In Java, there are a wide range of classes used to store particular types of data. Each class has its own features and the class used to store a type of data determines how it can be accessed and manipulated.
One of the most important classes in Java is the Vector class. Vector is an implementation of the List interface and is used to create resizable arrays.
This tutorial will walk through, with reference to examples, how to use the Vector class in Java to create resizable arrays. In addition, this tutorial will discuss how Vector compares to the ArrayList class, which is very similar to Vector.
Java List Interface
The List interface is used to create ordered sets of data in Java. For instance, a List may store a list of shoes sold in a shoe store or a list of the names of every employee that works for a bank.
However, since List is an interface, you cannot create an object from List. So, if you want to create a list, you need to use one of the classes that extend the List interface. These are: ArrayList, LinkedList, Vector, and Stack. This tutorial will focus on how you can use the Vector class to create a List object in Java.
Java Vector
Vector uses the List interface to create resizable arrays. To create a vector in Java, you can use the following syntax:
Vector<DataType> vector_name = new Vector<>();
Let’s break this syntax down into its basic parts:
- Vector tells our program we want to declare a vector.
- DataType is the type of data our vector will store.
- vector_name is the name of our vector.
- new Vector<>(); creates a new vector and assigns it to the
vector_name
variable.
For instance, suppose we wanted to declare a vector that stores all the colors of lamps our department store sells. We could use this code to declare the vector:
Vector<String> lamp_colors = new Vector>?();
Now we have a vector called lamp_colors
which can store all the colors of lamps our department store sells.
Java Vector Methods
The Java Vector class provides a number of methods that are used to retrieve and manipulate the data stored in a vector. Let’s break down a few of the most important methods offered by the Vector class.
Add Items to a Vector
There are three methods that are used to add items to vectors. The one you use will depend on how you want to add an item to a vector.
Add Element to Vector
The add()
method is used to add an element to a vector. The syntax for the add()
method is:
add(index, elementName);
The add()
method accepts two parameters:
- index is the index value at which the item you specify should be added to a vector.
- elementName is the item you want to add to a vector.
If you want to add an item to the end of a vector, the index
parameter is not necessary.
Suppose we are operating a department store chain and we are making a list of the colors in which a specific desk lamp is sold for our new furniture range. The manufacturer has just notified us that orange and blue lamps will accompany the next order, so we want to add orange and blue to our list of colors.
We could do so using this code:
import java.util.Vector; class Main { public static void main(String[] args) { Vector<String> lamp_colors = new Vector<>(); lamp_colors.add("Orange"); lamp_colors.add("Blue"); System.out.println("Vector: " + lamp_colors); } }
Our code returns:
Vector: [Orange, Blue]
As you can see, our code has added Orange
and Blue
to our lamp_colors
vector.
Suppose the manufacturer has just reached out to tell us that they are also going to deliver our gray lamps. We want to add gray lamps to the start of our list because we expect them to be more popular than other colors. We could do so using the example from above and adding this code:
import java.util.Vector; class Main { public static void main(String[] args) { Vector<String> lamp_colors = new Vector<>(); lamp_colors.add("Orange"); lamp_colors.add("Blue"); lamp_colors.add(0, "Gray"); System.out.println("Vector: " + lamp_colors); } }
Our code returns:
Vector: [Gray, Orange, Blue]
In this example, we have used the add()
method to add Gray
to the lamp_colors
vector. We specified the index parameter 0 in our code which tells add()
to add the Gray
item to the index position 0. In other words, we have added Gray
to the start of our vector. Then we tell our program to print out the entire vector.
Merge Vectors
The addAll()
method is used to add all elements from a vector to another vector.
