Conditional statements are a common feature in all programming languages. We use conditional statements to control the flow of programs. In Java, if...else
statements are used to control program flow based on a certain set of conditions.
Additionally, Java offers a feature called the switch
statement, which will evaluate an expression against multiple cases. If a statement matches a specified case, the block of code corresponding to that case will be executed.
In this tutorial, we’ll discuss how to use the “switch” statement in Java, and how to use the default
, case
and break
keywords. We’ll also walk through an example of these keywords being used in a Java switch statement.
Conditional Statements Refresher
Conditional statements allow programs to execute code when a certain condition evaluates to True. For instance, a conditional statement could tell a program to run a block of code only if the variable name
contained the letter F.
In Java, there are two conditional statements you can use to control the flow of your code: if...else
statements and switch
statements.
if...else
statements execute a block of code if a condition evaluates to true. Here’s an example of an if
statement in Java:
if (15 > 5) { System.out.println("15 is greater than 5."); };
Our code evaluates the expression 15 > 5
, which is true, and so returns the following:
15 is greater than 5.
Additionally, you can specify else
blocks, which will run code if all conditions evaluate to false, and else...if
blocks which specify a new condition to test if the first condition is false.
The switch
statement can also be used to perform a conditional evaluation in your code.
Switch Statement Java
The Java switch
statement is used to evaluate a statement against one or more conditions and will execute the blocks of code corresponding to the conditions that evaluate to True.
The switch
statement contains a case
statement, which is used to specify conditions against which an expression should be evaluated. Here’s the syntax for a Java switch statement:
switch(expression) { case a: break; case b: break; case c: break; default: break; }
Let’s break down how it works.
The expression contained in the switch statement is evaluated once. Then, the value of the expression is compared with the values of each case
, starting from the top of the switch statement. If the expression matches a case, the block of code associated with the case statement is executed. If an expression does not match a case, the subsequent case label will be evaluated.
If none of our conditions evaluate to true, the contents of the default
statement will be executed.
Switch Statement Example
We’ll walk through a working example of the switch statement to illustrate how it works. Let’s say we want to create a program that tells us the name of the month based on the numeric value of the month. We only want our program to work with the first six months of the year.
To create this program, we’ll use the time.LocalDate
Java method to get the numeric value corresponding to this month. The number 1 represents January, 2 represents February, and so on.
Before we begin, we’ll first need to set up the code that gets the numeric value corresponding to this month. Here’s the code we can use to retrieve this data:
LocalDate today = LocalDate.now(); int month = today.getMonthValue(); System.out.println(month);
Our code returns the numeric value which represents the current month, which in this case is 2 (this article was written in February).
Using the switch
statement, we can send a message to the console with the name of the month based on the numeric value we have calculated in our code above. The program will run from top to bottom and look for a match. Once a match is found, the break
statement will stop the switch
statement and continue executing the program.
Here’s the code we could use for our calendar program:
LocalDate today = LocalDate.now(); int month = today.getMonthValue(); switch (month) { case 1: System.out.println("January"); break; case 2: System.out.println("February"); break; case 3: System.out.println("March"); break; case 4: System.out.println("April"); break; case 5: System.out.println("May"); break; case 6: System.out.println("June"); break; }
When we execute our code, the following response is returned: February
.
Let’s break down how our code works. At the start, we use the LocalDate method to get the numeric value, which represents this month. Then, we define a switch
statement that has six cases.
The program executes each case one-by-one until it finds one that evaluates to true. In this case, the case 2
statement evaluates to true, because it’s February, which has the numeric value 2. Then, our program prints out the name of the month to the console and executes a break
statement, which stops our program from continuing.
If it was May, for example, the month value would be 5, and so May
would be printed to the console.
Break Keyword
In our code above, we used the break
keyword. When Java executes a break
statement, it’ll stop running the code within the switch
block and continue running the program.
This statement is important because it stops a program from testing for more cases when a case has already been met. This saves time on execution because the program doesn’t need to evaluate more cases after the right case has been found. You should use break
statements at the end of every case
.
