How to Use the Java this Keyword
When you’re starting to code in Java, you’ll likely notice the keyword this
being used in methods or constructors. The this
keyword refers to the current object inside methods or constructors, and has a wide range of uses in Java.
In this tutorial, you’ll learn about the Java this keyword and about how and why it is used. We’ll also refer to a few examples throughout the article to demonstrate the this keyword in action.
Java Methods and Constructors
Methods and constructors are two important parts of programming in Java.
Functions, or methods
as they are called in object-oriented programming, are blocks of code that perform a specific task. For instance, a method, when executed, may print out the contents of an array to the console, or facilitate user input in a program.
Here is an example of a method in Java that prints It’s Monday!
to the console:
public class PrintMessage { public static void main(String[] args) { System.out.println("It's Monday!"); } }
When we execute our code, the following output is returned to the console: It’s Monday!
Constructors are similar to methods and are invoked when an object is instantiated, or created, in a program. Here’s an example of a constructor that sets the value of an instance variable called day
to Monday
when an instance of Monday is instantiate and prints out It’s
, followed by the day of the week, to the console:
class Monday { private Monday() { day = "Monday"; } public static void main(String[] args) { Monday day_of_the_week = new Monday(); System.out.println("It's " + day_of_the_week.day); } }
Our code returns: It’s Monday!
If you’re interested in learning more about constructors, you can read our full guide to Java constructors. Now that we’ve explored the basics of Java methods and constructors, we can start to discuss the this
keyword and how you can use it in your code.
Java this Keyword
In Java, the this
keyword refers to the current object inside a method or a constructor.
To illustrate this, let’s use our example constructor from above that prints out the day of the week to the console. In the below example, we have printed out the value of this
and the value of the Monday()
object we instantiated:
class Monday { private String day; private Monday() { day = "Monday"; System.out.println(this); } public static void main(String[] args) { Monday day_of_the_week = new Monday(); System.out.println("It's " + day_of_the_week.day); System.out.println(day_of_the_week); } }
Our code returns the following:
Main@d716361 It's Monday Main@d716361
The first line in our output is the value of this
, which is printed to the console when the Monday()
object is instantiated. The second line in our output is the result of printing out It’s
, followed by the value of day
in the day_of_the_week
object we declared. Finally, we print out the value of the day_of_the_week
object.
As you can see, the value of this
and the day_of_the_week
object are the same. This means that the this
keyword refers to the current object inside a method or a constructor.
Java this Use Cases
There are two main situations where using the this keyword is valuable. These are:
- Using this to disambiguate variable names
- Passing this as an argument
A variant of the this keyword, this()
, is also used when overloading constructors. However, this is a more advanced usage of this, and so we won’t discuss it in this article.
Using this to Disambiguate Variable Names
Two or more variables cannot have the same name inside a scope in Java. This means that if you try to declare two variables and use the same name, your program’s logic will be affected.
However, we can circumvent this limitation by using the this
method. this
allows us to assign a specific variable to an object, which allows us to store both the value of the variable in the object and the value of the initial variable.
Let’s walk through an example to illustrate how this works. Suppose we are building a program that keeps track of the name of a student who is being added to the honor roll in a fifth grade class. This application should print out the student’s name to the console.
Here’s the code we will use to build this application:
class HonorRoll { String studentName; HonorRoll(String studentName) { this.studentName = studentName; } public static void main(String[] args) { HonorRoll alex = new HonorRoll("Alex"); System.out.println("New honor roll inductee: " + alex.studentName); } }
When we run our program, the following is returned:
New honor roll inductee: Alex
In this example, we use this
to keep track of the student’s name in the HonorRoll
method. We use the following line of code to assign the value of the studentName
constructor parameter (which is Alex
in the above example) to the variable this.studentName
. If we didn’t do this, the following would be returned:
New honor roll inductee: null
This is because, in order for our HonorRoll
method to store the student’s name, we need to use this
to remove any ambiguity from the Java compiler. So, if we remove the word this
from our code, we get a null value.
Passing this as an Argument
The this
keyword is often used to pass the current object in a method or constructor to another method.
Suppose we are building an application that tracks how many bags of coffee beans a local coffee shop has in stock. This application should allow the coffee shop owner to add to the total of bags left in stock, which will be used when more stock becomes available. Here’s the code we could use to create this application:
class AddStock { int stockToAdd; int stockTotal; AddStock(int stockToAdd, int stockTotal) { this.stockToAdd = stockToAdd; this.stockTotal = stockTotal; System.out.println("Current stock: " + this.stockTotal); calculateNewStock(this); System.out.println("New stock: " + this.stockTotal); } void calculateNewStock(AddStock s) { s.stockTotal += s.stockToAdd; } } class Main { public static void main(String[] args) { AddStock newStockValue = new AddStock(10, 9); } }
Our code returns:
Current stock: 9 New stock: 19
Let’s break down the code. In our code, we have defined a class called AddStock
. This class contains a method which takes in two values—stockToAdd
and stockTotal
—and invokes the calculateNewStock()
method to calculate how many bags of coffee the coffee shop has in stock.
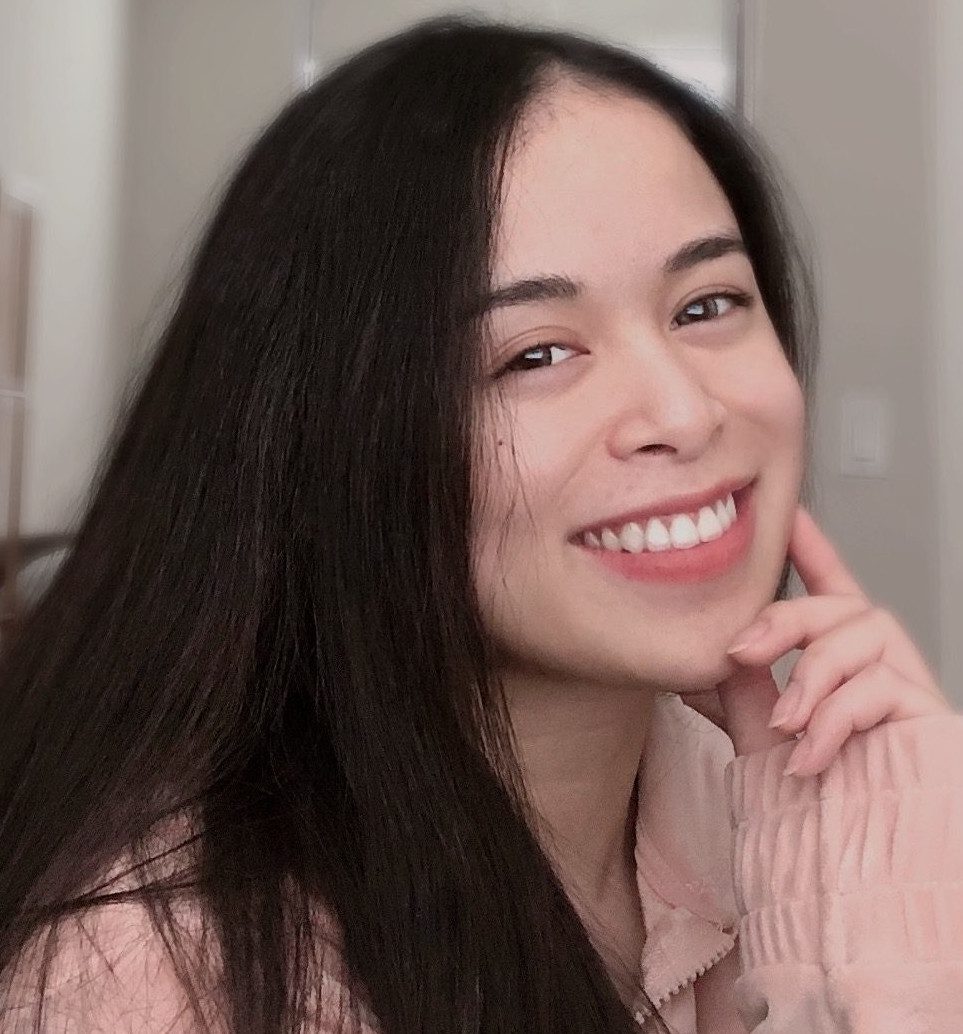
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
We then use the Main()
class to initialize an instance of the AddStock class and add two values together: 10 and 9. After our program executes, our code adds the two values we specified—the stock to add and the current total of stock, respectively—and prints out both the current stock and the revised stock levels to the console.
In this example, the this
keyword is used as an argument when we call the calculateNewStock()
method. This allows us to pass down the variables we are working with in the AddStock class to the calculateNewStock()
method, which needs those variables to calculate the revised stock levels.
Conclusion
The Java this keyword refers to the current object inside a method or constructor. In Java, the this keyword has two main uses: to disambiguate variable names, and to pass this as an argument
This tutorial discussed, with reference to examples, how to use the Java this keyword in those three contexts. Now you’re equipped with the knowledge you need to start using the Java this keyword like an expert.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.