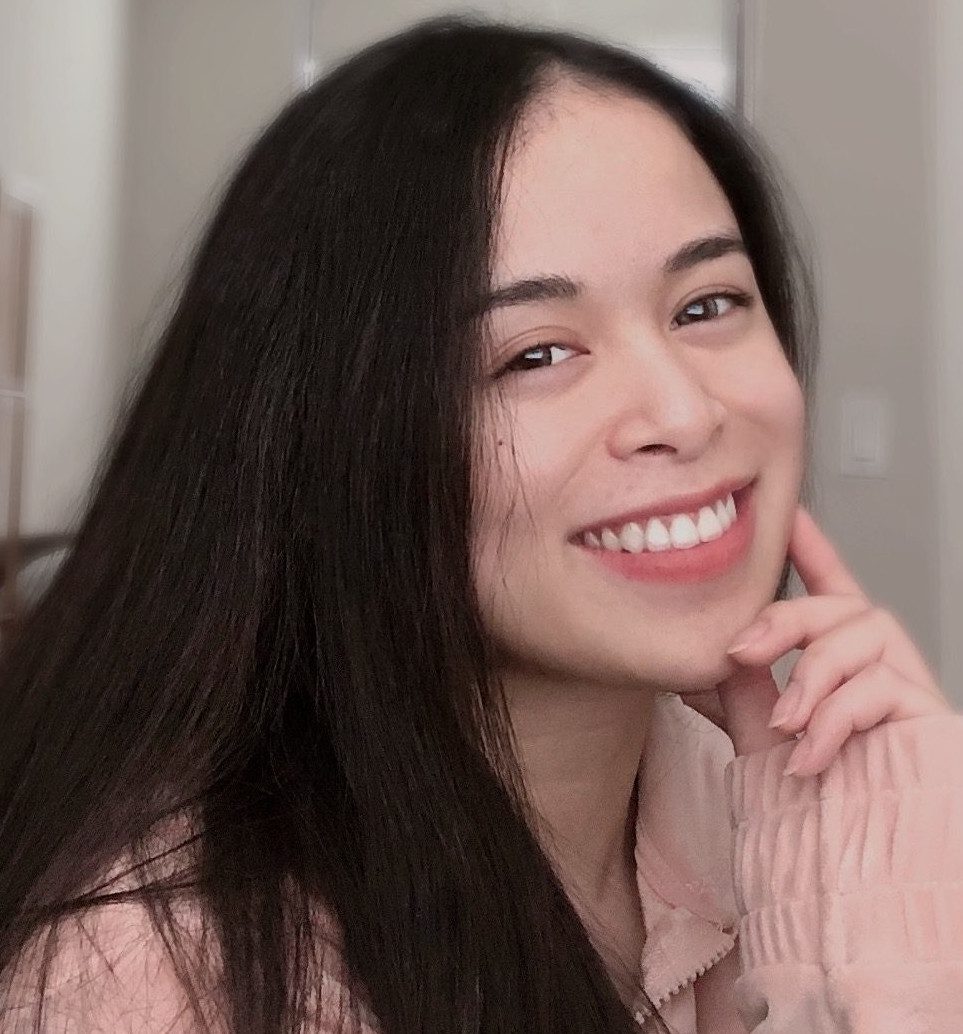
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose we have two lists: color range and lamp colors. We want to merge these two lists together because our lamp manufacturer has told us they will be able to manufacture lamps in every color in our color range. We could use the following code to merge these two vector lists together:
import java.util.Vector; class Main { public static void main(String[] args) { Vector<String> color_range = new Vector<>(); Vector<String> lamp_colors = new Vector<>(); color_range.add("Blue"); color_range.add("Black"); color_range.add("Gray"); color_range.add("Pink"); color_range.add("Orange"); lamp_colors.addAll(color_range); System.out.println("Color range: " + color_range); System.out.println("Lamp colors: " + lamp_colors); } }
Our code returns:
Color range: [Blue, Black, Gray, Pink, Orange] Lamp colors: [Blue, Black, Gray, Pink, Orange]
Our code first added the colors in our color range to the vector color_range
. Then we used addAll()
to add all colors from color_range
to the lamp_colors
vector. On the final lines in our code, we print out both the contents of color_range
and lamp_colors
to the console.
Retrieve Elements from a Vector
The get()
method is used to retrieve a particular element from a vector. get()
accepts one parameter: the index value of the item you want to retrieve.
