Even the best programmers make mistakes in their code. An error could occur because of a typo, an unexpected user input, or for many other reasons which are difficult to track.
That’s where exception handling comes in. Good code includes exception handlers, which will respond to an error in code in a predefined way. Exception handlers are useful because they instruct a program on how to respond if and when an error occurs.
In Java, the try...catch
block is used for exception handling. This tutorial will discuss the basics of exception handling, how to use the try...catch
block to handle exceptions, and how to use the finally
statement with try...catch
. We’ll also walk through a few examples of the try...catch
block in action.
Java Exceptions
Exceptions are unexpected events that occur when a program is being executed. An exception will disrupt the flow of a program and can cause a program to stop running before it has completed.
Exceptions occur for a wide variety of reasons, which include: being unable to access a server, code errors, typos, opening non-existent files, erroneous user input, and more.
Error messages are unlike exceptions in Java. Errors refer to conditions being met from which a program cannot recover, such as running out of memory. So, errors are not usually handled by developers.
But exceptions are problems within a program that can be handled. When an exception is raised, an object is created called an exception object. These objects contain data on the exception, such as its name and where it was raised in the program.
Now you know the basics of exception handling in Java, we can start using the try...catch
statement to handle those exceptions.
Try Catch Java
The try...catch
statement is used to handle exceptions in Java. In order to handle exceptions, a block of code will be placed within a try_catch
block.
When a program encounters a try_catch
block, the code within the try
block will be executed. If an exception is raised, the code within the catch
block will be immediately executed. catch
blocks must come after a try
block in a try..catch
statement.
Here’s the syntax for a try_catch
statement in Java:
try { // Code to run } catch (ExceptionType error) { // Code to run when exception is raised } finally { // Code to run even if exception is not raised }
We’ll talk about the finally
block later in this tutorial, but for the moment, we’ll focus on the try_catch
block. Let’s walk through an example to illustrate how this block of code works.
Try Catch Java Example
Say that we are creating a program that uses an array called studentNames
. We want to print out the fifth name in our studentNames array to the console. However, there are only six students in our class.
This would result in an error being raised in our code because we would be referencing a value that did not exist. Here’s an example program that tries to print out the student name with the index value 5 (the sixth student in our class, because index values start at 0):
class Main { public static void main(String[] args) { String[] studentNames = {"Linda", "Greg", "Ron", "Graham", "Alexis"}; System.out.println(studentNames[5]); }
Our code returns:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5 at Main.main(Main.java:4)
As you can see, our program returned a long error because we tried to reference a value that doesn’t exist.
Now, let’s try to use a try...catch
block to handle our errors. When an error is encountered, our program should print out “There was an error”, so that we know something has gone wrong. We could use the following code to accomplish this task:
class Main { public static void main(String[] args) { try { String[] studentNames = {"Linda", "Greg", "Ron", "Graham", "Alexis"}; System.out.println(studentNames[5]); } catch (Exception e) { System.out.println("There was an error."); } }
Now when we run our code, the following response is returned:
There was an error.
Additionally, we could specify multiple catch
blocks. This is useful because it allows us to handle each exception differently.
In the example above, an ArrayIndexOutOfBoundsException
was raised. If we wanted to test for that error specifically, have a custom message printed to the console, and test for other general exceptions, we could use multiple catch statements.
Here’s an example of a program that tests for both an ArrayIndexOutOfBoundsException
and a general Exception and has custom responses for each error:
class Main { public static void main(String[] args) { try { String[] studentNames = {"Linda", "Greg", "Ron", "Graham", "Alexis"}; System.out.println(studentNames[5]); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("ArrayIndexOutOfBoundsException was raised."); } catch (Exception e) { System.out.println("There was an error."); } } }
Now, when we run our program, the following message is printed to the console:
ArrayIndexOutOfBoundsException was raised.
This response is returned because an ArrayIndexOutOfBoundsException
was raised in our program. But if another error occurred, the code in the Exception e
catch block would run, and the following message would be printed to the console:
There was an error.
Finally Statement
The finally
statement is used to execute a block of code after the try...catch
block has been executed. Thefinally
block is optional, and is always executed if it’s defined. If no exception is raised, the finally
block is executed after the try block; if an exception is raised, it’s executed after the catch
block.