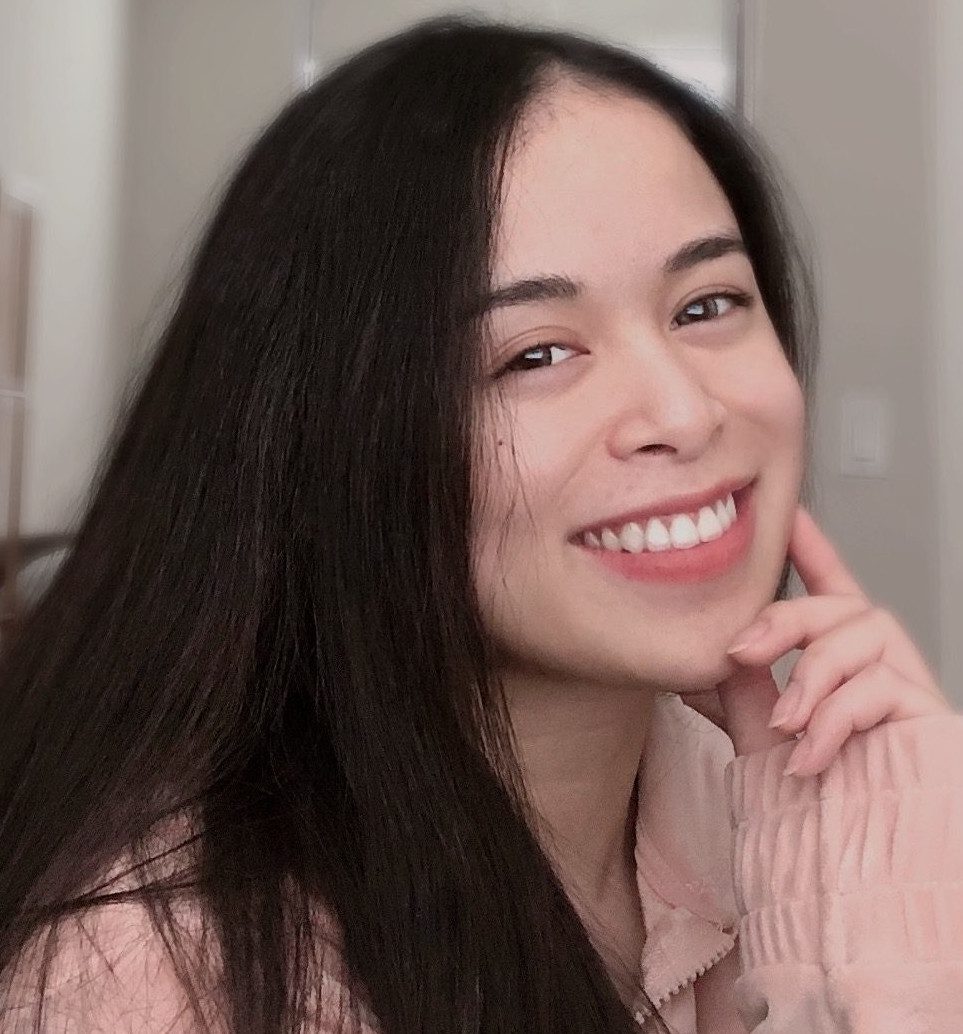
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here’s an example of the break
statement from our code above:
… case 4: System.out.println("April"); break; …
If it was April, this case would be executed, and then the program would break out of the switch statement because a break
statement is present.
Default Keyword
The default
keyword is used to specify code that should be run if no case is found. In our example above, we defined a program that returns the name of a month, but only if it’s between January and June.
But what if we want our user to be presented with a default message stating, It’s after June
if no case evaluates to true? That’s where the default
keyword comes in.
Here’s an example of the default
keyword being used with the above example to specify a message that will appear if no cases evaluate to true:
case 4: System.out.println("April"); break; case 5: System.out.println("May"); break; case 6: System.out.println("June"); break; default: System.out.println("It's after June!") break; …
If it were July and we were to execute our program, the contents of the default
statement would be executed because no case would evaluate to true. Then, the message It’s after June!
would be printed to the console.
Conclusion
The Java switch statement is used to evaluate a statement against multiple cases and execute code if a particular case is met. Switch statements are a form of conditional statement used to control the flow of a program.
This tutorial discussed how to use a switch
statement in Java, and explored how to use the case
, break
, and default
statements with the switch
method. Further, we walked through an example of a switch statement in action, which uses these keywords.
Now you’re ready to use switch statements in Java like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.