Suppose our list of lamp colors is stored in alphabetical order and we want to retrieve the first item in our list. We could do so using this code:
import java.util.Vector; class Main { public static void main(String[] args) { Vector<String> lamp_colors = new Vector<>(); lamp_colors.add("Black"); lamp_colors.add("Blue"); lamp_colors.add("Gray"); lamp_colors.add("Orange"); lamp_colors.add("Pink"); String first_element = lamp_colors.get(0); System.out.println("First element in list: " + first_element); } }
Our code returns:
First element in list: Black
In our code, we use the get()
method to retrieve the first item in the lamp_colors
vector. We specify the index parameter 0, which tells our code to retrieve the first item in the vector, or in other words the item at the index position 0. Then, we print out the first element in the list to the console, preceded by the message First element in list:
.
Remove Elements from a Vector
There are three methods which can be used to remove elements from a vector: remove()
, removeAll()
, and clear().
Remove Single Element
The remove()
method is used to remove a single element from a vector. remove() a
ccepts one parameter: the index position of the element you want to remove from a vector.
Let’s return to the department store. Our manufacturer has notified us that they are no longer able to manufacture our orange lamps, and so we need to remove them from our list of colors in which our lamps are sold. We could do so using this code:
import java.util.Vector; class Main { public static void main(String[] args) { Vector<String> lamp_colors = new Vector<>(); lamp_colors.add("Black"); lamp_colors.add("Blue"); lamp_colors.add("Gray"); lamp_colors.add("Orange"); lamp_colors.add("Pink"); lamp_colors.remove(3); System.out.println("New color list: " + lamp_colors); } }
Our code returns:
New color list: [Black, Blue, Gray, Pink]
We have used the remove()
method to remove the item at index position 3 in our list. In this case, the value of that item is Orange
. Then we print out the revised color list to the console, preceded by the message New color list:
.
Remove All Elements
The removeAll()
and clear()
methods are used to remove all elements from a list. Generally, using clear()
is favored because it is more efficient, but you can also use the removeAll()
method.
Suppose the manufacturer has decided that they will no longer be able to manufacture our lamps because they are downsizing their operations. So, we will no longer be selling lamps until we find a new manufacturer. We could use the following list to remove all the colors of the lamps we sell:
import java.util.Vector; class Main { public static void main(String[] args) { Vector<String> lamp_colors = new Vector<>(); lamp_colors.add("Black"); lamp_colors.add("Blue"); lamp_colors.add("Gray"); lamp_colors.add("Orange"); lamp_colors.add("Pink"); lamp_colors.clear(); System.out.println("New color list: " + lamp_colors); } }
Our code returns:
New color list: []
We have used the clear()
method to remove all elements in the lamp_colors
vector. Then, on the final line of our code, we printed out the phrase New color list: “, followed by the empty vector.
Java Vector and ArrayList
Both ArrayList and Vector implement the Java list interface. These classes also provide the same methods. However, there are a few differences between the two classes.
The main difference to note is that the Vector class is synchronized, which means that only one thread at a time can access the code. ArrayList, on the other hand, is unsynchronized, and so multiple threads can work on the list at the same time.
Additional Vector Methods
In addition, there are a few other methods that can be used to manipulate the data stored within a vector. Here is a reference table with these methods:
Method Name | Description |
contains() | Searches a vector for a specific value |
iterator() | Iterates through a vector |
set() | Changes an element in a vector |
size() | Returns the length of a vector |
toArray() | Converts a vector to an array |
toString() | Converts a vector to a string |
Conclusion
The Vector class is used in Java to store data using the List interface. For instance, a Vector may be used to store a list of products sold at a department store or a list of supplements available at a local drug store.
This tutorial discussed the basics of the Java Vector class, how to create a vector, and the main difference between Vector and ArrayList. In addition, this tutorial walked through the main methods that can be used to retrieve and manipulate the contents of a vector, with reference to examples.
Now you have the skills you need to start using the Vector class in Java like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.