The Java ternary operator lets you write an if statement on one line of code. A ternary operator can either evaluate to true or false. It returns a specified value depending on whether the statement evaluates to true or false.
We use Java if…else statements to control the flow of a program. An if statement will evaluate whether an expression is true or false. This statement executes a specific block of code if the expression is equal to true.
However, if…else statements span multiple lines. If you’re evaluating a basic expression, your syntax can become unnecessarily wordy. That’s where the ternary operator comes in. The Java ternary operator is used to replace simple if…else statements to make your code easier to read.
This tutorial will discuss how to use the Java ternary operator. We’ll walk through an example so you can learn how to use this operator. Let’s get started!
Java Ternary Operator
The Java ternary operator lets you write concise if…else statements. Ternary statements get their name because they take three conditions. A ternary operator evaluates whether a statement is true or false and returns a specified value depending on the result of the operator.
Here is the syntax for a ternary operator in Java:
variable = (expression) ? expressionIsTrue : expressionIsFalse;
The origin of the name “ternary” refers to how a ternary operator has three parts. Our statement takes in three operands:
- expression is the expression the operator should evaluate
- expressionIsTrue is the value assigned to variable if the expression is true
- expressionIsFalse is the value assigned to variable if the expression is false
You do not need to assign the contents of the ternary operator to a variable. For instance, you could write a ternary operator in a System.out.println() statement. This will let you see the result of your ternary operator in the Java console.
Unlike an “if” statement, the ternary operator does not accept an “else” keyword. The ternary statement uses the colon (:) to represent an “else” condition.
Let’s use an example to show this operator in action.
Ternary Operator Java Example
Suppose that we are building a shopping website. We only want people to be allowed to buy products if they are aged 16 or over.
To verify the age of our customers, we could use a ternary operator. This is more efficient than using an “if” statement because a user can only be under 16 or aged 16 and over. Here is an example program that would accomplish the task of verifying the age of a user:
public class EvaluateAge { public static void main(String[] args) { int age = 22; String result = (age >= 16) ? "This user is over 16." : "This user is under 16." System.out.println(result); } }
Our code evaluates our ternary. Our condition is true so our code returns:
This user is over 16.
First, we define a class called EvaluateAge. Then, we declare a Java variable called age. This variable stores the value of our customer’s age. age is assigned the value 22.
We declare a variable called “result” whose value is equal to the result of our ternary operator. The ternary operator evaluates whether the user’s “age” is equal to or greater than 16 (“age >= 16”).
If the expression evaluates to true, the operator returns “This user is over 16.”. Otherwise, the operator returns This user is under 16. On the final line of our code, we print out the message returned by the result variable.
If our user’s age was equal to 15, our code would return the following Java string result:
This user is under 16.
This is because our ternary evaluates to false if the user’s age is not equal to or greater than 16. We have successfully built a system to check whether a user is old enough to use our services.
When to Use Ternary Java Operators
Termaru operators should be used if you have a simple “if” statement that you want to appear more concise in your code. The ternary operator makes your code more readable.
In our example above, we evaluated one expression. If we wrote out the code to evaluate our user’s age as a full “if” statement, we would have written:
if (age >= 16) { String result = "This user is over 16." } else { String result = "This user is under 16." }
This if statement is simple, but it spans across five lines. By using a ternary statement, we reduced our if statement down to a single line.
Overall, you should only use ternary statements when the resulting statement is short. Otherwise, write a normal if statement. The purpose of a ternary operator is to make your code more concise and readable. Moving a complex if statement into a ternary operator goes against that goal.
Both the Java ternary and if statement conditional operators evaluate Boolean expressions. Boolean expressions are those where the only output can be true or false. To learn more about Java Booleans, read our complete guide to Java Booleans.
Conclusion
The ternary operator is a feature in Java that allows you to write more concise if statements to control the flow of your code. These operators are referred to as ternary because they accept three operands.
In this tutorial, we covered the basics of Java ternary operators. We also explored how ternary operators compare with Java if statements, along with examples of each in action.
Do you want to learn more about coding in Java? Check out our complete How to Learn Java guide. You will find a list of top online courses as well as expert advice on learning the Java programming language.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
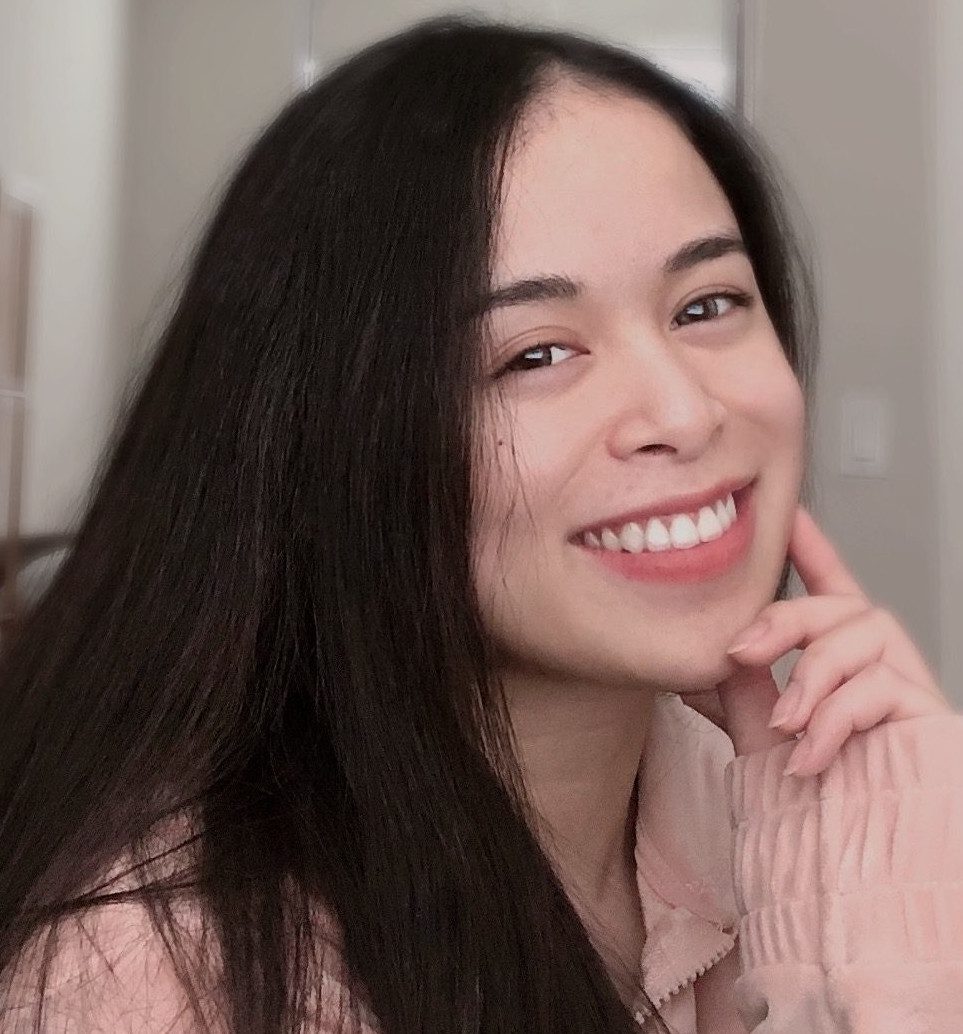
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot