Exceptions are unexpected events that occur during program execution. When your code encounters an exception, the flow of your program will be terminated.
It’s important that you handle exceptions correctly when you’re coding in Java. Otherwise, your code may terminate during execution and affect the end-user experience.
That’s where the throw and throws keywords come in. The Java throw and throws keywords are used to handle exceptions in Java. This tutorial will discuss, with reference to examples, how to use these keywords in Java.
Java Exceptions
In Java, there are two events that can impact the flow of your program: errors and exceptions.
The first event is an error, which represents problems in Java Virtual Machine such as memory leaks, the system running out of memory, and library compatibility issues. When an error is encountered, a program is not likely to recover. However, errors are usually outside of the control of the coder, and so in Java, we do not handle errors.
Exceptions are the second type of event that can impact your program. Exceptions can be caught and handled in your code. When an exception is encountered, an object is created with information about the exception, which you can then use to tell your code what should happen if an exception is encountered.
Some reasons exceptions occur include:
- Errors in your code
- Opening non-existent files
- Invalid user input
- Losing network connectivity
There are two types of exceptions you should know about before you start handling exceptions:
- Unchecked exceptions are checked at run-time. These include
ArithmeticException
andArrayIndexOutOfBoundsException
. - Checked exceptions are checked at compile-time, and include
IOException
andInterruptedException
.
For the most part, you want to handle checked exceptions in your code. This is because unchecked exceptions are generally a result of programming errors, rather than unexpected behavior in your code.
Java Throws Keyword
The Java throws
keyword is used to declare the type of exceptions that could arise in a block of code. Here is the syntax for the throws clause:
accessModifier return functionName() throws Exception1, Exception2 { // Run code }
Let’s use an example to illustrate how this may work. Suppose we are building a program for a cinema that checks the age of a customer attending a movie for over 16s. If the customer is under the age of 16, they should not be allowed into the movie; if they are 16 or over, they should be permitted to enter.
Here’s the code we would use to check the age of the customer and throw an ArithmeticException exception if the customer is under the age of 16:
class Main { public static void verifyAge(int age) throws ArithmeticException { if (age < 16) { throw new ArithmeticException("This customer is not old enough to see the movie."); } else { System.out.println("This customer is old enough to see the movie."); } } public static void main(String[] args) { verifyAge(14); } }
Our code returns:
Exception in thread “main” java.lang.ArithmeticException: This customer is not old enough to see the movie.
at Main.verifyAge(Main.java:4)
at Main.main(Main.java:11)
When we run this program, the age parameter we specify in our main program is set to 14. This is less than 16, so when the verifyAge()
method is executed, the statement age < 16
evaluates to true, and an ArithmeticException is thrown.
The throws keyword is used to define the type of exception that will be returned by the verifyAge()
method. The throw keyword is used to throw the exception in our program.
Java Throw Keyword
The Java throw keyword is used to throw a single exception in your code. The throw keyword is followed by an object that will be thrown in the program if an exception is encountered.
Here’s the syntax for the Java throw keyword:
throw throwObject;
Let’s walk through a few examples of the throw statement being used to handle exceptions in Java.
Throw Checked Exception
Suppose we want a message to appear that states, “This file does not exist.” when an IOException
is encountered in our program. IOExceptions
are checked exceptions, and so we should handle them using the “throw” keyword. Here’s the code we would use to handle the exception:
import java.io.*; class Main public static void getFile() throws IOException { throw new IOException("This file does not exist."); } public static void main(String[] args) { try { getFile(); } catch (IOException error) { System.out.println(e.getMessage()); } } }
Our code returns:
This file does not exist.
Let’s break down our code. First, we import the java.io
library, which we would use in any program that handles files. Then we create a class called Main, which stores the code for our program.
In our Main
class, we define a function called getFile()
which throws an IOException
. This function will throw an IOException accompanied by the message “This file does not exist.” to our program.
