Strings are a built-in data type in Java used to store text. When you’re working with a string, you may want to convert the case of the string to all-uppercase or to all-lowercase. For instance, if you’re building an app that compares two names, you would want to convert the case of the names because comparisons are case-sensitive.
That’s where the Java toUpperCase() and toLowerCase() methods can be useful. These string methods are used to convert a string to all-uppercase and all-lowercase, respectively. This tutorial will discuss how to use these methods, with reference to examples, and explain where you may want to use toUpperCase() and toLowerCase() in your code.
Java Strings
Strings are sequences of one or more characters and can include letters, numbers, whitespaces, and symbols. In Java, strings are declared by surrounding a sequence of characters by double quotes. Here’s an example of a string in Java:
String fruit = “Strawberry”;
In Java, strings are stored as objects, which means they offer a number of methods that can be used to manipulate their contents. toUpperCase() and toLowerCase() are two examples of the many string methods available in Java.
Strings in Java are case sensitive, and so if you compare two strings, a true value will only be returned if the strings are identical and use the same cases.
This is important to note in cases where you are comparing two strings that may have different cases. For instance, if you store a user’s email address in all-lowercase (a common practice for security reasons), then you’ll want to convert their email address to lowercase when they try to sign in. That way, you can perform an equal comparison between the two values.
Java toUpperCase
The toUpperCase() method is used to convert a string to all-uppercase. toUpperCase() iterates through every character in a string and changes it to an uppercase character.
Here’s the syntax for the toUpperCase() method:
string_name.toUpperCase();
toUpperCase() is appended at the end of the string value that you want to convert to uppercase. This is because toUpperCase() is a string method, which is appended at the end of string values in Java.
toUpperCase() does not modify our original string. Instead, it creates a new copy of the original string but in all-uppercase.
So, suppose we wanted to convert the string Java is great
to all-uppercase. We could do so using this code:
String java_is_great = "Java is great"; System.out.println(java_is_great.toUpperCase();
Our code returns: JAVA IS GREAT
.
Let’s walk through an example of the Java toUpperCase() method.
Suppose we are creating an application that prints out a list of expected attendees at an exclusive local club. This list will be given to the door attendants who will check the name customers give against the list to see if the customer is allowed to enter the club. We want every name on our list to be written in uppercase so that it is easy for our door attendants to read each name.
We could use the toUpperCase() method to convert each name on our list of attendees to uppercase. Here’s an example program that accomplishes this task:
public class PrintAttendees { public static void main(String[] args) { String[] attendees = {"Liam Miller", "Michael Curtis", "Andrew Tamera", "Joan Beverly", "Sarah Klein", "Mary Blackwood"}; for (String i : attendees) { System.out.println(i.toUpperCase()); } } }
Our code outputs the following:
LIAM MILLER MICHAEL CURTIS ANDREW TAMERA JOAN BEVERLY SARAH KLEIN MARY BLACKWOOD
As you can see, our code has converted each string to uppercase. Let’s break down our code step-by-step and discuss how it works. First, we declare a class called PrintAttendees, which stores our code for this example.
Then, we initialize an array of string values called attendees
, which stores the names of people who are on the guestlist for the club. On the next line, we create a “for each” loop that loops through each item in the attendees
array.
Finally, our code uses toUpperCase() to convert each individual name to uppercase, and prints out the uppercase value to the console.
Java toLowerCase
The string toLowerCase() method is the opposite of the toUpperCase() method. Instead of converting a string to all-uppercase, the toLowerCase() method converts a string to all-lowercase.
Here’s the syntax for the toLowerCase() method:
string_name.toLowerCase();
toLowerCase() is a string method, and so it is appended to the end of a string value.
Suppose we are creating an “update profile” form, and before a user can update their email address, we first want to check if the new email a user has submitted is the same as their old one. If so, we want to ask the user to choose a new email.
Because email addresses are case sensitive and we store all email addresses in lowercase, we want to convert the email the user has submitted to lowercase so that we can compare the two strings.
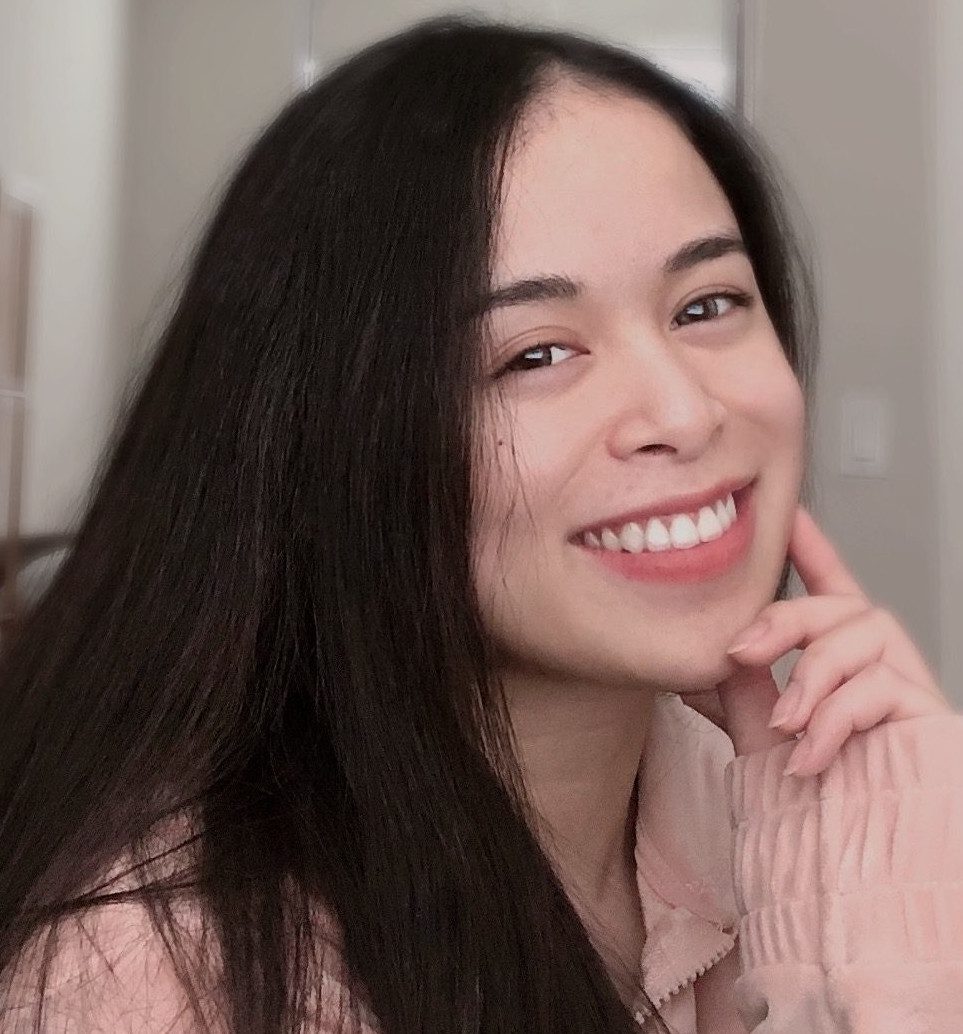
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
We could use the following code to accomplish this task:
public class CompareEmails { public static void main(String[] args) { String old_email = "linda.craig@gmail.com"; String new_email = "Linda.craiG@gmail.com".toLowerCase(); if (old_email.equals(new_email)) { System.out.println("Choose a new email address."); } else { System.out.println("Your email address has been changed."); } } }
Our code returns:
Choose a new email address.
Let’s break down our code. First, we define a class called CompareEmails, in which we have written the code for our program. Then, we declare a variable called old_email
which stores the user’s old email address.
On the next line, we declare a variable that contains the user’s new email address. We use the toLowerCase() method to convert the value of the email address to lowercase so that our program can compare the two email addresses.
Next, we declare an if
statement that checks whether the user’s new email is equal to their old one. If it is, the message Choose a new email address.
is printed to the console; otherwise, Your email address has been changed.
is printed to the console.
In this case, while the email the user has submitted contains a capital L
, there are no differences in the contents of the string after we convert it to all-lowercase. So, our program printed out Choose a new email address.
to the console.
Conclusion
The string toUpperCase()
method is used in Java to convert a string to upper case, and the toLowerCase()
method is used to convert the contents of a string to lower case.
This tutorial discussed how to use these methods in Java programs, with reference to examples, and explored where they may be useful. Now you’re ready to start converting string cases using toUpperCase()
and toLowerCase()
like a Java master!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.