the drink they ordered. Then, we declare a JavaScript variable called check_orders. This variable uses the filter() function to check if anyone by the name of John Appleseed ordered a coffee today.
We print out the value of the check_orders variable to the JavaScript console. Because John Appleseed did place an order today, our function returns the record of his order. If he did not place an order, our program would return nothing.
If you’re interested in learning more about the filter() method, check out our tutorial on the JavaScript filter() and reduce() methods.
Conclusion
The includes() method checks if a value is in an array. This method returns true or false depending on the outcome. The filter() method determines if an array of objects contains a particular value. This method returns the object that meets a certain criterion if it exists in the array.
Do you want to learn more about JavaScript? Check out our How to Learn JavaScript guide. You’ll find expert advice and a list of top online courses, books, and resources you can use to help you learn.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
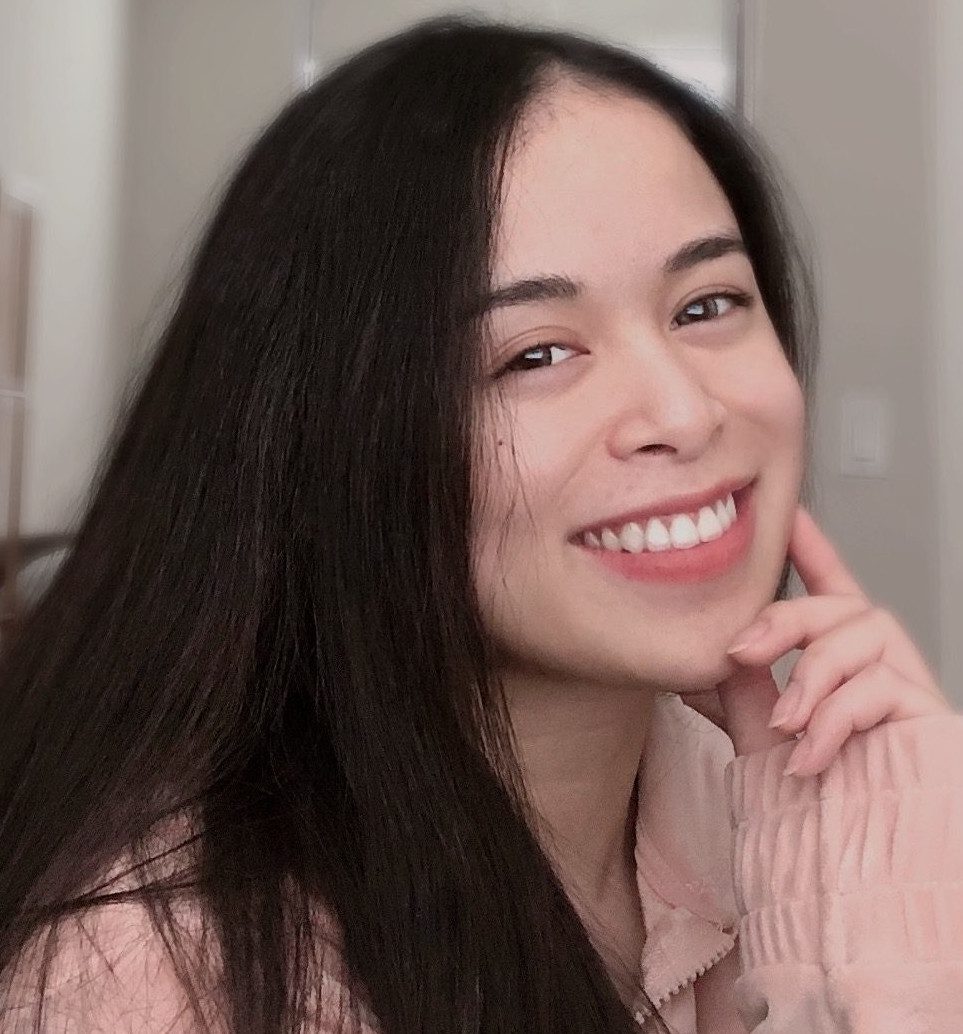
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot