Let’s create ourselves a list of fruits. We’ll call our list “fruit”, and add the following values: Banana, melon, grapes. Oh, how I love fruit! While this may sound like a tutorial on shopping lists, we’ve actually just created ourselves an array.
In this guide, we’re going to walk through what arrays are, how they work, and why you should use them in your code. We’ll discuss a few examples of arrays in action so you can become a JavaScript array ninja!
What is an Array?
An array is an object that stores data. Arrays contain an ordered collection of items, and can store zero or more items. Arrays are useful in programming because they allow you to store similar values in one variable.
You don’t have to write ten single variables to list your favorite fruits, for example. With an array, you can add all of your favorite fruits into one variable.
Consider the following example:
let fruits = [ "Banana", "Grapes", "Melon" ];
Here we’ve created an array called “fruits”. It stores three different values, each of which reflects a fruit we want to buy at a grocery store. To declare our array, we’ve surrounded our list of items in square brackets.
Declaring an Array
There are two ways to declare an array. The way that you’ll see most people do it is by surrounding a list of items with square brackets, like we did earlier:
let berries = [ "Strawberry", "Blueberry", "Gooseberry" ];
We’ve declared an array with three values in this example. We can also use the array constructor, which uses the new
keyword to declare an array:
let berries = new Array( "Strawberry", "Blueberry", "Gooseberry" );
These code snippets create the same array, but using different methods. Notice that in our second example we’ve used circular brackets to declare our array. We’ve also had to use the new Array
constructor to declare an array. It’s for that reason that most people prefer the first method; square brackets are much easier to remember and are faster to type.
Arrays don’t have to include the same data type. Our array could store any number of different types of data:
let berries = [ "Strawberry", 1.50, true ];
This array stores three different types of data: a string, a number, and a boolean. Now that you’re familiar with how to declare an array, you’re ready to start accessing their contents.
Reading an Array
Arrays wouldn’t be very useful if we couldn’t read their values. That’s why indexing exists. Indexing is a way of accessing individual items in an iterable object, such as a list.
Every element of an array in an array is given its own index number. These numbers start with 0, and can be used to access individual items in an array. Remember our “fruits” array?
let fruits = [ "Banana", "Grapes", "Melon" ];
Here are the index values that are assigned to this array:
- 0: Banana
- 1: Grapes
- 2: Melon
By using these numbers, we can access individual items in our list. Let’s say you want to identify which fruit is in index position 1 in your array. You could do so by stating the index value in square brackets after the name of the array:
fruits[1];
Our code returns: Grapes. We’re referring to the index position 1, which stores the value “Grapes”. If you passed the value 0, “Banana” would be returned. If you try to access an item that doesn’t exist, “undefined” will be returned:
fruits[10];
Output: undefined. Where indexing is particularly useful is when you want to loop through the items in an array. Let’s say that you want to print out every fruit in your array to the console. You could do so using a for loop like this:
for (var i = 0; i < fruits.length; i++) { console.log(fruits[i]); }
This code returns:
Banana Grapes Melon
Our code loops through every item in our list, then prints each item out to the console. You’ll also notice we’ve used the length
property. This returns the length of our list.
Add Items to an Array
Most of the time, you’ll want to add a new value to an array after you’ve initially declared your array. You can do so using the push()
array method.
We’ve forgotten to add “Strawberry” to our list of fruits. Oh no! Don’t worry, we can always add it using push()
method:
let fruits = [ "Banana", "Grapes", "Melon" ]; fruits.push("Strawberry"); console.log(fruits);
Now that we’ve run this code, our array looks like this:
Banana | Grapes | Melon | Strawberry |
0 | 1 | 2 | 3 |
You can add data to the start of your array using unshift()
. Strawberries are so important on our shopping list that we want them to appear first:
let fruits = [ "Banana", "Grapes", "Melon" ]; fruits.push("Strawberry"); console.log(fruits);
This would make our original “fruits” list appear like this:
Strawberry | Banana | Grapes | Melon |
0 | 1 | 2 | 3 |
Remove Items from an Array
It turns out that we’ve already got a melon at home, so we don’t need to shop for one. What would be the point in buying another melon, right? Because melon is at the end of our list, we have two options to remove it:
splice()
: Removes elements by their index number.pop()
: Removes an element from the end of an array.