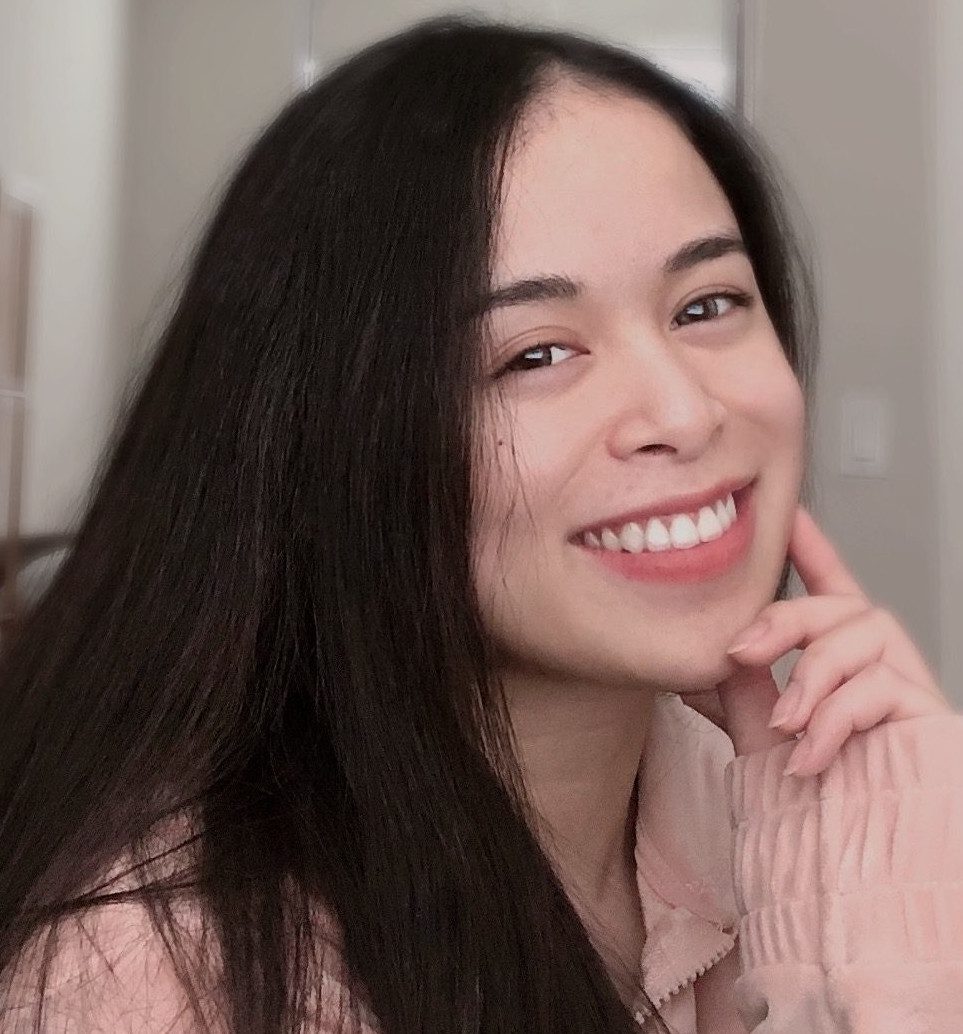
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
In the main function in our Main class, we create a try/catch block that tries to execute the getFile()
method. If an IOException is encountered, the code within the catch block will execute, and the message from the IOException will be printed to the console. So, our code returns, “This file does not exist.”, which is the message that we declared earlier.
Throw Unchecked Exception
So, suppose we wanted to throw a StringIndexOutOfBounds
exception if our program tries to access an index value in a string that does not exist. We could do so using this code:
class Main { public static void outOfBounds() { throw new StringIndexOutOfBoundsException("This index value is invalid."); } public static void main(String[] args) { outOfBounds(); } }
There’s a lot going on in our code, so let’s break it down. First, we have declared a class called Main in which our code for this program exists.
Then we have created a function called outOfBounds()
which, when executed, will throw a StringIndexOutOfBounds
exception. The message This index value is invalid.
will accompany the exception. Finally, in our main program, we call the outOfBounds()
function, which then returns:
Exception in thread “main” java.lang.StringIndexOutOfBoundsException: This index value is invalid.
at Main.outOfBounds(Main.java:3)
at Main.main(Main.java:7)
In this example, we have thrown an unchecked exception. This is usually not necessary, as we discussed earlier, but we have thrown an unchecked exception anyway to show you the syntax.
Conclusion
Handling checked exceptions is an important part of writing maintainable code in Java. In Java, the throw and throws keywords are used to throw and handle exceptions.
This tutorial covered how to use the throw and throws keywords in Java, and provided relevant examples to help you learn the process. After reading this tutorial, you are ready to start throwing and handling exceptions in Java like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.