The JavaScript += operator adds two values together and assigns the result to a variable. This operator is called the addition assignment operator. It is more convenient than the regular variable = X + Y syntax.
A plus sign and an equals sign together? Is that a typo? In JavaScript, a plus and an equals sign side-by-side has its own meaning. It is the JavaScript addition assignment operator.
In this tutorial, we’re going to talk about what the JavaScript += operator is and how it works. We’ll walk through an example of this operator in action to help you learn how to use it.
What is the JavaScript += Operator?
The JavaScript += operator adds the value on the right of the operator to the variable on the left. The resultant value is then assigned to the variable on the left. This operator is called the addition assignment operator.
Let’s take a look at the syntax for this operator:
let welcome = "Hello there, "; console.log(welcome += "Sophie.");
We have declared a JavaScript variable called “welcome” whose value is “Hello there, “. Then, we have added “Sophie. to this value. The addition assignment operator adds these two values and then assigns the result to the “welcome” variable.
Our code returns:
Hello there, Sophie.
This operator has two uses. It is used to add two numbers together. It is also used to add the values of two strings together.
The assignment operator is another way of saying:
x = x + y
The addition assignment operator is a way to make your code easier to read. A += sign is much clearer than writing “variable = x + y” to add two values and assign the result to a variable.
You will often see the addition assignment operator in loops with a counter that tracks how many times the loop has executed.
JavaScript += Operator: Adding Numbers
The addition assignment operator allows you to add two numbers together. Let’s create a program which counts how many times “The Count of Monte Cristo” appears in a list. This list contains the results of a book club’s “Book of the Year” poll.
We’ll start by defining a JavaScript array containing the names of books. We shall also declare a variable to track how many times the book for which we are searching appears:
var books = ["The Count of Monte Cristo", "All My Sons", "Of Mice and Men", "The Count of Monte Cristo", "To Have and Have Not"]; var count = 0;
Next, we’ll write a JavaScript for loop which loops through this list and counts how many times “The Count of Monte Cristo” appears:
for (b in books) { if (books[b] === "The Count of Monte Cristo") { count += 1 } } console.log(`The Count of Monte Cristo was voted Book of the Year ${count} times.`);
This for loop iterates through the “books” list. For each book in the list, our program checks if the title is equal to “The Count of Monte Cristo”. If it is, we use the addition assignment operator to increment the value of “count” by 1. Otherwise, nothing happens.
Once our loop has run, our program prints out how many times the book has appeared in our list. Let’s try out our code:
The Count of Monte Cristo was voted Book of the Year 2 times.
Our code has counted how many times the book appears in the list.
Our code has counted how many times the book appears in the list.
+= Operator JavaScript: Strings
The JavaScript += operator can merge two strings together. This operator is more convenient than the long-form “variable = x + y” syntax.
For instance, say you have a user’s forename and the surname in two strings. You could use the += operator to merge these values into one string.
Let’s create a program that checks for any cake that begins with “B” in a list. If that cake begins with a “B”, it should be added to a new string. Otherwise, nothing should happen.
We’ll start by defining a list and a string:
var cakes = ["Babka", "Raspberry Ganache", "Strawberry Cheesecake", "Baked Alaska"]; var start_with_b = "| ";
The variable “start_with_b” will contain all the cakes that start with “B”. Initially, its value is “| ”.
Next, we’ll create a for loop to iterate through every cake and check if each cake starts with “B”:
for (cake in cakes) { if (cakes[cake].startsWith("B")) { var message = cakes[cake] + " | "; start_with_b += message } } console.log(start_with_b);
We use the JavaScript startsWith() method to check if each cake in our list starts with “B”.
If a cake starts with “B”, our if statement runs. Inside the if statement we declare a variable called “message”. This adds “ | ” to the end of every cake name. We do this so that they will appear separately in our string.
Next, we use the assignment operator to add the contents of “message” to the end of the “start_with_b” variable.
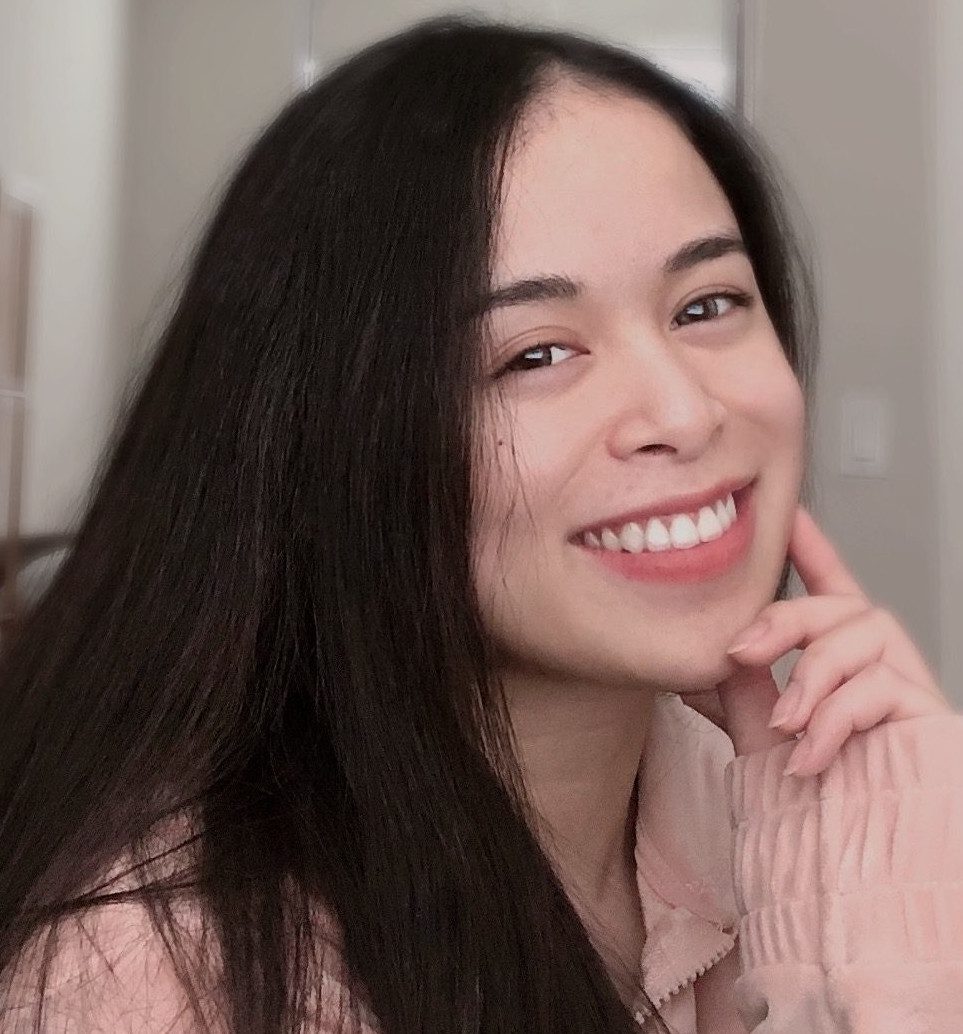
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Let’s run our code:
| Babka | Baked Alaska |
Our code returns a list of all cakes that begin with “B”.
The alternative to merge two strings is to use the concatenation operator or the concat() method. To learn more about these methods, check out our JavaScript string concatenation guide.
Conclusion
The addition assignment (+=) operator adds a value to another value and assigns the resultant value to a variable. It is often used to add values to the end of a string or to add numerical values together.
Do you want to learn more about JavaScript? Check out our complete How to Learn JavaScript guide for expert learning tips and guidance on top online books and courses.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.