Let’s use our example from above to illustrate the finally statement in action. Say that we want a message to be printed to the console stating This block has finished executing
. to tell us that the try_catch
statement is complete. We could try using the following code:
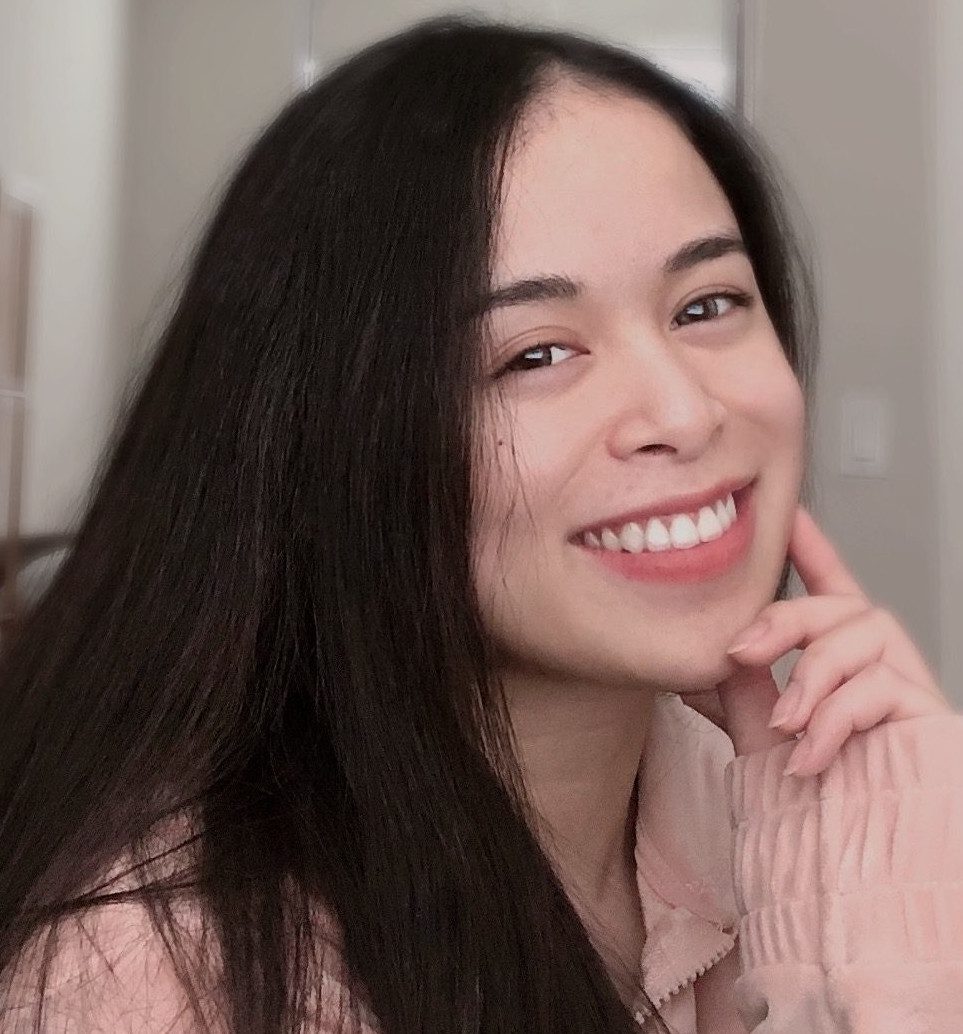
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
class Main { public static void main(String[] args) { try { String[] studentNames = {"Linda", "Greg", "Ron", "Graham", "Alexis"}; System.out.println(studentNames[5]); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("ArrayIndexOutOfBoundsException was raised."); } catch (Exception e) { System.out.println("There was an error."); } finally { System.out.println("This block of code has finished executing."); } } }
When we run our code, the following response is returned:
ArrayIndexOutOfBoundsException was raised.
This block of code has finished executing.
As you can see, our code raised the ArrayIndexOutOfBoundsException
exception, as we saw above, and it executed the code within the relevant catch
block.
In our example above, we specified a finally
statement. So, our code printed out the message This block of code has finished executing
. after the catch
block executed.
Conclusion
The try...catch
block is used in Java to handle exceptions gracefully. The code within the try
block is executed, and if an exception is raised, the code within the relevant catch
block is executed. In addition, the finally
statement is used to execute code after the try...catch
block has executed, even if no exception was raised.
This tutorial discussed how to use the try...catch
block to handle exceptions in Java and how to use the Java finally
block. We also walked through an example of both the try...catch
block and the finally
statement being used to handle errors.
You’re now equipped with the information you need to handle exceptions in Java with confidence!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.