How to Use Java Variables
What is a variable, you ask? It’s an essential concept of programming, and without it developing applications would be significantly more difficult.
A variable is a way to label values that you’re using in your code. Variables are useful because once a variable has been declared, it can be reused as many times as you want in your program. There’s no need to repeat values in your code when you are using variables.
In this guide, we’re going to talk about what variables are, how they work, and how to work with variables in your Java programs. Let’s begin!
What Are Java Variables?
A variable is a value that is associated with a name, or label. This name can be used to reference the value that a variable stores.
Think about a variable as a label on a jam jar. The label tells you what is in the jam jar, whether it’s strawberry jam, raspberry jam, or another flavor entirely.
Consider the following code:
String jam = "Raspberry";
We have created a Java variable called “jam”. Every time that we reference the “jam” variable in our code, we will be able to access the value it stores. This means that we don’t have to repeat the word “Raspberry” multiple times in our code.
Java variables use the following syntax:
type name = value;
Type is the type of data that is stored in a variable. Once this has been set, it cannot be changed. Name
is the name of the variable and Value
is the value stored alongside that name.
Now that we have our variable declared, we can access its value. Let’s print out the value of the “jam” variable:
System.out.println(jam);
When we run our code, Raspberry
is returned.
Variables do not have to store strings; they can store any data type. For example, let’s say that we want to store the value 10 in a variable. We could do so like this:
int a = 10; System.out.println(a);
Our code returns 10. When you store values in a variable, you can manipulate them. We could use our variable from above to do some math:
int a = 10; int b = a - 5; System.out.println(b);
Our program returns the value 5; Java does all the math. Assigning the value of a math sum to a variable is useful because it means we can reference the answer multiple times.
You only need to perform that sum once—when you declare the variable—and you can use its answer throughout your program.
Variables can use other data types. For instance, a variable could be assigned to an object of a class. Here are a few examples of variables using floating-point numbers, a character, and a boolean:
float price = 2.99; char grade = "F"; boolean lightsOn = true;
Variables are like labels that allow you to store values in your code.
Variable Reassignment
The clue is in the name: variables can have variable values. There’s no rule in Java that says that once a variable is declared its values cannot be changed. As a result, your variable does not have to stay the same throughout your program.
To reassign a variable, you need to specify the name of a variable, followed by an equal sign, and then the new value you want to assign to that variable:
String jam = "Raspberry"' System.out.println(jam); jam = "Strawberry"; System.out.println(jam);
Our code returns:
Raspberry Strawberry
There is no need to state the data type of a variable when you reassign it. That’s because Java keeps track of the data type you have assigned to a variable.
Reassigning variables is important because it means that as your program runs, it can change values.
For instance, a guessing game may need to change the value of a counter so that you can only guess a number three times. Every time you guess, the counter should increase by one.
It’s important to note that variables can only be reassigned values of the same type assigned to that variable. Our “jam” variable from above could not be assigned an array, for example, because it was initially declared as a string. If we wanted to store a list of jams, we would need to declare a new variable and assign it an array.
Java Variable Types
There are three types of variables: local, static, and instance variables.
Local variables are declared inside the body of a method. These methods can only be accessed within a given method. In our examples so far, we have declared local variables, because they have been declared inside a method in our program.
Instance variables are declared inside a class but outside a method. Here’s an example of an instance variable:
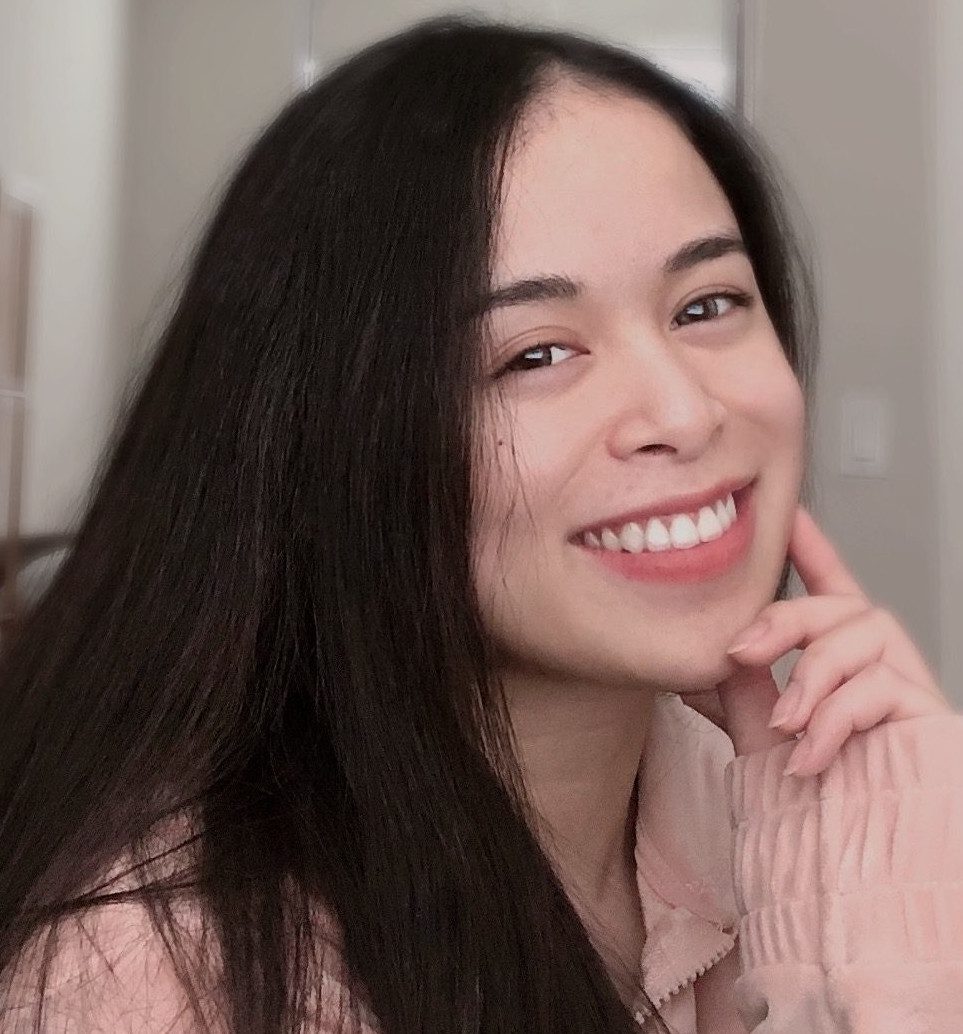
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
class Main { String jam = "Raspberry"; public static void main(String args[]) { // Our code } }
We have declared the variable “jam” inside our Main class but outside of our “main” method. This makes it an instance variable.
Static variables are any variable that is declared as static. To make a variable static, we need to declare it as static using the “static” keyword. Static variables cannot be local. Here’s an example of a static variable in Java:
class Main { static String jam = "Raspberry"; public static void main(String args[]) { System.out.println(jam); } }
We have declared a variable inside our class called “jam” which has the value “Raspberry”.
Declaring Multiple Variables
In Java, it’s possible to declare multiple variables on the same line of code. This is useful because it helps make your variable declarations more concise, and therefore more readable.
You should only declare a few variables on the same line if you decide to use this syntax. This will ensure that you don’t assign too many variables at once, which may introduce complexity into your code. You can only declare multiple variables at once that have the same type.
To declare multiple variables on the same line, you first need to specify the type of data those variables will have. Then, you can make a list of all the variables you want to declare.
Consider this example:
int a = 1, b = 2, c = 3; System.out.println(a + b - c);
Our code returns: 0. We have declared three variables: a, b, and c. Each of these variables has its own value: a is equal to 1, b is equal to 2, and c is equal to 3.
How to Name a Java Variable
Every developer has their own way of naming variables; it’s something that you pick up as you learn more about programming. With that said, there are a few rules that you should think about when you are deciding on what a variable should be called.
Variable names are often called identifiers.
In Java, variables cannot include any spaces. Variables can include letters, digits, and the underscore and dollar sign characters. However, a variable must begin with a letter.
Here are a few examples of valid variable names:
- raspberry
- peanutButter
- book
The following variable names are invalid:
- 1raspberry: This variable begins with a number, which is not allowed.
- _peanutButter: This variable begins with a special symbol.
- book.: This variable ends with a special character that is not an underscore or a dollar sign.
When you are naming a variable, you should make sure the purpose of that variable is clear. A program that asks a user for their age may store that value in a variable called “age”; it wouldn’t make sense if that value was stored in a variable like “a” or “number”.
Most Java developers prefer camel case when naming variables. This is where the first word inside a variable will begin with a lower case character and each subsequent word will begin with an upper-case character. Consider this variable:
boolean javaIsCool = true;
This variable “javaIsCool” contains three words and therefore it uses camel case. “Is” and “Cool” are both capitalized.
Java variable names are case sensitive. This means that “javaIsCool” and “javaiscool” are two separate variables. They may contain the same basic characters but they use different cases.
Declaring a Final Variable
Earlier in this guide, we mentioned that variables can be reassigned. This is only true if a variable is not declared with the final
keyword. This sets the value of a variable as final, which means that it is only readable by a program.
To declare a final variable, you must begin a variable declaration with the keyword final
:
final boolean javaIsCool = true;
If we try to reassign this variable, an error will be returned:
final boolean javaIsCool = true; javaIsCool = false;
This code fails and returns the following error:
error: cannot assign a variable to a final variable
Conclusion
Variables allow you to store values alongside a label in your code. Once you have declared a variable, you can reference it throughout your program. This means that you don’t need to repeat values that you intend to use more than once.
Variables can be reassigned as long as they were not declared using the final
keyword.
Now you’re ready to start working with variables in your Java code like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.