Let’s use splice()
as an example:
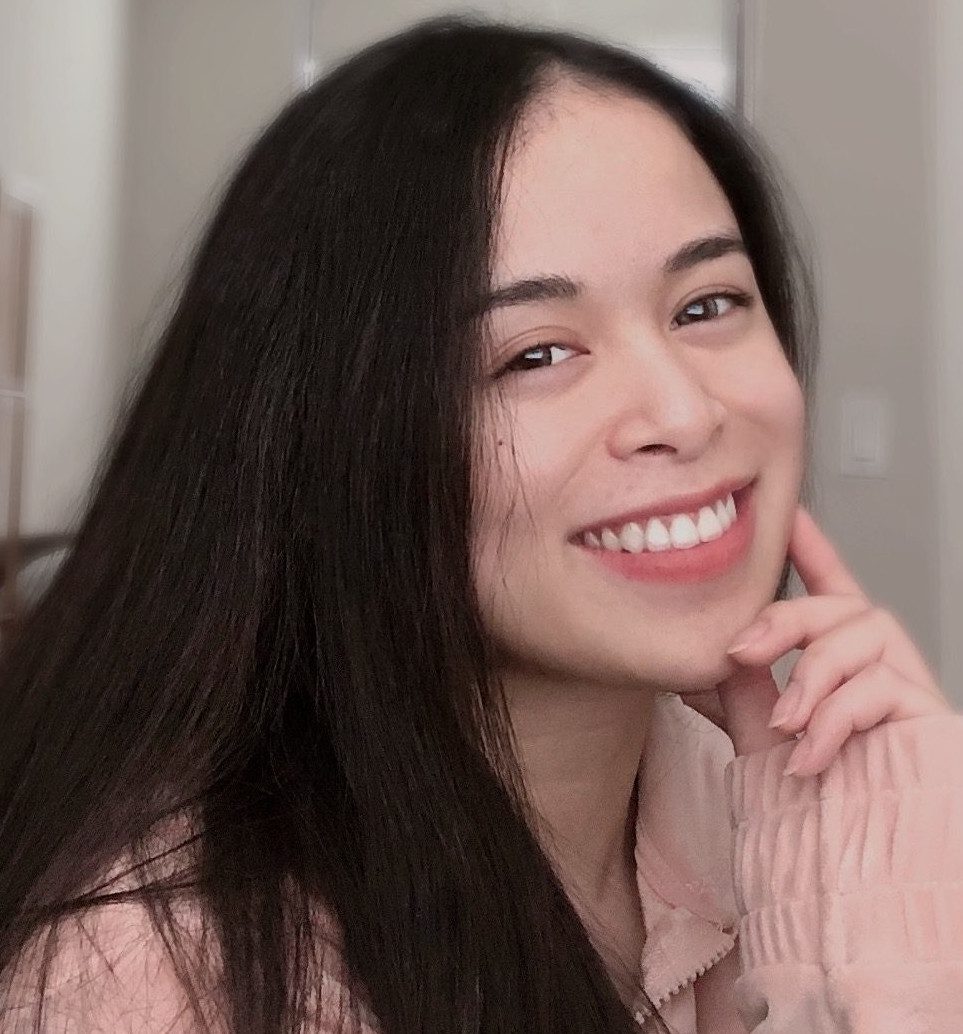
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
let fruits = [ "Banana", "Grapes", "Melon" ]; fruits.splice(3, 1); console.log(fruits);
Our code returns:
Strawberry Banana Grapes
splice()
accepts two parameters. The first is the index number to be removed from the array, and the second is how many items to remove. In this case, we wanted to remove the item with the index value 3, so we specified “3, 1” as our parameters.
pop()
doesn’t accept any parameters. It just removes the last item of a list:
let fruits = [ "Banana", "Grapes", "Melon" ]; fruits.pop(); console.log(fruits);
Our code returns:
Banana Grapes
You can also use shift()
to remove an item from the start of a list:
let fruits = [ "Banana", "Grapes", "Melon" ]; fruits.shift(); console.log(fruits);
Our code returns:
Grapes Melon
push()
and unshift()
add items to lists. pop()
and shift()
remove items from lists. The splice method can also be used to remove an item from a list based on its index value.
Changing Items in an Array
While bananas are a tasty fruit, often they are so big that you can’t eat one just as a snack when you are feeling a little bit peckish. Baby bananas are easier to eat.
Now, let’s say that we want to change our “fruits” list so that we shop for baby bananas instead of regular bananas. We could do so using the assignment operator like we would with any variable:
fruits[0] = "Baby bananas"; console.log(fruits);
Our code returns:
Baby bananas Grapes Melon
We’ve modified the item at the index position 0 to store the value “Baby bananas”. You don’t need to use any special methods to make this change; the assignment operator is used to change values in an array.
Conclusion
That’s the basics of what you need to know about JavaScript arrays. There is more for you to learn, but that’s what makes programming fun: there’s always a new challenge for you to tackle. If nothing else, you’ve learned about my favorite fruits. Although I’d say that I do love blackcurrants, which I didn’t mention in this tutorial.
Now you’re ready to start working with JavaScript arrays like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.